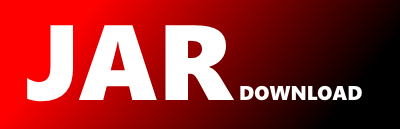
com.google.api.services.androidmanagement.v1.model.ChoosePrivateKeyRule Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Controls apps' access to private keys. The rule determines which private key, if any, Android
* Device Policy grants to the specified app. Access is granted either when the app calls
* KeyChain.choosePrivateKeyAlias (https://developer.android.com/reference/android/security/KeyChain
* #choosePrivateKeyAlias%28android.app.Activity,%20android.security.KeyChainAliasCallback,%20java.l
* ang.String[],%20java.security.Principal[],%20java.lang.String,%20int,%20java.lang.String%29) (or
* any overloads) to request a private key alias for a given URL, or for rules that are not URL-
* specific (that is, if urlPattern is not set, or set to the empty string or .*) on Android 11 and
* above, directly so that the app can call KeyChain.getPrivateKey (https://developer.android.com/re
* ference/android/security/KeyChain#getPrivateKey%28android.content.Context,%20java.lang.String%29)
* , without first having to call KeyChain.choosePrivateKeyAlias.When an app calls
* KeyChain.choosePrivateKeyAlias if more than one choosePrivateKeyRules matches, the last matching
* rule defines which key alias to return.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ChoosePrivateKeyRule extends com.google.api.client.json.GenericJson {
/**
* The package names to which this rule applies. The hash of the signing certificate for each app
* is verified against the hash provided by Play. If no package names are specified, then the
* alias is provided to all apps that call KeyChain.choosePrivateKeyAlias (https://developer.andro
* id.com/reference/android/security/KeyChain#choosePrivateKeyAlias%28android.app.Activity,%20andr
* oid.security.KeyChainAliasCallback,%20java.lang.String[],%20java.security.Principal[],%20java.l
* ang.String,%20int,%20java.lang.String%29) or any overloads (but not without calling
* KeyChain.choosePrivateKeyAlias, even on Android 11 and above). Any app with the same Android
* UID as a package specified here will have access when they call KeyChain.choosePrivateKeyAlias.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List packageNames;
/**
* The alias of the private key to be used.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String privateKeyAlias;
/**
* The URL pattern to match against the URL of the request. If not set or empty, it matches all
* URLs. This uses the regular expression syntax of java.util.regex.Pattern.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String urlPattern;
/**
* The package names to which this rule applies. The hash of the signing certificate for each app
* is verified against the hash provided by Play. If no package names are specified, then the
* alias is provided to all apps that call KeyChain.choosePrivateKeyAlias (https://developer.andro
* id.com/reference/android/security/KeyChain#choosePrivateKeyAlias%28android.app.Activity,%20andr
* oid.security.KeyChainAliasCallback,%20java.lang.String[],%20java.security.Principal[],%20java.l
* ang.String,%20int,%20java.lang.String%29) or any overloads (but not without calling
* KeyChain.choosePrivateKeyAlias, even on Android 11 and above). Any app with the same Android
* UID as a package specified here will have access when they call KeyChain.choosePrivateKeyAlias.
* @return value or {@code null} for none
*/
public java.util.List getPackageNames() {
return packageNames;
}
/**
* The package names to which this rule applies. The hash of the signing certificate for each app
* is verified against the hash provided by Play. If no package names are specified, then the
* alias is provided to all apps that call KeyChain.choosePrivateKeyAlias (https://developer.andro
* id.com/reference/android/security/KeyChain#choosePrivateKeyAlias%28android.app.Activity,%20andr
* oid.security.KeyChainAliasCallback,%20java.lang.String[],%20java.security.Principal[],%20java.l
* ang.String,%20int,%20java.lang.String%29) or any overloads (but not without calling
* KeyChain.choosePrivateKeyAlias, even on Android 11 and above). Any app with the same Android
* UID as a package specified here will have access when they call KeyChain.choosePrivateKeyAlias.
* @param packageNames packageNames or {@code null} for none
*/
public ChoosePrivateKeyRule setPackageNames(java.util.List packageNames) {
this.packageNames = packageNames;
return this;
}
/**
* The alias of the private key to be used.
* @return value or {@code null} for none
*/
public java.lang.String getPrivateKeyAlias() {
return privateKeyAlias;
}
/**
* The alias of the private key to be used.
* @param privateKeyAlias privateKeyAlias or {@code null} for none
*/
public ChoosePrivateKeyRule setPrivateKeyAlias(java.lang.String privateKeyAlias) {
this.privateKeyAlias = privateKeyAlias;
return this;
}
/**
* The URL pattern to match against the URL of the request. If not set or empty, it matches all
* URLs. This uses the regular expression syntax of java.util.regex.Pattern.
* @return value or {@code null} for none
*/
public java.lang.String getUrlPattern() {
return urlPattern;
}
/**
* The URL pattern to match against the URL of the request. If not set or empty, it matches all
* URLs. This uses the regular expression syntax of java.util.regex.Pattern.
* @param urlPattern urlPattern or {@code null} for none
*/
public ChoosePrivateKeyRule setUrlPattern(java.lang.String urlPattern) {
this.urlPattern = urlPattern;
return this;
}
@Override
public ChoosePrivateKeyRule set(String fieldName, Object value) {
return (ChoosePrivateKeyRule) super.set(fieldName, value);
}
@Override
public ChoosePrivateKeyRule clone() {
return (ChoosePrivateKeyRule) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy