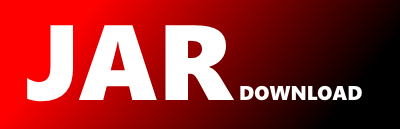
com.google.api.services.androidmanagement.v1.model.ApplicationPolicy Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Policy for an individual app. Note: Application availability on a given device cannot be changed
* using this policy if installAppsDisabled is enabled. The maximum number of applications that you
* can specify per policy is 3,000.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ApplicationPolicy extends com.google.api.client.json.GenericJson {
/**
* List of the app’s track IDs that a device belonging to the enterprise can access. If the list
* contains multiple track IDs, devices receive the latest version among all accessible tracks. If
* the list contains no track IDs, devices only have access to the app’s production track. More
* details about each track are available in AppTrackInfo.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List accessibleTrackIds;
/**
* Specifies whether the app is allowed networking when the VPN is not connected and
* alwaysOnVpnPackage.lockdownEnabled is enabled. If set to VPN_LOCKDOWN_ENFORCED, the app is not
* allowed networking, and if set to VPN_LOCKDOWN_EXEMPTION, the app is allowed networking. Only
* supported on devices running Android 10 and above. If this is not supported by the device, the
* device will contain a NonComplianceDetail with non_compliance_reason set to API_LEVEL and a
* fieldPath. If this is not applicable to the app, the device will contain a NonComplianceDetail
* with non_compliance_reason set to UNSUPPORTED and a fieldPath. The fieldPath is set to
* applications[i].alwaysOnVpnLockdownExemption, where i is the index of the package in the
* applications policy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String alwaysOnVpnLockdownExemption;
/**
* Controls the auto-update mode for the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String autoUpdateMode;
/**
* Controls whether the app can communicate with itself across a device’s work and personal
* profiles, subject to user consent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String connectedWorkAndPersonalApp;
/**
* Optional. Whether the app is allowed to act as a credential provider on Android 14 and above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String credentialProviderPolicy;
/**
* The default policy for all permissions requested by the app. If specified, this overrides the
* policy-level default_permission_policy which applies to all apps. It does not override the
* permission_grants which applies to all apps.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultPermissionPolicy;
/**
* The scopes delegated to the app from Android Device Policy. These provide additional privileges
* for the applications they are applied to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List delegatedScopes;
/**
* Whether the app is disabled. When disabled, the app data is still preserved.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean disabled;
/**
* Configuration to enable this app as an extension app, with the capability of interacting with
* Android Device Policy offline.This field can be set for at most one app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ExtensionConfig extensionConfig;
/**
* Optional. The constraints for installing the app. You can specify a maximum of one
* InstallConstraint. Multiple constraints are rejected.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List installConstraint;
/**
* Optional. Amongst apps with installType set to: FORCE_INSTALLED PREINSTALLEDthis controls the
* relative priority of installation. A value of 0 (default) means this app has no priority over
* other apps. For values between 1 and 10,000, a lower value means a higher priority. Values
* outside of the range 0 to 10,000 inclusive are rejected.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer installPriority;
/**
* The type of installation to perform.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String installType;
/**
* Whether the app is allowed to lock itself in full-screen mode. DEPRECATED. Use InstallType
* KIOSK or kioskCustomLauncherEnabled to configure a dedicated device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean lockTaskAllowed;
/**
* Managed configuration applied to the app. The format for the configuration is dictated by the
* ManagedProperty values supported by the app. Each field name in the managed configuration must
* match the key field of the ManagedProperty. The field value must be compatible with the type of
* the ManagedProperty: *type* *JSON value* BOOL true or false STRING string INTEGER number CHOICE
* string MULTISELECT array of strings HIDDEN string BUNDLE_ARRAY array of objects
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map managedConfiguration;
/**
* The managed configurations template for the app, saved from the managed configurations iframe.
* This field is ignored if managed_configuration is set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ManagedConfigurationTemplate managedConfigurationTemplate;
/**
* The minimum version of the app that runs on the device. If set, the device attempts to update
* the app to at least this version code. If the app is not up-to-date, the device will contain a
* NonComplianceDetail with non_compliance_reason set to APP_NOT_UPDATED. The app must already be
* published to Google Play with a version code greater than or equal to this value. At most 20
* apps may specify a minimum version code per policy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer minimumVersionCode;
/**
* The package name of the app. For example, com.google.android.youtube for the YouTube app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/**
* Explicit permission grants or denials for the app. These values override the
* default_permission_policy and permission_grants which apply to all apps.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List permissionGrants;
/**
* Optional. Specifies whether user control is permitted for the app. User control includes user
* actions like force-stopping and clearing app data. Supported on Android 11 and above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String userControlSettings;
/**
* Specifies whether the app installed in the work profile is allowed to add widgets to the home
* screen.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String workProfileWidgets;
/**
* List of the app’s track IDs that a device belonging to the enterprise can access. If the list
* contains multiple track IDs, devices receive the latest version among all accessible tracks. If
* the list contains no track IDs, devices only have access to the app’s production track. More
* details about each track are available in AppTrackInfo.
* @return value or {@code null} for none
*/
public java.util.List getAccessibleTrackIds() {
return accessibleTrackIds;
}
/**
* List of the app’s track IDs that a device belonging to the enterprise can access. If the list
* contains multiple track IDs, devices receive the latest version among all accessible tracks. If
* the list contains no track IDs, devices only have access to the app’s production track. More
* details about each track are available in AppTrackInfo.
* @param accessibleTrackIds accessibleTrackIds or {@code null} for none
*/
public ApplicationPolicy setAccessibleTrackIds(java.util.List accessibleTrackIds) {
this.accessibleTrackIds = accessibleTrackIds;
return this;
}
/**
* Specifies whether the app is allowed networking when the VPN is not connected and
* alwaysOnVpnPackage.lockdownEnabled is enabled. If set to VPN_LOCKDOWN_ENFORCED, the app is not
* allowed networking, and if set to VPN_LOCKDOWN_EXEMPTION, the app is allowed networking. Only
* supported on devices running Android 10 and above. If this is not supported by the device, the
* device will contain a NonComplianceDetail with non_compliance_reason set to API_LEVEL and a
* fieldPath. If this is not applicable to the app, the device will contain a NonComplianceDetail
* with non_compliance_reason set to UNSUPPORTED and a fieldPath. The fieldPath is set to
* applications[i].alwaysOnVpnLockdownExemption, where i is the index of the package in the
* applications policy.
* @return value or {@code null} for none
*/
public java.lang.String getAlwaysOnVpnLockdownExemption() {
return alwaysOnVpnLockdownExemption;
}
/**
* Specifies whether the app is allowed networking when the VPN is not connected and
* alwaysOnVpnPackage.lockdownEnabled is enabled. If set to VPN_LOCKDOWN_ENFORCED, the app is not
* allowed networking, and if set to VPN_LOCKDOWN_EXEMPTION, the app is allowed networking. Only
* supported on devices running Android 10 and above. If this is not supported by the device, the
* device will contain a NonComplianceDetail with non_compliance_reason set to API_LEVEL and a
* fieldPath. If this is not applicable to the app, the device will contain a NonComplianceDetail
* with non_compliance_reason set to UNSUPPORTED and a fieldPath. The fieldPath is set to
* applications[i].alwaysOnVpnLockdownExemption, where i is the index of the package in the
* applications policy.
* @param alwaysOnVpnLockdownExemption alwaysOnVpnLockdownExemption or {@code null} for none
*/
public ApplicationPolicy setAlwaysOnVpnLockdownExemption(java.lang.String alwaysOnVpnLockdownExemption) {
this.alwaysOnVpnLockdownExemption = alwaysOnVpnLockdownExemption;
return this;
}
/**
* Controls the auto-update mode for the app.
* @return value or {@code null} for none
*/
public java.lang.String getAutoUpdateMode() {
return autoUpdateMode;
}
/**
* Controls the auto-update mode for the app.
* @param autoUpdateMode autoUpdateMode or {@code null} for none
*/
public ApplicationPolicy setAutoUpdateMode(java.lang.String autoUpdateMode) {
this.autoUpdateMode = autoUpdateMode;
return this;
}
/**
* Controls whether the app can communicate with itself across a device’s work and personal
* profiles, subject to user consent.
* @return value or {@code null} for none
*/
public java.lang.String getConnectedWorkAndPersonalApp() {
return connectedWorkAndPersonalApp;
}
/**
* Controls whether the app can communicate with itself across a device’s work and personal
* profiles, subject to user consent.
* @param connectedWorkAndPersonalApp connectedWorkAndPersonalApp or {@code null} for none
*/
public ApplicationPolicy setConnectedWorkAndPersonalApp(java.lang.String connectedWorkAndPersonalApp) {
this.connectedWorkAndPersonalApp = connectedWorkAndPersonalApp;
return this;
}
/**
* Optional. Whether the app is allowed to act as a credential provider on Android 14 and above.
* @return value or {@code null} for none
*/
public java.lang.String getCredentialProviderPolicy() {
return credentialProviderPolicy;
}
/**
* Optional. Whether the app is allowed to act as a credential provider on Android 14 and above.
* @param credentialProviderPolicy credentialProviderPolicy or {@code null} for none
*/
public ApplicationPolicy setCredentialProviderPolicy(java.lang.String credentialProviderPolicy) {
this.credentialProviderPolicy = credentialProviderPolicy;
return this;
}
/**
* The default policy for all permissions requested by the app. If specified, this overrides the
* policy-level default_permission_policy which applies to all apps. It does not override the
* permission_grants which applies to all apps.
* @return value or {@code null} for none
*/
public java.lang.String getDefaultPermissionPolicy() {
return defaultPermissionPolicy;
}
/**
* The default policy for all permissions requested by the app. If specified, this overrides the
* policy-level default_permission_policy which applies to all apps. It does not override the
* permission_grants which applies to all apps.
* @param defaultPermissionPolicy defaultPermissionPolicy or {@code null} for none
*/
public ApplicationPolicy setDefaultPermissionPolicy(java.lang.String defaultPermissionPolicy) {
this.defaultPermissionPolicy = defaultPermissionPolicy;
return this;
}
/**
* The scopes delegated to the app from Android Device Policy. These provide additional privileges
* for the applications they are applied to.
* @return value or {@code null} for none
*/
public java.util.List getDelegatedScopes() {
return delegatedScopes;
}
/**
* The scopes delegated to the app from Android Device Policy. These provide additional privileges
* for the applications they are applied to.
* @param delegatedScopes delegatedScopes or {@code null} for none
*/
public ApplicationPolicy setDelegatedScopes(java.util.List delegatedScopes) {
this.delegatedScopes = delegatedScopes;
return this;
}
/**
* Whether the app is disabled. When disabled, the app data is still preserved.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDisabled() {
return disabled;
}
/**
* Whether the app is disabled. When disabled, the app data is still preserved.
* @param disabled disabled or {@code null} for none
*/
public ApplicationPolicy setDisabled(java.lang.Boolean disabled) {
this.disabled = disabled;
return this;
}
/**
* Configuration to enable this app as an extension app, with the capability of interacting with
* Android Device Policy offline.This field can be set for at most one app.
* @return value or {@code null} for none
*/
public ExtensionConfig getExtensionConfig() {
return extensionConfig;
}
/**
* Configuration to enable this app as an extension app, with the capability of interacting with
* Android Device Policy offline.This field can be set for at most one app.
* @param extensionConfig extensionConfig or {@code null} for none
*/
public ApplicationPolicy setExtensionConfig(ExtensionConfig extensionConfig) {
this.extensionConfig = extensionConfig;
return this;
}
/**
* Optional. The constraints for installing the app. You can specify a maximum of one
* InstallConstraint. Multiple constraints are rejected.
* @return value or {@code null} for none
*/
public java.util.List getInstallConstraint() {
return installConstraint;
}
/**
* Optional. The constraints for installing the app. You can specify a maximum of one
* InstallConstraint. Multiple constraints are rejected.
* @param installConstraint installConstraint or {@code null} for none
*/
public ApplicationPolicy setInstallConstraint(java.util.List installConstraint) {
this.installConstraint = installConstraint;
return this;
}
/**
* Optional. Amongst apps with installType set to: FORCE_INSTALLED PREINSTALLEDthis controls the
* relative priority of installation. A value of 0 (default) means this app has no priority over
* other apps. For values between 1 and 10,000, a lower value means a higher priority. Values
* outside of the range 0 to 10,000 inclusive are rejected.
* @return value or {@code null} for none
*/
public java.lang.Integer getInstallPriority() {
return installPriority;
}
/**
* Optional. Amongst apps with installType set to: FORCE_INSTALLED PREINSTALLEDthis controls the
* relative priority of installation. A value of 0 (default) means this app has no priority over
* other apps. For values between 1 and 10,000, a lower value means a higher priority. Values
* outside of the range 0 to 10,000 inclusive are rejected.
* @param installPriority installPriority or {@code null} for none
*/
public ApplicationPolicy setInstallPriority(java.lang.Integer installPriority) {
this.installPriority = installPriority;
return this;
}
/**
* The type of installation to perform.
* @return value or {@code null} for none
*/
public java.lang.String getInstallType() {
return installType;
}
/**
* The type of installation to perform.
* @param installType installType or {@code null} for none
*/
public ApplicationPolicy setInstallType(java.lang.String installType) {
this.installType = installType;
return this;
}
/**
* Whether the app is allowed to lock itself in full-screen mode. DEPRECATED. Use InstallType
* KIOSK or kioskCustomLauncherEnabled to configure a dedicated device.
* @return value or {@code null} for none
*/
public java.lang.Boolean getLockTaskAllowed() {
return lockTaskAllowed;
}
/**
* Whether the app is allowed to lock itself in full-screen mode. DEPRECATED. Use InstallType
* KIOSK or kioskCustomLauncherEnabled to configure a dedicated device.
* @param lockTaskAllowed lockTaskAllowed or {@code null} for none
*/
public ApplicationPolicy setLockTaskAllowed(java.lang.Boolean lockTaskAllowed) {
this.lockTaskAllowed = lockTaskAllowed;
return this;
}
/**
* Managed configuration applied to the app. The format for the configuration is dictated by the
* ManagedProperty values supported by the app. Each field name in the managed configuration must
* match the key field of the ManagedProperty. The field value must be compatible with the type of
* the ManagedProperty: *type* *JSON value* BOOL true or false STRING string INTEGER number CHOICE
* string MULTISELECT array of strings HIDDEN string BUNDLE_ARRAY array of objects
* @return value or {@code null} for none
*/
public java.util.Map getManagedConfiguration() {
return managedConfiguration;
}
/**
* Managed configuration applied to the app. The format for the configuration is dictated by the
* ManagedProperty values supported by the app. Each field name in the managed configuration must
* match the key field of the ManagedProperty. The field value must be compatible with the type of
* the ManagedProperty: *type* *JSON value* BOOL true or false STRING string INTEGER number CHOICE
* string MULTISELECT array of strings HIDDEN string BUNDLE_ARRAY array of objects
* @param managedConfiguration managedConfiguration or {@code null} for none
*/
public ApplicationPolicy setManagedConfiguration(java.util.Map managedConfiguration) {
this.managedConfiguration = managedConfiguration;
return this;
}
/**
* The managed configurations template for the app, saved from the managed configurations iframe.
* This field is ignored if managed_configuration is set.
* @return value or {@code null} for none
*/
public ManagedConfigurationTemplate getManagedConfigurationTemplate() {
return managedConfigurationTemplate;
}
/**
* The managed configurations template for the app, saved from the managed configurations iframe.
* This field is ignored if managed_configuration is set.
* @param managedConfigurationTemplate managedConfigurationTemplate or {@code null} for none
*/
public ApplicationPolicy setManagedConfigurationTemplate(ManagedConfigurationTemplate managedConfigurationTemplate) {
this.managedConfigurationTemplate = managedConfigurationTemplate;
return this;
}
/**
* The minimum version of the app that runs on the device. If set, the device attempts to update
* the app to at least this version code. If the app is not up-to-date, the device will contain a
* NonComplianceDetail with non_compliance_reason set to APP_NOT_UPDATED. The app must already be
* published to Google Play with a version code greater than or equal to this value. At most 20
* apps may specify a minimum version code per policy.
* @return value or {@code null} for none
*/
public java.lang.Integer getMinimumVersionCode() {
return minimumVersionCode;
}
/**
* The minimum version of the app that runs on the device. If set, the device attempts to update
* the app to at least this version code. If the app is not up-to-date, the device will contain a
* NonComplianceDetail with non_compliance_reason set to APP_NOT_UPDATED. The app must already be
* published to Google Play with a version code greater than or equal to this value. At most 20
* apps may specify a minimum version code per policy.
* @param minimumVersionCode minimumVersionCode or {@code null} for none
*/
public ApplicationPolicy setMinimumVersionCode(java.lang.Integer minimumVersionCode) {
this.minimumVersionCode = minimumVersionCode;
return this;
}
/**
* The package name of the app. For example, com.google.android.youtube for the YouTube app.
* @return value or {@code null} for none
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the app. For example, com.google.android.youtube for the YouTube app.
* @param packageName packageName or {@code null} for none
*/
public ApplicationPolicy setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/**
* Explicit permission grants or denials for the app. These values override the
* default_permission_policy and permission_grants which apply to all apps.
* @return value or {@code null} for none
*/
public java.util.List getPermissionGrants() {
return permissionGrants;
}
/**
* Explicit permission grants or denials for the app. These values override the
* default_permission_policy and permission_grants which apply to all apps.
* @param permissionGrants permissionGrants or {@code null} for none
*/
public ApplicationPolicy setPermissionGrants(java.util.List permissionGrants) {
this.permissionGrants = permissionGrants;
return this;
}
/**
* Optional. Specifies whether user control is permitted for the app. User control includes user
* actions like force-stopping and clearing app data. Supported on Android 11 and above.
* @return value or {@code null} for none
*/
public java.lang.String getUserControlSettings() {
return userControlSettings;
}
/**
* Optional. Specifies whether user control is permitted for the app. User control includes user
* actions like force-stopping and clearing app data. Supported on Android 11 and above.
* @param userControlSettings userControlSettings or {@code null} for none
*/
public ApplicationPolicy setUserControlSettings(java.lang.String userControlSettings) {
this.userControlSettings = userControlSettings;
return this;
}
/**
* Specifies whether the app installed in the work profile is allowed to add widgets to the home
* screen.
* @return value or {@code null} for none
*/
public java.lang.String getWorkProfileWidgets() {
return workProfileWidgets;
}
/**
* Specifies whether the app installed in the work profile is allowed to add widgets to the home
* screen.
* @param workProfileWidgets workProfileWidgets or {@code null} for none
*/
public ApplicationPolicy setWorkProfileWidgets(java.lang.String workProfileWidgets) {
this.workProfileWidgets = workProfileWidgets;
return this;
}
@Override
public ApplicationPolicy set(String fieldName, Object value) {
return (ApplicationPolicy) super.set(fieldName, value);
}
@Override
public ApplicationPolicy clone() {
return (ApplicationPolicy) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy