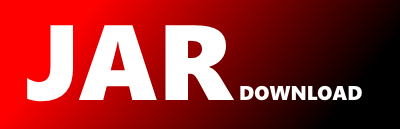
com.google.api.services.androidmanagement.v1.model.Application Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Information about an app.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Application extends com.google.api.client.json.GenericJson {
/**
* Whether this app is free, free with in-app purchases, or paid. If the pricing is unspecified,
* this means the app is not generally available anymore (even though it might still be available
* to people who own it).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String appPricing;
/**
* Application tracks visible to the enterprise.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List appTracks;
static {
// hack to force ProGuard to consider AppTrackInfo used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AppTrackInfo.class);
}
/**
* Versions currently available for this app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List appVersions;
static {
// hack to force ProGuard to consider AppVersion used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AppVersion.class);
}
/**
* The name of the author of the apps (for example, the app developer).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String author;
/**
* The countries which this app is available in as per ISO 3166-1 alpha-2.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List availableCountries;
/**
* The app category (e.g. RACING, SOCIAL, etc.)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String category;
/**
* The content rating for this app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String contentRating;
/**
* The localized promotional description, if available.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* How and to whom the package is made available.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String distributionChannel;
/**
* Noteworthy features (if any) of this app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List features;
/**
* Full app description, if available.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fullDescription;
/**
* A link to an image that can be used as an icon for the app. This image is suitable for use up
* to a pixel size of 512 x 512.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String iconUrl;
/**
* The set of managed properties available to be pre-configured for the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List managedProperties;
/**
* The minimum Android SDK necessary to run the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer minAndroidSdkVersion;
/**
* The name of the app in the form enterprises/{enterprise}/applications/{package_name}.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* The permissions required by the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List permissions;
/**
* A link to the (consumer) Google Play details page for the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String playStoreUrl;
/**
* A localised description of the recent changes made to the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String recentChanges;
/**
* A list of screenshot links representing the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List screenshotUrls;
/**
* A link to a smaller image that can be used as an icon for the app. This image is suitable for
* use up to a pixel size of 128 x 128.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String smallIconUrl;
/**
* The title of the app. Localized.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* Output only. The approximate time (within 7 days) the app was last published.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Whether this app is free, free with in-app purchases, or paid. If the pricing is unspecified,
* this means the app is not generally available anymore (even though it might still be available
* to people who own it).
* @return value or {@code null} for none
*/
public java.lang.String getAppPricing() {
return appPricing;
}
/**
* Whether this app is free, free with in-app purchases, or paid. If the pricing is unspecified,
* this means the app is not generally available anymore (even though it might still be available
* to people who own it).
* @param appPricing appPricing or {@code null} for none
*/
public Application setAppPricing(java.lang.String appPricing) {
this.appPricing = appPricing;
return this;
}
/**
* Application tracks visible to the enterprise.
* @return value or {@code null} for none
*/
public java.util.List getAppTracks() {
return appTracks;
}
/**
* Application tracks visible to the enterprise.
* @param appTracks appTracks or {@code null} for none
*/
public Application setAppTracks(java.util.List appTracks) {
this.appTracks = appTracks;
return this;
}
/**
* Versions currently available for this app.
* @return value or {@code null} for none
*/
public java.util.List getAppVersions() {
return appVersions;
}
/**
* Versions currently available for this app.
* @param appVersions appVersions or {@code null} for none
*/
public Application setAppVersions(java.util.List appVersions) {
this.appVersions = appVersions;
return this;
}
/**
* The name of the author of the apps (for example, the app developer).
* @return value or {@code null} for none
*/
public java.lang.String getAuthor() {
return author;
}
/**
* The name of the author of the apps (for example, the app developer).
* @param author author or {@code null} for none
*/
public Application setAuthor(java.lang.String author) {
this.author = author;
return this;
}
/**
* The countries which this app is available in as per ISO 3166-1 alpha-2.
* @return value or {@code null} for none
*/
public java.util.List getAvailableCountries() {
return availableCountries;
}
/**
* The countries which this app is available in as per ISO 3166-1 alpha-2.
* @param availableCountries availableCountries or {@code null} for none
*/
public Application setAvailableCountries(java.util.List availableCountries) {
this.availableCountries = availableCountries;
return this;
}
/**
* The app category (e.g. RACING, SOCIAL, etc.)
* @return value or {@code null} for none
*/
public java.lang.String getCategory() {
return category;
}
/**
* The app category (e.g. RACING, SOCIAL, etc.)
* @param category category or {@code null} for none
*/
public Application setCategory(java.lang.String category) {
this.category = category;
return this;
}
/**
* The content rating for this app.
* @return value or {@code null} for none
*/
public java.lang.String getContentRating() {
return contentRating;
}
/**
* The content rating for this app.
* @param contentRating contentRating or {@code null} for none
*/
public Application setContentRating(java.lang.String contentRating) {
this.contentRating = contentRating;
return this;
}
/**
* The localized promotional description, if available.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* The localized promotional description, if available.
* @param description description or {@code null} for none
*/
public Application setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* How and to whom the package is made available.
* @return value or {@code null} for none
*/
public java.lang.String getDistributionChannel() {
return distributionChannel;
}
/**
* How and to whom the package is made available.
* @param distributionChannel distributionChannel or {@code null} for none
*/
public Application setDistributionChannel(java.lang.String distributionChannel) {
this.distributionChannel = distributionChannel;
return this;
}
/**
* Noteworthy features (if any) of this app.
* @return value or {@code null} for none
*/
public java.util.List getFeatures() {
return features;
}
/**
* Noteworthy features (if any) of this app.
* @param features features or {@code null} for none
*/
public Application setFeatures(java.util.List features) {
this.features = features;
return this;
}
/**
* Full app description, if available.
* @return value or {@code null} for none
*/
public java.lang.String getFullDescription() {
return fullDescription;
}
/**
* Full app description, if available.
* @param fullDescription fullDescription or {@code null} for none
*/
public Application setFullDescription(java.lang.String fullDescription) {
this.fullDescription = fullDescription;
return this;
}
/**
* A link to an image that can be used as an icon for the app. This image is suitable for use up
* to a pixel size of 512 x 512.
* @return value or {@code null} for none
*/
public java.lang.String getIconUrl() {
return iconUrl;
}
/**
* A link to an image that can be used as an icon for the app. This image is suitable for use up
* to a pixel size of 512 x 512.
* @param iconUrl iconUrl or {@code null} for none
*/
public Application setIconUrl(java.lang.String iconUrl) {
this.iconUrl = iconUrl;
return this;
}
/**
* The set of managed properties available to be pre-configured for the app.
* @return value or {@code null} for none
*/
public java.util.List getManagedProperties() {
return managedProperties;
}
/**
* The set of managed properties available to be pre-configured for the app.
* @param managedProperties managedProperties or {@code null} for none
*/
public Application setManagedProperties(java.util.List managedProperties) {
this.managedProperties = managedProperties;
return this;
}
/**
* The minimum Android SDK necessary to run the app.
* @return value or {@code null} for none
*/
public java.lang.Integer getMinAndroidSdkVersion() {
return minAndroidSdkVersion;
}
/**
* The minimum Android SDK necessary to run the app.
* @param minAndroidSdkVersion minAndroidSdkVersion or {@code null} for none
*/
public Application setMinAndroidSdkVersion(java.lang.Integer minAndroidSdkVersion) {
this.minAndroidSdkVersion = minAndroidSdkVersion;
return this;
}
/**
* The name of the app in the form enterprises/{enterprise}/applications/{package_name}.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the app in the form enterprises/{enterprise}/applications/{package_name}.
* @param name name or {@code null} for none
*/
public Application setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* The permissions required by the app.
* @return value or {@code null} for none
*/
public java.util.List getPermissions() {
return permissions;
}
/**
* The permissions required by the app.
* @param permissions permissions or {@code null} for none
*/
public Application setPermissions(java.util.List permissions) {
this.permissions = permissions;
return this;
}
/**
* A link to the (consumer) Google Play details page for the app.
* @return value or {@code null} for none
*/
public java.lang.String getPlayStoreUrl() {
return playStoreUrl;
}
/**
* A link to the (consumer) Google Play details page for the app.
* @param playStoreUrl playStoreUrl or {@code null} for none
*/
public Application setPlayStoreUrl(java.lang.String playStoreUrl) {
this.playStoreUrl = playStoreUrl;
return this;
}
/**
* A localised description of the recent changes made to the app.
* @return value or {@code null} for none
*/
public java.lang.String getRecentChanges() {
return recentChanges;
}
/**
* A localised description of the recent changes made to the app.
* @param recentChanges recentChanges or {@code null} for none
*/
public Application setRecentChanges(java.lang.String recentChanges) {
this.recentChanges = recentChanges;
return this;
}
/**
* A list of screenshot links representing the app.
* @return value or {@code null} for none
*/
public java.util.List getScreenshotUrls() {
return screenshotUrls;
}
/**
* A list of screenshot links representing the app.
* @param screenshotUrls screenshotUrls or {@code null} for none
*/
public Application setScreenshotUrls(java.util.List screenshotUrls) {
this.screenshotUrls = screenshotUrls;
return this;
}
/**
* A link to a smaller image that can be used as an icon for the app. This image is suitable for
* use up to a pixel size of 128 x 128.
* @return value or {@code null} for none
*/
public java.lang.String getSmallIconUrl() {
return smallIconUrl;
}
/**
* A link to a smaller image that can be used as an icon for the app. This image is suitable for
* use up to a pixel size of 128 x 128.
* @param smallIconUrl smallIconUrl or {@code null} for none
*/
public Application setSmallIconUrl(java.lang.String smallIconUrl) {
this.smallIconUrl = smallIconUrl;
return this;
}
/**
* The title of the app. Localized.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* The title of the app. Localized.
* @param title title or {@code null} for none
*/
public Application setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* Output only. The approximate time (within 7 days) the app was last published.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The approximate time (within 7 days) the app was last published.
* @param updateTime updateTime or {@code null} for none
*/
public Application setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public Application set(String fieldName, Object value) {
return (Application) super.set(fieldName, value);
}
@Override
public Application clone() {
return (Application) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy