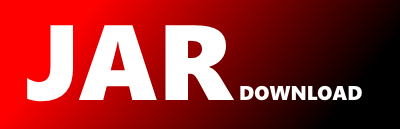
com.google.api.services.androidmanagement.v1.model.DeviceConnectivityManagement Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Covers controls for device connectivity such as Wi-Fi, USB data access, keyboard/mouse
* connections, and more.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DeviceConnectivityManagement extends com.google.api.client.json.GenericJson {
/**
* Controls Wi-Fi configuring privileges. Based on the option set, user will have either full or
* limited or no control in configuring Wi-Fi networks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String configureWifi;
/**
* Controls tethering settings. Based on the value set, the user is partially or fully disallowed
* from using different forms of tethering.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String tetheringSettings;
/**
* Controls what files and/or data can be transferred via USB. Supported only on company-owned
* devices.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String usbDataAccess;
/**
* Controls configuring and using Wi-Fi direct settings. Supported on company-owned devices
* running Android 13 and above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String wifiDirectSettings;
/**
* Optional. Wi-Fi roaming policy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WifiRoamingPolicy wifiRoamingPolicy;
/**
* Restrictions on which Wi-Fi SSIDs the device can connect to. Note that this does not affect
* which networks can be configured on the device. Supported on company-owned devices running
* Android 13 and above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WifiSsidPolicy wifiSsidPolicy;
/**
* Controls Wi-Fi configuring privileges. Based on the option set, user will have either full or
* limited or no control in configuring Wi-Fi networks.
* @return value or {@code null} for none
*/
public java.lang.String getConfigureWifi() {
return configureWifi;
}
/**
* Controls Wi-Fi configuring privileges. Based on the option set, user will have either full or
* limited or no control in configuring Wi-Fi networks.
* @param configureWifi configureWifi or {@code null} for none
*/
public DeviceConnectivityManagement setConfigureWifi(java.lang.String configureWifi) {
this.configureWifi = configureWifi;
return this;
}
/**
* Controls tethering settings. Based on the value set, the user is partially or fully disallowed
* from using different forms of tethering.
* @return value or {@code null} for none
*/
public java.lang.String getTetheringSettings() {
return tetheringSettings;
}
/**
* Controls tethering settings. Based on the value set, the user is partially or fully disallowed
* from using different forms of tethering.
* @param tetheringSettings tetheringSettings or {@code null} for none
*/
public DeviceConnectivityManagement setTetheringSettings(java.lang.String tetheringSettings) {
this.tetheringSettings = tetheringSettings;
return this;
}
/**
* Controls what files and/or data can be transferred via USB. Supported only on company-owned
* devices.
* @return value or {@code null} for none
*/
public java.lang.String getUsbDataAccess() {
return usbDataAccess;
}
/**
* Controls what files and/or data can be transferred via USB. Supported only on company-owned
* devices.
* @param usbDataAccess usbDataAccess or {@code null} for none
*/
public DeviceConnectivityManagement setUsbDataAccess(java.lang.String usbDataAccess) {
this.usbDataAccess = usbDataAccess;
return this;
}
/**
* Controls configuring and using Wi-Fi direct settings. Supported on company-owned devices
* running Android 13 and above.
* @return value or {@code null} for none
*/
public java.lang.String getWifiDirectSettings() {
return wifiDirectSettings;
}
/**
* Controls configuring and using Wi-Fi direct settings. Supported on company-owned devices
* running Android 13 and above.
* @param wifiDirectSettings wifiDirectSettings or {@code null} for none
*/
public DeviceConnectivityManagement setWifiDirectSettings(java.lang.String wifiDirectSettings) {
this.wifiDirectSettings = wifiDirectSettings;
return this;
}
/**
* Optional. Wi-Fi roaming policy.
* @return value or {@code null} for none
*/
public WifiRoamingPolicy getWifiRoamingPolicy() {
return wifiRoamingPolicy;
}
/**
* Optional. Wi-Fi roaming policy.
* @param wifiRoamingPolicy wifiRoamingPolicy or {@code null} for none
*/
public DeviceConnectivityManagement setWifiRoamingPolicy(WifiRoamingPolicy wifiRoamingPolicy) {
this.wifiRoamingPolicy = wifiRoamingPolicy;
return this;
}
/**
* Restrictions on which Wi-Fi SSIDs the device can connect to. Note that this does not affect
* which networks can be configured on the device. Supported on company-owned devices running
* Android 13 and above.
* @return value or {@code null} for none
*/
public WifiSsidPolicy getWifiSsidPolicy() {
return wifiSsidPolicy;
}
/**
* Restrictions on which Wi-Fi SSIDs the device can connect to. Note that this does not affect
* which networks can be configured on the device. Supported on company-owned devices running
* Android 13 and above.
* @param wifiSsidPolicy wifiSsidPolicy or {@code null} for none
*/
public DeviceConnectivityManagement setWifiSsidPolicy(WifiSsidPolicy wifiSsidPolicy) {
this.wifiSsidPolicy = wifiSsidPolicy;
return this;
}
@Override
public DeviceConnectivityManagement set(String fieldName, Object value) {
return (DeviceConnectivityManagement) super.set(fieldName, value);
}
@Override
public DeviceConnectivityManagement clone() {
return (DeviceConnectivityManagement) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy