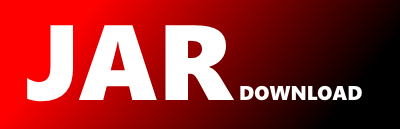
com.google.api.services.androiddeviceprovisioning.v1.model.FindDevicesByOwnerRequest Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androiddeviceprovisioning.v1.model;
/**
* Request to find devices by customers.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Device Provisioning Partner API. For a
* detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class FindDevicesByOwnerRequest extends com.google.api.client.json.GenericJson {
/**
* The list of customer IDs to search for.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.util.List customerId;
/**
* The list of IDs of Google Workspace accounts to search for.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List googleWorkspaceCustomerId;
/**
* Required. The maximum number of devices to show in a page of results. Must be between 1 and 100
* inclusive.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long limit;
/**
* A token specifying which result page to return.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/**
* Required. The section type of the device's provisioning record.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sectionType;
/**
* The list of customer IDs to search for.
* @return value or {@code null} for none
*/
public java.util.List getCustomerId() {
return customerId;
}
/**
* The list of customer IDs to search for.
* @param customerId customerId or {@code null} for none
*/
public FindDevicesByOwnerRequest setCustomerId(java.util.List customerId) {
this.customerId = customerId;
return this;
}
/**
* The list of IDs of Google Workspace accounts to search for.
* @return value or {@code null} for none
*/
public java.util.List getGoogleWorkspaceCustomerId() {
return googleWorkspaceCustomerId;
}
/**
* The list of IDs of Google Workspace accounts to search for.
* @param googleWorkspaceCustomerId googleWorkspaceCustomerId or {@code null} for none
*/
public FindDevicesByOwnerRequest setGoogleWorkspaceCustomerId(java.util.List googleWorkspaceCustomerId) {
this.googleWorkspaceCustomerId = googleWorkspaceCustomerId;
return this;
}
/**
* Required. The maximum number of devices to show in a page of results. Must be between 1 and 100
* inclusive.
* @return value or {@code null} for none
*/
public java.lang.Long getLimit() {
return limit;
}
/**
* Required. The maximum number of devices to show in a page of results. Must be between 1 and 100
* inclusive.
* @param limit limit or {@code null} for none
*/
public FindDevicesByOwnerRequest setLimit(java.lang.Long limit) {
this.limit = limit;
return this;
}
/**
* A token specifying which result page to return.
* @return value or {@code null} for none
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A token specifying which result page to return.
* @param pageToken pageToken or {@code null} for none
*/
public FindDevicesByOwnerRequest setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. The section type of the device's provisioning record.
* @return value or {@code null} for none
*/
public java.lang.String getSectionType() {
return sectionType;
}
/**
* Required. The section type of the device's provisioning record.
* @param sectionType sectionType or {@code null} for none
*/
public FindDevicesByOwnerRequest setSectionType(java.lang.String sectionType) {
this.sectionType = sectionType;
return this;
}
@Override
public FindDevicesByOwnerRequest set(String fieldName, Object value) {
return (FindDevicesByOwnerRequest) super.set(fieldName, value);
}
@Override
public FindDevicesByOwnerRequest clone() {
return (FindDevicesByOwnerRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy