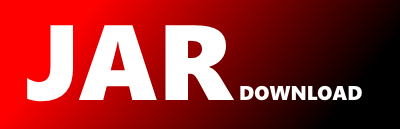
com.google.api.services.androidmanagement.v1.model.CrossProfilePolicies Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Controls the data from the work profile that can be accessed from the personal profile and vice
* versa. A nonComplianceDetail with MANAGEMENT_MODE is reported if the device does not have a work
* profile.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class CrossProfilePolicies extends com.google.api.client.json.GenericJson {
/**
* Whether text copied from one profile (personal or work) can be pasted in the other profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String crossProfileCopyPaste;
/**
* Whether data from one profile (personal or work) can be shared with apps in the other profile.
* Specifically controls simple data sharing via intents. Management of other cross-profile
* communication channels, such as contact search, copy/paste, or connected work & personal apps,
* are configured separately.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String crossProfileDataSharing;
/**
* List of apps which are excluded from the ShowWorkContactsInPersonalProfile setting. For this to
* be set, ShowWorkContactsInPersonalProfile must be set to one of the following values:
* SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_ALLOWED. In this case, these exemptions act as a
* blocklist. SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_DISALLOWED. In this case, these exemptions
* act as an allowlist. SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_DISALLOWED_EXCEPT_SYSTEM. In this
* case, these exemptions act as an allowlist, in addition to the already allowlisted system
* apps.Supported on Android 14 and above. A nonComplianceDetail with API_LEVEL is reported if the
* Android version is less than 14.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PackageNameList exemptionsToShowWorkContactsInPersonalProfile;
/**
* Whether personal apps can access contacts stored in the work profile.See also
* exemptions_to_show_work_contacts_in_personal_profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String showWorkContactsInPersonalProfile;
/**
* Specifies the default behaviour for work profile widgets. If the policy does not specify
* work_profile_widgets for a specific application, it will behave according to the value
* specified here.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String workProfileWidgetsDefault;
/**
* Whether text copied from one profile (personal or work) can be pasted in the other profile.
* @return value or {@code null} for none
*/
public java.lang.String getCrossProfileCopyPaste() {
return crossProfileCopyPaste;
}
/**
* Whether text copied from one profile (personal or work) can be pasted in the other profile.
* @param crossProfileCopyPaste crossProfileCopyPaste or {@code null} for none
*/
public CrossProfilePolicies setCrossProfileCopyPaste(java.lang.String crossProfileCopyPaste) {
this.crossProfileCopyPaste = crossProfileCopyPaste;
return this;
}
/**
* Whether data from one profile (personal or work) can be shared with apps in the other profile.
* Specifically controls simple data sharing via intents. Management of other cross-profile
* communication channels, such as contact search, copy/paste, or connected work & personal apps,
* are configured separately.
* @return value or {@code null} for none
*/
public java.lang.String getCrossProfileDataSharing() {
return crossProfileDataSharing;
}
/**
* Whether data from one profile (personal or work) can be shared with apps in the other profile.
* Specifically controls simple data sharing via intents. Management of other cross-profile
* communication channels, such as contact search, copy/paste, or connected work & personal apps,
* are configured separately.
* @param crossProfileDataSharing crossProfileDataSharing or {@code null} for none
*/
public CrossProfilePolicies setCrossProfileDataSharing(java.lang.String crossProfileDataSharing) {
this.crossProfileDataSharing = crossProfileDataSharing;
return this;
}
/**
* List of apps which are excluded from the ShowWorkContactsInPersonalProfile setting. For this to
* be set, ShowWorkContactsInPersonalProfile must be set to one of the following values:
* SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_ALLOWED. In this case, these exemptions act as a
* blocklist. SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_DISALLOWED. In this case, these exemptions
* act as an allowlist. SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_DISALLOWED_EXCEPT_SYSTEM. In this
* case, these exemptions act as an allowlist, in addition to the already allowlisted system
* apps.Supported on Android 14 and above. A nonComplianceDetail with API_LEVEL is reported if the
* Android version is less than 14.
* @return value or {@code null} for none
*/
public PackageNameList getExemptionsToShowWorkContactsInPersonalProfile() {
return exemptionsToShowWorkContactsInPersonalProfile;
}
/**
* List of apps which are excluded from the ShowWorkContactsInPersonalProfile setting. For this to
* be set, ShowWorkContactsInPersonalProfile must be set to one of the following values:
* SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_ALLOWED. In this case, these exemptions act as a
* blocklist. SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_DISALLOWED. In this case, these exemptions
* act as an allowlist. SHOW_WORK_CONTACTS_IN_PERSONAL_PROFILE_DISALLOWED_EXCEPT_SYSTEM. In this
* case, these exemptions act as an allowlist, in addition to the already allowlisted system
* apps.Supported on Android 14 and above. A nonComplianceDetail with API_LEVEL is reported if the
* Android version is less than 14.
* @param exemptionsToShowWorkContactsInPersonalProfile exemptionsToShowWorkContactsInPersonalProfile or {@code null} for none
*/
public CrossProfilePolicies setExemptionsToShowWorkContactsInPersonalProfile(PackageNameList exemptionsToShowWorkContactsInPersonalProfile) {
this.exemptionsToShowWorkContactsInPersonalProfile = exemptionsToShowWorkContactsInPersonalProfile;
return this;
}
/**
* Whether personal apps can access contacts stored in the work profile.See also
* exemptions_to_show_work_contacts_in_personal_profile.
* @return value or {@code null} for none
*/
public java.lang.String getShowWorkContactsInPersonalProfile() {
return showWorkContactsInPersonalProfile;
}
/**
* Whether personal apps can access contacts stored in the work profile.See also
* exemptions_to_show_work_contacts_in_personal_profile.
* @param showWorkContactsInPersonalProfile showWorkContactsInPersonalProfile or {@code null} for none
*/
public CrossProfilePolicies setShowWorkContactsInPersonalProfile(java.lang.String showWorkContactsInPersonalProfile) {
this.showWorkContactsInPersonalProfile = showWorkContactsInPersonalProfile;
return this;
}
/**
* Specifies the default behaviour for work profile widgets. If the policy does not specify
* work_profile_widgets for a specific application, it will behave according to the value
* specified here.
* @return value or {@code null} for none
*/
public java.lang.String getWorkProfileWidgetsDefault() {
return workProfileWidgetsDefault;
}
/**
* Specifies the default behaviour for work profile widgets. If the policy does not specify
* work_profile_widgets for a specific application, it will behave according to the value
* specified here.
* @param workProfileWidgetsDefault workProfileWidgetsDefault or {@code null} for none
*/
public CrossProfilePolicies setWorkProfileWidgetsDefault(java.lang.String workProfileWidgetsDefault) {
this.workProfileWidgetsDefault = workProfileWidgetsDefault;
return this;
}
@Override
public CrossProfilePolicies set(String fieldName, Object value) {
return (CrossProfilePolicies) super.set(fieldName, value);
}
@Override
public CrossProfilePolicies clone() {
return (CrossProfilePolicies) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy