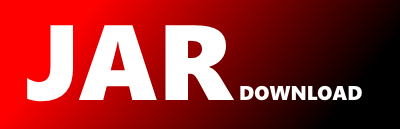
com.google.api.services.androiddeviceprovisioning.v1.model.PartnerClaim Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androiddeviceprovisioning.v1.model;
/**
* Identifies one claim request.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Device Provisioning Partner API. For a
* detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PartnerClaim extends com.google.api.client.json.GenericJson {
/**
* Optional. The ID of the configuration applied to the device section.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long configurationId;
/**
* The ID of the customer for whom the device is being claimed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long customerId;
/**
* Required. Required. Device identifier of the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceIdentifier deviceIdentifier;
/**
* Required. The metadata to attach to the device at claim.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceMetadata deviceMetadata;
/**
* The Google Workspace customer ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String googleWorkspaceCustomerId;
/**
* Optional. Must and can only be set for Chrome OS devices.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String preProvisioningToken;
/**
* Required. The section type of the device's provisioning record.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sectionType;
/**
* Optional. Must and can only be set when DeviceProvisioningSectionType is SECTION_TYPE_SIM_LOCK.
* The unique identifier of the SimLock profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long simlockProfileId;
/**
* Optional. The ID of the configuration applied to the device section.
* @return value or {@code null} for none
*/
public java.lang.Long getConfigurationId() {
return configurationId;
}
/**
* Optional. The ID of the configuration applied to the device section.
* @param configurationId configurationId or {@code null} for none
*/
public PartnerClaim setConfigurationId(java.lang.Long configurationId) {
this.configurationId = configurationId;
return this;
}
/**
* The ID of the customer for whom the device is being claimed.
* @return value or {@code null} for none
*/
public java.lang.Long getCustomerId() {
return customerId;
}
/**
* The ID of the customer for whom the device is being claimed.
* @param customerId customerId or {@code null} for none
*/
public PartnerClaim setCustomerId(java.lang.Long customerId) {
this.customerId = customerId;
return this;
}
/**
* Required. Required. Device identifier of the device.
* @return value or {@code null} for none
*/
public DeviceIdentifier getDeviceIdentifier() {
return deviceIdentifier;
}
/**
* Required. Required. Device identifier of the device.
* @param deviceIdentifier deviceIdentifier or {@code null} for none
*/
public PartnerClaim setDeviceIdentifier(DeviceIdentifier deviceIdentifier) {
this.deviceIdentifier = deviceIdentifier;
return this;
}
/**
* Required. The metadata to attach to the device at claim.
* @return value or {@code null} for none
*/
public DeviceMetadata getDeviceMetadata() {
return deviceMetadata;
}
/**
* Required. The metadata to attach to the device at claim.
* @param deviceMetadata deviceMetadata or {@code null} for none
*/
public PartnerClaim setDeviceMetadata(DeviceMetadata deviceMetadata) {
this.deviceMetadata = deviceMetadata;
return this;
}
/**
* The Google Workspace customer ID.
* @return value or {@code null} for none
*/
public java.lang.String getGoogleWorkspaceCustomerId() {
return googleWorkspaceCustomerId;
}
/**
* The Google Workspace customer ID.
* @param googleWorkspaceCustomerId googleWorkspaceCustomerId or {@code null} for none
*/
public PartnerClaim setGoogleWorkspaceCustomerId(java.lang.String googleWorkspaceCustomerId) {
this.googleWorkspaceCustomerId = googleWorkspaceCustomerId;
return this;
}
/**
* Optional. Must and can only be set for Chrome OS devices.
* @return value or {@code null} for none
*/
public java.lang.String getPreProvisioningToken() {
return preProvisioningToken;
}
/**
* Optional. Must and can only be set for Chrome OS devices.
* @param preProvisioningToken preProvisioningToken or {@code null} for none
*/
public PartnerClaim setPreProvisioningToken(java.lang.String preProvisioningToken) {
this.preProvisioningToken = preProvisioningToken;
return this;
}
/**
* Required. The section type of the device's provisioning record.
* @return value or {@code null} for none
*/
public java.lang.String getSectionType() {
return sectionType;
}
/**
* Required. The section type of the device's provisioning record.
* @param sectionType sectionType or {@code null} for none
*/
public PartnerClaim setSectionType(java.lang.String sectionType) {
this.sectionType = sectionType;
return this;
}
/**
* Optional. Must and can only be set when DeviceProvisioningSectionType is SECTION_TYPE_SIM_LOCK.
* The unique identifier of the SimLock profile.
* @return value or {@code null} for none
*/
public java.lang.Long getSimlockProfileId() {
return simlockProfileId;
}
/**
* Optional. Must and can only be set when DeviceProvisioningSectionType is SECTION_TYPE_SIM_LOCK.
* The unique identifier of the SimLock profile.
* @param simlockProfileId simlockProfileId or {@code null} for none
*/
public PartnerClaim setSimlockProfileId(java.lang.Long simlockProfileId) {
this.simlockProfileId = simlockProfileId;
return this;
}
@Override
public PartnerClaim set(String fieldName, Object value) {
return (PartnerClaim) super.set(fieldName, value);
}
@Override
public PartnerClaim clone() {
return (PartnerClaim) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy