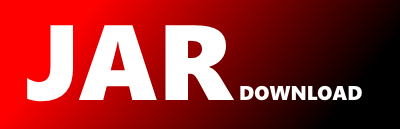
com.google.api.services.androidmanagement.v1.model.AdvancedSecurityOverrides Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Advanced security settings. In most cases, setting these is not needed.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AdvancedSecurityOverrides extends com.google.api.client.json.GenericJson {
/**
* Controls Common Criteria Mode—security standards defined in the Common Criteria for Information
* Technology Security Evaluation (https://www.commoncriteriaportal.org/) (CC). Enabling Common
* Criteria Mode increases certain security components on a device, see CommonCriteriaMode for
* details.Warning: Common Criteria Mode enforces a strict security model typically only required
* for IT products used in national security systems and other highly sensitive organizations.
* Standard device use may be affected. Only enabled if required. If Common Criteria Mode is
* turned off after being enabled previously, all user-configured Wi-Fi networks may be lost and
* any enterprise-configured Wi-Fi networks that require user input may need to be reconfigured.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String commonCriteriaMode;
/**
* Optional. Controls whether content protection, which scans for deceptive apps, is enabled. This
* is supported on Android 15 and above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contentProtectionPolicy;
/**
* Controls access to developer settings: developer options and safe boot. Replaces
* safeBootDisabled (deprecated) and debuggingFeaturesAllowed (deprecated).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String developerSettings;
/**
* Whether Google Play Protect verification (https://support.google.com/accounts/answer/2812853)
* is enforced. Replaces ensureVerifyAppsEnabled (deprecated).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String googlePlayProtectVerifyApps;
/**
* Optional. Controls Memory Tagging Extension (MTE)
* (https://source.android.com/docs/security/test/memory-safety/arm-mte) on the device. The device
* needs to be rebooted to apply changes to the MTE policy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mtePolicy;
/**
* Personal apps that can read work profile notifications using a NotificationListenerService (htt
* ps://developer.android.com/reference/android/service/notification/NotificationListenerService).
* By default, no personal apps (aside from system apps) can read work notifications. Each value
* in the list must be a package name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List personalAppsThatCanReadWorkNotifications;
/**
* The policy for untrusted apps (apps from unknown sources) enforced on the device. Replaces
* install_unknown_sources_allowed (deprecated).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String untrustedAppsPolicy;
/**
* Controls Common Criteria Mode—security standards defined in the Common Criteria for Information
* Technology Security Evaluation (https://www.commoncriteriaportal.org/) (CC). Enabling Common
* Criteria Mode increases certain security components on a device, see CommonCriteriaMode for
* details.Warning: Common Criteria Mode enforces a strict security model typically only required
* for IT products used in national security systems and other highly sensitive organizations.
* Standard device use may be affected. Only enabled if required. If Common Criteria Mode is
* turned off after being enabled previously, all user-configured Wi-Fi networks may be lost and
* any enterprise-configured Wi-Fi networks that require user input may need to be reconfigured.
* @return value or {@code null} for none
*/
public java.lang.String getCommonCriteriaMode() {
return commonCriteriaMode;
}
/**
* Controls Common Criteria Mode—security standards defined in the Common Criteria for Information
* Technology Security Evaluation (https://www.commoncriteriaportal.org/) (CC). Enabling Common
* Criteria Mode increases certain security components on a device, see CommonCriteriaMode for
* details.Warning: Common Criteria Mode enforces a strict security model typically only required
* for IT products used in national security systems and other highly sensitive organizations.
* Standard device use may be affected. Only enabled if required. If Common Criteria Mode is
* turned off after being enabled previously, all user-configured Wi-Fi networks may be lost and
* any enterprise-configured Wi-Fi networks that require user input may need to be reconfigured.
* @param commonCriteriaMode commonCriteriaMode or {@code null} for none
*/
public AdvancedSecurityOverrides setCommonCriteriaMode(java.lang.String commonCriteriaMode) {
this.commonCriteriaMode = commonCriteriaMode;
return this;
}
/**
* Optional. Controls whether content protection, which scans for deceptive apps, is enabled. This
* is supported on Android 15 and above.
* @return value or {@code null} for none
*/
public java.lang.String getContentProtectionPolicy() {
return contentProtectionPolicy;
}
/**
* Optional. Controls whether content protection, which scans for deceptive apps, is enabled. This
* is supported on Android 15 and above.
* @param contentProtectionPolicy contentProtectionPolicy or {@code null} for none
*/
public AdvancedSecurityOverrides setContentProtectionPolicy(java.lang.String contentProtectionPolicy) {
this.contentProtectionPolicy = contentProtectionPolicy;
return this;
}
/**
* Controls access to developer settings: developer options and safe boot. Replaces
* safeBootDisabled (deprecated) and debuggingFeaturesAllowed (deprecated).
* @return value or {@code null} for none
*/
public java.lang.String getDeveloperSettings() {
return developerSettings;
}
/**
* Controls access to developer settings: developer options and safe boot. Replaces
* safeBootDisabled (deprecated) and debuggingFeaturesAllowed (deprecated).
* @param developerSettings developerSettings or {@code null} for none
*/
public AdvancedSecurityOverrides setDeveloperSettings(java.lang.String developerSettings) {
this.developerSettings = developerSettings;
return this;
}
/**
* Whether Google Play Protect verification (https://support.google.com/accounts/answer/2812853)
* is enforced. Replaces ensureVerifyAppsEnabled (deprecated).
* @return value or {@code null} for none
*/
public java.lang.String getGooglePlayProtectVerifyApps() {
return googlePlayProtectVerifyApps;
}
/**
* Whether Google Play Protect verification (https://support.google.com/accounts/answer/2812853)
* is enforced. Replaces ensureVerifyAppsEnabled (deprecated).
* @param googlePlayProtectVerifyApps googlePlayProtectVerifyApps or {@code null} for none
*/
public AdvancedSecurityOverrides setGooglePlayProtectVerifyApps(java.lang.String googlePlayProtectVerifyApps) {
this.googlePlayProtectVerifyApps = googlePlayProtectVerifyApps;
return this;
}
/**
* Optional. Controls Memory Tagging Extension (MTE)
* (https://source.android.com/docs/security/test/memory-safety/arm-mte) on the device. The device
* needs to be rebooted to apply changes to the MTE policy.
* @return value or {@code null} for none
*/
public java.lang.String getMtePolicy() {
return mtePolicy;
}
/**
* Optional. Controls Memory Tagging Extension (MTE)
* (https://source.android.com/docs/security/test/memory-safety/arm-mte) on the device. The device
* needs to be rebooted to apply changes to the MTE policy.
* @param mtePolicy mtePolicy or {@code null} for none
*/
public AdvancedSecurityOverrides setMtePolicy(java.lang.String mtePolicy) {
this.mtePolicy = mtePolicy;
return this;
}
/**
* Personal apps that can read work profile notifications using a NotificationListenerService (htt
* ps://developer.android.com/reference/android/service/notification/NotificationListenerService).
* By default, no personal apps (aside from system apps) can read work notifications. Each value
* in the list must be a package name.
* @return value or {@code null} for none
*/
public java.util.List getPersonalAppsThatCanReadWorkNotifications() {
return personalAppsThatCanReadWorkNotifications;
}
/**
* Personal apps that can read work profile notifications using a NotificationListenerService (htt
* ps://developer.android.com/reference/android/service/notification/NotificationListenerService).
* By default, no personal apps (aside from system apps) can read work notifications. Each value
* in the list must be a package name.
* @param personalAppsThatCanReadWorkNotifications personalAppsThatCanReadWorkNotifications or {@code null} for none
*/
public AdvancedSecurityOverrides setPersonalAppsThatCanReadWorkNotifications(java.util.List personalAppsThatCanReadWorkNotifications) {
this.personalAppsThatCanReadWorkNotifications = personalAppsThatCanReadWorkNotifications;
return this;
}
/**
* The policy for untrusted apps (apps from unknown sources) enforced on the device. Replaces
* install_unknown_sources_allowed (deprecated).
* @return value or {@code null} for none
*/
public java.lang.String getUntrustedAppsPolicy() {
return untrustedAppsPolicy;
}
/**
* The policy for untrusted apps (apps from unknown sources) enforced on the device. Replaces
* install_unknown_sources_allowed (deprecated).
* @param untrustedAppsPolicy untrustedAppsPolicy or {@code null} for none
*/
public AdvancedSecurityOverrides setUntrustedAppsPolicy(java.lang.String untrustedAppsPolicy) {
this.untrustedAppsPolicy = untrustedAppsPolicy;
return this;
}
@Override
public AdvancedSecurityOverrides set(String fieldName, Object value) {
return (AdvancedSecurityOverrides) super.set(fieldName, value);
}
@Override
public AdvancedSecurityOverrides clone() {
return (AdvancedSecurityOverrides) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy