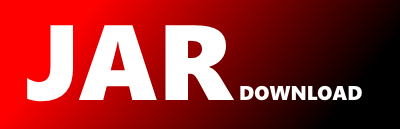
target.apidocs.com.google.api.services.androidenterprise.model.Device.html Maven / Gradle / Ivy
The newest version!
Device (Google Play EMM API v1-rev20241113-2.0.0)
com.google.api.services.androidenterprise.model
Class Device
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.androidenterprise.model.Device
-
public final class Device
extends com.google.api.client.json.GenericJson
A Devices resource represents a mobile device managed by the EMM and belonging to a specific
enterprise user.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Google Play EMM API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Device()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Device
clone()
String
getAndroidId()
The Google Play Services Android ID for the device encoded as a lowercase hex string.
String
getDevice()
The internal hardware codename of the device.
String
getLatestBuildFingerprint()
The build fingerprint of the device if known.
String
getMaker()
The manufacturer of the device.
String
getManagementType()
Identifies the extent to which the device is controlled by a managed Google Play EMM in various
deployment configurations.
String
getModel()
The model name of the device.
Policy
getPolicy()
The policy enforced on the device.
String
getProduct()
The product name of the device.
DeviceReport
getReport()
The device report updated with the latest app states.
String
getRetailBrand()
Retail brand for the device, if set.
Integer
getSdkVersion()
API compatibility version.
Device
set(String fieldName,
Object value)
Device
setAndroidId(String androidId)
The Google Play Services Android ID for the device encoded as a lowercase hex string.
Device
setDevice(String device)
The internal hardware codename of the device.
Device
setLatestBuildFingerprint(String latestBuildFingerprint)
The build fingerprint of the device if known.
Device
setMaker(String maker)
The manufacturer of the device.
Device
setManagementType(String managementType)
Identifies the extent to which the device is controlled by a managed Google Play EMM in various
deployment configurations.
Device
setModel(String model)
The model name of the device.
Device
setPolicy(Policy policy)
The policy enforced on the device.
Device
setProduct(String product)
The product name of the device.
Device
setReport(DeviceReport report)
The device report updated with the latest app states.
Device
setRetailBrand(String retailBrand)
Retail brand for the device, if set.
Device
setSdkVersion(Integer sdkVersion)
API compatibility version.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAndroidId
public String getAndroidId()
The Google Play Services Android ID for the device encoded as a lowercase hex string. For
example, "123456789abcdef0".
- Returns:
- value or
null
for none
-
setAndroidId
public Device setAndroidId(String androidId)
The Google Play Services Android ID for the device encoded as a lowercase hex string. For
example, "123456789abcdef0".
- Parameters:
androidId
- androidId or null
for none
-
getDevice
public String getDevice()
The internal hardware codename of the device. This comes from android.os.Build.DEVICE. (field
named "device" per logs/wireless/android/android_checkin.proto)
- Returns:
- value or
null
for none
-
setDevice
public Device setDevice(String device)
The internal hardware codename of the device. This comes from android.os.Build.DEVICE. (field
named "device" per logs/wireless/android/android_checkin.proto)
- Parameters:
device
- device or null
for none
-
getLatestBuildFingerprint
public String getLatestBuildFingerprint()
The build fingerprint of the device if known.
- Returns:
- value or
null
for none
-
setLatestBuildFingerprint
public Device setLatestBuildFingerprint(String latestBuildFingerprint)
The build fingerprint of the device if known.
- Parameters:
latestBuildFingerprint
- latestBuildFingerprint or null
for none
-
getMaker
public String getMaker()
The manufacturer of the device. This comes from android.os.Build.MANUFACTURER.
- Returns:
- value or
null
for none
-
setMaker
public Device setMaker(String maker)
The manufacturer of the device. This comes from android.os.Build.MANUFACTURER.
- Parameters:
maker
- maker or null
for none
-
getManagementType
public String getManagementType()
Identifies the extent to which the device is controlled by a managed Google Play EMM in various
deployment configurations. Possible values include: - "managedDevice", a device that has the
EMM's device policy controller (DPC) as the device owner. - "managedProfile", a device that has
a profile managed by the DPC (DPC is profile owner) in addition to a separate, personal profile
that is unavailable to the DPC. - "containerApp", no longer used (deprecated). -
"unmanagedProfile", a device that has been allowed (by the domain's admin, using the Admin
Console to enable the privilege) to use managed Google Play, but the profile is itself not
owned by a DPC.
- Returns:
- value or
null
for none
-
setManagementType
public Device setManagementType(String managementType)
Identifies the extent to which the device is controlled by a managed Google Play EMM in various
deployment configurations. Possible values include: - "managedDevice", a device that has the
EMM's device policy controller (DPC) as the device owner. - "managedProfile", a device that has
a profile managed by the DPC (DPC is profile owner) in addition to a separate, personal profile
that is unavailable to the DPC. - "containerApp", no longer used (deprecated). -
"unmanagedProfile", a device that has been allowed (by the domain's admin, using the Admin
Console to enable the privilege) to use managed Google Play, but the profile is itself not
owned by a DPC.
- Parameters:
managementType
- managementType or null
for none
-
getModel
public String getModel()
The model name of the device. This comes from android.os.Build.MODEL.
- Returns:
- value or
null
for none
-
setModel
public Device setModel(String model)
The model name of the device. This comes from android.os.Build.MODEL.
- Parameters:
model
- model or null
for none
-
getPolicy
public Policy getPolicy()
The policy enforced on the device.
- Returns:
- value or
null
for none
-
setPolicy
public Device setPolicy(Policy policy)
The policy enforced on the device.
- Parameters:
policy
- policy or null
for none
-
getProduct
public String getProduct()
The product name of the device. This comes from android.os.Build.PRODUCT.
- Returns:
- value or
null
for none
-
setProduct
public Device setProduct(String product)
The product name of the device. This comes from android.os.Build.PRODUCT.
- Parameters:
product
- product or null
for none
-
getReport
public DeviceReport getReport()
The device report updated with the latest app states.
- Returns:
- value or
null
for none
-
setReport
public Device setReport(DeviceReport report)
The device report updated with the latest app states.
- Parameters:
report
- report or null
for none
-
getRetailBrand
public String getRetailBrand()
Retail brand for the device, if set. See android.os.Build.BRAND
- Returns:
- value or
null
for none
-
setRetailBrand
public Device setRetailBrand(String retailBrand)
Retail brand for the device, if set. See android.os.Build.BRAND
- Parameters:
retailBrand
- retailBrand or null
for none
-
getSdkVersion
public Integer getSdkVersion()
API compatibility version.
- Returns:
- value or
null
for none
-
setSdkVersion
public Device setSdkVersion(Integer sdkVersion)
API compatibility version.
- Parameters:
sdkVersion
- sdkVersion or null
for none
-
set
public Device set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Device clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy