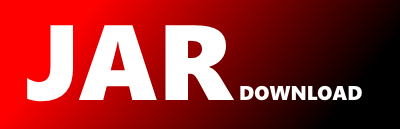
com.google.api.services.androidenterprise.model.Device Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidenterprise.model;
/**
* A Devices resource represents a mobile device managed by the EMM and belonging to a specific
* enterprise user.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Play EMM API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Device extends com.google.api.client.json.GenericJson {
/**
* The Google Play Services Android ID for the device encoded as a lowercase hex string. For
* example, "123456789abcdef0".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String androidId;
/**
* The internal hardware codename of the device. This comes from android.os.Build.DEVICE. (field
* named "device" per logs/wireless/android/android_checkin.proto)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String device;
/**
* The build fingerprint of the device if known.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String latestBuildFingerprint;
/**
* The manufacturer of the device. This comes from android.os.Build.MANUFACTURER.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String maker;
/**
* Identifies the extent to which the device is controlled by a managed Google Play EMM in various
* deployment configurations. Possible values include: - "managedDevice", a device that has the
* EMM's device policy controller (DPC) as the device owner. - "managedProfile", a device that has
* a profile managed by the DPC (DPC is profile owner) in addition to a separate, personal profile
* that is unavailable to the DPC. - "containerApp", no longer used (deprecated). -
* "unmanagedProfile", a device that has been allowed (by the domain's admin, using the Admin
* Console to enable the privilege) to use managed Google Play, but the profile is itself not
* owned by a DPC.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String managementType;
/**
* The model name of the device. This comes from android.os.Build.MODEL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String model;
/**
* The policy enforced on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Policy policy;
/**
* The product name of the device. This comes from android.os.Build.PRODUCT.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String product;
/**
* The device report updated with the latest app states.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceReport report;
/**
* Retail brand for the device, if set. See android.os.Build.BRAND
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String retailBrand;
/**
* API compatibility version.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer sdkVersion;
/**
* The Google Play Services Android ID for the device encoded as a lowercase hex string. For
* example, "123456789abcdef0".
* @return value or {@code null} for none
*/
public java.lang.String getAndroidId() {
return androidId;
}
/**
* The Google Play Services Android ID for the device encoded as a lowercase hex string. For
* example, "123456789abcdef0".
* @param androidId androidId or {@code null} for none
*/
public Device setAndroidId(java.lang.String androidId) {
this.androidId = androidId;
return this;
}
/**
* The internal hardware codename of the device. This comes from android.os.Build.DEVICE. (field
* named "device" per logs/wireless/android/android_checkin.proto)
* @return value or {@code null} for none
*/
public java.lang.String getDevice() {
return device;
}
/**
* The internal hardware codename of the device. This comes from android.os.Build.DEVICE. (field
* named "device" per logs/wireless/android/android_checkin.proto)
* @param device device or {@code null} for none
*/
public Device setDevice(java.lang.String device) {
this.device = device;
return this;
}
/**
* The build fingerprint of the device if known.
* @return value or {@code null} for none
*/
public java.lang.String getLatestBuildFingerprint() {
return latestBuildFingerprint;
}
/**
* The build fingerprint of the device if known.
* @param latestBuildFingerprint latestBuildFingerprint or {@code null} for none
*/
public Device setLatestBuildFingerprint(java.lang.String latestBuildFingerprint) {
this.latestBuildFingerprint = latestBuildFingerprint;
return this;
}
/**
* The manufacturer of the device. This comes from android.os.Build.MANUFACTURER.
* @return value or {@code null} for none
*/
public java.lang.String getMaker() {
return maker;
}
/**
* The manufacturer of the device. This comes from android.os.Build.MANUFACTURER.
* @param maker maker or {@code null} for none
*/
public Device setMaker(java.lang.String maker) {
this.maker = maker;
return this;
}
/**
* Identifies the extent to which the device is controlled by a managed Google Play EMM in various
* deployment configurations. Possible values include: - "managedDevice", a device that has the
* EMM's device policy controller (DPC) as the device owner. - "managedProfile", a device that has
* a profile managed by the DPC (DPC is profile owner) in addition to a separate, personal profile
* that is unavailable to the DPC. - "containerApp", no longer used (deprecated). -
* "unmanagedProfile", a device that has been allowed (by the domain's admin, using the Admin
* Console to enable the privilege) to use managed Google Play, but the profile is itself not
* owned by a DPC.
* @return value or {@code null} for none
*/
public java.lang.String getManagementType() {
return managementType;
}
/**
* Identifies the extent to which the device is controlled by a managed Google Play EMM in various
* deployment configurations. Possible values include: - "managedDevice", a device that has the
* EMM's device policy controller (DPC) as the device owner. - "managedProfile", a device that has
* a profile managed by the DPC (DPC is profile owner) in addition to a separate, personal profile
* that is unavailable to the DPC. - "containerApp", no longer used (deprecated). -
* "unmanagedProfile", a device that has been allowed (by the domain's admin, using the Admin
* Console to enable the privilege) to use managed Google Play, but the profile is itself not
* owned by a DPC.
* @param managementType managementType or {@code null} for none
*/
public Device setManagementType(java.lang.String managementType) {
this.managementType = managementType;
return this;
}
/**
* The model name of the device. This comes from android.os.Build.MODEL.
* @return value or {@code null} for none
*/
public java.lang.String getModel() {
return model;
}
/**
* The model name of the device. This comes from android.os.Build.MODEL.
* @param model model or {@code null} for none
*/
public Device setModel(java.lang.String model) {
this.model = model;
return this;
}
/**
* The policy enforced on the device.
* @return value or {@code null} for none
*/
public Policy getPolicy() {
return policy;
}
/**
* The policy enforced on the device.
* @param policy policy or {@code null} for none
*/
public Device setPolicy(Policy policy) {
this.policy = policy;
return this;
}
/**
* The product name of the device. This comes from android.os.Build.PRODUCT.
* @return value or {@code null} for none
*/
public java.lang.String getProduct() {
return product;
}
/**
* The product name of the device. This comes from android.os.Build.PRODUCT.
* @param product product or {@code null} for none
*/
public Device setProduct(java.lang.String product) {
this.product = product;
return this;
}
/**
* The device report updated with the latest app states.
* @return value or {@code null} for none
*/
public DeviceReport getReport() {
return report;
}
/**
* The device report updated with the latest app states.
* @param report report or {@code null} for none
*/
public Device setReport(DeviceReport report) {
this.report = report;
return this;
}
/**
* Retail brand for the device, if set. See android.os.Build.BRAND
* @return value or {@code null} for none
*/
public java.lang.String getRetailBrand() {
return retailBrand;
}
/**
* Retail brand for the device, if set. See android.os.Build.BRAND
* @param retailBrand retailBrand or {@code null} for none
*/
public Device setRetailBrand(java.lang.String retailBrand) {
this.retailBrand = retailBrand;
return this;
}
/**
* API compatibility version.
* @return value or {@code null} for none
*/
public java.lang.Integer getSdkVersion() {
return sdkVersion;
}
/**
* API compatibility version.
* @param sdkVersion sdkVersion or {@code null} for none
*/
public Device setSdkVersion(java.lang.Integer sdkVersion) {
this.sdkVersion = sdkVersion;
return this;
}
@Override
public Device set(String fieldName, Object value) {
return (Device) super.set(fieldName, value);
}
@Override
public Device clone() {
return (Device) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy