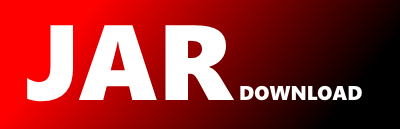
com.google.api.services.androidenterprise.model.Notification Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidenterprise.model;
/**
* A notification of one event relating to an enterprise.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Play EMM API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Notification extends com.google.api.client.json.GenericJson {
/**
* Notifications about new app restrictions schema changes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AppRestrictionsSchemaChangeEvent appRestrictionsSchemaChangeEvent;
/**
* Notifications about app updates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AppUpdateEvent appUpdateEvent;
/**
* Notifications about device report updates.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DeviceReportUpdateEvent deviceReportUpdateEvent;
/**
* The ID of the enterprise for which the notification is sent. This will always be present.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String enterpriseId;
/**
* Notifications about an app installation failure.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private InstallFailureEvent installFailureEvent;
/**
* Notifications about new devices.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NewDeviceEvent newDeviceEvent;
/**
* Notifications about new app permissions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NewPermissionsEvent newPermissionsEvent;
/**
* Type of the notification.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String notificationType;
/**
* Notifications about changes to a product's approval status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductApprovalEvent productApprovalEvent;
/**
* Notifications about product availability changes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ProductAvailabilityChangeEvent productAvailabilityChangeEvent;
/**
* The time when the notification was published in milliseconds since 1970-01-01T00:00:00Z. This
* will always be present.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long timestampMillis;
/**
* Notifications about new app restrictions schema changes.
* @return value or {@code null} for none
*/
public AppRestrictionsSchemaChangeEvent getAppRestrictionsSchemaChangeEvent() {
return appRestrictionsSchemaChangeEvent;
}
/**
* Notifications about new app restrictions schema changes.
* @param appRestrictionsSchemaChangeEvent appRestrictionsSchemaChangeEvent or {@code null} for none
*/
public Notification setAppRestrictionsSchemaChangeEvent(AppRestrictionsSchemaChangeEvent appRestrictionsSchemaChangeEvent) {
this.appRestrictionsSchemaChangeEvent = appRestrictionsSchemaChangeEvent;
return this;
}
/**
* Notifications about app updates.
* @return value or {@code null} for none
*/
public AppUpdateEvent getAppUpdateEvent() {
return appUpdateEvent;
}
/**
* Notifications about app updates.
* @param appUpdateEvent appUpdateEvent or {@code null} for none
*/
public Notification setAppUpdateEvent(AppUpdateEvent appUpdateEvent) {
this.appUpdateEvent = appUpdateEvent;
return this;
}
/**
* Notifications about device report updates.
* @return value or {@code null} for none
*/
public DeviceReportUpdateEvent getDeviceReportUpdateEvent() {
return deviceReportUpdateEvent;
}
/**
* Notifications about device report updates.
* @param deviceReportUpdateEvent deviceReportUpdateEvent or {@code null} for none
*/
public Notification setDeviceReportUpdateEvent(DeviceReportUpdateEvent deviceReportUpdateEvent) {
this.deviceReportUpdateEvent = deviceReportUpdateEvent;
return this;
}
/**
* The ID of the enterprise for which the notification is sent. This will always be present.
* @return value or {@code null} for none
*/
public java.lang.String getEnterpriseId() {
return enterpriseId;
}
/**
* The ID of the enterprise for which the notification is sent. This will always be present.
* @param enterpriseId enterpriseId or {@code null} for none
*/
public Notification setEnterpriseId(java.lang.String enterpriseId) {
this.enterpriseId = enterpriseId;
return this;
}
/**
* Notifications about an app installation failure.
* @return value or {@code null} for none
*/
public InstallFailureEvent getInstallFailureEvent() {
return installFailureEvent;
}
/**
* Notifications about an app installation failure.
* @param installFailureEvent installFailureEvent or {@code null} for none
*/
public Notification setInstallFailureEvent(InstallFailureEvent installFailureEvent) {
this.installFailureEvent = installFailureEvent;
return this;
}
/**
* Notifications about new devices.
* @return value or {@code null} for none
*/
public NewDeviceEvent getNewDeviceEvent() {
return newDeviceEvent;
}
/**
* Notifications about new devices.
* @param newDeviceEvent newDeviceEvent or {@code null} for none
*/
public Notification setNewDeviceEvent(NewDeviceEvent newDeviceEvent) {
this.newDeviceEvent = newDeviceEvent;
return this;
}
/**
* Notifications about new app permissions.
* @return value or {@code null} for none
*/
public NewPermissionsEvent getNewPermissionsEvent() {
return newPermissionsEvent;
}
/**
* Notifications about new app permissions.
* @param newPermissionsEvent newPermissionsEvent or {@code null} for none
*/
public Notification setNewPermissionsEvent(NewPermissionsEvent newPermissionsEvent) {
this.newPermissionsEvent = newPermissionsEvent;
return this;
}
/**
* Type of the notification.
* @return value or {@code null} for none
*/
public java.lang.String getNotificationType() {
return notificationType;
}
/**
* Type of the notification.
* @param notificationType notificationType or {@code null} for none
*/
public Notification setNotificationType(java.lang.String notificationType) {
this.notificationType = notificationType;
return this;
}
/**
* Notifications about changes to a product's approval status.
* @return value or {@code null} for none
*/
public ProductApprovalEvent getProductApprovalEvent() {
return productApprovalEvent;
}
/**
* Notifications about changes to a product's approval status.
* @param productApprovalEvent productApprovalEvent or {@code null} for none
*/
public Notification setProductApprovalEvent(ProductApprovalEvent productApprovalEvent) {
this.productApprovalEvent = productApprovalEvent;
return this;
}
/**
* Notifications about product availability changes.
* @return value or {@code null} for none
*/
public ProductAvailabilityChangeEvent getProductAvailabilityChangeEvent() {
return productAvailabilityChangeEvent;
}
/**
* Notifications about product availability changes.
* @param productAvailabilityChangeEvent productAvailabilityChangeEvent or {@code null} for none
*/
public Notification setProductAvailabilityChangeEvent(ProductAvailabilityChangeEvent productAvailabilityChangeEvent) {
this.productAvailabilityChangeEvent = productAvailabilityChangeEvent;
return this;
}
/**
* The time when the notification was published in milliseconds since 1970-01-01T00:00:00Z. This
* will always be present.
* @return value or {@code null} for none
*/
public java.lang.Long getTimestampMillis() {
return timestampMillis;
}
/**
* The time when the notification was published in milliseconds since 1970-01-01T00:00:00Z. This
* will always be present.
* @param timestampMillis timestampMillis or {@code null} for none
*/
public Notification setTimestampMillis(java.lang.Long timestampMillis) {
this.timestampMillis = timestampMillis;
return this;
}
@Override
public Notification set(String fieldName, Object value) {
return (Notification) super.set(fieldName, value);
}
@Override
public Notification clone() {
return (Notification) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy