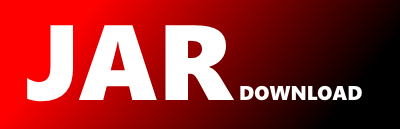
com.google.api.services.androidenterprise.AndroidEnterprise Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidenterprise;
/**
* Service definition for AndroidEnterprise (v1).
*
*
* Manages the deployment of apps to Android Enterprise devices.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AndroidEnterpriseRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class AndroidEnterprise extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Google Play EMM API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://androidenterprise.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://androidenterprise.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public AndroidEnterprise(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
AndroidEnterprise(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Devices collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Devices.List request = androidenterprise.devices().list(parameters ...)}
*
*
* @return the resource collection
*/
public Devices devices() {
return new Devices();
}
/**
* The "devices" collection of methods.
*/
public class Devices {
/**
* Uploads a report containing any changes in app states on the device since the last report was
* generated. You can call this method up to 3 times every 24 hours for a given device. If you
* exceed the quota, then the Google Play EMM API returns HTTP 429 Too Many Requests.
*
* Create a request for the method "devices.forceReportUpload".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link ForceReportUpload#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @return the request
*/
public ForceReportUpload forceReportUpload(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) throws java.io.IOException {
ForceReportUpload result = new ForceReportUpload(enterpriseId, userId, deviceId);
initialize(result);
return result;
}
public class ForceReportUpload extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/forceReportUpload";
/**
* Uploads a report containing any changes in app states on the device since the last report was
* generated. You can call this method up to 3 times every 24 hours for a given device. If you
* exceed the quota, then the Google Play EMM API returns HTTP 429 Too Many Requests.
*
* Create a request for the method "devices.forceReportUpload".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link ForceReportUpload#execute()} method to invoke the
* remote operation. {@link ForceReportUpload#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @since 1.13
*/
protected ForceReportUpload(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) {
super(AndroidEnterprise.this, "POST", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
}
@Override
public ForceReportUpload set$Xgafv(java.lang.String $Xgafv) {
return (ForceReportUpload) super.set$Xgafv($Xgafv);
}
@Override
public ForceReportUpload setAccessToken(java.lang.String accessToken) {
return (ForceReportUpload) super.setAccessToken(accessToken);
}
@Override
public ForceReportUpload setAlt(java.lang.String alt) {
return (ForceReportUpload) super.setAlt(alt);
}
@Override
public ForceReportUpload setCallback(java.lang.String callback) {
return (ForceReportUpload) super.setCallback(callback);
}
@Override
public ForceReportUpload setFields(java.lang.String fields) {
return (ForceReportUpload) super.setFields(fields);
}
@Override
public ForceReportUpload setKey(java.lang.String key) {
return (ForceReportUpload) super.setKey(key);
}
@Override
public ForceReportUpload setOauthToken(java.lang.String oauthToken) {
return (ForceReportUpload) super.setOauthToken(oauthToken);
}
@Override
public ForceReportUpload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ForceReportUpload) super.setPrettyPrint(prettyPrint);
}
@Override
public ForceReportUpload setQuotaUser(java.lang.String quotaUser) {
return (ForceReportUpload) super.setQuotaUser(quotaUser);
}
@Override
public ForceReportUpload setUploadType(java.lang.String uploadType) {
return (ForceReportUpload) super.setUploadType(uploadType);
}
@Override
public ForceReportUpload setUploadProtocol(java.lang.String uploadProtocol) {
return (ForceReportUpload) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public ForceReportUpload setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public ForceReportUpload setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The ID of the device. */
public ForceReportUpload setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
@Override
public ForceReportUpload set(String parameterName, Object value) {
return (ForceReportUpload) super.set(parameterName, value);
}
}
/**
* Retrieves the details of a device.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) throws java.io.IOException {
Get result = new Get(enterpriseId, userId, deviceId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}";
/**
* Retrieves the details of a device.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.Device.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The ID of the device. */
public Get setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves whether a device's access to Google services is enabled or disabled. The device state
* takes effect only if enforcing EMM policies on Android devices is enabled in the Google Admin
* Console. Otherwise, the device state is ignored and all devices are allowed access to Google
* services. This is only supported for Google-managed users.
*
* Create a request for the method "devices.getState".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GetState#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @return the request
*/
public GetState getState(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) throws java.io.IOException {
GetState result = new GetState(enterpriseId, userId, deviceId);
initialize(result);
return result;
}
public class GetState extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/state";
/**
* Retrieves whether a device's access to Google services is enabled or disabled. The device state
* takes effect only if enforcing EMM policies on Android devices is enabled in the Google Admin
* Console. Otherwise, the device state is ignored and all devices are allowed access to Google
* services. This is only supported for Google-managed users.
*
* Create a request for the method "devices.getState".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GetState#execute()} method to invoke the remote
* operation. {@link
* GetState#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @since 1.13
*/
protected GetState(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.DeviceState.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetState set$Xgafv(java.lang.String $Xgafv) {
return (GetState) super.set$Xgafv($Xgafv);
}
@Override
public GetState setAccessToken(java.lang.String accessToken) {
return (GetState) super.setAccessToken(accessToken);
}
@Override
public GetState setAlt(java.lang.String alt) {
return (GetState) super.setAlt(alt);
}
@Override
public GetState setCallback(java.lang.String callback) {
return (GetState) super.setCallback(callback);
}
@Override
public GetState setFields(java.lang.String fields) {
return (GetState) super.setFields(fields);
}
@Override
public GetState setKey(java.lang.String key) {
return (GetState) super.setKey(key);
}
@Override
public GetState setOauthToken(java.lang.String oauthToken) {
return (GetState) super.setOauthToken(oauthToken);
}
@Override
public GetState setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetState) super.setPrettyPrint(prettyPrint);
}
@Override
public GetState setQuotaUser(java.lang.String quotaUser) {
return (GetState) super.setQuotaUser(quotaUser);
}
@Override
public GetState setUploadType(java.lang.String uploadType) {
return (GetState) super.setUploadType(uploadType);
}
@Override
public GetState setUploadProtocol(java.lang.String uploadProtocol) {
return (GetState) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public GetState setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public GetState setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The ID of the device. */
public GetState setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
@Override
public GetState set(String parameterName, Object value) {
return (GetState) super.set(parameterName, value);
}
}
/**
* Retrieves the IDs of all of a user's devices.
*
* Create a request for the method "devices.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String userId) throws java.io.IOException {
List result = new List(enterpriseId, userId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices";
/**
* Retrieves the IDs of all of a user's devices.
*
* Create a request for the method "devices.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String userId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.DevicesListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets whether a device's access to Google services is enabled or disabled. The device state takes
* effect only if enforcing EMM policies on Android devices is enabled in the Google Admin Console.
* Otherwise, the device state is ignored and all devices are allowed access to Google services.
* This is only supported for Google-managed users.
*
* Create a request for the method "devices.setState".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link SetState#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @param content the {@link com.google.api.services.androidenterprise.model.DeviceState}
* @return the request
*/
public SetState setState(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, com.google.api.services.androidenterprise.model.DeviceState content) throws java.io.IOException {
SetState result = new SetState(enterpriseId, userId, deviceId, content);
initialize(result);
return result;
}
public class SetState extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/state";
/**
* Sets whether a device's access to Google services is enabled or disabled. The device state
* takes effect only if enforcing EMM policies on Android devices is enabled in the Google Admin
* Console. Otherwise, the device state is ignored and all devices are allowed access to Google
* services. This is only supported for Google-managed users.
*
* Create a request for the method "devices.setState".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link SetState#execute()} method to invoke the remote
* operation. {@link
* SetState#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @param content the {@link com.google.api.services.androidenterprise.model.DeviceState}
* @since 1.13
*/
protected SetState(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, com.google.api.services.androidenterprise.model.DeviceState content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.DeviceState.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
}
@Override
public SetState set$Xgafv(java.lang.String $Xgafv) {
return (SetState) super.set$Xgafv($Xgafv);
}
@Override
public SetState setAccessToken(java.lang.String accessToken) {
return (SetState) super.setAccessToken(accessToken);
}
@Override
public SetState setAlt(java.lang.String alt) {
return (SetState) super.setAlt(alt);
}
@Override
public SetState setCallback(java.lang.String callback) {
return (SetState) super.setCallback(callback);
}
@Override
public SetState setFields(java.lang.String fields) {
return (SetState) super.setFields(fields);
}
@Override
public SetState setKey(java.lang.String key) {
return (SetState) super.setKey(key);
}
@Override
public SetState setOauthToken(java.lang.String oauthToken) {
return (SetState) super.setOauthToken(oauthToken);
}
@Override
public SetState setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetState) super.setPrettyPrint(prettyPrint);
}
@Override
public SetState setQuotaUser(java.lang.String quotaUser) {
return (SetState) super.setQuotaUser(quotaUser);
}
@Override
public SetState setUploadType(java.lang.String uploadType) {
return (SetState) super.setUploadType(uploadType);
}
@Override
public SetState setUploadProtocol(java.lang.String uploadProtocol) {
return (SetState) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public SetState setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public SetState setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The ID of the device. */
public SetState setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
@Override
public SetState set(String parameterName, Object value) {
return (SetState) super.set(parameterName, value);
}
}
/**
* Updates the device policy. To ensure the policy is properly enforced, you need to prevent
* unmanaged accounts from accessing Google Play by setting the allowed_accounts in the managed
* configuration for the Google Play package. See restrict accounts in Google Play. When
* provisioning a new device, you should set the device policy using this method before adding the
* managed Google Play Account to the device, otherwise the policy will not be applied for a short
* period of time after adding the account to the device.
*
* Create a request for the method "devices.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @param content the {@link com.google.api.services.androidenterprise.model.Device}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, com.google.api.services.androidenterprise.model.Device content) throws java.io.IOException {
Update result = new Update(enterpriseId, userId, deviceId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}";
/**
* Updates the device policy. To ensure the policy is properly enforced, you need to prevent
* unmanaged accounts from accessing Google Play by setting the allowed_accounts in the managed
* configuration for the Google Play package. See restrict accounts in Google Play. When
* provisioning a new device, you should set the device policy using this method before adding the
* managed Google Play Account to the device, otherwise the policy will not be applied for a short
* period of time after adding the account to the device.
*
* Create a request for the method "devices.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The ID of the device.
* @param content the {@link com.google.api.services.androidenterprise.model.Device}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, com.google.api.services.androidenterprise.model.Device content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.Device.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Update setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The ID of the device. */
public Update setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/**
* Mask that identifies which fields to update. If not set, all modifiable fields will be
* modified. When set in a query parameter, this field should be specified as updateMask=,,...
*/
@com.google.api.client.util.Key
private java.lang.String updateMask;
/** Mask that identifies which fields to update. If not set, all modifiable fields will be modified.
When set in a query parameter, this field should be specified as updateMask=,,...
*/
public java.lang.String getUpdateMask() {
return updateMask;
}
/**
* Mask that identifies which fields to update. If not set, all modifiable fields will be
* modified. When set in a query parameter, this field should be specified as updateMask=,,...
*/
public Update setUpdateMask(java.lang.String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Enterprises collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Enterprises.List request = androidenterprise.enterprises().list(parameters ...)}
*
*
* @return the resource collection
*/
public Enterprises enterprises() {
return new Enterprises();
}
/**
* The "enterprises" collection of methods.
*/
public class Enterprises {
/**
* Acknowledges notifications that were received from Enterprises.PullNotificationSet to prevent
* subsequent calls from returning the same notifications.
*
* Create a request for the method "enterprises.acknowledgeNotificationSet".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link AcknowledgeNotificationSet#execute()} method to invoke the
* remote operation.
*
* @return the request
*/
public AcknowledgeNotificationSet acknowledgeNotificationSet() throws java.io.IOException {
AcknowledgeNotificationSet result = new AcknowledgeNotificationSet();
initialize(result);
return result;
}
public class AcknowledgeNotificationSet extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/acknowledgeNotificationSet";
/**
* Acknowledges notifications that were received from Enterprises.PullNotificationSet to prevent
* subsequent calls from returning the same notifications.
*
* Create a request for the method "enterprises.acknowledgeNotificationSet".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link AcknowledgeNotificationSet#execute()} method to invoke
* the remote operation. {@link AcknowledgeNotificationSet#initialize(com.google.api.client.go
* ogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @since 1.13
*/
protected AcknowledgeNotificationSet() {
super(AndroidEnterprise.this, "POST", REST_PATH, null, Void.class);
}
@Override
public AcknowledgeNotificationSet set$Xgafv(java.lang.String $Xgafv) {
return (AcknowledgeNotificationSet) super.set$Xgafv($Xgafv);
}
@Override
public AcknowledgeNotificationSet setAccessToken(java.lang.String accessToken) {
return (AcknowledgeNotificationSet) super.setAccessToken(accessToken);
}
@Override
public AcknowledgeNotificationSet setAlt(java.lang.String alt) {
return (AcknowledgeNotificationSet) super.setAlt(alt);
}
@Override
public AcknowledgeNotificationSet setCallback(java.lang.String callback) {
return (AcknowledgeNotificationSet) super.setCallback(callback);
}
@Override
public AcknowledgeNotificationSet setFields(java.lang.String fields) {
return (AcknowledgeNotificationSet) super.setFields(fields);
}
@Override
public AcknowledgeNotificationSet setKey(java.lang.String key) {
return (AcknowledgeNotificationSet) super.setKey(key);
}
@Override
public AcknowledgeNotificationSet setOauthToken(java.lang.String oauthToken) {
return (AcknowledgeNotificationSet) super.setOauthToken(oauthToken);
}
@Override
public AcknowledgeNotificationSet setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AcknowledgeNotificationSet) super.setPrettyPrint(prettyPrint);
}
@Override
public AcknowledgeNotificationSet setQuotaUser(java.lang.String quotaUser) {
return (AcknowledgeNotificationSet) super.setQuotaUser(quotaUser);
}
@Override
public AcknowledgeNotificationSet setUploadType(java.lang.String uploadType) {
return (AcknowledgeNotificationSet) super.setUploadType(uploadType);
}
@Override
public AcknowledgeNotificationSet setUploadProtocol(java.lang.String uploadProtocol) {
return (AcknowledgeNotificationSet) super.setUploadProtocol(uploadProtocol);
}
/**
* The notification set ID as returned by Enterprises.PullNotificationSet. This must be
* provided.
*/
@com.google.api.client.util.Key
private java.lang.String notificationSetId;
/** The notification set ID as returned by Enterprises.PullNotificationSet. This must be provided.
*/
public java.lang.String getNotificationSetId() {
return notificationSetId;
}
/**
* The notification set ID as returned by Enterprises.PullNotificationSet. This must be
* provided.
*/
public AcknowledgeNotificationSet setNotificationSetId(java.lang.String notificationSetId) {
this.notificationSetId = notificationSetId;
return this;
}
@Override
public AcknowledgeNotificationSet set(String parameterName, Object value) {
return (AcknowledgeNotificationSet) super.set(parameterName, value);
}
}
/**
* Completes the signup flow, by specifying the Completion token and Enterprise token. This request
* must not be called multiple times for a given Enterprise Token.
*
* Create a request for the method "enterprises.completeSignup".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link CompleteSignup#execute()} method to invoke the remote
* operation.
*
* @return the request
*/
public CompleteSignup completeSignup() throws java.io.IOException {
CompleteSignup result = new CompleteSignup();
initialize(result);
return result;
}
public class CompleteSignup extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/completeSignup";
/**
* Completes the signup flow, by specifying the Completion token and Enterprise token. This
* request must not be called multiple times for a given Enterprise Token.
*
* Create a request for the method "enterprises.completeSignup".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link CompleteSignup#execute()} method to invoke the remote
* operation. {@link CompleteSignup#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @since 1.13
*/
protected CompleteSignup() {
super(AndroidEnterprise.this, "POST", REST_PATH, null, com.google.api.services.androidenterprise.model.Enterprise.class);
}
@Override
public CompleteSignup set$Xgafv(java.lang.String $Xgafv) {
return (CompleteSignup) super.set$Xgafv($Xgafv);
}
@Override
public CompleteSignup setAccessToken(java.lang.String accessToken) {
return (CompleteSignup) super.setAccessToken(accessToken);
}
@Override
public CompleteSignup setAlt(java.lang.String alt) {
return (CompleteSignup) super.setAlt(alt);
}
@Override
public CompleteSignup setCallback(java.lang.String callback) {
return (CompleteSignup) super.setCallback(callback);
}
@Override
public CompleteSignup setFields(java.lang.String fields) {
return (CompleteSignup) super.setFields(fields);
}
@Override
public CompleteSignup setKey(java.lang.String key) {
return (CompleteSignup) super.setKey(key);
}
@Override
public CompleteSignup setOauthToken(java.lang.String oauthToken) {
return (CompleteSignup) super.setOauthToken(oauthToken);
}
@Override
public CompleteSignup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CompleteSignup) super.setPrettyPrint(prettyPrint);
}
@Override
public CompleteSignup setQuotaUser(java.lang.String quotaUser) {
return (CompleteSignup) super.setQuotaUser(quotaUser);
}
@Override
public CompleteSignup setUploadType(java.lang.String uploadType) {
return (CompleteSignup) super.setUploadType(uploadType);
}
@Override
public CompleteSignup setUploadProtocol(java.lang.String uploadProtocol) {
return (CompleteSignup) super.setUploadProtocol(uploadProtocol);
}
/** The Completion token initially returned by GenerateSignupUrl. */
@com.google.api.client.util.Key
private java.lang.String completionToken;
/** The Completion token initially returned by GenerateSignupUrl.
*/
public java.lang.String getCompletionToken() {
return completionToken;
}
/** The Completion token initially returned by GenerateSignupUrl. */
public CompleteSignup setCompletionToken(java.lang.String completionToken) {
this.completionToken = completionToken;
return this;
}
/** The Enterprise token appended to the Callback URL. */
@com.google.api.client.util.Key
private java.lang.String enterpriseToken;
/** The Enterprise token appended to the Callback URL.
*/
public java.lang.String getEnterpriseToken() {
return enterpriseToken;
}
/** The Enterprise token appended to the Callback URL. */
public CompleteSignup setEnterpriseToken(java.lang.String enterpriseToken) {
this.enterpriseToken = enterpriseToken;
return this;
}
@Override
public CompleteSignup set(String parameterName, Object value) {
return (CompleteSignup) super.set(parameterName, value);
}
}
/**
* Returns a token for device enrollment. The DPC can encode this token within the QR/NFC/zero-touch
* enrollment payload or fetch it before calling the on-device API to authenticate the user. The
* token can be generated for each device or reused across multiple devices.
*
* Create a request for the method "enterprises.createEnrollmentToken".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link CreateEnrollmentToken#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId Required. The ID of the enterprise.
* @return the request
*/
public CreateEnrollmentToken createEnrollmentToken(java.lang.String enterpriseId) throws java.io.IOException {
CreateEnrollmentToken result = new CreateEnrollmentToken(enterpriseId);
initialize(result);
return result;
}
public class CreateEnrollmentToken extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/createEnrollmentToken";
/**
* Returns a token for device enrollment. The DPC can encode this token within the QR/NFC/zero-
* touch enrollment payload or fetch it before calling the on-device API to authenticate the user.
* The token can be generated for each device or reused across multiple devices.
*
* Create a request for the method "enterprises.createEnrollmentToken".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link CreateEnrollmentToken#execute()} method to invoke the
* remote operation. {@link CreateEnrollmentToken#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param enterpriseId Required. The ID of the enterprise.
* @since 1.13
*/
protected CreateEnrollmentToken(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "POST", REST_PATH, null, com.google.api.services.androidenterprise.model.CreateEnrollmentTokenResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public CreateEnrollmentToken set$Xgafv(java.lang.String $Xgafv) {
return (CreateEnrollmentToken) super.set$Xgafv($Xgafv);
}
@Override
public CreateEnrollmentToken setAccessToken(java.lang.String accessToken) {
return (CreateEnrollmentToken) super.setAccessToken(accessToken);
}
@Override
public CreateEnrollmentToken setAlt(java.lang.String alt) {
return (CreateEnrollmentToken) super.setAlt(alt);
}
@Override
public CreateEnrollmentToken setCallback(java.lang.String callback) {
return (CreateEnrollmentToken) super.setCallback(callback);
}
@Override
public CreateEnrollmentToken setFields(java.lang.String fields) {
return (CreateEnrollmentToken) super.setFields(fields);
}
@Override
public CreateEnrollmentToken setKey(java.lang.String key) {
return (CreateEnrollmentToken) super.setKey(key);
}
@Override
public CreateEnrollmentToken setOauthToken(java.lang.String oauthToken) {
return (CreateEnrollmentToken) super.setOauthToken(oauthToken);
}
@Override
public CreateEnrollmentToken setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateEnrollmentToken) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateEnrollmentToken setQuotaUser(java.lang.String quotaUser) {
return (CreateEnrollmentToken) super.setQuotaUser(quotaUser);
}
@Override
public CreateEnrollmentToken setUploadType(java.lang.String uploadType) {
return (CreateEnrollmentToken) super.setUploadType(uploadType);
}
@Override
public CreateEnrollmentToken setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateEnrollmentToken) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** Required. The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** Required. The ID of the enterprise. */
public CreateEnrollmentToken setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** Deprecated: Use enrollment_token instead. this field will be removed in the future. */
@com.google.api.client.util.Key
private java.lang.String deviceType;
/** Deprecated: Use enrollment_token instead. this field will be removed in the future.
*/
public java.lang.String getDeviceType() {
return deviceType;
}
/** Deprecated: Use enrollment_token instead. this field will be removed in the future. */
public CreateEnrollmentToken setDeviceType(java.lang.String deviceType) {
this.deviceType = deviceType;
return this;
}
/**
* [Optional] The length of time the enrollment token is valid, ranging from 1 minute to
* [`Durations.MAX_VALUE`](https://developers.google.com/protocol-
* buffers/docs/reference/java/com/google/protobuf/util/Durations.html#MAX_VALUE),
* approximately 10,000 years. If not specified, the default duration is 1 hour.
*/
@com.google.api.client.util.Key("enrollmentToken.duration")
private String enrollmentTokenDuration;
/**[ Optional] The length of time the enrollment token is valid, ranging from 1 minute to
[ [`Durations.MAX_VALUE`](https://developers.google.com/protocol-
[ buffers/docs/reference/java/com/google/protobuf/util/Durations.html#MAX_VALUE), approximately
[ 10,000 years. If not specified, the default duration is 1 hour.
[
*/
public String getEnrollmentTokenDuration() {
return enrollmentTokenDuration;
}
/**
* [Optional] The length of time the enrollment token is valid, ranging from 1 minute to
* [`Durations.MAX_VALUE`](https://developers.google.com/protocol-
* buffers/docs/reference/java/com/google/protobuf/util/Durations.html#MAX_VALUE),
* approximately 10,000 years. If not specified, the default duration is 1 hour.
*/
public CreateEnrollmentToken setEnrollmentTokenDuration(String enrollmentTokenDuration) {
this.enrollmentTokenDuration = enrollmentTokenDuration;
return this;
}
/** [Required] The type of the enrollment token. */
@com.google.api.client.util.Key("enrollmentToken.enrollmentTokenType")
private java.lang.String enrollmentTokenEnrollmentTokenType;
/**[ Required] The type of the enrollment token.
[
*/
public java.lang.String getEnrollmentTokenEnrollmentTokenType() {
return enrollmentTokenEnrollmentTokenType;
}
/** [Required] The type of the enrollment token. */
public CreateEnrollmentToken setEnrollmentTokenEnrollmentTokenType(java.lang.String enrollmentTokenEnrollmentTokenType) {
this.enrollmentTokenEnrollmentTokenType = enrollmentTokenEnrollmentTokenType;
return this;
}
/**
* The token value that's passed to the device and authorizes the device to enroll. This is a
* read-only field generated by the server.
*/
@com.google.api.client.util.Key("enrollmentToken.token")
private java.lang.String enrollmentTokenToken;
/** The token value that's passed to the device and authorizes the device to enroll. This is a read-
only field generated by the server.
*/
public java.lang.String getEnrollmentTokenToken() {
return enrollmentTokenToken;
}
/**
* The token value that's passed to the device and authorizes the device to enroll. This is a
* read-only field generated by the server.
*/
public CreateEnrollmentToken setEnrollmentTokenToken(java.lang.String enrollmentTokenToken) {
this.enrollmentTokenToken = enrollmentTokenToken;
return this;
}
@Override
public CreateEnrollmentToken set(String parameterName, Object value) {
return (CreateEnrollmentToken) super.set(parameterName, value);
}
}
/**
* Returns a unique token to access an embeddable UI. To generate a web UI, pass the generated token
* into the managed Google Play javascript API. Each token may only be used to start one UI session.
* See the JavaScript API documentation for further information.
*
* Create a request for the method "enterprises.createWebToken".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link CreateWebToken#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.AdministratorWebTokenSpec}
* @return the request
*/
public CreateWebToken createWebToken(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.AdministratorWebTokenSpec content) throws java.io.IOException {
CreateWebToken result = new CreateWebToken(enterpriseId, content);
initialize(result);
return result;
}
public class CreateWebToken extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/createWebToken";
/**
* Returns a unique token to access an embeddable UI. To generate a web UI, pass the generated
* token into the managed Google Play javascript API. Each token may only be used to start one UI
* session. See the JavaScript API documentation for further information.
*
* Create a request for the method "enterprises.createWebToken".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link CreateWebToken#execute()} method to invoke the remote
* operation. {@link CreateWebToken#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.AdministratorWebTokenSpec}
* @since 1.13
*/
protected CreateWebToken(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.AdministratorWebTokenSpec content) {
super(AndroidEnterprise.this, "POST", REST_PATH, content, com.google.api.services.androidenterprise.model.AdministratorWebToken.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public CreateWebToken set$Xgafv(java.lang.String $Xgafv) {
return (CreateWebToken) super.set$Xgafv($Xgafv);
}
@Override
public CreateWebToken setAccessToken(java.lang.String accessToken) {
return (CreateWebToken) super.setAccessToken(accessToken);
}
@Override
public CreateWebToken setAlt(java.lang.String alt) {
return (CreateWebToken) super.setAlt(alt);
}
@Override
public CreateWebToken setCallback(java.lang.String callback) {
return (CreateWebToken) super.setCallback(callback);
}
@Override
public CreateWebToken setFields(java.lang.String fields) {
return (CreateWebToken) super.setFields(fields);
}
@Override
public CreateWebToken setKey(java.lang.String key) {
return (CreateWebToken) super.setKey(key);
}
@Override
public CreateWebToken setOauthToken(java.lang.String oauthToken) {
return (CreateWebToken) super.setOauthToken(oauthToken);
}
@Override
public CreateWebToken setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreateWebToken) super.setPrettyPrint(prettyPrint);
}
@Override
public CreateWebToken setQuotaUser(java.lang.String quotaUser) {
return (CreateWebToken) super.setQuotaUser(quotaUser);
}
@Override
public CreateWebToken setUploadType(java.lang.String uploadType) {
return (CreateWebToken) super.setUploadType(uploadType);
}
@Override
public CreateWebToken setUploadProtocol(java.lang.String uploadProtocol) {
return (CreateWebToken) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public CreateWebToken setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public CreateWebToken set(String parameterName, Object value) {
return (CreateWebToken) super.set(parameterName, value);
}
}
/**
* Enrolls an enterprise with the calling EMM.
*
* Create a request for the method "enterprises.enroll".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Enroll#execute()} method to invoke the remote operation.
*
* @param token Required. The token provided by the enterprise to register the EMM.
* @param content the {@link com.google.api.services.androidenterprise.model.Enterprise}
* @return the request
*/
public Enroll enroll(java.lang.String token, com.google.api.services.androidenterprise.model.Enterprise content) throws java.io.IOException {
Enroll result = new Enroll(token, content);
initialize(result);
return result;
}
public class Enroll extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/enroll";
/**
* Enrolls an enterprise with the calling EMM.
*
* Create a request for the method "enterprises.enroll".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Enroll#execute()} method to invoke the remote
* operation. {@link
* Enroll#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param token Required. The token provided by the enterprise to register the EMM.
* @param content the {@link com.google.api.services.androidenterprise.model.Enterprise}
* @since 1.13
*/
protected Enroll(java.lang.String token, com.google.api.services.androidenterprise.model.Enterprise content) {
super(AndroidEnterprise.this, "POST", REST_PATH, content, com.google.api.services.androidenterprise.model.Enterprise.class);
this.token = com.google.api.client.util.Preconditions.checkNotNull(token, "Required parameter token must be specified.");
}
@Override
public Enroll set$Xgafv(java.lang.String $Xgafv) {
return (Enroll) super.set$Xgafv($Xgafv);
}
@Override
public Enroll setAccessToken(java.lang.String accessToken) {
return (Enroll) super.setAccessToken(accessToken);
}
@Override
public Enroll setAlt(java.lang.String alt) {
return (Enroll) super.setAlt(alt);
}
@Override
public Enroll setCallback(java.lang.String callback) {
return (Enroll) super.setCallback(callback);
}
@Override
public Enroll setFields(java.lang.String fields) {
return (Enroll) super.setFields(fields);
}
@Override
public Enroll setKey(java.lang.String key) {
return (Enroll) super.setKey(key);
}
@Override
public Enroll setOauthToken(java.lang.String oauthToken) {
return (Enroll) super.setOauthToken(oauthToken);
}
@Override
public Enroll setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Enroll) super.setPrettyPrint(prettyPrint);
}
@Override
public Enroll setQuotaUser(java.lang.String quotaUser) {
return (Enroll) super.setQuotaUser(quotaUser);
}
@Override
public Enroll setUploadType(java.lang.String uploadType) {
return (Enroll) super.setUploadType(uploadType);
}
@Override
public Enroll setUploadProtocol(java.lang.String uploadProtocol) {
return (Enroll) super.setUploadProtocol(uploadProtocol);
}
/** Required. The token provided by the enterprise to register the EMM. */
@com.google.api.client.util.Key
private java.lang.String token;
/** Required. The token provided by the enterprise to register the EMM.
*/
public java.lang.String getToken() {
return token;
}
/** Required. The token provided by the enterprise to register the EMM. */
public Enroll setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public Enroll set(String parameterName, Object value) {
return (Enroll) super.set(parameterName, value);
}
}
/**
* Generates a sign-up URL.
*
* Create a request for the method "enterprises.generateSignupUrl".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GenerateSignupUrl#execute()} method to invoke the remote
* operation.
*
* @return the request
*/
public GenerateSignupUrl generateSignupUrl() throws java.io.IOException {
GenerateSignupUrl result = new GenerateSignupUrl();
initialize(result);
return result;
}
public class GenerateSignupUrl extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/signupUrl";
/**
* Generates a sign-up URL.
*
* Create a request for the method "enterprises.generateSignupUrl".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GenerateSignupUrl#execute()} method to invoke the
* remote operation. {@link GenerateSignupUrl#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @since 1.13
*/
protected GenerateSignupUrl() {
super(AndroidEnterprise.this, "POST", REST_PATH, null, com.google.api.services.androidenterprise.model.SignupInfo.class);
}
@Override
public GenerateSignupUrl set$Xgafv(java.lang.String $Xgafv) {
return (GenerateSignupUrl) super.set$Xgafv($Xgafv);
}
@Override
public GenerateSignupUrl setAccessToken(java.lang.String accessToken) {
return (GenerateSignupUrl) super.setAccessToken(accessToken);
}
@Override
public GenerateSignupUrl setAlt(java.lang.String alt) {
return (GenerateSignupUrl) super.setAlt(alt);
}
@Override
public GenerateSignupUrl setCallback(java.lang.String callback) {
return (GenerateSignupUrl) super.setCallback(callback);
}
@Override
public GenerateSignupUrl setFields(java.lang.String fields) {
return (GenerateSignupUrl) super.setFields(fields);
}
@Override
public GenerateSignupUrl setKey(java.lang.String key) {
return (GenerateSignupUrl) super.setKey(key);
}
@Override
public GenerateSignupUrl setOauthToken(java.lang.String oauthToken) {
return (GenerateSignupUrl) super.setOauthToken(oauthToken);
}
@Override
public GenerateSignupUrl setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenerateSignupUrl) super.setPrettyPrint(prettyPrint);
}
@Override
public GenerateSignupUrl setQuotaUser(java.lang.String quotaUser) {
return (GenerateSignupUrl) super.setQuotaUser(quotaUser);
}
@Override
public GenerateSignupUrl setUploadType(java.lang.String uploadType) {
return (GenerateSignupUrl) super.setUploadType(uploadType);
}
@Override
public GenerateSignupUrl setUploadProtocol(java.lang.String uploadProtocol) {
return (GenerateSignupUrl) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Email address used to prefill the admin field of the enterprise signup form. This
* value is a hint only and can be altered by the user.
*/
@com.google.api.client.util.Key
private java.lang.String adminEmail;
/** Optional. Email address used to prefill the admin field of the enterprise signup form. This value
is a hint only and can be altered by the user.
*/
public java.lang.String getAdminEmail() {
return adminEmail;
}
/**
* Optional. Email address used to prefill the admin field of the enterprise signup form. This
* value is a hint only and can be altered by the user.
*/
public GenerateSignupUrl setAdminEmail(java.lang.String adminEmail) {
this.adminEmail = adminEmail;
return this;
}
/**
* The callback URL to which the Admin will be redirected after successfully creating an
* enterprise. Before redirecting there the system will add a single query parameter to this
* URL named "enterpriseToken" which will contain an opaque token to be used for the
* CompleteSignup request. Beware that this means that the URL will be parsed, the parameter
* added and then a new URL formatted, i.e. there may be some minor formatting changes and,
* more importantly, the URL must be well-formed so that it can be parsed.
*/
@com.google.api.client.util.Key
private java.lang.String callbackUrl;
/** The callback URL to which the Admin will be redirected after successfully creating an enterprise.
Before redirecting there the system will add a single query parameter to this URL named
"enterpriseToken" which will contain an opaque token to be used for the CompleteSignup request.
Beware that this means that the URL will be parsed, the parameter added and then a new URL
formatted, i.e. there may be some minor formatting changes and, more importantly, the URL must be
well-formed so that it can be parsed.
*/
public java.lang.String getCallbackUrl() {
return callbackUrl;
}
/**
* The callback URL to which the Admin will be redirected after successfully creating an
* enterprise. Before redirecting there the system will add a single query parameter to this
* URL named "enterpriseToken" which will contain an opaque token to be used for the
* CompleteSignup request. Beware that this means that the URL will be parsed, the parameter
* added and then a new URL formatted, i.e. there may be some minor formatting changes and,
* more importantly, the URL must be well-formed so that it can be parsed.
*/
public GenerateSignupUrl setCallbackUrl(java.lang.String callbackUrl) {
this.callbackUrl = callbackUrl;
return this;
}
@Override
public GenerateSignupUrl set(String parameterName, Object value) {
return (GenerateSignupUrl) super.set(parameterName, value);
}
}
/**
* Retrieves the name and domain of an enterprise.
*
* Create a request for the method "enterprises.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public Get get(java.lang.String enterpriseId) throws java.io.IOException {
Get result = new Get(enterpriseId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}";
/**
* Retrieves the name and domain of an enterprise.
*
* Create a request for the method "enterprises.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected Get(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.Enterprise.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns a service account and credentials. The service account can be bound to the enterprise by
* calling setAccount. The service account is unique to this enterprise and EMM, and will be deleted
* if the enterprise is unbound. The credentials contain private key data and are not stored server-
* side. This method can only be called after calling Enterprises.Enroll or
* Enterprises.CompleteSignup, and before Enterprises.SetAccount; at other times it will return an
* error. Subsequent calls after the first will generate a new, unique set of credentials, and
* invalidate the previously generated credentials. Once the service account is bound to the
* enterprise, it can be managed using the serviceAccountKeys resource.
*
* Create a request for the method "enterprises.getServiceAccount".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GetServiceAccount#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public GetServiceAccount getServiceAccount(java.lang.String enterpriseId) throws java.io.IOException {
GetServiceAccount result = new GetServiceAccount(enterpriseId);
initialize(result);
return result;
}
public class GetServiceAccount extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/serviceAccount";
/**
* Returns a service account and credentials. The service account can be bound to the enterprise
* by calling setAccount. The service account is unique to this enterprise and EMM, and will be
* deleted if the enterprise is unbound. The credentials contain private key data and are not
* stored server-side. This method can only be called after calling Enterprises.Enroll or
* Enterprises.CompleteSignup, and before Enterprises.SetAccount; at other times it will return an
* error. Subsequent calls after the first will generate a new, unique set of credentials, and
* invalidate the previously generated credentials. Once the service account is bound to the
* enterprise, it can be managed using the serviceAccountKeys resource.
*
* Create a request for the method "enterprises.getServiceAccount".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GetServiceAccount#execute()} method to invoke the
* remote operation. {@link GetServiceAccount#initialize(com.google.api.client.googleapis.serv
* ices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected GetServiceAccount(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ServiceAccount.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetServiceAccount set$Xgafv(java.lang.String $Xgafv) {
return (GetServiceAccount) super.set$Xgafv($Xgafv);
}
@Override
public GetServiceAccount setAccessToken(java.lang.String accessToken) {
return (GetServiceAccount) super.setAccessToken(accessToken);
}
@Override
public GetServiceAccount setAlt(java.lang.String alt) {
return (GetServiceAccount) super.setAlt(alt);
}
@Override
public GetServiceAccount setCallback(java.lang.String callback) {
return (GetServiceAccount) super.setCallback(callback);
}
@Override
public GetServiceAccount setFields(java.lang.String fields) {
return (GetServiceAccount) super.setFields(fields);
}
@Override
public GetServiceAccount setKey(java.lang.String key) {
return (GetServiceAccount) super.setKey(key);
}
@Override
public GetServiceAccount setOauthToken(java.lang.String oauthToken) {
return (GetServiceAccount) super.setOauthToken(oauthToken);
}
@Override
public GetServiceAccount setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetServiceAccount) super.setPrettyPrint(prettyPrint);
}
@Override
public GetServiceAccount setQuotaUser(java.lang.String quotaUser) {
return (GetServiceAccount) super.setQuotaUser(quotaUser);
}
@Override
public GetServiceAccount setUploadType(java.lang.String uploadType) {
return (GetServiceAccount) super.setUploadType(uploadType);
}
@Override
public GetServiceAccount setUploadProtocol(java.lang.String uploadProtocol) {
return (GetServiceAccount) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public GetServiceAccount setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The type of credential to return with the service account. Required. */
@com.google.api.client.util.Key
private java.lang.String keyType;
/** The type of credential to return with the service account. Required.
*/
public java.lang.String getKeyType() {
return keyType;
}
/** The type of credential to return with the service account. Required. */
public GetServiceAccount setKeyType(java.lang.String keyType) {
this.keyType = keyType;
return this;
}
@Override
public GetServiceAccount set(String parameterName, Object value) {
return (GetServiceAccount) super.set(parameterName, value);
}
}
/**
* Returns the store layout for the enterprise. If the store layout has not been set, returns
* "basic" as the store layout type and no homepage.
*
* Create a request for the method "enterprises.getStoreLayout".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GetStoreLayout#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public GetStoreLayout getStoreLayout(java.lang.String enterpriseId) throws java.io.IOException {
GetStoreLayout result = new GetStoreLayout(enterpriseId);
initialize(result);
return result;
}
public class GetStoreLayout extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout";
/**
* Returns the store layout for the enterprise. If the store layout has not been set, returns
* "basic" as the store layout type and no homepage.
*
* Create a request for the method "enterprises.getStoreLayout".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GetStoreLayout#execute()} method to invoke the remote
* operation. {@link GetStoreLayout#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected GetStoreLayout(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.StoreLayout.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetStoreLayout set$Xgafv(java.lang.String $Xgafv) {
return (GetStoreLayout) super.set$Xgafv($Xgafv);
}
@Override
public GetStoreLayout setAccessToken(java.lang.String accessToken) {
return (GetStoreLayout) super.setAccessToken(accessToken);
}
@Override
public GetStoreLayout setAlt(java.lang.String alt) {
return (GetStoreLayout) super.setAlt(alt);
}
@Override
public GetStoreLayout setCallback(java.lang.String callback) {
return (GetStoreLayout) super.setCallback(callback);
}
@Override
public GetStoreLayout setFields(java.lang.String fields) {
return (GetStoreLayout) super.setFields(fields);
}
@Override
public GetStoreLayout setKey(java.lang.String key) {
return (GetStoreLayout) super.setKey(key);
}
@Override
public GetStoreLayout setOauthToken(java.lang.String oauthToken) {
return (GetStoreLayout) super.setOauthToken(oauthToken);
}
@Override
public GetStoreLayout setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetStoreLayout) super.setPrettyPrint(prettyPrint);
}
@Override
public GetStoreLayout setQuotaUser(java.lang.String quotaUser) {
return (GetStoreLayout) super.setQuotaUser(quotaUser);
}
@Override
public GetStoreLayout setUploadType(java.lang.String uploadType) {
return (GetStoreLayout) super.setUploadType(uploadType);
}
@Override
public GetStoreLayout setUploadProtocol(java.lang.String uploadProtocol) {
return (GetStoreLayout) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public GetStoreLayout setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public GetStoreLayout set(String parameterName, Object value) {
return (GetStoreLayout) super.set(parameterName, value);
}
}
/**
* Looks up an enterprise by domain name. This is only supported for enterprises created via the
* Google-initiated creation flow. Lookup of the id is not needed for enterprises created via the
* EMM-initiated flow since the EMM learns the enterprise ID in the callback specified in the
* Enterprises.generateSignupUrl call.
*
* Create a request for the method "enterprises.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param domain Required. The exact primary domain name of the enterprise to look up.
* @return the request
*/
public List list(java.lang.String domain) throws java.io.IOException {
List result = new List(domain);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises";
/**
* Looks up an enterprise by domain name. This is only supported for enterprises created via the
* Google-initiated creation flow. Lookup of the id is not needed for enterprises created via the
* EMM-initiated flow since the EMM learns the enterprise ID in the callback specified in the
* Enterprises.generateSignupUrl call.
*
* Create a request for the method "enterprises.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param domain Required. The exact primary domain name of the enterprise to look up.
* @since 1.13
*/
protected List(java.lang.String domain) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.EnterprisesListResponse.class);
this.domain = com.google.api.client.util.Preconditions.checkNotNull(domain, "Required parameter domain must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The exact primary domain name of the enterprise to look up. */
@com.google.api.client.util.Key
private java.lang.String domain;
/** Required. The exact primary domain name of the enterprise to look up.
*/
public java.lang.String getDomain() {
return domain;
}
/** Required. The exact primary domain name of the enterprise to look up. */
public List setDomain(java.lang.String domain) {
this.domain = domain;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Pulls and returns a notification set for the enterprises associated with the service account
* authenticated for the request. The notification set may be empty if no notification are pending.
* A notification set returned needs to be acknowledged within 20 seconds by calling
* Enterprises.AcknowledgeNotificationSet, unless the notification set is empty. Notifications that
* are not acknowledged within the 20 seconds will eventually be included again in the response to
* another PullNotificationSet request, and those that are never acknowledged will ultimately be
* deleted according to the Google Cloud Platform Pub/Sub system policy. Multiple requests might be
* performed concurrently to retrieve notifications, in which case the pending notifications (if
* any) will be split among each caller, if any are pending. If no notifications are present, an
* empty notification list is returned. Subsequent requests may return more notifications once they
* become available.
*
* Create a request for the method "enterprises.pullNotificationSet".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link PullNotificationSet#execute()} method to invoke the remote
* operation.
*
* @return the request
*/
public PullNotificationSet pullNotificationSet() throws java.io.IOException {
PullNotificationSet result = new PullNotificationSet();
initialize(result);
return result;
}
public class PullNotificationSet extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/pullNotificationSet";
/**
* Pulls and returns a notification set for the enterprises associated with the service account
* authenticated for the request. The notification set may be empty if no notification are
* pending. A notification set returned needs to be acknowledged within 20 seconds by calling
* Enterprises.AcknowledgeNotificationSet, unless the notification set is empty. Notifications
* that are not acknowledged within the 20 seconds will eventually be included again in the
* response to another PullNotificationSet request, and those that are never acknowledged will
* ultimately be deleted according to the Google Cloud Platform Pub/Sub system policy. Multiple
* requests might be performed concurrently to retrieve notifications, in which case the pending
* notifications (if any) will be split among each caller, if any are pending. If no notifications
* are present, an empty notification list is returned. Subsequent requests may return more
* notifications once they become available.
*
* Create a request for the method "enterprises.pullNotificationSet".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link PullNotificationSet#execute()} method to invoke the
* remote operation. {@link PullNotificationSet#initialize(com.google.api.client.googleapis.se
* rvices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @since 1.13
*/
protected PullNotificationSet() {
super(AndroidEnterprise.this, "POST", REST_PATH, null, com.google.api.services.androidenterprise.model.NotificationSet.class);
}
@Override
public PullNotificationSet set$Xgafv(java.lang.String $Xgafv) {
return (PullNotificationSet) super.set$Xgafv($Xgafv);
}
@Override
public PullNotificationSet setAccessToken(java.lang.String accessToken) {
return (PullNotificationSet) super.setAccessToken(accessToken);
}
@Override
public PullNotificationSet setAlt(java.lang.String alt) {
return (PullNotificationSet) super.setAlt(alt);
}
@Override
public PullNotificationSet setCallback(java.lang.String callback) {
return (PullNotificationSet) super.setCallback(callback);
}
@Override
public PullNotificationSet setFields(java.lang.String fields) {
return (PullNotificationSet) super.setFields(fields);
}
@Override
public PullNotificationSet setKey(java.lang.String key) {
return (PullNotificationSet) super.setKey(key);
}
@Override
public PullNotificationSet setOauthToken(java.lang.String oauthToken) {
return (PullNotificationSet) super.setOauthToken(oauthToken);
}
@Override
public PullNotificationSet setPrettyPrint(java.lang.Boolean prettyPrint) {
return (PullNotificationSet) super.setPrettyPrint(prettyPrint);
}
@Override
public PullNotificationSet setQuotaUser(java.lang.String quotaUser) {
return (PullNotificationSet) super.setQuotaUser(quotaUser);
}
@Override
public PullNotificationSet setUploadType(java.lang.String uploadType) {
return (PullNotificationSet) super.setUploadType(uploadType);
}
@Override
public PullNotificationSet setUploadProtocol(java.lang.String uploadProtocol) {
return (PullNotificationSet) super.setUploadProtocol(uploadProtocol);
}
/**
* The request mode for pulling notifications. Specifying waitForNotifications will cause the
* request to block and wait until one or more notifications are present, or return an empty
* notification list if no notifications are present after some time. Specifying
* returnImmediately will cause the request to immediately return the pending notifications,
* or an empty list if no notifications are present. If omitted, defaults to
* waitForNotifications.
*/
@com.google.api.client.util.Key
private java.lang.String requestMode;
/** The request mode for pulling notifications. Specifying waitForNotifications will cause the request
to block and wait until one or more notifications are present, or return an empty notification list
if no notifications are present after some time. Specifying returnImmediately will cause the
request to immediately return the pending notifications, or an empty list if no notifications are
present. If omitted, defaults to waitForNotifications.
*/
public java.lang.String getRequestMode() {
return requestMode;
}
/**
* The request mode for pulling notifications. Specifying waitForNotifications will cause the
* request to block and wait until one or more notifications are present, or return an empty
* notification list if no notifications are present after some time. Specifying
* returnImmediately will cause the request to immediately return the pending notifications,
* or an empty list if no notifications are present. If omitted, defaults to
* waitForNotifications.
*/
public PullNotificationSet setRequestMode(java.lang.String requestMode) {
this.requestMode = requestMode;
return this;
}
@Override
public PullNotificationSet set(String parameterName, Object value) {
return (PullNotificationSet) super.set(parameterName, value);
}
}
/**
* Sends a test notification to validate the EMM integration with the Google Cloud Pub/Sub service
* for this enterprise.
*
* Create a request for the method "enterprises.sendTestPushNotification".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link SendTestPushNotification#execute()} method to invoke the
* remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public SendTestPushNotification sendTestPushNotification(java.lang.String enterpriseId) throws java.io.IOException {
SendTestPushNotification result = new SendTestPushNotification(enterpriseId);
initialize(result);
return result;
}
public class SendTestPushNotification extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/sendTestPushNotification";
/**
* Sends a test notification to validate the EMM integration with the Google Cloud Pub/Sub service
* for this enterprise.
*
* Create a request for the method "enterprises.sendTestPushNotification".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link SendTestPushNotification#execute()} method to invoke
* the remote operation. {@link SendTestPushNotification#initialize(com.google.api.client.goog
* leapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected SendTestPushNotification(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "POST", REST_PATH, null, com.google.api.services.androidenterprise.model.EnterprisesSendTestPushNotificationResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public SendTestPushNotification set$Xgafv(java.lang.String $Xgafv) {
return (SendTestPushNotification) super.set$Xgafv($Xgafv);
}
@Override
public SendTestPushNotification setAccessToken(java.lang.String accessToken) {
return (SendTestPushNotification) super.setAccessToken(accessToken);
}
@Override
public SendTestPushNotification setAlt(java.lang.String alt) {
return (SendTestPushNotification) super.setAlt(alt);
}
@Override
public SendTestPushNotification setCallback(java.lang.String callback) {
return (SendTestPushNotification) super.setCallback(callback);
}
@Override
public SendTestPushNotification setFields(java.lang.String fields) {
return (SendTestPushNotification) super.setFields(fields);
}
@Override
public SendTestPushNotification setKey(java.lang.String key) {
return (SendTestPushNotification) super.setKey(key);
}
@Override
public SendTestPushNotification setOauthToken(java.lang.String oauthToken) {
return (SendTestPushNotification) super.setOauthToken(oauthToken);
}
@Override
public SendTestPushNotification setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SendTestPushNotification) super.setPrettyPrint(prettyPrint);
}
@Override
public SendTestPushNotification setQuotaUser(java.lang.String quotaUser) {
return (SendTestPushNotification) super.setQuotaUser(quotaUser);
}
@Override
public SendTestPushNotification setUploadType(java.lang.String uploadType) {
return (SendTestPushNotification) super.setUploadType(uploadType);
}
@Override
public SendTestPushNotification setUploadProtocol(java.lang.String uploadProtocol) {
return (SendTestPushNotification) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public SendTestPushNotification setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public SendTestPushNotification set(String parameterName, Object value) {
return (SendTestPushNotification) super.set(parameterName, value);
}
}
/**
* Sets the account that will be used to authenticate to the API as the enterprise.
*
* Create a request for the method "enterprises.setAccount".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link SetAccount#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.EnterpriseAccount}
* @return the request
*/
public SetAccount setAccount(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.EnterpriseAccount content) throws java.io.IOException {
SetAccount result = new SetAccount(enterpriseId, content);
initialize(result);
return result;
}
public class SetAccount extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/account";
/**
* Sets the account that will be used to authenticate to the API as the enterprise.
*
* Create a request for the method "enterprises.setAccount".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link SetAccount#execute()} method to invoke the remote
* operation. {@link
* SetAccount#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.EnterpriseAccount}
* @since 1.13
*/
protected SetAccount(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.EnterpriseAccount content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.EnterpriseAccount.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public SetAccount set$Xgafv(java.lang.String $Xgafv) {
return (SetAccount) super.set$Xgafv($Xgafv);
}
@Override
public SetAccount setAccessToken(java.lang.String accessToken) {
return (SetAccount) super.setAccessToken(accessToken);
}
@Override
public SetAccount setAlt(java.lang.String alt) {
return (SetAccount) super.setAlt(alt);
}
@Override
public SetAccount setCallback(java.lang.String callback) {
return (SetAccount) super.setCallback(callback);
}
@Override
public SetAccount setFields(java.lang.String fields) {
return (SetAccount) super.setFields(fields);
}
@Override
public SetAccount setKey(java.lang.String key) {
return (SetAccount) super.setKey(key);
}
@Override
public SetAccount setOauthToken(java.lang.String oauthToken) {
return (SetAccount) super.setOauthToken(oauthToken);
}
@Override
public SetAccount setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetAccount) super.setPrettyPrint(prettyPrint);
}
@Override
public SetAccount setQuotaUser(java.lang.String quotaUser) {
return (SetAccount) super.setQuotaUser(quotaUser);
}
@Override
public SetAccount setUploadType(java.lang.String uploadType) {
return (SetAccount) super.setUploadType(uploadType);
}
@Override
public SetAccount setUploadProtocol(java.lang.String uploadProtocol) {
return (SetAccount) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public SetAccount setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public SetAccount set(String parameterName, Object value) {
return (SetAccount) super.set(parameterName, value);
}
}
/**
* Sets the store layout for the enterprise. By default, storeLayoutType is set to "basic" and the
* basic store layout is enabled. The basic layout only contains apps approved by the admin, and
* that have been added to the available product set for a user (using the setAvailableProductSet
* call). Apps on the page are sorted in order of their product ID value. If you create a custom
* store layout (by setting storeLayoutType = "custom" and setting a homepage), the basic store
* layout is disabled.
*
* Create a request for the method "enterprises.setStoreLayout".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link SetStoreLayout#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.StoreLayout}
* @return the request
*/
public SetStoreLayout setStoreLayout(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.StoreLayout content) throws java.io.IOException {
SetStoreLayout result = new SetStoreLayout(enterpriseId, content);
initialize(result);
return result;
}
public class SetStoreLayout extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout";
/**
* Sets the store layout for the enterprise. By default, storeLayoutType is set to "basic" and the
* basic store layout is enabled. The basic layout only contains apps approved by the admin, and
* that have been added to the available product set for a user (using the setAvailableProductSet
* call). Apps on the page are sorted in order of their product ID value. If you create a custom
* store layout (by setting storeLayoutType = "custom" and setting a homepage), the basic store
* layout is disabled.
*
* Create a request for the method "enterprises.setStoreLayout".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link SetStoreLayout#execute()} method to invoke the remote
* operation. {@link SetStoreLayout#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.StoreLayout}
* @since 1.13
*/
protected SetStoreLayout(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.StoreLayout content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.StoreLayout.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public SetStoreLayout set$Xgafv(java.lang.String $Xgafv) {
return (SetStoreLayout) super.set$Xgafv($Xgafv);
}
@Override
public SetStoreLayout setAccessToken(java.lang.String accessToken) {
return (SetStoreLayout) super.setAccessToken(accessToken);
}
@Override
public SetStoreLayout setAlt(java.lang.String alt) {
return (SetStoreLayout) super.setAlt(alt);
}
@Override
public SetStoreLayout setCallback(java.lang.String callback) {
return (SetStoreLayout) super.setCallback(callback);
}
@Override
public SetStoreLayout setFields(java.lang.String fields) {
return (SetStoreLayout) super.setFields(fields);
}
@Override
public SetStoreLayout setKey(java.lang.String key) {
return (SetStoreLayout) super.setKey(key);
}
@Override
public SetStoreLayout setOauthToken(java.lang.String oauthToken) {
return (SetStoreLayout) super.setOauthToken(oauthToken);
}
@Override
public SetStoreLayout setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetStoreLayout) super.setPrettyPrint(prettyPrint);
}
@Override
public SetStoreLayout setQuotaUser(java.lang.String quotaUser) {
return (SetStoreLayout) super.setQuotaUser(quotaUser);
}
@Override
public SetStoreLayout setUploadType(java.lang.String uploadType) {
return (SetStoreLayout) super.setUploadType(uploadType);
}
@Override
public SetStoreLayout setUploadProtocol(java.lang.String uploadProtocol) {
return (SetStoreLayout) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public SetStoreLayout setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public SetStoreLayout set(String parameterName, Object value) {
return (SetStoreLayout) super.set(parameterName, value);
}
}
/**
* Unenrolls an enterprise from the calling EMM.
*
* Create a request for the method "enterprises.unenroll".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Unenroll#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public Unenroll unenroll(java.lang.String enterpriseId) throws java.io.IOException {
Unenroll result = new Unenroll(enterpriseId);
initialize(result);
return result;
}
public class Unenroll extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/unenroll";
/**
* Unenrolls an enterprise from the calling EMM.
*
* Create a request for the method "enterprises.unenroll".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Unenroll#execute()} method to invoke the remote
* operation. {@link
* Unenroll#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected Unenroll(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "POST", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public Unenroll set$Xgafv(java.lang.String $Xgafv) {
return (Unenroll) super.set$Xgafv($Xgafv);
}
@Override
public Unenroll setAccessToken(java.lang.String accessToken) {
return (Unenroll) super.setAccessToken(accessToken);
}
@Override
public Unenroll setAlt(java.lang.String alt) {
return (Unenroll) super.setAlt(alt);
}
@Override
public Unenroll setCallback(java.lang.String callback) {
return (Unenroll) super.setCallback(callback);
}
@Override
public Unenroll setFields(java.lang.String fields) {
return (Unenroll) super.setFields(fields);
}
@Override
public Unenroll setKey(java.lang.String key) {
return (Unenroll) super.setKey(key);
}
@Override
public Unenroll setOauthToken(java.lang.String oauthToken) {
return (Unenroll) super.setOauthToken(oauthToken);
}
@Override
public Unenroll setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unenroll) super.setPrettyPrint(prettyPrint);
}
@Override
public Unenroll setQuotaUser(java.lang.String quotaUser) {
return (Unenroll) super.setQuotaUser(quotaUser);
}
@Override
public Unenroll setUploadType(java.lang.String uploadType) {
return (Unenroll) super.setUploadType(uploadType);
}
@Override
public Unenroll setUploadProtocol(java.lang.String uploadProtocol) {
return (Unenroll) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Unenroll setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public Unenroll set(String parameterName, Object value) {
return (Unenroll) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Entitlements collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Entitlements.List request = androidenterprise.entitlements().list(parameters ...)}
*
*
* @return the resource collection
*/
public Entitlements entitlements() {
return new Entitlements();
}
/**
* The "entitlements" collection of methods.
*/
public class Entitlements {
/**
* Removes an entitlement to an app for a user. **Note:** This item has been deprecated. New
* integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "entitlements.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param entitlementId The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String userId, java.lang.String entitlementId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, userId, entitlementId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/entitlements/{entitlementId}";
/**
* Removes an entitlement to an app for a user. **Note:** This item has been deprecated. New
* integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "entitlements.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param entitlementId The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String userId, java.lang.String entitlementId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.entitlementId = com.google.api.client.util.Preconditions.checkNotNull(entitlementId, "Required parameter entitlementId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String entitlementId;
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getEntitlementId() {
return entitlementId;
}
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm". */
public Delete setEntitlementId(java.lang.String entitlementId) {
this.entitlementId = entitlementId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves details of an entitlement. **Note:** This item has been deprecated. New integrations
* cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "entitlements.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param entitlementId The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String entitlementId) throws java.io.IOException {
Get result = new Get(enterpriseId, userId, entitlementId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/entitlements/{entitlementId}";
/**
* Retrieves details of an entitlement. **Note:** This item has been deprecated. New integrations
* cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "entitlements.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param entitlementId The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String entitlementId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.Entitlement.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.entitlementId = com.google.api.client.util.Preconditions.checkNotNull(entitlementId, "Required parameter entitlementId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String entitlementId;
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getEntitlementId() {
return entitlementId;
}
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm". */
public Get setEntitlementId(java.lang.String entitlementId) {
this.entitlementId = entitlementId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all entitlements for the specified user. Only the ID is set. **Note:** This item has been
* deprecated. New integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "entitlements.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String userId) throws java.io.IOException {
List result = new List(enterpriseId, userId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/entitlements";
/**
* Lists all entitlements for the specified user. Only the ID is set. **Note:** This item has been
* deprecated. New integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "entitlements.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String userId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.EntitlementsListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Adds or updates an entitlement to an app for a user. **Note:** This item has been deprecated. New
* integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "entitlements.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param entitlementId The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
* @param content the {@link com.google.api.services.androidenterprise.model.Entitlement}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String entitlementId, com.google.api.services.androidenterprise.model.Entitlement content) throws java.io.IOException {
Update result = new Update(enterpriseId, userId, entitlementId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/entitlements/{entitlementId}";
/**
* Adds or updates an entitlement to an app for a user. **Note:** This item has been deprecated.
* New integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "entitlements.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param entitlementId The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
* @param content the {@link com.google.api.services.androidenterprise.model.Entitlement}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String entitlementId, com.google.api.services.androidenterprise.model.Entitlement content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.Entitlement.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.entitlementId = com.google.api.client.util.Preconditions.checkNotNull(entitlementId, "Required parameter entitlementId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Update setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String entitlementId;
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getEntitlementId() {
return entitlementId;
}
/** The ID of the entitlement (a product ID), e.g. "app:com.google.android.gm". */
public Update setEntitlementId(java.lang.String entitlementId) {
this.entitlementId = entitlementId;
return this;
}
/**
* Set to true to also install the product on all the user's devices where possible. Failure
* to install on one or more devices will not prevent this operation from returning
* successfully, as long as the entitlement was successfully assigned to the user.
*/
@com.google.api.client.util.Key
private java.lang.Boolean install;
/** Set to true to also install the product on all the user's devices where possible. Failure to
install on one or more devices will not prevent this operation from returning successfully, as long
as the entitlement was successfully assigned to the user.
*/
public java.lang.Boolean getInstall() {
return install;
}
/**
* Set to true to also install the product on all the user's devices where possible. Failure
* to install on one or more devices will not prevent this operation from returning
* successfully, as long as the entitlement was successfully assigned to the user.
*/
public Update setInstall(java.lang.Boolean install) {
this.install = install;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Grouplicenses collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Grouplicenses.List request = androidenterprise.grouplicenses().list(parameters ...)}
*
*
* @return the resource collection
*/
public Grouplicenses grouplicenses() {
return new Grouplicenses();
}
/**
* The "grouplicenses" collection of methods.
*/
public class Grouplicenses {
/**
* Retrieves details of an enterprise's group license for a product. **Note:** This item has been
* deprecated. New integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "grouplicenses.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param groupLicenseId The ID of the product the group license is for, e.g. "app:com.google.android.gm".
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String groupLicenseId) throws java.io.IOException {
Get result = new Get(enterpriseId, groupLicenseId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/groupLicenses/{groupLicenseId}";
/**
* Retrieves details of an enterprise's group license for a product. **Note:** This item has been
* deprecated. New integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "grouplicenses.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param groupLicenseId The ID of the product the group license is for, e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String groupLicenseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.GroupLicense.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.groupLicenseId = com.google.api.client.util.Preconditions.checkNotNull(groupLicenseId, "Required parameter groupLicenseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product the group license is for, e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String groupLicenseId;
/** The ID of the product the group license is for, e.g. "app:com.google.android.gm".
*/
public java.lang.String getGroupLicenseId() {
return groupLicenseId;
}
/** The ID of the product the group license is for, e.g. "app:com.google.android.gm". */
public Get setGroupLicenseId(java.lang.String groupLicenseId) {
this.groupLicenseId = groupLicenseId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves IDs of all products for which the enterprise has a group license. **Note:** This item
* has been deprecated. New integrations cannot use this method and can refer to our new
* recommendations.
*
* Create a request for the method "grouplicenses.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public List list(java.lang.String enterpriseId) throws java.io.IOException {
List result = new List(enterpriseId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/groupLicenses";
/**
* Retrieves IDs of all products for which the enterprise has a group license. **Note:** This item
* has been deprecated. New integrations cannot use this method and can refer to our new
* recommendations.
*
* Create a request for the method "grouplicenses.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected List(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.GroupLicensesListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Grouplicenseusers collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Grouplicenseusers.List request = androidenterprise.grouplicenseusers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Grouplicenseusers grouplicenseusers() {
return new Grouplicenseusers();
}
/**
* The "grouplicenseusers" collection of methods.
*/
public class Grouplicenseusers {
/**
* Retrieves the IDs of the users who have been granted entitlements under the license. **Note:**
* This item has been deprecated. New integrations cannot use this method and can refer to our new
* recommendations.
*
* Create a request for the method "grouplicenseusers.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param groupLicenseId The ID of the product the group license is for, e.g. "app:com.google.android.gm".
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String groupLicenseId) throws java.io.IOException {
List result = new List(enterpriseId, groupLicenseId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/groupLicenses/{groupLicenseId}/users";
/**
* Retrieves the IDs of the users who have been granted entitlements under the license. **Note:**
* This item has been deprecated. New integrations cannot use this method and can refer to our new
* recommendations.
*
* Create a request for the method "grouplicenseusers.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param groupLicenseId The ID of the product the group license is for, e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String groupLicenseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.GroupLicenseUsersListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.groupLicenseId = com.google.api.client.util.Preconditions.checkNotNull(groupLicenseId, "Required parameter groupLicenseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product the group license is for, e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String groupLicenseId;
/** The ID of the product the group license is for, e.g. "app:com.google.android.gm".
*/
public java.lang.String getGroupLicenseId() {
return groupLicenseId;
}
/** The ID of the product the group license is for, e.g. "app:com.google.android.gm". */
public List setGroupLicenseId(java.lang.String groupLicenseId) {
this.groupLicenseId = groupLicenseId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Installs collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Installs.List request = androidenterprise.installs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Installs installs() {
return new Installs();
}
/**
* The "installs" collection of methods.
*/
public class Installs {
/**
* Requests to remove an app from a device. A call to get or list will still show the app as
* installed on the device until it is actually removed. A successful response indicates that a
* removal request has been sent to the device. The call will be considered successful even if the
* app is not present on the device (e.g. it was never installed, or was removed by the user).
*
* Create a request for the method "installs.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param installId The ID of the product represented by the install, e.g. "app:com.google.android.gm".
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String installId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, userId, deviceId, installId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/installs/{installId}";
/**
* Requests to remove an app from a device. A call to get or list will still show the app as
* installed on the device until it is actually removed. A successful response indicates that a
* removal request has been sent to the device. The call will be considered successful even if the
* app is not present on the device (e.g. it was never installed, or was removed by the user).
*
* Create a request for the method "installs.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param installId The ID of the product represented by the install, e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String installId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
this.installId = com.google.api.client.util.Preconditions.checkNotNull(installId, "Required parameter installId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The Android ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The Android ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The Android ID of the device. */
public Delete setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String installId;
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm".
*/
public java.lang.String getInstallId() {
return installId;
}
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm". */
public Delete setInstallId(java.lang.String installId) {
this.installId = installId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves details of an installation of an app on a device.
*
* Create a request for the method "installs.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param installId The ID of the product represented by the install, e.g. "app:com.google.android.gm".
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String installId) throws java.io.IOException {
Get result = new Get(enterpriseId, userId, deviceId, installId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/installs/{installId}";
/**
* Retrieves details of an installation of an app on a device.
*
* Create a request for the method "installs.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param installId The ID of the product represented by the install, e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String installId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.Install.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
this.installId = com.google.api.client.util.Preconditions.checkNotNull(installId, "Required parameter installId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The Android ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The Android ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The Android ID of the device. */
public Get setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String installId;
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm".
*/
public java.lang.String getInstallId() {
return installId;
}
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm". */
public Get setInstallId(java.lang.String installId) {
this.installId = installId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the details of all apps installed on the specified device.
*
* Create a request for the method "installs.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) throws java.io.IOException {
List result = new List(enterpriseId, userId, deviceId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/installs";
/**
* Retrieves the details of all apps installed on the specified device.
*
* Create a request for the method "installs.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.InstallsListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The Android ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The Android ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The Android ID of the device. */
public List setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Requests to install the latest version of an app to a device. If the app is already installed,
* then it is updated to the latest version if necessary.
*
* Create a request for the method "installs.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param installId The ID of the product represented by the install, e.g. "app:com.google.android.gm".
* @param content the {@link com.google.api.services.androidenterprise.model.Install}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String installId, com.google.api.services.androidenterprise.model.Install content) throws java.io.IOException {
Update result = new Update(enterpriseId, userId, deviceId, installId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/installs/{installId}";
/**
* Requests to install the latest version of an app to a device. If the app is already installed,
* then it is updated to the latest version if necessary.
*
* Create a request for the method "installs.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param installId The ID of the product represented by the install, e.g. "app:com.google.android.gm".
* @param content the {@link com.google.api.services.androidenterprise.model.Install}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String installId, com.google.api.services.androidenterprise.model.Install content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.Install.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
this.installId = com.google.api.client.util.Preconditions.checkNotNull(installId, "Required parameter installId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Update setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The Android ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The Android ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The Android ID of the device. */
public Update setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String installId;
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm".
*/
public java.lang.String getInstallId() {
return installId;
}
/** The ID of the product represented by the install, e.g. "app:com.google.android.gm". */
public Update setInstallId(java.lang.String installId) {
this.installId = installId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Managedconfigurationsfordevice collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Managedconfigurationsfordevice.List request = androidenterprise.managedconfigurationsfordevice().list(parameters ...)}
*
*
* @return the resource collection
*/
public Managedconfigurationsfordevice managedconfigurationsfordevice() {
return new Managedconfigurationsfordevice();
}
/**
* The "managedconfigurationsfordevice" collection of methods.
*/
public class Managedconfigurationsfordevice {
/**
* Removes a per-device managed configuration for an app for the specified device.
*
* Create a request for the method "managedconfigurationsfordevice.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param managedConfigurationForDeviceId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String managedConfigurationForDeviceId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, userId, deviceId, managedConfigurationForDeviceId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/managedConfigurationsForDevice/{managedConfigurationForDeviceId}";
/**
* Removes a per-device managed configuration for an app for the specified device.
*
* Create a request for the method "managedconfigurationsfordevice.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param managedConfigurationForDeviceId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String managedConfigurationForDeviceId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
this.managedConfigurationForDeviceId = com.google.api.client.util.Preconditions.checkNotNull(managedConfigurationForDeviceId, "Required parameter managedConfigurationForDeviceId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The Android ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The Android ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The Android ID of the device. */
public Delete setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String managedConfigurationForDeviceId;
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getManagedConfigurationForDeviceId() {
return managedConfigurationForDeviceId;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
public Delete setManagedConfigurationForDeviceId(java.lang.String managedConfigurationForDeviceId) {
this.managedConfigurationForDeviceId = managedConfigurationForDeviceId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves details of a per-device managed configuration.
*
* Create a request for the method "managedconfigurationsfordevice.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param managedConfigurationForDeviceId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String managedConfigurationForDeviceId) throws java.io.IOException {
Get result = new Get(enterpriseId, userId, deviceId, managedConfigurationForDeviceId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/managedConfigurationsForDevice/{managedConfigurationForDeviceId}";
/**
* Retrieves details of a per-device managed configuration.
*
* Create a request for the method "managedconfigurationsfordevice.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param managedConfigurationForDeviceId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String managedConfigurationForDeviceId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ManagedConfiguration.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
this.managedConfigurationForDeviceId = com.google.api.client.util.Preconditions.checkNotNull(managedConfigurationForDeviceId, "Required parameter managedConfigurationForDeviceId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The Android ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The Android ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The Android ID of the device. */
public Get setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String managedConfigurationForDeviceId;
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getManagedConfigurationForDeviceId() {
return managedConfigurationForDeviceId;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
public Get setManagedConfigurationForDeviceId(java.lang.String managedConfigurationForDeviceId) {
this.managedConfigurationForDeviceId = managedConfigurationForDeviceId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the per-device managed configurations for the specified device. Only the ID is set.
*
* Create a request for the method "managedconfigurationsfordevice.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) throws java.io.IOException {
List result = new List(enterpriseId, userId, deviceId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/managedConfigurationsForDevice";
/**
* Lists all the per-device managed configurations for the specified device. Only the ID is set.
*
* Create a request for the method "managedconfigurationsfordevice.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ManagedConfigurationsForDeviceListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The Android ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The Android ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The Android ID of the device. */
public List setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Adds or updates a per-device managed configuration for an app for the specified device.
*
* Create a request for the method "managedconfigurationsfordevice.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param managedConfigurationForDeviceId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @param content the {@link com.google.api.services.androidenterprise.model.ManagedConfiguration}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String managedConfigurationForDeviceId, com.google.api.services.androidenterprise.model.ManagedConfiguration content) throws java.io.IOException {
Update result = new Update(enterpriseId, userId, deviceId, managedConfigurationForDeviceId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/devices/{deviceId}/managedConfigurationsForDevice/{managedConfigurationForDeviceId}";
/**
* Adds or updates a per-device managed configuration for an app for the specified device.
*
* Create a request for the method "managedconfigurationsfordevice.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param deviceId The Android ID of the device.
* @param managedConfigurationForDeviceId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @param content the {@link com.google.api.services.androidenterprise.model.ManagedConfiguration}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String deviceId, java.lang.String managedConfigurationForDeviceId, com.google.api.services.androidenterprise.model.ManagedConfiguration content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.ManagedConfiguration.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.deviceId = com.google.api.client.util.Preconditions.checkNotNull(deviceId, "Required parameter deviceId must be specified.");
this.managedConfigurationForDeviceId = com.google.api.client.util.Preconditions.checkNotNull(managedConfigurationForDeviceId, "Required parameter managedConfigurationForDeviceId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Update setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The Android ID of the device. */
@com.google.api.client.util.Key
private java.lang.String deviceId;
/** The Android ID of the device.
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/** The Android ID of the device. */
public Update setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String managedConfigurationForDeviceId;
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getManagedConfigurationForDeviceId() {
return managedConfigurationForDeviceId;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
public Update setManagedConfigurationForDeviceId(java.lang.String managedConfigurationForDeviceId) {
this.managedConfigurationForDeviceId = managedConfigurationForDeviceId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Managedconfigurationsforuser collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Managedconfigurationsforuser.List request = androidenterprise.managedconfigurationsforuser().list(parameters ...)}
*
*
* @return the resource collection
*/
public Managedconfigurationsforuser managedconfigurationsforuser() {
return new Managedconfigurationsforuser();
}
/**
* The "managedconfigurationsforuser" collection of methods.
*/
public class Managedconfigurationsforuser {
/**
* Removes a per-user managed configuration for an app for the specified user.
*
* Create a request for the method "managedconfigurationsforuser.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param managedConfigurationForUserId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String userId, java.lang.String managedConfigurationForUserId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, userId, managedConfigurationForUserId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/managedConfigurationsForUser/{managedConfigurationForUserId}";
/**
* Removes a per-user managed configuration for an app for the specified user.
*
* Create a request for the method "managedconfigurationsforuser.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param managedConfigurationForUserId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String userId, java.lang.String managedConfigurationForUserId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.managedConfigurationForUserId = com.google.api.client.util.Preconditions.checkNotNull(managedConfigurationForUserId, "Required parameter managedConfigurationForUserId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String managedConfigurationForUserId;
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getManagedConfigurationForUserId() {
return managedConfigurationForUserId;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
public Delete setManagedConfigurationForUserId(java.lang.String managedConfigurationForUserId) {
this.managedConfigurationForUserId = managedConfigurationForUserId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves details of a per-user managed configuration for an app for the specified user.
*
* Create a request for the method "managedconfigurationsforuser.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param managedConfigurationForUserId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String managedConfigurationForUserId) throws java.io.IOException {
Get result = new Get(enterpriseId, userId, managedConfigurationForUserId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/managedConfigurationsForUser/{managedConfigurationForUserId}";
/**
* Retrieves details of a per-user managed configuration for an app for the specified user.
*
* Create a request for the method "managedconfigurationsforuser.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param managedConfigurationForUserId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String userId, java.lang.String managedConfigurationForUserId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ManagedConfiguration.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.managedConfigurationForUserId = com.google.api.client.util.Preconditions.checkNotNull(managedConfigurationForUserId, "Required parameter managedConfigurationForUserId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String managedConfigurationForUserId;
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getManagedConfigurationForUserId() {
return managedConfigurationForUserId;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
public Get setManagedConfigurationForUserId(java.lang.String managedConfigurationForUserId) {
this.managedConfigurationForUserId = managedConfigurationForUserId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the per-user managed configurations for the specified user. Only the ID is set.
*
* Create a request for the method "managedconfigurationsforuser.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String userId) throws java.io.IOException {
List result = new List(enterpriseId, userId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/managedConfigurationsForUser";
/**
* Lists all the per-user managed configurations for the specified user. Only the ID is set.
*
* Create a request for the method "managedconfigurationsforuser.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String userId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ManagedConfigurationsForUserListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Adds or updates the managed configuration settings for an app for the specified user. If you
* support the Managed configurations iframe, you can apply managed configurations to a user by
* specifying an mcmId and its associated configuration variables (if any) in the request.
* Alternatively, all EMMs can apply managed configurations by passing a list of managed properties.
*
* Create a request for the method "managedconfigurationsforuser.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param managedConfigurationForUserId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @param content the {@link com.google.api.services.androidenterprise.model.ManagedConfiguration}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String managedConfigurationForUserId, com.google.api.services.androidenterprise.model.ManagedConfiguration content) throws java.io.IOException {
Update result = new Update(enterpriseId, userId, managedConfigurationForUserId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/managedConfigurationsForUser/{managedConfigurationForUserId}";
/**
* Adds or updates the managed configuration settings for an app for the specified user. If you
* support the Managed configurations iframe, you can apply managed configurations to a user by
* specifying an mcmId and its associated configuration variables (if any) in the request.
* Alternatively, all EMMs can apply managed configurations by passing a list of managed
* properties.
*
* Create a request for the method "managedconfigurationsforuser.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param managedConfigurationForUserId The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
* @param content the {@link com.google.api.services.androidenterprise.model.ManagedConfiguration}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String userId, java.lang.String managedConfigurationForUserId, com.google.api.services.androidenterprise.model.ManagedConfiguration content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.ManagedConfiguration.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.managedConfigurationForUserId = com.google.api.client.util.Preconditions.checkNotNull(managedConfigurationForUserId, "Required parameter managedConfigurationForUserId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Update setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String managedConfigurationForUserId;
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm".
*/
public java.lang.String getManagedConfigurationForUserId() {
return managedConfigurationForUserId;
}
/** The ID of the managed configuration (a product ID), e.g. "app:com.google.android.gm". */
public Update setManagedConfigurationForUserId(java.lang.String managedConfigurationForUserId) {
this.managedConfigurationForUserId = managedConfigurationForUserId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Managedconfigurationssettings collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Managedconfigurationssettings.List request = androidenterprise.managedconfigurationssettings().list(parameters ...)}
*
*
* @return the resource collection
*/
public Managedconfigurationssettings managedconfigurationssettings() {
return new Managedconfigurationssettings();
}
/**
* The "managedconfigurationssettings" collection of methods.
*/
public class Managedconfigurationssettings {
/**
* Lists all the managed configurations settings for the specified app.
*
* Create a request for the method "managedconfigurationssettings.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product for which the managed configurations settings applies to.
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String productId) throws java.io.IOException {
List result = new List(enterpriseId, productId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/products/{productId}/managedConfigurationsSettings";
/**
* Lists all the managed configurations settings for the specified app.
*
* Create a request for the method "managedconfigurationssettings.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product for which the managed configurations settings applies to.
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String productId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ManagedConfigurationsSettingsListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product for which the managed configurations settings applies to. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The ID of the product for which the managed configurations settings applies to.
*/
public java.lang.String getProductId() {
return productId;
}
/** The ID of the product for which the managed configurations settings applies to. */
public List setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Permissions collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Permissions.List request = androidenterprise.permissions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Permissions permissions() {
return new Permissions();
}
/**
* The "permissions" collection of methods.
*/
public class Permissions {
/**
* Retrieves details of an Android app permission for display to an enterprise admin.
*
* Create a request for the method "permissions.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param permissionId The ID of the permission.
* @return the request
*/
public Get get(java.lang.String permissionId) throws java.io.IOException {
Get result = new Get(permissionId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/permissions/{permissionId}";
/**
* Retrieves details of an Android app permission for display to an enterprise admin.
*
* Create a request for the method "permissions.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param permissionId The ID of the permission.
* @since 1.13
*/
protected Get(java.lang.String permissionId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.Permission.class);
this.permissionId = com.google.api.client.util.Preconditions.checkNotNull(permissionId, "Required parameter permissionId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the permission. */
@com.google.api.client.util.Key
private java.lang.String permissionId;
/** The ID of the permission.
*/
public java.lang.String getPermissionId() {
return permissionId;
}
/** The ID of the permission. */
public Get setPermissionId(java.lang.String permissionId) {
this.permissionId = permissionId;
return this;
}
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de") */
@com.google.api.client.util.Key
private java.lang.String language;
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de")
*/
public java.lang.String getLanguage() {
return language;
}
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de") */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Products collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Products.List request = androidenterprise.products().list(parameters ...)}
*
*
* @return the resource collection
*/
public Products products() {
return new Products();
}
/**
* The "products" collection of methods.
*/
public class Products {
/**
* Approves the specified product and the relevant app permissions, if any. The maximum number of
* products that you can approve per enterprise customer is 1,000. To learn how to use managed
* Google Play to design and create a store layout to display approved products to your users, see
* Store Layout Design. **Note:** This item has been deprecated. New integrations cannot use this
* method and can refer to our new recommendations.
*
* Create a request for the method "products.approve".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Approve#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @param content the {@link com.google.api.services.androidenterprise.model.ProductsApproveRequest}
* @return the request
*/
public Approve approve(java.lang.String enterpriseId, java.lang.String productId, com.google.api.services.androidenterprise.model.ProductsApproveRequest content) throws java.io.IOException {
Approve result = new Approve(enterpriseId, productId, content);
initialize(result);
return result;
}
public class Approve extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/products/{productId}/approve";
/**
* Approves the specified product and the relevant app permissions, if any. The maximum number of
* products that you can approve per enterprise customer is 1,000. To learn how to use managed
* Google Play to design and create a store layout to display approved products to your users, see
* Store Layout Design. **Note:** This item has been deprecated. New integrations cannot use this
* method and can refer to our new recommendations.
*
* Create a request for the method "products.approve".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Approve#execute()} method to invoke the remote
* operation. {@link
* Approve#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @param content the {@link com.google.api.services.androidenterprise.model.ProductsApproveRequest}
* @since 1.13
*/
protected Approve(java.lang.String enterpriseId, java.lang.String productId, com.google.api.services.androidenterprise.model.ProductsApproveRequest content) {
super(AndroidEnterprise.this, "POST", REST_PATH, content, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public Approve set$Xgafv(java.lang.String $Xgafv) {
return (Approve) super.set$Xgafv($Xgafv);
}
@Override
public Approve setAccessToken(java.lang.String accessToken) {
return (Approve) super.setAccessToken(accessToken);
}
@Override
public Approve setAlt(java.lang.String alt) {
return (Approve) super.setAlt(alt);
}
@Override
public Approve setCallback(java.lang.String callback) {
return (Approve) super.setCallback(callback);
}
@Override
public Approve setFields(java.lang.String fields) {
return (Approve) super.setFields(fields);
}
@Override
public Approve setKey(java.lang.String key) {
return (Approve) super.setKey(key);
}
@Override
public Approve setOauthToken(java.lang.String oauthToken) {
return (Approve) super.setOauthToken(oauthToken);
}
@Override
public Approve setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Approve) super.setPrettyPrint(prettyPrint);
}
@Override
public Approve setQuotaUser(java.lang.String quotaUser) {
return (Approve) super.setQuotaUser(quotaUser);
}
@Override
public Approve setUploadType(java.lang.String uploadType) {
return (Approve) super.setUploadType(uploadType);
}
@Override
public Approve setUploadProtocol(java.lang.String uploadProtocol) {
return (Approve) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Approve setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The ID of the product.
*/
public java.lang.String getProductId() {
return productId;
}
/** The ID of the product. */
public Approve setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
@Override
public Approve set(String parameterName, Object value) {
return (Approve) super.set(parameterName, value);
}
}
/**
* Generates a URL that can be rendered in an iframe to display the permissions (if any) of a
* product. An enterprise admin must view these permissions and accept them on behalf of their
* organization in order to approve that product. Admins should accept the displayed permissions by
* interacting with a separate UI element in the EMM console, which in turn should trigger the use
* of this URL as the approvalUrlInfo.approvalUrl property in a Products.approve call to approve the
* product. This URL can only be used to display permissions for up to 1 day. **Note:** This item
* has been deprecated. New integrations cannot use this method and can refer to our new
* recommendations.
*
* Create a request for the method "products.generateApprovalUrl".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GenerateApprovalUrl#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @return the request
*/
public GenerateApprovalUrl generateApprovalUrl(java.lang.String enterpriseId, java.lang.String productId) throws java.io.IOException {
GenerateApprovalUrl result = new GenerateApprovalUrl(enterpriseId, productId);
initialize(result);
return result;
}
public class GenerateApprovalUrl extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/products/{productId}/generateApprovalUrl";
/**
* Generates a URL that can be rendered in an iframe to display the permissions (if any) of a
* product. An enterprise admin must view these permissions and accept them on behalf of their
* organization in order to approve that product. Admins should accept the displayed permissions
* by interacting with a separate UI element in the EMM console, which in turn should trigger the
* use of this URL as the approvalUrlInfo.approvalUrl property in a Products.approve call to
* approve the product. This URL can only be used to display permissions for up to 1 day.
* **Note:** This item has been deprecated. New integrations cannot use this method and can refer
* to our new recommendations.
*
* Create a request for the method "products.generateApprovalUrl".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GenerateApprovalUrl#execute()} method to invoke the
* remote operation. {@link GenerateApprovalUrl#initialize(com.google.api.client.googleapis.se
* rvices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @since 1.13
*/
protected GenerateApprovalUrl(java.lang.String enterpriseId, java.lang.String productId) {
super(AndroidEnterprise.this, "POST", REST_PATH, null, com.google.api.services.androidenterprise.model.ProductsGenerateApprovalUrlResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public GenerateApprovalUrl set$Xgafv(java.lang.String $Xgafv) {
return (GenerateApprovalUrl) super.set$Xgafv($Xgafv);
}
@Override
public GenerateApprovalUrl setAccessToken(java.lang.String accessToken) {
return (GenerateApprovalUrl) super.setAccessToken(accessToken);
}
@Override
public GenerateApprovalUrl setAlt(java.lang.String alt) {
return (GenerateApprovalUrl) super.setAlt(alt);
}
@Override
public GenerateApprovalUrl setCallback(java.lang.String callback) {
return (GenerateApprovalUrl) super.setCallback(callback);
}
@Override
public GenerateApprovalUrl setFields(java.lang.String fields) {
return (GenerateApprovalUrl) super.setFields(fields);
}
@Override
public GenerateApprovalUrl setKey(java.lang.String key) {
return (GenerateApprovalUrl) super.setKey(key);
}
@Override
public GenerateApprovalUrl setOauthToken(java.lang.String oauthToken) {
return (GenerateApprovalUrl) super.setOauthToken(oauthToken);
}
@Override
public GenerateApprovalUrl setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenerateApprovalUrl) super.setPrettyPrint(prettyPrint);
}
@Override
public GenerateApprovalUrl setQuotaUser(java.lang.String quotaUser) {
return (GenerateApprovalUrl) super.setQuotaUser(quotaUser);
}
@Override
public GenerateApprovalUrl setUploadType(java.lang.String uploadType) {
return (GenerateApprovalUrl) super.setUploadType(uploadType);
}
@Override
public GenerateApprovalUrl setUploadProtocol(java.lang.String uploadProtocol) {
return (GenerateApprovalUrl) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public GenerateApprovalUrl setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The ID of the product.
*/
public java.lang.String getProductId() {
return productId;
}
/** The ID of the product. */
public GenerateApprovalUrl setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
/**
* The BCP 47 language code used for permission names and descriptions in the returned iframe,
* for instance "en-US".
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The BCP 47 language code used for permission names and descriptions in the returned iframe, for
instance "en-US".
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The BCP 47 language code used for permission names and descriptions in the returned iframe,
* for instance "en-US".
*/
public GenerateApprovalUrl setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public GenerateApprovalUrl set(String parameterName, Object value) {
return (GenerateApprovalUrl) super.set(parameterName, value);
}
}
/**
* Retrieves details of a product for display to an enterprise admin.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product, e.g. "app:com.google.android.gm".
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String productId) throws java.io.IOException {
Get result = new Get(enterpriseId, productId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/products/{productId}";
/**
* Retrieves details of a product for display to an enterprise admin.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product, e.g. "app:com.google.android.gm".
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String productId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.Product.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product, e.g. "app:com.google.android.gm". */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The ID of the product, e.g. "app:com.google.android.gm".
*/
public java.lang.String getProductId() {
return productId;
}
/** The ID of the product, e.g. "app:com.google.android.gm". */
public Get setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de"). */
@com.google.api.client.util.Key
private java.lang.String language;
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de").
*/
public java.lang.String getLanguage() {
return language;
}
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de"). */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the schema that defines the configurable properties for this product. All products have
* a schema, but this schema may be empty if no managed configurations have been defined. This
* schema can be used to populate a UI that allows an admin to configure the product. To apply a
* managed configuration based on the schema obtained using this API, see Managed Configurations
* through Play.
*
* Create a request for the method "products.getAppRestrictionsSchema".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GetAppRestrictionsSchema#execute()} method to invoke the
* remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @return the request
*/
public GetAppRestrictionsSchema getAppRestrictionsSchema(java.lang.String enterpriseId, java.lang.String productId) throws java.io.IOException {
GetAppRestrictionsSchema result = new GetAppRestrictionsSchema(enterpriseId, productId);
initialize(result);
return result;
}
public class GetAppRestrictionsSchema extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/products/{productId}/appRestrictionsSchema";
/**
* Retrieves the schema that defines the configurable properties for this product. All products
* have a schema, but this schema may be empty if no managed configurations have been defined.
* This schema can be used to populate a UI that allows an admin to configure the product. To
* apply a managed configuration based on the schema obtained using this API, see Managed
* Configurations through Play.
*
* Create a request for the method "products.getAppRestrictionsSchema".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GetAppRestrictionsSchema#execute()} method to invoke
* the remote operation. {@link GetAppRestrictionsSchema#initialize(com.google.api.client.goog
* leapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @since 1.13
*/
protected GetAppRestrictionsSchema(java.lang.String enterpriseId, java.lang.String productId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.AppRestrictionsSchema.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAppRestrictionsSchema set$Xgafv(java.lang.String $Xgafv) {
return (GetAppRestrictionsSchema) super.set$Xgafv($Xgafv);
}
@Override
public GetAppRestrictionsSchema setAccessToken(java.lang.String accessToken) {
return (GetAppRestrictionsSchema) super.setAccessToken(accessToken);
}
@Override
public GetAppRestrictionsSchema setAlt(java.lang.String alt) {
return (GetAppRestrictionsSchema) super.setAlt(alt);
}
@Override
public GetAppRestrictionsSchema setCallback(java.lang.String callback) {
return (GetAppRestrictionsSchema) super.setCallback(callback);
}
@Override
public GetAppRestrictionsSchema setFields(java.lang.String fields) {
return (GetAppRestrictionsSchema) super.setFields(fields);
}
@Override
public GetAppRestrictionsSchema setKey(java.lang.String key) {
return (GetAppRestrictionsSchema) super.setKey(key);
}
@Override
public GetAppRestrictionsSchema setOauthToken(java.lang.String oauthToken) {
return (GetAppRestrictionsSchema) super.setOauthToken(oauthToken);
}
@Override
public GetAppRestrictionsSchema setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAppRestrictionsSchema) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAppRestrictionsSchema setQuotaUser(java.lang.String quotaUser) {
return (GetAppRestrictionsSchema) super.setQuotaUser(quotaUser);
}
@Override
public GetAppRestrictionsSchema setUploadType(java.lang.String uploadType) {
return (GetAppRestrictionsSchema) super.setUploadType(uploadType);
}
@Override
public GetAppRestrictionsSchema setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAppRestrictionsSchema) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public GetAppRestrictionsSchema setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The ID of the product.
*/
public java.lang.String getProductId() {
return productId;
}
/** The ID of the product. */
public GetAppRestrictionsSchema setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de"). */
@com.google.api.client.util.Key
private java.lang.String language;
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de").
*/
public java.lang.String getLanguage() {
return language;
}
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de"). */
public GetAppRestrictionsSchema setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public GetAppRestrictionsSchema set(String parameterName, Object value) {
return (GetAppRestrictionsSchema) super.set(parameterName, value);
}
}
/**
* Retrieves the Android app permissions required by this app.
*
* Create a request for the method "products.getPermissions".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GetPermissions#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @return the request
*/
public GetPermissions getPermissions(java.lang.String enterpriseId, java.lang.String productId) throws java.io.IOException {
GetPermissions result = new GetPermissions(enterpriseId, productId);
initialize(result);
return result;
}
public class GetPermissions extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/products/{productId}/permissions";
/**
* Retrieves the Android app permissions required by this app.
*
* Create a request for the method "products.getPermissions".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GetPermissions#execute()} method to invoke the remote
* operation. {@link GetPermissions#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @since 1.13
*/
protected GetPermissions(java.lang.String enterpriseId, java.lang.String productId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ProductPermissions.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetPermissions set$Xgafv(java.lang.String $Xgafv) {
return (GetPermissions) super.set$Xgafv($Xgafv);
}
@Override
public GetPermissions setAccessToken(java.lang.String accessToken) {
return (GetPermissions) super.setAccessToken(accessToken);
}
@Override
public GetPermissions setAlt(java.lang.String alt) {
return (GetPermissions) super.setAlt(alt);
}
@Override
public GetPermissions setCallback(java.lang.String callback) {
return (GetPermissions) super.setCallback(callback);
}
@Override
public GetPermissions setFields(java.lang.String fields) {
return (GetPermissions) super.setFields(fields);
}
@Override
public GetPermissions setKey(java.lang.String key) {
return (GetPermissions) super.setKey(key);
}
@Override
public GetPermissions setOauthToken(java.lang.String oauthToken) {
return (GetPermissions) super.setOauthToken(oauthToken);
}
@Override
public GetPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public GetPermissions setQuotaUser(java.lang.String quotaUser) {
return (GetPermissions) super.setQuotaUser(quotaUser);
}
@Override
public GetPermissions setUploadType(java.lang.String uploadType) {
return (GetPermissions) super.setUploadType(uploadType);
}
@Override
public GetPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (GetPermissions) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public GetPermissions setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The ID of the product.
*/
public java.lang.String getProductId() {
return productId;
}
/** The ID of the product. */
public GetPermissions setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
@Override
public GetPermissions set(String parameterName, Object value) {
return (GetPermissions) super.set(parameterName, value);
}
}
/**
* Finds approved products that match a query, or all approved products if there is no query.
* **Note:** This item has been deprecated. New integrations cannot use this method and can refer to
* our new recommendations.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public List list(java.lang.String enterpriseId) throws java.io.IOException {
List result = new List(enterpriseId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/products";
/**
* Finds approved products that match a query, or all approved products if there is no query.
* **Note:** This item has been deprecated. New integrations cannot use this method and can refer
* to our new recommendations.
*
* Create a request for the method "products.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected List(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ProductsListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/**
* Specifies whether to search among all products (false) or among only products that have
* been approved (true). Only "true" is supported, and should be specified.
*/
@com.google.api.client.util.Key
private java.lang.Boolean approved;
/** Specifies whether to search among all products (false) or among only products that have been
approved (true). Only "true" is supported, and should be specified.
*/
public java.lang.Boolean getApproved() {
return approved;
}
/**
* Specifies whether to search among all products (false) or among only products that have
* been approved (true). Only "true" is supported, and should be specified.
*/
public List setApproved(java.lang.Boolean approved) {
this.approved = approved;
return this;
}
/**
* The BCP47 tag for the user's preferred language (e.g. "en-US", "de"). Results are returned
* in the language best matching the preferred language.
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The BCP47 tag for the user's preferred language (e.g. "en-US", "de"). Results are returned in the
language best matching the preferred language.
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The BCP47 tag for the user's preferred language (e.g. "en-US", "de"). Results are returned
* in the language best matching the preferred language.
*/
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* Defines how many results the list operation should return. The default number depends on
* the resource collection.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Defines how many results the list operation should return. The default number depends on the
resource collection.
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* Defines how many results the list operation should return. The default number depends on
* the resource collection.
*/
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The search query as typed in the Google Play store search box. If omitted, all approved
* apps will be returned (using the pagination parameters), including apps that are not
* available in the store (e.g. unpublished apps).
*/
@com.google.api.client.util.Key
private java.lang.String query;
/** The search query as typed in the Google Play store search box. If omitted, all approved apps will
be returned (using the pagination parameters), including apps that are not available in the store
(e.g. unpublished apps).
*/
public java.lang.String getQuery() {
return query;
}
/**
* The search query as typed in the Google Play store search box. If omitted, all approved
* apps will be returned (using the pagination parameters), including apps that are not
* available in the store (e.g. unpublished apps).
*/
public List setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* Defines the token of the page to return, usually taken from TokenPagination. This can only
* be used if token paging is enabled.
*/
@com.google.api.client.util.Key
private java.lang.String token;
/** Defines the token of the page to return, usually taken from TokenPagination. This can only be used
if token paging is enabled.
*/
public java.lang.String getToken() {
return token;
}
/**
* Defines the token of the page to return, usually taken from TokenPagination. This can only
* be used if token paging is enabled.
*/
public List setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Unapproves the specified product (and the relevant app permissions, if any) **Note:** This item
* has been deprecated. New integrations cannot use this method and can refer to our new
* recommendations.
*
* Create a request for the method "products.unapprove".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Unapprove#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @return the request
*/
public Unapprove unapprove(java.lang.String enterpriseId, java.lang.String productId) throws java.io.IOException {
Unapprove result = new Unapprove(enterpriseId, productId);
initialize(result);
return result;
}
public class Unapprove extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/products/{productId}/unapprove";
/**
* Unapproves the specified product (and the relevant app permissions, if any) **Note:** This item
* has been deprecated. New integrations cannot use this method and can refer to our new
* recommendations.
*
* Create a request for the method "products.unapprove".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Unapprove#execute()} method to invoke the remote
* operation. {@link
* Unapprove#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param productId The ID of the product.
* @since 1.13
*/
protected Unapprove(java.lang.String enterpriseId, java.lang.String productId) {
super(AndroidEnterprise.this, "POST", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
}
@Override
public Unapprove set$Xgafv(java.lang.String $Xgafv) {
return (Unapprove) super.set$Xgafv($Xgafv);
}
@Override
public Unapprove setAccessToken(java.lang.String accessToken) {
return (Unapprove) super.setAccessToken(accessToken);
}
@Override
public Unapprove setAlt(java.lang.String alt) {
return (Unapprove) super.setAlt(alt);
}
@Override
public Unapprove setCallback(java.lang.String callback) {
return (Unapprove) super.setCallback(callback);
}
@Override
public Unapprove setFields(java.lang.String fields) {
return (Unapprove) super.setFields(fields);
}
@Override
public Unapprove setKey(java.lang.String key) {
return (Unapprove) super.setKey(key);
}
@Override
public Unapprove setOauthToken(java.lang.String oauthToken) {
return (Unapprove) super.setOauthToken(oauthToken);
}
@Override
public Unapprove setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unapprove) super.setPrettyPrint(prettyPrint);
}
@Override
public Unapprove setQuotaUser(java.lang.String quotaUser) {
return (Unapprove) super.setQuotaUser(quotaUser);
}
@Override
public Unapprove setUploadType(java.lang.String uploadType) {
return (Unapprove) super.setUploadType(uploadType);
}
@Override
public Unapprove setUploadProtocol(java.lang.String uploadProtocol) {
return (Unapprove) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Unapprove setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the product. */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The ID of the product.
*/
public java.lang.String getProductId() {
return productId;
}
/** The ID of the product. */
public Unapprove setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
@Override
public Unapprove set(String parameterName, Object value) {
return (Unapprove) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Serviceaccountkeys collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Serviceaccountkeys.List request = androidenterprise.serviceaccountkeys().list(parameters ...)}
*
*
* @return the resource collection
*/
public Serviceaccountkeys serviceaccountkeys() {
return new Serviceaccountkeys();
}
/**
* The "serviceaccountkeys" collection of methods.
*/
public class Serviceaccountkeys {
/**
* Removes and invalidates the specified credentials for the service account associated with this
* enterprise. The calling service account must have been retrieved by calling
* Enterprises.GetServiceAccount and must have been set as the enterprise service account by calling
* Enterprises.SetAccount.
*
* Create a request for the method "serviceaccountkeys.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param keyId The ID of the key.
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String keyId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, keyId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/serviceAccountKeys/{keyId}";
/**
* Removes and invalidates the specified credentials for the service account associated with this
* enterprise. The calling service account must have been retrieved by calling
* Enterprises.GetServiceAccount and must have been set as the enterprise service account by
* calling Enterprises.SetAccount.
*
* Create a request for the method "serviceaccountkeys.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param keyId The ID of the key.
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String keyId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.keyId = com.google.api.client.util.Preconditions.checkNotNull(keyId, "Required parameter keyId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the key. */
@com.google.api.client.util.Key
private java.lang.String keyId;
/** The ID of the key.
*/
public java.lang.String getKeyId() {
return keyId;
}
/** The ID of the key. */
public Delete setKeyId(java.lang.String keyId) {
this.keyId = keyId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Generates new credentials for the service account associated with this enterprise. The calling
* service account must have been retrieved by calling Enterprises.GetServiceAccount and must have
* been set as the enterprise service account by calling Enterprises.SetAccount. Only the type of
* the key should be populated in the resource to be inserted.
*
* Create a request for the method "serviceaccountkeys.insert".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.ServiceAccountKey}
* @return the request
*/
public Insert insert(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.ServiceAccountKey content) throws java.io.IOException {
Insert result = new Insert(enterpriseId, content);
initialize(result);
return result;
}
public class Insert extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/serviceAccountKeys";
/**
* Generates new credentials for the service account associated with this enterprise. The calling
* service account must have been retrieved by calling Enterprises.GetServiceAccount and must have
* been set as the enterprise service account by calling Enterprises.SetAccount. Only the type of
* the key should be populated in the resource to be inserted.
*
* Create a request for the method "serviceaccountkeys.insert".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Insert#execute()} method to invoke the remote
* operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.ServiceAccountKey}
* @since 1.13
*/
protected Insert(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.ServiceAccountKey content) {
super(AndroidEnterprise.this, "POST", REST_PATH, content, com.google.api.services.androidenterprise.model.ServiceAccountKey.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getType(), "ServiceAccountKey.getType()");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Insert setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists all active credentials for the service account associated with this enterprise. Only the ID
* and key type are returned. The calling service account must have been retrieved by calling
* Enterprises.GetServiceAccount and must have been set as the enterprise service account by calling
* Enterprises.SetAccount.
*
* Create a request for the method "serviceaccountkeys.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public List list(java.lang.String enterpriseId) throws java.io.IOException {
List result = new List(enterpriseId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/serviceAccountKeys";
/**
* Lists all active credentials for the service account associated with this enterprise. Only the
* ID and key type are returned. The calling service account must have been retrieved by calling
* Enterprises.GetServiceAccount and must have been set as the enterprise service account by
* calling Enterprises.SetAccount.
*
* Create a request for the method "serviceaccountkeys.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected List(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ServiceAccountKeysListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Storelayoutclusters collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Storelayoutclusters.List request = androidenterprise.storelayoutclusters().list(parameters ...)}
*
*
* @return the resource collection
*/
public Storelayoutclusters storelayoutclusters() {
return new Storelayoutclusters();
}
/**
* The "storelayoutclusters" collection of methods.
*/
public class Storelayoutclusters {
/**
* Deletes a cluster.
*
* Create a request for the method "storelayoutclusters.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param clusterId The ID of the cluster.
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String pageId, java.lang.String clusterId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, pageId, clusterId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages/{pageId}/clusters/{clusterId}";
/**
* Deletes a cluster.
*
* Create a request for the method "storelayoutclusters.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param clusterId The ID of the cluster.
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String pageId, java.lang.String clusterId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.pageId = com.google.api.client.util.Preconditions.checkNotNull(pageId, "Required parameter pageId must be specified.");
this.clusterId = com.google.api.client.util.Preconditions.checkNotNull(clusterId, "Required parameter clusterId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the page. */
@com.google.api.client.util.Key
private java.lang.String pageId;
/** The ID of the page.
*/
public java.lang.String getPageId() {
return pageId;
}
/** The ID of the page. */
public Delete setPageId(java.lang.String pageId) {
this.pageId = pageId;
return this;
}
/** The ID of the cluster. */
@com.google.api.client.util.Key
private java.lang.String clusterId;
/** The ID of the cluster.
*/
public java.lang.String getClusterId() {
return clusterId;
}
/** The ID of the cluster. */
public Delete setClusterId(java.lang.String clusterId) {
this.clusterId = clusterId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves details of a cluster.
*
* Create a request for the method "storelayoutclusters.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param clusterId The ID of the cluster.
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String pageId, java.lang.String clusterId) throws java.io.IOException {
Get result = new Get(enterpriseId, pageId, clusterId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages/{pageId}/clusters/{clusterId}";
/**
* Retrieves details of a cluster.
*
* Create a request for the method "storelayoutclusters.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param clusterId The ID of the cluster.
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String pageId, java.lang.String clusterId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.StoreCluster.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.pageId = com.google.api.client.util.Preconditions.checkNotNull(pageId, "Required parameter pageId must be specified.");
this.clusterId = com.google.api.client.util.Preconditions.checkNotNull(clusterId, "Required parameter clusterId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the page. */
@com.google.api.client.util.Key
private java.lang.String pageId;
/** The ID of the page.
*/
public java.lang.String getPageId() {
return pageId;
}
/** The ID of the page. */
public Get setPageId(java.lang.String pageId) {
this.pageId = pageId;
return this;
}
/** The ID of the cluster. */
@com.google.api.client.util.Key
private java.lang.String clusterId;
/** The ID of the cluster.
*/
public java.lang.String getClusterId() {
return clusterId;
}
/** The ID of the cluster. */
public Get setClusterId(java.lang.String clusterId) {
this.clusterId = clusterId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new cluster in a page.
*
* Create a request for the method "storelayoutclusters.insert".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param content the {@link com.google.api.services.androidenterprise.model.StoreCluster}
* @return the request
*/
public Insert insert(java.lang.String enterpriseId, java.lang.String pageId, com.google.api.services.androidenterprise.model.StoreCluster content) throws java.io.IOException {
Insert result = new Insert(enterpriseId, pageId, content);
initialize(result);
return result;
}
public class Insert extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages/{pageId}/clusters";
/**
* Inserts a new cluster in a page.
*
* Create a request for the method "storelayoutclusters.insert".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Insert#execute()} method to invoke the remote
* operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param content the {@link com.google.api.services.androidenterprise.model.StoreCluster}
* @since 1.13
*/
protected Insert(java.lang.String enterpriseId, java.lang.String pageId, com.google.api.services.androidenterprise.model.StoreCluster content) {
super(AndroidEnterprise.this, "POST", REST_PATH, content, com.google.api.services.androidenterprise.model.StoreCluster.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.pageId = com.google.api.client.util.Preconditions.checkNotNull(pageId, "Required parameter pageId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Insert setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the page. */
@com.google.api.client.util.Key
private java.lang.String pageId;
/** The ID of the page.
*/
public java.lang.String getPageId() {
return pageId;
}
/** The ID of the page. */
public Insert setPageId(java.lang.String pageId) {
this.pageId = pageId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves the details of all clusters on the specified page.
*
* Create a request for the method "storelayoutclusters.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String pageId) throws java.io.IOException {
List result = new List(enterpriseId, pageId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages/{pageId}/clusters";
/**
* Retrieves the details of all clusters on the specified page.
*
* Create a request for the method "storelayoutclusters.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String pageId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.StoreLayoutClustersListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.pageId = com.google.api.client.util.Preconditions.checkNotNull(pageId, "Required parameter pageId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the page. */
@com.google.api.client.util.Key
private java.lang.String pageId;
/** The ID of the page.
*/
public java.lang.String getPageId() {
return pageId;
}
/** The ID of the page. */
public List setPageId(java.lang.String pageId) {
this.pageId = pageId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a cluster.
*
* Create a request for the method "storelayoutclusters.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param clusterId The ID of the cluster.
* @param content the {@link com.google.api.services.androidenterprise.model.StoreCluster}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String pageId, java.lang.String clusterId, com.google.api.services.androidenterprise.model.StoreCluster content) throws java.io.IOException {
Update result = new Update(enterpriseId, pageId, clusterId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages/{pageId}/clusters/{clusterId}";
/**
* Updates a cluster.
*
* Create a request for the method "storelayoutclusters.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param clusterId The ID of the cluster.
* @param content the {@link com.google.api.services.androidenterprise.model.StoreCluster}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String pageId, java.lang.String clusterId, com.google.api.services.androidenterprise.model.StoreCluster content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.StoreCluster.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.pageId = com.google.api.client.util.Preconditions.checkNotNull(pageId, "Required parameter pageId must be specified.");
this.clusterId = com.google.api.client.util.Preconditions.checkNotNull(clusterId, "Required parameter clusterId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the page. */
@com.google.api.client.util.Key
private java.lang.String pageId;
/** The ID of the page.
*/
public java.lang.String getPageId() {
return pageId;
}
/** The ID of the page. */
public Update setPageId(java.lang.String pageId) {
this.pageId = pageId;
return this;
}
/** The ID of the cluster. */
@com.google.api.client.util.Key
private java.lang.String clusterId;
/** The ID of the cluster.
*/
public java.lang.String getClusterId() {
return clusterId;
}
/** The ID of the cluster. */
public Update setClusterId(java.lang.String clusterId) {
this.clusterId = clusterId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Storelayoutpages collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Storelayoutpages.List request = androidenterprise.storelayoutpages().list(parameters ...)}
*
*
* @return the resource collection
*/
public Storelayoutpages storelayoutpages() {
return new Storelayoutpages();
}
/**
* The "storelayoutpages" collection of methods.
*/
public class Storelayoutpages {
/**
* Deletes a store page.
*
* Create a request for the method "storelayoutpages.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String pageId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, pageId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages/{pageId}";
/**
* Deletes a store page.
*
* Create a request for the method "storelayoutpages.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String pageId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.pageId = com.google.api.client.util.Preconditions.checkNotNull(pageId, "Required parameter pageId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the page. */
@com.google.api.client.util.Key
private java.lang.String pageId;
/** The ID of the page.
*/
public java.lang.String getPageId() {
return pageId;
}
/** The ID of the page. */
public Delete setPageId(java.lang.String pageId) {
this.pageId = pageId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves details of a store page.
*
* Create a request for the method "storelayoutpages.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String pageId) throws java.io.IOException {
Get result = new Get(enterpriseId, pageId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages/{pageId}";
/**
* Retrieves details of a store page.
*
* Create a request for the method "storelayoutpages.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String pageId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.StorePage.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.pageId = com.google.api.client.util.Preconditions.checkNotNull(pageId, "Required parameter pageId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the page. */
@com.google.api.client.util.Key
private java.lang.String pageId;
/** The ID of the page.
*/
public java.lang.String getPageId() {
return pageId;
}
/** The ID of the page. */
public Get setPageId(java.lang.String pageId) {
this.pageId = pageId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Inserts a new store page.
*
* Create a request for the method "storelayoutpages.insert".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.StorePage}
* @return the request
*/
public Insert insert(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.StorePage content) throws java.io.IOException {
Insert result = new Insert(enterpriseId, content);
initialize(result);
return result;
}
public class Insert extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages";
/**
* Inserts a new store page.
*
* Create a request for the method "storelayoutpages.insert".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Insert#execute()} method to invoke the remote
* operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.StorePage}
* @since 1.13
*/
protected Insert(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.StorePage content) {
super(AndroidEnterprise.this, "POST", REST_PATH, content, com.google.api.services.androidenterprise.model.StorePage.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Insert setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves the details of all pages in the store.
*
* Create a request for the method "storelayoutpages.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public List list(java.lang.String enterpriseId) throws java.io.IOException {
List result = new List(enterpriseId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages";
/**
* Retrieves the details of all pages in the store.
*
* Create a request for the method "storelayoutpages.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected List(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.StoreLayoutPagesListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the content of a store page.
*
* Create a request for the method "storelayoutpages.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param content the {@link com.google.api.services.androidenterprise.model.StorePage}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String pageId, com.google.api.services.androidenterprise.model.StorePage content) throws java.io.IOException {
Update result = new Update(enterpriseId, pageId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/storeLayout/pages/{pageId}";
/**
* Updates the content of a store page.
*
* Create a request for the method "storelayoutpages.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param pageId The ID of the page.
* @param content the {@link com.google.api.services.androidenterprise.model.StorePage}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String pageId, com.google.api.services.androidenterprise.model.StorePage content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.StorePage.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.pageId = com.google.api.client.util.Preconditions.checkNotNull(pageId, "Required parameter pageId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the page. */
@com.google.api.client.util.Key
private java.lang.String pageId;
/** The ID of the page.
*/
public java.lang.String getPageId() {
return pageId;
}
/** The ID of the page. */
public Update setPageId(java.lang.String pageId) {
this.pageId = pageId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Users collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Users.List request = androidenterprise.users().list(parameters ...)}
*
*
* @return the resource collection
*/
public Users users() {
return new Users();
}
/**
* The "users" collection of methods.
*/
public class Users {
/**
* Deleted an EMM-managed user.
*
* Create a request for the method "users.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String userId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, userId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}";
/**
* Deleted an EMM-managed user.
*
* Create a request for the method "users.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String userId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Generates an authentication token which the device policy client can use to provision the given
* EMM-managed user account on a device. The generated token is single-use and expires after a few
* minutes. You can provision a maximum of 10 devices per user. This call only works with EMM-
* managed accounts.
*
* Create a request for the method "users.generateAuthenticationToken".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GenerateAuthenticationToken#execute()} method to invoke the
* remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @return the request
*/
public GenerateAuthenticationToken generateAuthenticationToken(java.lang.String enterpriseId, java.lang.String userId) throws java.io.IOException {
GenerateAuthenticationToken result = new GenerateAuthenticationToken(enterpriseId, userId);
initialize(result);
return result;
}
public class GenerateAuthenticationToken extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/authenticationToken";
/**
* Generates an authentication token which the device policy client can use to provision the given
* EMM-managed user account on a device. The generated token is single-use and expires after a few
* minutes. You can provision a maximum of 10 devices per user. This call only works with EMM-
* managed accounts.
*
* Create a request for the method "users.generateAuthenticationToken".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GenerateAuthenticationToken#execute()} method to
* invoke the remote operation. {@link GenerateAuthenticationToken#initialize(com.google.api.c
* lient.googleapis.services.AbstractGoogleClientRequest)} must be called to initialize this
* instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @since 1.13
*/
protected GenerateAuthenticationToken(java.lang.String enterpriseId, java.lang.String userId) {
super(AndroidEnterprise.this, "POST", REST_PATH, null, com.google.api.services.androidenterprise.model.AuthenticationToken.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public GenerateAuthenticationToken set$Xgafv(java.lang.String $Xgafv) {
return (GenerateAuthenticationToken) super.set$Xgafv($Xgafv);
}
@Override
public GenerateAuthenticationToken setAccessToken(java.lang.String accessToken) {
return (GenerateAuthenticationToken) super.setAccessToken(accessToken);
}
@Override
public GenerateAuthenticationToken setAlt(java.lang.String alt) {
return (GenerateAuthenticationToken) super.setAlt(alt);
}
@Override
public GenerateAuthenticationToken setCallback(java.lang.String callback) {
return (GenerateAuthenticationToken) super.setCallback(callback);
}
@Override
public GenerateAuthenticationToken setFields(java.lang.String fields) {
return (GenerateAuthenticationToken) super.setFields(fields);
}
@Override
public GenerateAuthenticationToken setKey(java.lang.String key) {
return (GenerateAuthenticationToken) super.setKey(key);
}
@Override
public GenerateAuthenticationToken setOauthToken(java.lang.String oauthToken) {
return (GenerateAuthenticationToken) super.setOauthToken(oauthToken);
}
@Override
public GenerateAuthenticationToken setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenerateAuthenticationToken) super.setPrettyPrint(prettyPrint);
}
@Override
public GenerateAuthenticationToken setQuotaUser(java.lang.String quotaUser) {
return (GenerateAuthenticationToken) super.setQuotaUser(quotaUser);
}
@Override
public GenerateAuthenticationToken setUploadType(java.lang.String uploadType) {
return (GenerateAuthenticationToken) super.setUploadType(uploadType);
}
@Override
public GenerateAuthenticationToken setUploadProtocol(java.lang.String uploadProtocol) {
return (GenerateAuthenticationToken) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public GenerateAuthenticationToken setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public GenerateAuthenticationToken setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public GenerateAuthenticationToken set(String parameterName, Object value) {
return (GenerateAuthenticationToken) super.set(parameterName, value);
}
}
/**
* Retrieves a user's details.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String userId) throws java.io.IOException {
Get result = new Get(enterpriseId, userId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}";
/**
* Retrieves a user's details.
*
* Create a request for the method "users.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String userId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.User.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the set of products a user is entitled to access. **Note:** This item has been
* deprecated. New integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "users.getAvailableProductSet".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link GetAvailableProductSet#execute()} method to invoke the
* remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @return the request
*/
public GetAvailableProductSet getAvailableProductSet(java.lang.String enterpriseId, java.lang.String userId) throws java.io.IOException {
GetAvailableProductSet result = new GetAvailableProductSet(enterpriseId, userId);
initialize(result);
return result;
}
public class GetAvailableProductSet extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/availableProductSet";
/**
* Retrieves the set of products a user is entitled to access. **Note:** This item has been
* deprecated. New integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "users.getAvailableProductSet".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link GetAvailableProductSet#execute()} method to invoke the
* remote operation. {@link GetAvailableProductSet#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @since 1.13
*/
protected GetAvailableProductSet(java.lang.String enterpriseId, java.lang.String userId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.ProductSet.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetAvailableProductSet set$Xgafv(java.lang.String $Xgafv) {
return (GetAvailableProductSet) super.set$Xgafv($Xgafv);
}
@Override
public GetAvailableProductSet setAccessToken(java.lang.String accessToken) {
return (GetAvailableProductSet) super.setAccessToken(accessToken);
}
@Override
public GetAvailableProductSet setAlt(java.lang.String alt) {
return (GetAvailableProductSet) super.setAlt(alt);
}
@Override
public GetAvailableProductSet setCallback(java.lang.String callback) {
return (GetAvailableProductSet) super.setCallback(callback);
}
@Override
public GetAvailableProductSet setFields(java.lang.String fields) {
return (GetAvailableProductSet) super.setFields(fields);
}
@Override
public GetAvailableProductSet setKey(java.lang.String key) {
return (GetAvailableProductSet) super.setKey(key);
}
@Override
public GetAvailableProductSet setOauthToken(java.lang.String oauthToken) {
return (GetAvailableProductSet) super.setOauthToken(oauthToken);
}
@Override
public GetAvailableProductSet setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetAvailableProductSet) super.setPrettyPrint(prettyPrint);
}
@Override
public GetAvailableProductSet setQuotaUser(java.lang.String quotaUser) {
return (GetAvailableProductSet) super.setQuotaUser(quotaUser);
}
@Override
public GetAvailableProductSet setUploadType(java.lang.String uploadType) {
return (GetAvailableProductSet) super.setUploadType(uploadType);
}
@Override
public GetAvailableProductSet setUploadProtocol(java.lang.String uploadProtocol) {
return (GetAvailableProductSet) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public GetAvailableProductSet setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public GetAvailableProductSet setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public GetAvailableProductSet set(String parameterName, Object value) {
return (GetAvailableProductSet) super.set(parameterName, value);
}
}
/**
* Creates a new EMM-managed user. The Users resource passed in the body of the request should
* include an accountIdentifier and an accountType. If a corresponding user already exists with the
* same account identifier, the user will be updated with the resource. In this case only the
* displayName field can be changed.
*
* Create a request for the method "users.insert".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.User}
* @return the request
*/
public Insert insert(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.User content) throws java.io.IOException {
Insert result = new Insert(enterpriseId, content);
initialize(result);
return result;
}
public class Insert extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users";
/**
* Creates a new EMM-managed user. The Users resource passed in the body of the request should
* include an accountIdentifier and an accountType. If a corresponding user already exists with
* the same account identifier, the user will be updated with the resource. In this case only the
* displayName field can be changed.
*
* Create a request for the method "users.insert".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Insert#execute()} method to invoke the remote
* operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.User}
* @since 1.13
*/
protected Insert(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.User content) {
super(AndroidEnterprise.this, "POST", REST_PATH, content, com.google.api.services.androidenterprise.model.User.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getAccountIdentifier(), "User.getAccountIdentifier()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getAccountType(), "User.getAccountType()");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Insert setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Looks up a user by primary email address. This is only supported for Google-managed users. Lookup
* of the id is not needed for EMM-managed users because the id is already returned in the result of
* the Users.insert call.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param email Required. The exact primary email address of the user to look up.
* @return the request
*/
public List list(java.lang.String enterpriseId, java.lang.String email) throws java.io.IOException {
List result = new List(enterpriseId, email);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users";
/**
* Looks up a user by primary email address. This is only supported for Google-managed users.
* Lookup of the id is not needed for EMM-managed users because the id is already returned in the
* result of the Users.insert call.
*
* Create a request for the method "users.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param email Required. The exact primary email address of the user to look up.
* @since 1.13
*/
protected List(java.lang.String enterpriseId, java.lang.String email) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.UsersListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.email = com.google.api.client.util.Preconditions.checkNotNull(email, "Required parameter email must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** Required. The exact primary email address of the user to look up. */
@com.google.api.client.util.Key
private java.lang.String email;
/** Required. The exact primary email address of the user to look up.
*/
public java.lang.String getEmail() {
return email;
}
/** Required. The exact primary email address of the user to look up. */
public List setEmail(java.lang.String email) {
this.email = email;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Revokes access to all devices currently provisioned to the user. The user will no longer be able
* to use the managed Play store on any of their managed devices. This call only works with EMM-
* managed accounts.
*
* Create a request for the method "users.revokeDeviceAccess".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link RevokeDeviceAccess#execute()} method to invoke the remote
* operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @return the request
*/
public RevokeDeviceAccess revokeDeviceAccess(java.lang.String enterpriseId, java.lang.String userId) throws java.io.IOException {
RevokeDeviceAccess result = new RevokeDeviceAccess(enterpriseId, userId);
initialize(result);
return result;
}
public class RevokeDeviceAccess extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/deviceAccess";
/**
* Revokes access to all devices currently provisioned to the user. The user will no longer be
* able to use the managed Play store on any of their managed devices. This call only works with
* EMM-managed accounts.
*
* Create a request for the method "users.revokeDeviceAccess".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link RevokeDeviceAccess#execute()} method to invoke the
* remote operation. {@link RevokeDeviceAccess#initialize(com.google.api.client.googleapis.ser
* vices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @since 1.13
*/
protected RevokeDeviceAccess(java.lang.String enterpriseId, java.lang.String userId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public RevokeDeviceAccess set$Xgafv(java.lang.String $Xgafv) {
return (RevokeDeviceAccess) super.set$Xgafv($Xgafv);
}
@Override
public RevokeDeviceAccess setAccessToken(java.lang.String accessToken) {
return (RevokeDeviceAccess) super.setAccessToken(accessToken);
}
@Override
public RevokeDeviceAccess setAlt(java.lang.String alt) {
return (RevokeDeviceAccess) super.setAlt(alt);
}
@Override
public RevokeDeviceAccess setCallback(java.lang.String callback) {
return (RevokeDeviceAccess) super.setCallback(callback);
}
@Override
public RevokeDeviceAccess setFields(java.lang.String fields) {
return (RevokeDeviceAccess) super.setFields(fields);
}
@Override
public RevokeDeviceAccess setKey(java.lang.String key) {
return (RevokeDeviceAccess) super.setKey(key);
}
@Override
public RevokeDeviceAccess setOauthToken(java.lang.String oauthToken) {
return (RevokeDeviceAccess) super.setOauthToken(oauthToken);
}
@Override
public RevokeDeviceAccess setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RevokeDeviceAccess) super.setPrettyPrint(prettyPrint);
}
@Override
public RevokeDeviceAccess setQuotaUser(java.lang.String quotaUser) {
return (RevokeDeviceAccess) super.setQuotaUser(quotaUser);
}
@Override
public RevokeDeviceAccess setUploadType(java.lang.String uploadType) {
return (RevokeDeviceAccess) super.setUploadType(uploadType);
}
@Override
public RevokeDeviceAccess setUploadProtocol(java.lang.String uploadProtocol) {
return (RevokeDeviceAccess) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public RevokeDeviceAccess setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public RevokeDeviceAccess setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public RevokeDeviceAccess set(String parameterName, Object value) {
return (RevokeDeviceAccess) super.set(parameterName, value);
}
}
/**
* Modifies the set of products that a user is entitled to access (referred to as *whitelisted*
* products). Only products that are approved or products that were previously approved (products
* with revoked approval) can be whitelisted. **Note:** This item has been deprecated. New
* integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "users.setAvailableProductSet".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link SetAvailableProductSet#execute()} method to invoke the
* remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param content the {@link com.google.api.services.androidenterprise.model.ProductSet}
* @return the request
*/
public SetAvailableProductSet setAvailableProductSet(java.lang.String enterpriseId, java.lang.String userId, com.google.api.services.androidenterprise.model.ProductSet content) throws java.io.IOException {
SetAvailableProductSet result = new SetAvailableProductSet(enterpriseId, userId, content);
initialize(result);
return result;
}
public class SetAvailableProductSet extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}/availableProductSet";
/**
* Modifies the set of products that a user is entitled to access (referred to as *whitelisted*
* products). Only products that are approved or products that were previously approved (products
* with revoked approval) can be whitelisted. **Note:** This item has been deprecated. New
* integrations cannot use this method and can refer to our new recommendations.
*
* Create a request for the method "users.setAvailableProductSet".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link SetAvailableProductSet#execute()} method to invoke the
* remote operation. {@link SetAvailableProductSet#initialize(com.google.api.client.googleapis
* .services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param content the {@link com.google.api.services.androidenterprise.model.ProductSet}
* @since 1.13
*/
protected SetAvailableProductSet(java.lang.String enterpriseId, java.lang.String userId, com.google.api.services.androidenterprise.model.ProductSet content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.ProductSet.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public SetAvailableProductSet set$Xgafv(java.lang.String $Xgafv) {
return (SetAvailableProductSet) super.set$Xgafv($Xgafv);
}
@Override
public SetAvailableProductSet setAccessToken(java.lang.String accessToken) {
return (SetAvailableProductSet) super.setAccessToken(accessToken);
}
@Override
public SetAvailableProductSet setAlt(java.lang.String alt) {
return (SetAvailableProductSet) super.setAlt(alt);
}
@Override
public SetAvailableProductSet setCallback(java.lang.String callback) {
return (SetAvailableProductSet) super.setCallback(callback);
}
@Override
public SetAvailableProductSet setFields(java.lang.String fields) {
return (SetAvailableProductSet) super.setFields(fields);
}
@Override
public SetAvailableProductSet setKey(java.lang.String key) {
return (SetAvailableProductSet) super.setKey(key);
}
@Override
public SetAvailableProductSet setOauthToken(java.lang.String oauthToken) {
return (SetAvailableProductSet) super.setOauthToken(oauthToken);
}
@Override
public SetAvailableProductSet setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetAvailableProductSet) super.setPrettyPrint(prettyPrint);
}
@Override
public SetAvailableProductSet setQuotaUser(java.lang.String quotaUser) {
return (SetAvailableProductSet) super.setQuotaUser(quotaUser);
}
@Override
public SetAvailableProductSet setUploadType(java.lang.String uploadType) {
return (SetAvailableProductSet) super.setUploadType(uploadType);
}
@Override
public SetAvailableProductSet setUploadProtocol(java.lang.String uploadProtocol) {
return (SetAvailableProductSet) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public SetAvailableProductSet setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public SetAvailableProductSet setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public SetAvailableProductSet set(String parameterName, Object value) {
return (SetAvailableProductSet) super.set(parameterName, value);
}
}
/**
* Updates the details of an EMM-managed user. Can be used with EMM-managed users only (not Google
* managed users). Pass the new details in the Users resource in the request body. Only the
* displayName field can be changed. Other fields must either be unset or have the currently active
* value.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param content the {@link com.google.api.services.androidenterprise.model.User}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String userId, com.google.api.services.androidenterprise.model.User content) throws java.io.IOException {
Update result = new Update(enterpriseId, userId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/users/{userId}";
/**
* Updates the details of an EMM-managed user. Can be used with EMM-managed users only (not Google
* managed users). Pass the new details in the Users resource in the request body. Only the
* displayName field can be changed. Other fields must either be unset or have the currently
* active value.
*
* Create a request for the method "users.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param userId The ID of the user.
* @param content the {@link com.google.api.services.androidenterprise.model.User}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String userId, com.google.api.services.androidenterprise.model.User content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.User.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the user. */
@com.google.api.client.util.Key
private java.lang.String userId;
/** The ID of the user.
*/
public java.lang.String getUserId() {
return userId;
}
/** The ID of the user. */
public Update setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Webapps collection.
*
* The typical use is:
*
* {@code AndroidEnterprise androidenterprise = new AndroidEnterprise(...);}
* {@code AndroidEnterprise.Webapps.List request = androidenterprise.webapps().list(parameters ...)}
*
*
* @return the resource collection
*/
public Webapps webapps() {
return new Webapps();
}
/**
* The "webapps" collection of methods.
*/
public class Webapps {
/**
* Deletes an existing web app.
*
* Create a request for the method "webapps.delete".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param webAppId The ID of the web app.
* @return the request
*/
public Delete delete(java.lang.String enterpriseId, java.lang.String webAppId) throws java.io.IOException {
Delete result = new Delete(enterpriseId, webAppId);
initialize(result);
return result;
}
public class Delete extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/webApps/{webAppId}";
/**
* Deletes an existing web app.
*
* Create a request for the method "webapps.delete".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param webAppId The ID of the web app.
* @since 1.13
*/
protected Delete(java.lang.String enterpriseId, java.lang.String webAppId) {
super(AndroidEnterprise.this, "DELETE", REST_PATH, null, Void.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.webAppId = com.google.api.client.util.Preconditions.checkNotNull(webAppId, "Required parameter webAppId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Delete setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the web app. */
@com.google.api.client.util.Key
private java.lang.String webAppId;
/** The ID of the web app.
*/
public java.lang.String getWebAppId() {
return webAppId;
}
/** The ID of the web app. */
public Delete setWebAppId(java.lang.String webAppId) {
this.webAppId = webAppId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an existing web app.
*
* Create a request for the method "webapps.get".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param webAppId The ID of the web app.
* @return the request
*/
public Get get(java.lang.String enterpriseId, java.lang.String webAppId) throws java.io.IOException {
Get result = new Get(enterpriseId, webAppId);
initialize(result);
return result;
}
public class Get extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/webApps/{webAppId}";
/**
* Gets an existing web app.
*
* Create a request for the method "webapps.get".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param webAppId The ID of the web app.
* @since 1.13
*/
protected Get(java.lang.String enterpriseId, java.lang.String webAppId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.WebApp.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.webAppId = com.google.api.client.util.Preconditions.checkNotNull(webAppId, "Required parameter webAppId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Get setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the web app. */
@com.google.api.client.util.Key
private java.lang.String webAppId;
/** The ID of the web app.
*/
public java.lang.String getWebAppId() {
return webAppId;
}
/** The ID of the web app. */
public Get setWebAppId(java.lang.String webAppId) {
this.webAppId = webAppId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a new web app for the enterprise.
*
* Create a request for the method "webapps.insert".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.WebApp}
* @return the request
*/
public Insert insert(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.WebApp content) throws java.io.IOException {
Insert result = new Insert(enterpriseId, content);
initialize(result);
return result;
}
public class Insert extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/webApps";
/**
* Creates a new web app for the enterprise.
*
* Create a request for the method "webapps.insert".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Insert#execute()} method to invoke the remote
* operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param content the {@link com.google.api.services.androidenterprise.model.WebApp}
* @since 1.13
*/
protected Insert(java.lang.String enterpriseId, com.google.api.services.androidenterprise.model.WebApp content) {
super(AndroidEnterprise.this, "POST", REST_PATH, content, com.google.api.services.androidenterprise.model.WebApp.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public Insert set$Xgafv(java.lang.String $Xgafv) {
return (Insert) super.set$Xgafv($Xgafv);
}
@Override
public Insert setAccessToken(java.lang.String accessToken) {
return (Insert) super.setAccessToken(accessToken);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setCallback(java.lang.String callback) {
return (Insert) super.setCallback(callback);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUploadType(java.lang.String uploadType) {
return (Insert) super.setUploadType(uploadType);
}
@Override
public Insert setUploadProtocol(java.lang.String uploadProtocol) {
return (Insert) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Insert setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Retrieves the details of all web apps for a given enterprise.
*
* Create a request for the method "webapps.list".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @return the request
*/
public List list(java.lang.String enterpriseId) throws java.io.IOException {
List result = new List(enterpriseId);
initialize(result);
return result;
}
public class List extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/webApps";
/**
* Retrieves the details of all web apps for a given enterprise.
*
* Create a request for the method "webapps.list".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @since 1.13
*/
protected List(java.lang.String enterpriseId) {
super(AndroidEnterprise.this, "GET", REST_PATH, null, com.google.api.services.androidenterprise.model.WebAppsListResponse.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public List setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an existing web app.
*
* Create a request for the method "webapps.update".
*
* This request holds the parameters needed by the androidenterprise server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param enterpriseId The ID of the enterprise.
* @param webAppId The ID of the web app.
* @param content the {@link com.google.api.services.androidenterprise.model.WebApp}
* @return the request
*/
public Update update(java.lang.String enterpriseId, java.lang.String webAppId, com.google.api.services.androidenterprise.model.WebApp content) throws java.io.IOException {
Update result = new Update(enterpriseId, webAppId, content);
initialize(result);
return result;
}
public class Update extends AndroidEnterpriseRequest {
private static final String REST_PATH = "androidenterprise/v1/enterprises/{enterpriseId}/webApps/{webAppId}";
/**
* Updates an existing web app.
*
* Create a request for the method "webapps.update".
*
* This request holds the parameters needed by the the androidenterprise server. After setting
* any optional parameters, call the {@link Update#execute()} method to invoke the remote
* operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param enterpriseId The ID of the enterprise.
* @param webAppId The ID of the web app.
* @param content the {@link com.google.api.services.androidenterprise.model.WebApp}
* @since 1.13
*/
protected Update(java.lang.String enterpriseId, java.lang.String webAppId, com.google.api.services.androidenterprise.model.WebApp content) {
super(AndroidEnterprise.this, "PUT", REST_PATH, content, com.google.api.services.androidenterprise.model.WebApp.class);
this.enterpriseId = com.google.api.client.util.Preconditions.checkNotNull(enterpriseId, "Required parameter enterpriseId must be specified.");
this.webAppId = com.google.api.client.util.Preconditions.checkNotNull(webAppId, "Required parameter webAppId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the enterprise. */
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/** The ID of the enterprise.
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/** The ID of the enterprise. */
public Update setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/** The ID of the web app. */
@com.google.api.client.util.Key
private java.lang.String webAppId;
/** The ID of the web app.
*/
public java.lang.String getWebAppId() {
return webAppId;
}
/** The ID of the web app. */
public Update setWebAppId(java.lang.String webAppId) {
this.webAppId = webAppId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link AndroidEnterprise}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link AndroidEnterprise}. */
@Override
public AndroidEnterprise build() {
return new AndroidEnterprise(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AndroidEnterpriseRequestInitializer}.
*
* @since 1.12
*/
public Builder setAndroidEnterpriseRequestInitializer(
AndroidEnterpriseRequestInitializer androidenterpriseRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(androidenterpriseRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}