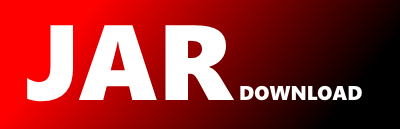
com.google.api.services.androiddeviceprovisioning.v1.model.DevicesLongRunningOperationMetadata Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androiddeviceprovisioning.v1.model;
/**
* Tracks the status of a long-running operation to asynchronously update a batch of reseller
* metadata attached to devices. To learn more, read [Long‑running batch operations](/zero-
* touch/guides/how-it-works#operations).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Device Provisioning Partner API. For a
* detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DevicesLongRunningOperationMetadata extends com.google.api.client.json.GenericJson {
/**
* The number of metadata updates in the operation. This might be different from the number of
* updates in the request if the API can't parse some of the updates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer devicesCount;
/**
* The processing status of the operation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String processingStatus;
/**
* The processing progress of the operation. Measured as a number from 0 to 100. A value of 10O
* doesn't always mean the operation completed—check for the inclusion of a `done` field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer progress;
/**
* The number of metadata updates in the operation. This might be different from the number of
* updates in the request if the API can't parse some of the updates.
* @return value or {@code null} for none
*/
public java.lang.Integer getDevicesCount() {
return devicesCount;
}
/**
* The number of metadata updates in the operation. This might be different from the number of
* updates in the request if the API can't parse some of the updates.
* @param devicesCount devicesCount or {@code null} for none
*/
public DevicesLongRunningOperationMetadata setDevicesCount(java.lang.Integer devicesCount) {
this.devicesCount = devicesCount;
return this;
}
/**
* The processing status of the operation.
* @return value or {@code null} for none
*/
public java.lang.String getProcessingStatus() {
return processingStatus;
}
/**
* The processing status of the operation.
* @param processingStatus processingStatus or {@code null} for none
*/
public DevicesLongRunningOperationMetadata setProcessingStatus(java.lang.String processingStatus) {
this.processingStatus = processingStatus;
return this;
}
/**
* The processing progress of the operation. Measured as a number from 0 to 100. A value of 10O
* doesn't always mean the operation completed—check for the inclusion of a `done` field.
* @return value or {@code null} for none
*/
public java.lang.Integer getProgress() {
return progress;
}
/**
* The processing progress of the operation. Measured as a number from 0 to 100. A value of 10O
* doesn't always mean the operation completed—check for the inclusion of a `done` field.
* @param progress progress or {@code null} for none
*/
public DevicesLongRunningOperationMetadata setProgress(java.lang.Integer progress) {
this.progress = progress;
return this;
}
@Override
public DevicesLongRunningOperationMetadata set(String fieldName, Object value) {
return (DevicesLongRunningOperationMetadata) super.set(fieldName, value);
}
@Override
public DevicesLongRunningOperationMetadata clone() {
return (DevicesLongRunningOperationMetadata) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy