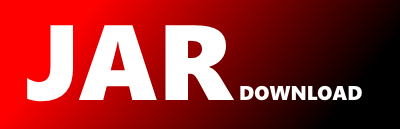
com.google.api.services.androiddeviceprovisioning.v1.model.DeviceIdentifier Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androiddeviceprovisioning.v1.model;
/**
* Encapsulates hardware and product IDs to identify a manufactured device. To understand
* requirements on identifier sets, read [Identifiers](https://developers.google.com/zero-
* touch/guides/identifiers).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Device Provisioning Partner API. For a
* detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DeviceIdentifier extends com.google.api.client.json.GenericJson {
/**
* An identifier provided by OEMs, carried through the production and sales process. Only
* applicable to Chrome OS devices.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String chromeOsAttestedDeviceId;
/**
* The type of the device
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String deviceType;
/**
* The device’s IMEI number. Validated on input.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imei;
/**
* The device’s second IMEI number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imei2;
/**
* The device manufacturer’s name. Matches the device's built-in value returned from
* `android.os.Build.MANUFACTURER`. Allowed values are listed in [Android manufacturers](/zero-
* touch/resources/manufacturer-names#manufacturers-names).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String manufacturer;
/**
* The device’s MEID number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String meid;
/**
* The device’s second MEID number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String meid2;
/**
* The device model's name. Allowed values are listed in [Android models](/zero-
* touch/resources/manufacturer-names#model-names) and [Chrome OS
* models](https://support.google.com/chrome/a/answer/10130175#identify_compatible).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String model;
/**
* The manufacturer's serial number for the device. This value might not be unique across
* different device models.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serialNumber;
/**
* An identifier provided by OEMs, carried through the production and sales process. Only
* applicable to Chrome OS devices.
* @return value or {@code null} for none
*/
public java.lang.String getChromeOsAttestedDeviceId() {
return chromeOsAttestedDeviceId;
}
/**
* An identifier provided by OEMs, carried through the production and sales process. Only
* applicable to Chrome OS devices.
* @param chromeOsAttestedDeviceId chromeOsAttestedDeviceId or {@code null} for none
*/
public DeviceIdentifier setChromeOsAttestedDeviceId(java.lang.String chromeOsAttestedDeviceId) {
this.chromeOsAttestedDeviceId = chromeOsAttestedDeviceId;
return this;
}
/**
* The type of the device
* @return value or {@code null} for none
*/
public java.lang.String getDeviceType() {
return deviceType;
}
/**
* The type of the device
* @param deviceType deviceType or {@code null} for none
*/
public DeviceIdentifier setDeviceType(java.lang.String deviceType) {
this.deviceType = deviceType;
return this;
}
/**
* The device’s IMEI number. Validated on input.
* @return value or {@code null} for none
*/
public java.lang.String getImei() {
return imei;
}
/**
* The device’s IMEI number. Validated on input.
* @param imei imei or {@code null} for none
*/
public DeviceIdentifier setImei(java.lang.String imei) {
this.imei = imei;
return this;
}
/**
* The device’s second IMEI number.
* @return value or {@code null} for none
*/
public java.lang.String getImei2() {
return imei2;
}
/**
* The device’s second IMEI number.
* @param imei2 imei2 or {@code null} for none
*/
public DeviceIdentifier setImei2(java.lang.String imei2) {
this.imei2 = imei2;
return this;
}
/**
* The device manufacturer’s name. Matches the device's built-in value returned from
* `android.os.Build.MANUFACTURER`. Allowed values are listed in [Android manufacturers](/zero-
* touch/resources/manufacturer-names#manufacturers-names).
* @return value or {@code null} for none
*/
public java.lang.String getManufacturer() {
return manufacturer;
}
/**
* The device manufacturer’s name. Matches the device's built-in value returned from
* `android.os.Build.MANUFACTURER`. Allowed values are listed in [Android manufacturers](/zero-
* touch/resources/manufacturer-names#manufacturers-names).
* @param manufacturer manufacturer or {@code null} for none
*/
public DeviceIdentifier setManufacturer(java.lang.String manufacturer) {
this.manufacturer = manufacturer;
return this;
}
/**
* The device’s MEID number.
* @return value or {@code null} for none
*/
public java.lang.String getMeid() {
return meid;
}
/**
* The device’s MEID number.
* @param meid meid or {@code null} for none
*/
public DeviceIdentifier setMeid(java.lang.String meid) {
this.meid = meid;
return this;
}
/**
* The device’s second MEID number.
* @return value or {@code null} for none
*/
public java.lang.String getMeid2() {
return meid2;
}
/**
* The device’s second MEID number.
* @param meid2 meid2 or {@code null} for none
*/
public DeviceIdentifier setMeid2(java.lang.String meid2) {
this.meid2 = meid2;
return this;
}
/**
* The device model's name. Allowed values are listed in [Android models](/zero-
* touch/resources/manufacturer-names#model-names) and [Chrome OS
* models](https://support.google.com/chrome/a/answer/10130175#identify_compatible).
* @return value or {@code null} for none
*/
public java.lang.String getModel() {
return model;
}
/**
* The device model's name. Allowed values are listed in [Android models](/zero-
* touch/resources/manufacturer-names#model-names) and [Chrome OS
* models](https://support.google.com/chrome/a/answer/10130175#identify_compatible).
* @param model model or {@code null} for none
*/
public DeviceIdentifier setModel(java.lang.String model) {
this.model = model;
return this;
}
/**
* The manufacturer's serial number for the device. This value might not be unique across
* different device models.
* @return value or {@code null} for none
*/
public java.lang.String getSerialNumber() {
return serialNumber;
}
/**
* The manufacturer's serial number for the device. This value might not be unique across
* different device models.
* @param serialNumber serialNumber or {@code null} for none
*/
public DeviceIdentifier setSerialNumber(java.lang.String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
@Override
public DeviceIdentifier set(String fieldName, Object value) {
return (DeviceIdentifier) super.set(fieldName, value);
}
@Override
public DeviceIdentifier clone() {
return (DeviceIdentifier) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy