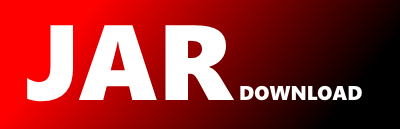
com.google.api.services.androidmanagement.v1.model.HardwareInfo Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Information about device hardware. The fields related to temperature thresholds are only
* available if hardwareStatusEnabled is true in the device's policy.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class HardwareInfo extends com.google.api.client.json.GenericJson {
/**
* Battery shutdown temperature thresholds in Celsius for each battery on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List batteryShutdownTemperatures;
/**
* Battery throttling temperature thresholds in Celsius for each battery on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List batteryThrottlingTemperatures;
/**
* Brand of the device. For example, Google.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String brand;
/**
* CPU shutdown temperature thresholds in Celsius for each CPU on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List cpuShutdownTemperatures;
/**
* CPU throttling temperature thresholds in Celsius for each CPU on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List cpuThrottlingTemperatures;
/**
* Baseband version. For example, MDM9625_104662.22.05.34p.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String deviceBasebandVersion;
/**
* Output only. ID that uniquely identifies a personally-owned device in a particular
* organization. On the same physical device when enrolled with the same organization, this ID
* persists across setups and even factory resets. This ID is available on personally-owned
* devices with a work profile on devices running Android 12 and above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String enterpriseSpecificId;
/**
* GPU shutdown temperature thresholds in Celsius for each GPU on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List gpuShutdownTemperatures;
/**
* GPU throttling temperature thresholds in Celsius for each GPU on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List gpuThrottlingTemperatures;
/**
* Name of the hardware. For example, Angler.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String hardware;
/**
* Manufacturer. For example, Motorola.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String manufacturer;
/**
* The model of the device. For example, Asus Nexus 7.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String model;
/**
* The device serial number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serialNumber;
/**
* Device skin shutdown temperature thresholds in Celsius.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List skinShutdownTemperatures;
/**
* Device skin throttling temperature thresholds in Celsius.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List skinThrottlingTemperatures;
/**
* Battery shutdown temperature thresholds in Celsius for each battery on the device.
* @return value or {@code null} for none
*/
public java.util.List getBatteryShutdownTemperatures() {
return batteryShutdownTemperatures;
}
/**
* Battery shutdown temperature thresholds in Celsius for each battery on the device.
* @param batteryShutdownTemperatures batteryShutdownTemperatures or {@code null} for none
*/
public HardwareInfo setBatteryShutdownTemperatures(java.util.List batteryShutdownTemperatures) {
this.batteryShutdownTemperatures = batteryShutdownTemperatures;
return this;
}
/**
* Battery throttling temperature thresholds in Celsius for each battery on the device.
* @return value or {@code null} for none
*/
public java.util.List getBatteryThrottlingTemperatures() {
return batteryThrottlingTemperatures;
}
/**
* Battery throttling temperature thresholds in Celsius for each battery on the device.
* @param batteryThrottlingTemperatures batteryThrottlingTemperatures or {@code null} for none
*/
public HardwareInfo setBatteryThrottlingTemperatures(java.util.List batteryThrottlingTemperatures) {
this.batteryThrottlingTemperatures = batteryThrottlingTemperatures;
return this;
}
/**
* Brand of the device. For example, Google.
* @return value or {@code null} for none
*/
public java.lang.String getBrand() {
return brand;
}
/**
* Brand of the device. For example, Google.
* @param brand brand or {@code null} for none
*/
public HardwareInfo setBrand(java.lang.String brand) {
this.brand = brand;
return this;
}
/**
* CPU shutdown temperature thresholds in Celsius for each CPU on the device.
* @return value or {@code null} for none
*/
public java.util.List getCpuShutdownTemperatures() {
return cpuShutdownTemperatures;
}
/**
* CPU shutdown temperature thresholds in Celsius for each CPU on the device.
* @param cpuShutdownTemperatures cpuShutdownTemperatures or {@code null} for none
*/
public HardwareInfo setCpuShutdownTemperatures(java.util.List cpuShutdownTemperatures) {
this.cpuShutdownTemperatures = cpuShutdownTemperatures;
return this;
}
/**
* CPU throttling temperature thresholds in Celsius for each CPU on the device.
* @return value or {@code null} for none
*/
public java.util.List getCpuThrottlingTemperatures() {
return cpuThrottlingTemperatures;
}
/**
* CPU throttling temperature thresholds in Celsius for each CPU on the device.
* @param cpuThrottlingTemperatures cpuThrottlingTemperatures or {@code null} for none
*/
public HardwareInfo setCpuThrottlingTemperatures(java.util.List cpuThrottlingTemperatures) {
this.cpuThrottlingTemperatures = cpuThrottlingTemperatures;
return this;
}
/**
* Baseband version. For example, MDM9625_104662.22.05.34p.
* @return value or {@code null} for none
*/
public java.lang.String getDeviceBasebandVersion() {
return deviceBasebandVersion;
}
/**
* Baseband version. For example, MDM9625_104662.22.05.34p.
* @param deviceBasebandVersion deviceBasebandVersion or {@code null} for none
*/
public HardwareInfo setDeviceBasebandVersion(java.lang.String deviceBasebandVersion) {
this.deviceBasebandVersion = deviceBasebandVersion;
return this;
}
/**
* Output only. ID that uniquely identifies a personally-owned device in a particular
* organization. On the same physical device when enrolled with the same organization, this ID
* persists across setups and even factory resets. This ID is available on personally-owned
* devices with a work profile on devices running Android 12 and above.
* @return value or {@code null} for none
*/
public java.lang.String getEnterpriseSpecificId() {
return enterpriseSpecificId;
}
/**
* Output only. ID that uniquely identifies a personally-owned device in a particular
* organization. On the same physical device when enrolled with the same organization, this ID
* persists across setups and even factory resets. This ID is available on personally-owned
* devices with a work profile on devices running Android 12 and above.
* @param enterpriseSpecificId enterpriseSpecificId or {@code null} for none
*/
public HardwareInfo setEnterpriseSpecificId(java.lang.String enterpriseSpecificId) {
this.enterpriseSpecificId = enterpriseSpecificId;
return this;
}
/**
* GPU shutdown temperature thresholds in Celsius for each GPU on the device.
* @return value or {@code null} for none
*/
public java.util.List getGpuShutdownTemperatures() {
return gpuShutdownTemperatures;
}
/**
* GPU shutdown temperature thresholds in Celsius for each GPU on the device.
* @param gpuShutdownTemperatures gpuShutdownTemperatures or {@code null} for none
*/
public HardwareInfo setGpuShutdownTemperatures(java.util.List gpuShutdownTemperatures) {
this.gpuShutdownTemperatures = gpuShutdownTemperatures;
return this;
}
/**
* GPU throttling temperature thresholds in Celsius for each GPU on the device.
* @return value or {@code null} for none
*/
public java.util.List getGpuThrottlingTemperatures() {
return gpuThrottlingTemperatures;
}
/**
* GPU throttling temperature thresholds in Celsius for each GPU on the device.
* @param gpuThrottlingTemperatures gpuThrottlingTemperatures or {@code null} for none
*/
public HardwareInfo setGpuThrottlingTemperatures(java.util.List gpuThrottlingTemperatures) {
this.gpuThrottlingTemperatures = gpuThrottlingTemperatures;
return this;
}
/**
* Name of the hardware. For example, Angler.
* @return value or {@code null} for none
*/
public java.lang.String getHardware() {
return hardware;
}
/**
* Name of the hardware. For example, Angler.
* @param hardware hardware or {@code null} for none
*/
public HardwareInfo setHardware(java.lang.String hardware) {
this.hardware = hardware;
return this;
}
/**
* Manufacturer. For example, Motorola.
* @return value or {@code null} for none
*/
public java.lang.String getManufacturer() {
return manufacturer;
}
/**
* Manufacturer. For example, Motorola.
* @param manufacturer manufacturer or {@code null} for none
*/
public HardwareInfo setManufacturer(java.lang.String manufacturer) {
this.manufacturer = manufacturer;
return this;
}
/**
* The model of the device. For example, Asus Nexus 7.
* @return value or {@code null} for none
*/
public java.lang.String getModel() {
return model;
}
/**
* The model of the device. For example, Asus Nexus 7.
* @param model model or {@code null} for none
*/
public HardwareInfo setModel(java.lang.String model) {
this.model = model;
return this;
}
/**
* The device serial number.
* @return value or {@code null} for none
*/
public java.lang.String getSerialNumber() {
return serialNumber;
}
/**
* The device serial number.
* @param serialNumber serialNumber or {@code null} for none
*/
public HardwareInfo setSerialNumber(java.lang.String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
/**
* Device skin shutdown temperature thresholds in Celsius.
* @return value or {@code null} for none
*/
public java.util.List getSkinShutdownTemperatures() {
return skinShutdownTemperatures;
}
/**
* Device skin shutdown temperature thresholds in Celsius.
* @param skinShutdownTemperatures skinShutdownTemperatures or {@code null} for none
*/
public HardwareInfo setSkinShutdownTemperatures(java.util.List skinShutdownTemperatures) {
this.skinShutdownTemperatures = skinShutdownTemperatures;
return this;
}
/**
* Device skin throttling temperature thresholds in Celsius.
* @return value or {@code null} for none
*/
public java.util.List getSkinThrottlingTemperatures() {
return skinThrottlingTemperatures;
}
/**
* Device skin throttling temperature thresholds in Celsius.
* @param skinThrottlingTemperatures skinThrottlingTemperatures or {@code null} for none
*/
public HardwareInfo setSkinThrottlingTemperatures(java.util.List skinThrottlingTemperatures) {
this.skinThrottlingTemperatures = skinThrottlingTemperatures;
return this;
}
@Override
public HardwareInfo set(String fieldName, Object value) {
return (HardwareInfo) super.set(fieldName, value);
}
@Override
public HardwareInfo clone() {
return (HardwareInfo) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy