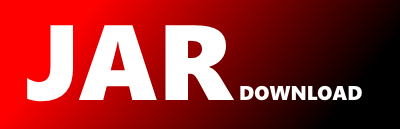
com.google.api.services.androidenterprise.model.AppRestrictionsSchemaRestriction Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidenterprise.model;
/**
* A restriction in the App Restriction Schema represents a piece of configuration that may be pre-
* applied.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Play EMM API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AppRestrictionsSchemaRestriction extends com.google.api.client.json.GenericJson {
/**
* The default value of the restriction. bundle and bundleArray restrictions never have a default
* value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AppRestrictionsSchemaRestrictionRestrictionValue defaultValue;
/**
* A longer description of the restriction, giving more detail of what it affects.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* For choice or multiselect restrictions, the list of possible entries' human-readable names.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("entry")
private java.util.List entry__;
/**
* For choice or multiselect restrictions, the list of possible entries' machine-readable values.
* These values should be used in the configuration, either as a single string value for a choice
* restriction or in a stringArray for a multiselect restriction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List entryValue;
/**
* The unique key that the product uses to identify the restriction, e.g.
* "com.google.android.gm.fieldname".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String key;
/**
* For bundle or bundleArray restrictions, the list of nested restrictions. A bundle restriction
* is always nested within a bundleArray restriction, and a bundleArray restriction is at most two
* levels deep.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List nestedRestriction;
/**
* The type of the restriction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String restrictionType;
/**
* The name of the restriction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* The default value of the restriction. bundle and bundleArray restrictions never have a default
* value.
* @return value or {@code null} for none
*/
public AppRestrictionsSchemaRestrictionRestrictionValue getDefaultValue() {
return defaultValue;
}
/**
* The default value of the restriction. bundle and bundleArray restrictions never have a default
* value.
* @param defaultValue defaultValue or {@code null} for none
*/
public AppRestrictionsSchemaRestriction setDefaultValue(AppRestrictionsSchemaRestrictionRestrictionValue defaultValue) {
this.defaultValue = defaultValue;
return this;
}
/**
* A longer description of the restriction, giving more detail of what it affects.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* A longer description of the restriction, giving more detail of what it affects.
* @param description description or {@code null} for none
*/
public AppRestrictionsSchemaRestriction setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* For choice or multiselect restrictions, the list of possible entries' human-readable names.
* @return value or {@code null} for none
*/
public java.util.List getEntry() {
return entry__;
}
/**
* For choice or multiselect restrictions, the list of possible entries' human-readable names.
* @param entry__ entry__ or {@code null} for none
*/
public AppRestrictionsSchemaRestriction setEntry(java.util.List entry__) {
this.entry__ = entry__;
return this;
}
/**
* For choice or multiselect restrictions, the list of possible entries' machine-readable values.
* These values should be used in the configuration, either as a single string value for a choice
* restriction or in a stringArray for a multiselect restriction.
* @return value or {@code null} for none
*/
public java.util.List getEntryValue() {
return entryValue;
}
/**
* For choice or multiselect restrictions, the list of possible entries' machine-readable values.
* These values should be used in the configuration, either as a single string value for a choice
* restriction or in a stringArray for a multiselect restriction.
* @param entryValue entryValue or {@code null} for none
*/
public AppRestrictionsSchemaRestriction setEntryValue(java.util.List entryValue) {
this.entryValue = entryValue;
return this;
}
/**
* The unique key that the product uses to identify the restriction, e.g.
* "com.google.android.gm.fieldname".
* @return value or {@code null} for none
*/
public java.lang.String getKey() {
return key;
}
/**
* The unique key that the product uses to identify the restriction, e.g.
* "com.google.android.gm.fieldname".
* @param key key or {@code null} for none
*/
public AppRestrictionsSchemaRestriction setKey(java.lang.String key) {
this.key = key;
return this;
}
/**
* For bundle or bundleArray restrictions, the list of nested restrictions. A bundle restriction
* is always nested within a bundleArray restriction, and a bundleArray restriction is at most two
* levels deep.
* @return value or {@code null} for none
*/
public java.util.List getNestedRestriction() {
return nestedRestriction;
}
/**
* For bundle or bundleArray restrictions, the list of nested restrictions. A bundle restriction
* is always nested within a bundleArray restriction, and a bundleArray restriction is at most two
* levels deep.
* @param nestedRestriction nestedRestriction or {@code null} for none
*/
public AppRestrictionsSchemaRestriction setNestedRestriction(java.util.List nestedRestriction) {
this.nestedRestriction = nestedRestriction;
return this;
}
/**
* The type of the restriction.
* @return value or {@code null} for none
*/
public java.lang.String getRestrictionType() {
return restrictionType;
}
/**
* The type of the restriction.
* @param restrictionType restrictionType or {@code null} for none
*/
public AppRestrictionsSchemaRestriction setRestrictionType(java.lang.String restrictionType) {
this.restrictionType = restrictionType;
return this;
}
/**
* The name of the restriction.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* The name of the restriction.
* @param title title or {@code null} for none
*/
public AppRestrictionsSchemaRestriction setTitle(java.lang.String title) {
this.title = title;
return this;
}
@Override
public AppRestrictionsSchemaRestriction set(String fieldName, Object value) {
return (AppRestrictionsSchemaRestriction) super.set(fieldName, value);
}
@Override
public AppRestrictionsSchemaRestriction clone() {
return (AppRestrictionsSchemaRestriction) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy