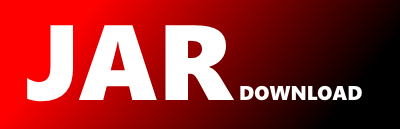
com.google.api.services.androidmanagement.v1.AndroidManagement Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1;
/**
* Service definition for AndroidManagement (v1).
*
*
* The Android Management API provides remote enterprise management of Android devices and apps.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AndroidManagementRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class AndroidManagement extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Android Management API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://androidmanagement.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://androidmanagement.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public AndroidManagement(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
AndroidManagement(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Enterprises collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.Enterprises.List request = androidmanagement.enterprises().list(parameters ...)}
*
*
* @return the resource collection
*/
public Enterprises enterprises() {
return new Enterprises();
}
/**
* The "enterprises" collection of methods.
*/
public class Enterprises {
/**
* Creates an enterprise. This is the last step in the enterprise signup flow. See also:
* SigninDetail
*
* Create a request for the method "enterprises.create".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Enterprise}
* @return the request
*/
public Create create(com.google.api.services.androidmanagement.v1.model.Enterprise content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends AndroidManagementRequest {
private static final String REST_PATH = "v1/enterprises";
/**
* Creates an enterprise. This is the last step in the enterprise signup flow. See also:
* SigninDetail
*
* Create a request for the method "enterprises.create".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Enterprise}
* @since 1.13
*/
protected Create(com.google.api.services.androidmanagement.v1.model.Enterprise content) {
super(AndroidManagement.this, "POST", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.Enterprise.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Whether the enterprise admin has seen and agreed to the managed Google Play Agreement
* (https://www.android.com/enterprise/terms/). Do not set this field for any customer-managed
* enterprise (https://developers.google.com/android/management/create-enterprise#customer-
* managed_enterprises). Set this to field to true for all EMM-managed enterprises
* (https://developers.google.com/android/management/create-enterprise#emm-
* managed_enterprises).
*/
@com.google.api.client.util.Key
private java.lang.Boolean agreementAccepted;
/** Whether the enterprise admin has seen and agreed to the managed Google Play Agreement
(https://www.android.com/enterprise/terms/). Do not set this field for any customer-managed
enterprise (https://developers.google.com/android/management/create-enterprise#customer-
managed_enterprises). Set this to field to true for all EMM-managed enterprises
(https://developers.google.com/android/management/create-enterprise#emm-managed_enterprises).
*/
public java.lang.Boolean getAgreementAccepted() {
return agreementAccepted;
}
/**
* Whether the enterprise admin has seen and agreed to the managed Google Play Agreement
* (https://www.android.com/enterprise/terms/). Do not set this field for any customer-managed
* enterprise (https://developers.google.com/android/management/create-enterprise#customer-
* managed_enterprises). Set this to field to true for all EMM-managed enterprises
* (https://developers.google.com/android/management/create-enterprise#emm-
* managed_enterprises).
*/
public Create setAgreementAccepted(java.lang.Boolean agreementAccepted) {
this.agreementAccepted = agreementAccepted;
return this;
}
/**
* The enterprise token appended to the callback URL. Set this when creating a customer-
* managed enterprise (https://developers.google.com/android/management/create-
* enterprise#customer-managed_enterprises) and not when creating a deprecated EMM-managed
* enterprise (https://developers.google.com/android/management/create-enterprise#emm-
* managed_enterprises).
*/
@com.google.api.client.util.Key
private java.lang.String enterpriseToken;
/** The enterprise token appended to the callback URL. Set this when creating a customer-managed
enterprise (https://developers.google.com/android/management/create-enterprise#customer-
managed_enterprises) and not when creating a deprecated EMM-managed enterprise
(https://developers.google.com/android/management/create-enterprise#emm-managed_enterprises).
*/
public java.lang.String getEnterpriseToken() {
return enterpriseToken;
}
/**
* The enterprise token appended to the callback URL. Set this when creating a customer-
* managed enterprise (https://developers.google.com/android/management/create-
* enterprise#customer-managed_enterprises) and not when creating a deprecated EMM-managed
* enterprise (https://developers.google.com/android/management/create-enterprise#emm-
* managed_enterprises).
*/
public Create setEnterpriseToken(java.lang.String enterpriseToken) {
this.enterpriseToken = enterpriseToken;
return this;
}
/** The ID of the Google Cloud Platform project which will own the enterprise. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of the Google Cloud Platform project which will own the enterprise.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The ID of the Google Cloud Platform project which will own the enterprise. */
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* The name of the SignupUrl used to sign up for the enterprise. Set this when creating a
* customer-managed enterprise (https://developers.google.com/android/management/create-
* enterprise#customer-managed_enterprises) and not when creating a deprecated EMM-managed
* enterprise (https://developers.google.com/android/management/create-enterprise#emm-
* managed_enterprises).
*/
@com.google.api.client.util.Key
private java.lang.String signupUrlName;
/** The name of the SignupUrl used to sign up for the enterprise. Set this when creating a customer-
managed enterprise (https://developers.google.com/android/management/create-enterprise#customer-
managed_enterprises) and not when creating a deprecated EMM-managed enterprise
(https://developers.google.com/android/management/create-enterprise#emm-managed_enterprises).
*/
public java.lang.String getSignupUrlName() {
return signupUrlName;
}
/**
* The name of the SignupUrl used to sign up for the enterprise. Set this when creating a
* customer-managed enterprise (https://developers.google.com/android/management/create-
* enterprise#customer-managed_enterprises) and not when creating a deprecated EMM-managed
* enterprise (https://developers.google.com/android/management/create-enterprise#emm-
* managed_enterprises).
*/
public Create setSignupUrlName(java.lang.String signupUrlName) {
this.signupUrlName = signupUrlName;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Permanently deletes an enterprise and all accounts and data associated with it. Warning: this
* will result in a cascaded deletion of all AM API devices associated with the deleted enterprise.
* Only available for EMM-managed enterprises.
*
* Create a request for the method "enterprises.delete".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the enterprise in the form enterprises/{enterpriseId}.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Permanently deletes an enterprise and all accounts and data associated with it. Warning: this
* will result in a cascaded deletion of all AM API devices associated with the deleted
* enterprise. Only available for EMM-managed enterprises.
*
* Create a request for the method "enterprises.delete".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the enterprise in the form enterprises/{enterpriseId}.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AndroidManagement.this, "DELETE", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an enterprise.
*
* Create a request for the method "enterprises.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the enterprise in the form enterprises/{enterpriseId}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Gets an enterprise.
*
* Create a request for the method "enterprises.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the enterprise in the form enterprises/{enterpriseId}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Enterprise.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists EMM-managed enterprises. Only BASIC fields are returned.
*
* Create a request for the method "enterprises.list".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends AndroidManagementRequest {
private static final String REST_PATH = "v1/enterprises";
/**
* Lists EMM-managed enterprises. Only BASIC fields are returned.
*
* Create a request for the method "enterprises.list".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.ListEnterprisesResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The requested page size. The actual page size may be fixed to a min or max value. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The requested page size. The actual page size may be fixed to a min or max value.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The requested page size. The actual page size may be fixed to a min or max value. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying a page of results returned by the server. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results returned by the server.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying a page of results returned by the server. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Required. The Cloud project ID of the EMM managing the enterprises. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The Cloud project ID of the EMM managing the enterprises.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The Cloud project ID of the EMM managing the enterprises. */
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/** Specifies which Enterprise fields to return. This method only supports BASIC. */
@com.google.api.client.util.Key
private java.lang.String view;
/** Specifies which Enterprise fields to return. This method only supports BASIC.
*/
public java.lang.String getView() {
return view;
}
/** Specifies which Enterprise fields to return. This method only supports BASIC. */
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates an enterprise. See also: SigninDetail
*
* Create a request for the method "enterprises.patch".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The name of the enterprise in the form enterprises/{enterpriseId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Enterprise}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.androidmanagement.v1.model.Enterprise content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Updates an enterprise. See also: SigninDetail
*
* Create a request for the method "enterprises.patch".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the enterprise in the form enterprises/{enterpriseId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Enterprise}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.androidmanagement.v1.model.Enterprise content) {
super(AndroidManagement.this, "PATCH", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.Enterprise.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.name = name;
return this;
}
/**
* The field mask indicating the fields to update. If not set, all modifiable fields will be
* modified.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The field mask indicating the fields to update. If not set, all modifiable fields will be modified.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The field mask indicating the fields to update. If not set, all modifiable fields will be
* modified.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Applications collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.Applications.List request = androidmanagement.applications().list(parameters ...)}
*
*
* @return the resource collection
*/
public Applications applications() {
return new Applications();
}
/**
* The "applications" collection of methods.
*/
public class Applications {
/**
* Gets info about an application.
*
* Create a request for the method "applications.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the application in the form enterprises/{enterpriseId}/applications/{package_name}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/applications/[^/]+$");
/**
* Gets info about an application.
*
* Create a request for the method "applications.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the application in the form enterprises/{enterpriseId}/applications/{package_name}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Application.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/applications/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the application in the form
* enterprises/{enterpriseId}/applications/{package_name}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the application in the form enterprises/{enterpriseId}/applications/{package_name}.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the application in the form
* enterprises/{enterpriseId}/applications/{package_name}.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/applications/[^/]+$");
}
this.name = name;
return this;
}
/**
* The preferred language for localized application info, as a BCP47 tag (e.g. "en-US",
* "de"). If not specified the default language of the application will be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The preferred language for localized application info, as a BCP47 tag (e.g. "en-US", "de"). If not
specified the default language of the application will be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The preferred language for localized application info, as a BCP47 tag (e.g. "en-US",
* "de"). If not specified the default language of the application will be used.
*/
public Get setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Devices collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.Devices.List request = androidmanagement.devices().list(parameters ...)}
*
*
* @return the resource collection
*/
public Devices devices() {
return new Devices();
}
/**
* The "devices" collection of methods.
*/
public class Devices {
/**
* Deletes a device. This operation wipes the device. Deleted devices do not show up in
* enterprises.devices.list calls and a 404 is returned from enterprises.devices.get.
*
* Create a request for the method "devices.delete".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/devices/[^/]+$");
/**
* Deletes a device. This operation wipes the device. Deleted devices do not show up in
* enterprises.devices.list calls and a 404 is returned from enterprises.devices.get.
*
* Create a request for the method "devices.delete".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AndroidManagement.this, "DELETE", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+$");
}
this.name = name;
return this;
}
/** Optional flags that control the device wiping behavior. */
@com.google.api.client.util.Key
private java.util.List wipeDataFlags;
/** Optional flags that control the device wiping behavior.
*/
public java.util.List getWipeDataFlags() {
return wipeDataFlags;
}
/** Optional flags that control the device wiping behavior. */
public Delete setWipeDataFlags(java.util.List wipeDataFlags) {
this.wipeDataFlags = wipeDataFlags;
return this;
}
/**
* Optional. A short message displayed to the user before wiping the work profile on
* personal devices. This has no effect on company owned devices. The maximum message length
* is 200 characters.
*/
@com.google.api.client.util.Key
private java.lang.String wipeReasonMessage;
/** Optional. A short message displayed to the user before wiping the work profile on personal devices.
This has no effect on company owned devices. The maximum message length is 200 characters.
*/
public java.lang.String getWipeReasonMessage() {
return wipeReasonMessage;
}
/**
* Optional. A short message displayed to the user before wiping the work profile on
* personal devices. This has no effect on company owned devices. The maximum message length
* is 200 characters.
*/
public Delete setWipeReasonMessage(java.lang.String wipeReasonMessage) {
this.wipeReasonMessage = wipeReasonMessage;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a device. Deleted devices will respond with a 404 error.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/devices/[^/]+$");
/**
* Gets a device. Deleted devices will respond with a 404 error.
*
* Create a request for the method "devices.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Device.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Issues a command to a device. The Operation resource returned contains a Command in its metadata
* field. Use the get operation method to get the status of the command.
*
* Create a request for the method "devices.issueCommand".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link IssueCommand#execute()} method to invoke the remote
* operation.
*
* @param name The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Command}
* @return the request
*/
public IssueCommand issueCommand(java.lang.String name, com.google.api.services.androidmanagement.v1.model.Command content) throws java.io.IOException {
IssueCommand result = new IssueCommand(name, content);
initialize(result);
return result;
}
public class IssueCommand extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}:issueCommand";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/devices/[^/]+$");
/**
* Issues a command to a device. The Operation resource returned contains a Command in its
* metadata field. Use the get operation method to get the status of the command.
*
* Create a request for the method "devices.issueCommand".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link IssueCommand#execute()} method to invoke the remote
* operation. {@link
* IssueCommand#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Command}
* @since 1.13
*/
protected IssueCommand(java.lang.String name, com.google.api.services.androidmanagement.v1.model.Command content) {
super(AndroidManagement.this, "POST", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+$");
}
}
@Override
public IssueCommand set$Xgafv(java.lang.String $Xgafv) {
return (IssueCommand) super.set$Xgafv($Xgafv);
}
@Override
public IssueCommand setAccessToken(java.lang.String accessToken) {
return (IssueCommand) super.setAccessToken(accessToken);
}
@Override
public IssueCommand setAlt(java.lang.String alt) {
return (IssueCommand) super.setAlt(alt);
}
@Override
public IssueCommand setCallback(java.lang.String callback) {
return (IssueCommand) super.setCallback(callback);
}
@Override
public IssueCommand setFields(java.lang.String fields) {
return (IssueCommand) super.setFields(fields);
}
@Override
public IssueCommand setKey(java.lang.String key) {
return (IssueCommand) super.setKey(key);
}
@Override
public IssueCommand setOauthToken(java.lang.String oauthToken) {
return (IssueCommand) super.setOauthToken(oauthToken);
}
@Override
public IssueCommand setPrettyPrint(java.lang.Boolean prettyPrint) {
return (IssueCommand) super.setPrettyPrint(prettyPrint);
}
@Override
public IssueCommand setQuotaUser(java.lang.String quotaUser) {
return (IssueCommand) super.setQuotaUser(quotaUser);
}
@Override
public IssueCommand setUploadType(java.lang.String uploadType) {
return (IssueCommand) super.setUploadType(uploadType);
}
@Override
public IssueCommand setUploadProtocol(java.lang.String uploadProtocol) {
return (IssueCommand) super.setUploadProtocol(uploadProtocol);
}
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}. */
public IssueCommand setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+$");
}
this.name = name;
return this;
}
@Override
public IssueCommand set(String parameterName, Object value) {
return (IssueCommand) super.set(parameterName, value);
}
}
/**
* Lists devices for a given enterprise. Deleted devices are not returned in the response.
*
* Create a request for the method "devices.list".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/devices";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Lists devices for a given enterprise. Deleted devices are not returned in the response.
*
* Create a request for the method "devices.list".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.ListDevicesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getParent() {
return parent;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
/** The requested page size. The actual page size may be fixed to a min or max value. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The requested page size. The actual page size may be fixed to a min or max value.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The requested page size. The actual page size may be fixed to a min or max value. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying a page of results returned by the server. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results returned by the server.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying a page of results returned by the server. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a device.
*
* Create a request for the method "devices.patch".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Device}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.androidmanagement.v1.model.Device content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/devices/[^/]+$");
/**
* Updates a device.
*
* Create a request for the method "devices.patch".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Device}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.androidmanagement.v1.model.Device content) {
super(AndroidManagement.this, "PATCH", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.Device.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the device in the form enterprises/{enterpriseId}/devices/{deviceId}. */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+$");
}
this.name = name;
return this;
}
/**
* The field mask indicating the fields to update. If not set, all modifiable fields will be
* modified.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The field mask indicating the fields to update. If not set, all modifiable fields will be modified.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The field mask indicating the fields to update. If not set, all modifiable fields will be
* modified.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.Operations.List request = androidmanagement.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/devices/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns google.rpc.Code.UNIMPLEMENTED. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* Code.CANCELLED.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Cancel#execute()} method to invoke the remote
* operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(AndroidManagement.this, "POST", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/devices/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/devices/[^/]+/operations$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+/operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/devices/[^/]+/operations$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the EnrollmentTokens collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.EnrollmentTokens.List request = androidmanagement.enrollmentTokens().list(parameters ...)}
*
*
* @return the resource collection
*/
public EnrollmentTokens enrollmentTokens() {
return new EnrollmentTokens();
}
/**
* The "enrollmentTokens" collection of methods.
*/
public class EnrollmentTokens {
/**
* Creates an enrollment token for a given enterprise. It's up to the caller's responsibility to
* manage the lifecycle of newly created tokens and deleting them when they're not intended to be
* used anymore.
*
* Create a request for the method "enrollmentTokens.create".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.EnrollmentToken}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.androidmanagement.v1.model.EnrollmentToken content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/enrollmentTokens";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Creates an enrollment token for a given enterprise. It's up to the caller's responsibility to
* manage the lifecycle of newly created tokens and deleting them when they're not intended to be
* used anymore.
*
* Create a request for the method "enrollmentTokens.create".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.EnrollmentToken}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.androidmanagement.v1.model.EnrollmentToken content) {
super(AndroidManagement.this, "POST", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.EnrollmentToken.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getParent() {
return parent;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an enrollment token. This operation invalidates the token, preventing its future use.
*
* Create a request for the method "enrollmentTokens.delete".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the enrollment token in the form
* enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/enrollmentTokens/[^/]+$");
/**
* Deletes an enrollment token. This operation invalidates the token, preventing its future use.
*
* Create a request for the method "enrollmentTokens.delete".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the enrollment token in the form
* enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AndroidManagement.this, "DELETE", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/enrollmentTokens/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the enrollment token in the form
* enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the enrollment token in the form
enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the enrollment token in the form
* enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/enrollmentTokens/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an active, unexpired enrollment token. A partial view of the enrollment token is returned.
* Only the following fields are populated: name, expirationTimestamp, allowPersonalUsage, value,
* qrCode. This method is meant to help manage active enrollment tokens lifecycle. For security
* reasons, it's recommended to delete active enrollment tokens as soon as they're not intended to
* be used anymore.
*
* Create a request for the method "enrollmentTokens.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the enrollment token in the form
* enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/enrollmentTokens/[^/]+$");
/**
* Gets an active, unexpired enrollment token. A partial view of the enrollment token is returned.
* Only the following fields are populated: name, expirationTimestamp, allowPersonalUsage, value,
* qrCode. This method is meant to help manage active enrollment tokens lifecycle. For security
* reasons, it's recommended to delete active enrollment tokens as soon as they're not intended to
* be used anymore.
*
* Create a request for the method "enrollmentTokens.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the enrollment token in the form
* enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.EnrollmentToken.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/enrollmentTokens/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the enrollment token in the form
* enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the enrollment token in the form
enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the enrollment token in the form
* enterprises/{enterpriseId}/enrollmentTokens/{enrollmentTokenId}.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/enrollmentTokens/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists active, unexpired enrollment tokens for a given enterprise. The list items contain only a
* partial view of EnrollmentToken object. Only the following fields are populated: name,
* expirationTimestamp, allowPersonalUsage, value, qrCode. This method is meant to help manage
* active enrollment tokens lifecycle. For security reasons, it's recommended to delete active
* enrollment tokens as soon as they're not intended to be used anymore.
*
* Create a request for the method "enrollmentTokens.list".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the enterprise in the form enterprises/{enterpriseId}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/enrollmentTokens";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Lists active, unexpired enrollment tokens for a given enterprise. The list items contain only a
* partial view of EnrollmentToken object. Only the following fields are populated: name,
* expirationTimestamp, allowPersonalUsage, value, qrCode. This method is meant to help manage
* active enrollment tokens lifecycle. For security reasons, it's recommended to delete active
* enrollment tokens as soon as they're not intended to be used anymore.
*
* Create a request for the method "enrollmentTokens.list".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the enterprise in the form enterprises/{enterpriseId}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.ListEnrollmentTokensResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The name of the enterprise in the form enterprises/{enterpriseId}. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The requested page size. The service may return fewer than this value. If unspecified, at
* most 10 items will be returned. The maximum value is 100; values above 100 will be
* coerced to 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The requested page size. The service may return fewer than this value. If unspecified, at most 10
items will be returned. The maximum value is 100; values above 100 will be coerced to 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The requested page size. The service may return fewer than this value. If unspecified, at
* most 10 items will be returned. The maximum value is 100; values above 100 will be
* coerced to 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying a page of results returned by the server. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results returned by the server.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying a page of results returned by the server. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the MigrationTokens collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.MigrationTokens.List request = androidmanagement.migrationTokens().list(parameters ...)}
*
*
* @return the resource collection
*/
public MigrationTokens migrationTokens() {
return new MigrationTokens();
}
/**
* The "migrationTokens" collection of methods.
*/
public class MigrationTokens {
/**
* Creates a migration token, to migrate an existing device from being managed by the EMM's Device
* Policy Controller (DPC) to being managed by the Android Management API. See the guide
* (https://developers.google.com/android/management/dpc-migration) for more details.
*
* Create a request for the method "migrationTokens.create".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The enterprise in which this migration token is created. This must be the same enterprise
* which already manages the device in the Play EMM API. Format: enterprises/{enterprise}
* @param content the {@link com.google.api.services.androidmanagement.v1.model.MigrationToken}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.androidmanagement.v1.model.MigrationToken content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/migrationTokens";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Creates a migration token, to migrate an existing device from being managed by the EMM's Device
* Policy Controller (DPC) to being managed by the Android Management API. See the guide
* (https://developers.google.com/android/management/dpc-migration) for more details.
*
* Create a request for the method "migrationTokens.create".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The enterprise in which this migration token is created. This must be the same enterprise
* which already manages the device in the Play EMM API. Format: enterprises/{enterprise}
* @param content the {@link com.google.api.services.androidmanagement.v1.model.MigrationToken}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.androidmanagement.v1.model.MigrationToken content) {
super(AndroidManagement.this, "POST", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.MigrationToken.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The enterprise in which this migration token is created. This must be the same
* enterprise which already manages the device in the Play EMM API. Format:
* enterprises/{enterprise}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The enterprise in which this migration token is created. This must be the same enterprise
which already manages the device in the Play EMM API. Format: enterprises/{enterprise}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The enterprise in which this migration token is created. This must be the same
* enterprise which already manages the device in the Play EMM API. Format:
* enterprises/{enterprise}
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Gets a migration token.
*
* Create a request for the method "migrationTokens.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the migration token to retrieve. Format:
* enterprises/{enterprise}/migrationTokens/{migration_token}
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/migrationTokens/[^/]+$");
/**
* Gets a migration token.
*
* Create a request for the method "migrationTokens.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the migration token to retrieve. Format:
* enterprises/{enterprise}/migrationTokens/{migration_token}
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.MigrationToken.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/migrationTokens/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the migration token to retrieve. Format:
* enterprises/{enterprise}/migrationTokens/{migration_token}
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the migration token to retrieve. Format:
enterprises/{enterprise}/migrationTokens/{migration_token}
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the migration token to retrieve. Format:
* enterprises/{enterprise}/migrationTokens/{migration_token}
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/migrationTokens/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists migration tokens.
*
* Create a request for the method "migrationTokens.list".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The enterprise which the migration tokens belong to. Format: enterprises/{enterprise}
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/migrationTokens";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Lists migration tokens.
*
* Create a request for the method "migrationTokens.list".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The enterprise which the migration tokens belong to. Format: enterprises/{enterprise}
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.ListMigrationTokensResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The enterprise which the migration tokens belong to. Format:
* enterprises/{enterprise}
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The enterprise which the migration tokens belong to. Format: enterprises/{enterprise}
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The enterprise which the migration tokens belong to. Format:
* enterprises/{enterprise}
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of migration tokens to return. Fewer migration tokens may be returned.
* If unspecified, at most 100 migration tokens will be returned. The maximum value is 100;
* values above 100 will be coerced to 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of migration tokens to return. Fewer migration tokens may be returned. If
unspecified, at most 100 migration tokens will be returned. The maximum value is 100; values above
100 will be coerced to 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of migration tokens to return. Fewer migration tokens may be returned.
* If unspecified, at most 100 migration tokens will be returned. The maximum value is 100;
* values above 100 will be coerced to 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous ListMigrationTokens call. Provide this to retrieve
* the subsequent page.When paginating, all other parameters provided to ListMigrationTokens
* must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous ListMigrationTokens call. Provide this to retrieve the
subsequent page.When paginating, all other parameters provided to ListMigrationTokens must match
the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous ListMigrationTokens call. Provide this to retrieve
* the subsequent page.When paginating, all other parameters provided to ListMigrationTokens
* must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Policies collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.Policies.List request = androidmanagement.policies().list(parameters ...)}
*
*
* @return the resource collection
*/
public Policies policies() {
return new Policies();
}
/**
* The "policies" collection of methods.
*/
public class Policies {
/**
* Deletes a policy. This operation is only permitted if no devices are currently referencing the
* policy.
*
* Create a request for the method "policies.delete".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/policies/[^/]+$");
/**
* Deletes a policy. This operation is only permitted if no devices are currently referencing the
* policy.
*
* Create a request for the method "policies.delete".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AndroidManagement.this, "DELETE", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/policies/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/policies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a policy.
*
* Create a request for the method "policies.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/policies/[^/]+$");
/**
* Gets a policy.
*
* Create a request for the method "policies.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Policy.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/policies/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/policies/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists policies for a given enterprise.
*
* Create a request for the method "policies.list".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/policies";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Lists policies for a given enterprise.
*
* Create a request for the method "policies.list".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.ListPoliciesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getParent() {
return parent;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
/** The requested page size. The actual page size may be fixed to a min or max value. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The requested page size. The actual page size may be fixed to a min or max value.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The requested page size. The actual page size may be fixed to a min or max value. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying a page of results returned by the server. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results returned by the server.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying a page of results returned by the server. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates or creates a policy.
*
* Create a request for the method "policies.patch".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Policy}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.androidmanagement.v1.model.Policy content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/policies/[^/]+$");
/**
* Updates or creates a policy.
*
* Create a request for the method "policies.patch".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.Policy}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.androidmanagement.v1.model.Policy content) {
super(AndroidManagement.this, "PATCH", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.Policy.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/policies/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the policy in the form enterprises/{enterpriseId}/policies/{policyId}. */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/policies/[^/]+$");
}
this.name = name;
return this;
}
/**
* The field mask indicating the fields to update. If not set, all modifiable fields will be
* modified.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The field mask indicating the fields to update. If not set, all modifiable fields will be modified.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The field mask indicating the fields to update. If not set, all modifiable fields will be
* modified.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the WebApps collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.WebApps.List request = androidmanagement.webApps().list(parameters ...)}
*
*
* @return the resource collection
*/
public WebApps webApps() {
return new WebApps();
}
/**
* The "webApps" collection of methods.
*/
public class WebApps {
/**
* Creates a web app.
*
* Create a request for the method "webApps.create".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.WebApp}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.androidmanagement.v1.model.WebApp content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/webApps";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Creates a web app.
*
* Create a request for the method "webApps.create".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.WebApp}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.androidmanagement.v1.model.WebApp content) {
super(AndroidManagement.this, "POST", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.WebApp.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getParent() {
return parent;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a web app.
*
* Create a request for the method "webApps.delete".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/webApps/[^/]+$");
/**
* Deletes a web app.
*
* Create a request for the method "webApps.delete".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Delete#execute()} method to invoke the remote
* operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(AndroidManagement.this, "DELETE", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/webApps/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/webApps/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a web app.
*
* Create a request for the method "webApps.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the web app in the form enterprises/{enterpriseId}/webApp/{packageName}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/webApps/[^/]+$");
/**
* Gets a web app.
*
* Create a request for the method "webApps.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the web app in the form enterprises/{enterpriseId}/webApp/{packageName}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.WebApp.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/webApps/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the web app in the form enterprises/{enterpriseId}/webApp/{packageName}. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the web app in the form enterprises/{enterpriseId}/webApp/{packageName}.
*/
public java.lang.String getName() {
return name;
}
/** The name of the web app in the form enterprises/{enterpriseId}/webApp/{packageName}. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/webApps/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists web apps for a given enterprise.
*
* Create a request for the method "webApps.list".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/webApps";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Lists web apps for a given enterprise.
*
* Create a request for the method "webApps.list".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.ListWebAppsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getParent() {
return parent;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The requested page size. This is a hint and the actual page size in the response may be
* different.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The requested page size. This is a hint and the actual page size in the response may be different.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The requested page size. This is a hint and the actual page size in the response may be
* different.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** A token identifying a page of results returned by the server. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A token identifying a page of results returned by the server.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** A token identifying a page of results returned by the server. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a web app.
*
* Create a request for the method "webApps.patch".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.WebApp}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.androidmanagement.v1.model.WebApp content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+/webApps/[^/]+$");
/**
* Updates a web app.
*
* Create a request for the method "webApps.patch".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Patch#execute()} method to invoke the remote
* operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.WebApp}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.androidmanagement.v1.model.WebApp content) {
super(AndroidManagement.this, "PATCH", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.WebApp.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/webApps/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the web app in the form enterprises/{enterpriseId}/webApps/{packageName}.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^enterprises/[^/]+/webApps/[^/]+$");
}
this.name = name;
return this;
}
/**
* The field mask indicating the fields to update. If not set, all modifiable fields will be
* modified.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The field mask indicating the fields to update. If not set, all modifiable fields will be modified.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The field mask indicating the fields to update. If not set, all modifiable fields will be
* modified.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the WebTokens collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.WebTokens.List request = androidmanagement.webTokens().list(parameters ...)}
*
*
* @return the resource collection
*/
public WebTokens webTokens() {
return new WebTokens();
}
/**
* The "webTokens" collection of methods.
*/
public class WebTokens {
/**
* Creates a web token to access an embeddable managed Google Play web UI for a given enterprise.
*
* Create a request for the method "webTokens.create".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.WebToken}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.androidmanagement.v1.model.WebToken content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+parent}/webTokens";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^enterprises/[^/]+$");
/**
* Creates a web token to access an embeddable managed Google Play web UI for a given enterprise.
*
* Create a request for the method "webTokens.create".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent The name of the enterprise in the form enterprises/{enterpriseId}.
* @param content the {@link com.google.api.services.androidmanagement.v1.model.WebToken}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.androidmanagement.v1.model.WebToken content) {
super(AndroidManagement.this, "POST", REST_PATH, content, com.google.api.services.androidmanagement.v1.model.WebToken.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** The name of the enterprise in the form enterprises/{enterpriseId}.
*/
public java.lang.String getParent() {
return parent;
}
/** The name of the enterprise in the form enterprises/{enterpriseId}. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^enterprises/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the ProvisioningInfo collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.ProvisioningInfo.List request = androidmanagement.provisioningInfo().list(parameters ...)}
*
*
* @return the resource collection
*/
public ProvisioningInfo provisioningInfo() {
return new ProvisioningInfo();
}
/**
* The "provisioningInfo" collection of methods.
*/
public class ProvisioningInfo {
/**
* Get the device provisioning information by the identifier provided in the sign-in url.
*
* Create a request for the method "provisioningInfo.get".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The identifier that Android Device Policy passes to the 3P sign-in page in the form of
* provisioningInfo/{provisioning_info}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends AndroidManagementRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^provisioningInfo/[^/]+$");
/**
* Get the device provisioning information by the identifier provided in the sign-in url.
*
* Create a request for the method "provisioningInfo.get".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The identifier that Android Device Policy passes to the 3P sign-in page in the form of
* provisioningInfo/{provisioning_info}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(AndroidManagement.this, "GET", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.ProvisioningInfo.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^provisioningInfo/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The identifier that Android Device Policy passes to the 3P sign-in page in the
* form of provisioningInfo/{provisioning_info}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The identifier that Android Device Policy passes to the 3P sign-in page in the form of
provisioningInfo/{provisioning_info}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The identifier that Android Device Policy passes to the 3P sign-in page in the
* form of provisioningInfo/{provisioning_info}.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^provisioningInfo/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SignupUrls collection.
*
* The typical use is:
*
* {@code AndroidManagement androidmanagement = new AndroidManagement(...);}
* {@code AndroidManagement.SignupUrls.List request = androidmanagement.signupUrls().list(parameters ...)}
*
*
* @return the resource collection
*/
public SignupUrls signupUrls() {
return new SignupUrls();
}
/**
* The "signupUrls" collection of methods.
*/
public class SignupUrls {
/**
* Creates an enterprise signup URL.
*
* Create a request for the method "signupUrls.create".
*
* This request holds the parameters needed by the androidmanagement server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Create create() throws java.io.IOException {
Create result = new Create();
initialize(result);
return result;
}
public class Create extends AndroidManagementRequest {
private static final String REST_PATH = "v1/signupUrls";
/**
* Creates an enterprise signup URL.
*
* Create a request for the method "signupUrls.create".
*
* This request holds the parameters needed by the the androidmanagement server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Create() {
super(AndroidManagement.this, "POST", REST_PATH, null, com.google.api.services.androidmanagement.v1.model.SignupUrl.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Optional. Email address used to prefill the admin field of the enterprise signup form. This
* value is a hint only and can be altered by the user.
*/
@com.google.api.client.util.Key
private java.lang.String adminEmail;
/** Optional. Email address used to prefill the admin field of the enterprise signup form. This value
is a hint only and can be altered by the user.
*/
public java.lang.String getAdminEmail() {
return adminEmail;
}
/**
* Optional. Email address used to prefill the admin field of the enterprise signup form. This
* value is a hint only and can be altered by the user.
*/
public Create setAdminEmail(java.lang.String adminEmail) {
this.adminEmail = adminEmail;
return this;
}
/**
* The callback URL that the admin will be redirected to after successfully creating an
* enterprise. Before redirecting there the system will add a query parameter to this URL
* named enterpriseToken which will contain an opaque token to be used for the create
* enterprise request. The URL will be parsed then reformatted in order to add the
* enterpriseToken parameter, so there may be some minor formatting changes.
*/
@com.google.api.client.util.Key
private java.lang.String callbackUrl;
/** The callback URL that the admin will be redirected to after successfully creating an enterprise.
Before redirecting there the system will add a query parameter to this URL named enterpriseToken
which will contain an opaque token to be used for the create enterprise request. The URL will be
parsed then reformatted in order to add the enterpriseToken parameter, so there may be some minor
formatting changes.
*/
public java.lang.String getCallbackUrl() {
return callbackUrl;
}
/**
* The callback URL that the admin will be redirected to after successfully creating an
* enterprise. Before redirecting there the system will add a query parameter to this URL
* named enterpriseToken which will contain an opaque token to be used for the create
* enterprise request. The URL will be parsed then reformatted in order to add the
* enterpriseToken parameter, so there may be some minor formatting changes.
*/
public Create setCallbackUrl(java.lang.String callbackUrl) {
this.callbackUrl = callbackUrl;
return this;
}
/** The ID of the Google Cloud Platform project which will own the enterprise. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** The ID of the Google Cloud Platform project which will own the enterprise.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** The ID of the Google Cloud Platform project which will own the enterprise. */
public Create setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link AndroidManagement}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link AndroidManagement}. */
@Override
public AndroidManagement build() {
return new AndroidManagement(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AndroidManagementRequestInitializer}.
*
* @since 1.12
*/
public Builder setAndroidManagementRequestInitializer(
AndroidManagementRequestInitializer androidmanagementRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(androidmanagementRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}