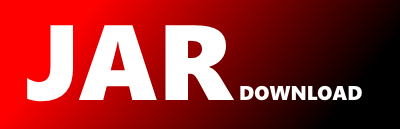
com.google.api.services.androiddeviceprovisioning.v1.model.Configuration Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androiddeviceprovisioning.v1.model;
/**
* A configuration collects the provisioning options for Android devices. Each configuration
* combines the following: * The EMM device policy controller (DPC) installed on the devices. * EMM
* policies enforced on the devices. * Metadata displayed on the device to help users during setup.
* Customers can add as many configurations as they need. However, zero-touch enrollment works best
* when a customer sets a default configuration that's applied to any new devices the organization
* purchases.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Device Provisioning Partner API. For a
* detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Configuration extends com.google.api.client.json.GenericJson {
/**
* Required. The name of the organization. Zero-touch enrollment shows this organization name to
* device users during device provisioning.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String companyName;
/**
* Output only. The ID of the configuration. Assigned by the server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long configurationId;
/**
* Required. A short name that describes the configuration's purpose. For example, _Sales team_ or
* _Temporary employees_. The zero-touch enrollment portal displays this name to IT admins.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String configurationName;
/**
* Required. The email address that device users can contact to get help. Zero-touch enrollment
* shows this email address to device users before device provisioning. The value is validated on
* input.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contactEmail;
/**
* Required. The telephone number that device users can call, using another device, to get help.
* Zero-touch enrollment shows this number to device users before device provisioning. Accepts
* numerals, spaces, the plus sign, hyphens, and parentheses.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contactPhone;
/**
* A message, containing one or two sentences, to help device users get help or give them more
* details about what’s happening to their device. Zero-touch enrollment shows this message before
* the device is provisioned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String customMessage;
/**
* The JSON-formatted EMM provisioning extras that are passed to the DPC.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dpcExtras;
/**
* Required. The resource name of the selected DPC (device policy controller) in the format
* `customers/[CUSTOMER_ID]/dpcs`. To list the supported DPCs, call `customers.dpcs.list`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dpcResourcePath;
/**
* Optional. The timeout before forcing factory reset the device if the device doesn't go through
* provisioning in the setup wizard, usually due to lack of network connectivity during setup
* wizard. Ranges from 0-6 hours, with 2 hours being the default if unset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String forcedResetTime;
/**
* Required. Whether this is the default configuration that zero-touch enrollment applies to any
* new devices the organization purchases in the future. Only one customer configuration can be
* the default. Setting this value to `true`, changes the previous default configuration's
* `isDefault` value to `false`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isDefault;
/**
* Output only. The API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. Assigned by the server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Required. The name of the organization. Zero-touch enrollment shows this organization name to
* device users during device provisioning.
* @return value or {@code null} for none
*/
public java.lang.String getCompanyName() {
return companyName;
}
/**
* Required. The name of the organization. Zero-touch enrollment shows this organization name to
* device users during device provisioning.
* @param companyName companyName or {@code null} for none
*/
public Configuration setCompanyName(java.lang.String companyName) {
this.companyName = companyName;
return this;
}
/**
* Output only. The ID of the configuration. Assigned by the server.
* @return value or {@code null} for none
*/
public java.lang.Long getConfigurationId() {
return configurationId;
}
/**
* Output only. The ID of the configuration. Assigned by the server.
* @param configurationId configurationId or {@code null} for none
*/
public Configuration setConfigurationId(java.lang.Long configurationId) {
this.configurationId = configurationId;
return this;
}
/**
* Required. A short name that describes the configuration's purpose. For example, _Sales team_ or
* _Temporary employees_. The zero-touch enrollment portal displays this name to IT admins.
* @return value or {@code null} for none
*/
public java.lang.String getConfigurationName() {
return configurationName;
}
/**
* Required. A short name that describes the configuration's purpose. For example, _Sales team_ or
* _Temporary employees_. The zero-touch enrollment portal displays this name to IT admins.
* @param configurationName configurationName or {@code null} for none
*/
public Configuration setConfigurationName(java.lang.String configurationName) {
this.configurationName = configurationName;
return this;
}
/**
* Required. The email address that device users can contact to get help. Zero-touch enrollment
* shows this email address to device users before device provisioning. The value is validated on
* input.
* @return value or {@code null} for none
*/
public java.lang.String getContactEmail() {
return contactEmail;
}
/**
* Required. The email address that device users can contact to get help. Zero-touch enrollment
* shows this email address to device users before device provisioning. The value is validated on
* input.
* @param contactEmail contactEmail or {@code null} for none
*/
public Configuration setContactEmail(java.lang.String contactEmail) {
this.contactEmail = contactEmail;
return this;
}
/**
* Required. The telephone number that device users can call, using another device, to get help.
* Zero-touch enrollment shows this number to device users before device provisioning. Accepts
* numerals, spaces, the plus sign, hyphens, and parentheses.
* @return value or {@code null} for none
*/
public java.lang.String getContactPhone() {
return contactPhone;
}
/**
* Required. The telephone number that device users can call, using another device, to get help.
* Zero-touch enrollment shows this number to device users before device provisioning. Accepts
* numerals, spaces, the plus sign, hyphens, and parentheses.
* @param contactPhone contactPhone or {@code null} for none
*/
public Configuration setContactPhone(java.lang.String contactPhone) {
this.contactPhone = contactPhone;
return this;
}
/**
* A message, containing one or two sentences, to help device users get help or give them more
* details about what’s happening to their device. Zero-touch enrollment shows this message before
* the device is provisioned.
* @return value or {@code null} for none
*/
public java.lang.String getCustomMessage() {
return customMessage;
}
/**
* A message, containing one or two sentences, to help device users get help or give them more
* details about what’s happening to their device. Zero-touch enrollment shows this message before
* the device is provisioned.
* @param customMessage customMessage or {@code null} for none
*/
public Configuration setCustomMessage(java.lang.String customMessage) {
this.customMessage = customMessage;
return this;
}
/**
* The JSON-formatted EMM provisioning extras that are passed to the DPC.
* @return value or {@code null} for none
*/
public java.lang.String getDpcExtras() {
return dpcExtras;
}
/**
* The JSON-formatted EMM provisioning extras that are passed to the DPC.
* @param dpcExtras dpcExtras or {@code null} for none
*/
public Configuration setDpcExtras(java.lang.String dpcExtras) {
this.dpcExtras = dpcExtras;
return this;
}
/**
* Required. The resource name of the selected DPC (device policy controller) in the format
* `customers/[CUSTOMER_ID]/dpcs`. To list the supported DPCs, call `customers.dpcs.list`.
* @return value or {@code null} for none
*/
public java.lang.String getDpcResourcePath() {
return dpcResourcePath;
}
/**
* Required. The resource name of the selected DPC (device policy controller) in the format
* `customers/[CUSTOMER_ID]/dpcs`. To list the supported DPCs, call `customers.dpcs.list`.
* @param dpcResourcePath dpcResourcePath or {@code null} for none
*/
public Configuration setDpcResourcePath(java.lang.String dpcResourcePath) {
this.dpcResourcePath = dpcResourcePath;
return this;
}
/**
* Optional. The timeout before forcing factory reset the device if the device doesn't go through
* provisioning in the setup wizard, usually due to lack of network connectivity during setup
* wizard. Ranges from 0-6 hours, with 2 hours being the default if unset.
* @return value or {@code null} for none
*/
public String getForcedResetTime() {
return forcedResetTime;
}
/**
* Optional. The timeout before forcing factory reset the device if the device doesn't go through
* provisioning in the setup wizard, usually due to lack of network connectivity during setup
* wizard. Ranges from 0-6 hours, with 2 hours being the default if unset.
* @param forcedResetTime forcedResetTime or {@code null} for none
*/
public Configuration setForcedResetTime(String forcedResetTime) {
this.forcedResetTime = forcedResetTime;
return this;
}
/**
* Required. Whether this is the default configuration that zero-touch enrollment applies to any
* new devices the organization purchases in the future. Only one customer configuration can be
* the default. Setting this value to `true`, changes the previous default configuration's
* `isDefault` value to `false`.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsDefault() {
return isDefault;
}
/**
* Required. Whether this is the default configuration that zero-touch enrollment applies to any
* new devices the organization purchases in the future. Only one customer configuration can be
* the default. Setting this value to `true`, changes the previous default configuration's
* `isDefault` value to `false`.
* @param isDefault isDefault or {@code null} for none
*/
public Configuration setIsDefault(java.lang.Boolean isDefault) {
this.isDefault = isDefault;
return this;
}
/**
* Output only. The API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. Assigned by the server.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The API resource name in the format
* `customers/[CUSTOMER_ID]/configurations/[CONFIGURATION_ID]`. Assigned by the server.
* @param name name or {@code null} for none
*/
public Configuration setName(java.lang.String name) {
this.name = name;
return this;
}
@Override
public Configuration set(String fieldName, Object value) {
return (Configuration) super.set(fieldName, value);
}
@Override
public Configuration clone() {
return (Configuration) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy