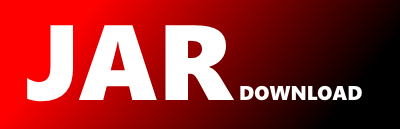
com.google.api.services.androidmanagement.v1.model.ProvisioningInfo Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Information about a device that is available during setup.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ProvisioningInfo extends com.google.api.client.json.GenericJson {
/**
* The API level of the Android platform version running on the device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer apiLevel;
/**
* The email address of the authenticated user (only present for Google Account provisioning
* method).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String authenticatedUserEmail;
/**
* The brand of the device. For example, Google.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String brand;
/**
* The name of the enterprise in the form enterprises/{enterprise}.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String enterprise;
/**
* For corporate-owned devices, IMEI number of the GSM device. For example, A1000031212.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imei;
/**
* The management mode of the device or profile.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String managementMode;
/**
* For corporate-owned devices, MEID number of the CDMA device. For example, A00000292788E1.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String meid;
/**
* The model of the device. For example, Asus Nexus 7.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String model;
/**
* The name of this resource in the form provisioningInfo/{provisioning_info}.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Ownership of the managed device.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ownership;
/**
* For corporate-owned devices, The device serial number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serialNumber;
/**
* The API level of the Android platform version running on the device.
* @return value or {@code null} for none
*/
public java.lang.Integer getApiLevel() {
return apiLevel;
}
/**
* The API level of the Android platform version running on the device.
* @param apiLevel apiLevel or {@code null} for none
*/
public ProvisioningInfo setApiLevel(java.lang.Integer apiLevel) {
this.apiLevel = apiLevel;
return this;
}
/**
* The email address of the authenticated user (only present for Google Account provisioning
* method).
* @return value or {@code null} for none
*/
public java.lang.String getAuthenticatedUserEmail() {
return authenticatedUserEmail;
}
/**
* The email address of the authenticated user (only present for Google Account provisioning
* method).
* @param authenticatedUserEmail authenticatedUserEmail or {@code null} for none
*/
public ProvisioningInfo setAuthenticatedUserEmail(java.lang.String authenticatedUserEmail) {
this.authenticatedUserEmail = authenticatedUserEmail;
return this;
}
/**
* The brand of the device. For example, Google.
* @return value or {@code null} for none
*/
public java.lang.String getBrand() {
return brand;
}
/**
* The brand of the device. For example, Google.
* @param brand brand or {@code null} for none
*/
public ProvisioningInfo setBrand(java.lang.String brand) {
this.brand = brand;
return this;
}
/**
* The name of the enterprise in the form enterprises/{enterprise}.
* @return value or {@code null} for none
*/
public java.lang.String getEnterprise() {
return enterprise;
}
/**
* The name of the enterprise in the form enterprises/{enterprise}.
* @param enterprise enterprise or {@code null} for none
*/
public ProvisioningInfo setEnterprise(java.lang.String enterprise) {
this.enterprise = enterprise;
return this;
}
/**
* For corporate-owned devices, IMEI number of the GSM device. For example, A1000031212.
* @return value or {@code null} for none
*/
public java.lang.String getImei() {
return imei;
}
/**
* For corporate-owned devices, IMEI number of the GSM device. For example, A1000031212.
* @param imei imei or {@code null} for none
*/
public ProvisioningInfo setImei(java.lang.String imei) {
this.imei = imei;
return this;
}
/**
* The management mode of the device or profile.
* @return value or {@code null} for none
*/
public java.lang.String getManagementMode() {
return managementMode;
}
/**
* The management mode of the device or profile.
* @param managementMode managementMode or {@code null} for none
*/
public ProvisioningInfo setManagementMode(java.lang.String managementMode) {
this.managementMode = managementMode;
return this;
}
/**
* For corporate-owned devices, MEID number of the CDMA device. For example, A00000292788E1.
* @return value or {@code null} for none
*/
public java.lang.String getMeid() {
return meid;
}
/**
* For corporate-owned devices, MEID number of the CDMA device. For example, A00000292788E1.
* @param meid meid or {@code null} for none
*/
public ProvisioningInfo setMeid(java.lang.String meid) {
this.meid = meid;
return this;
}
/**
* The model of the device. For example, Asus Nexus 7.
* @return value or {@code null} for none
*/
public java.lang.String getModel() {
return model;
}
/**
* The model of the device. For example, Asus Nexus 7.
* @param model model or {@code null} for none
*/
public ProvisioningInfo setModel(java.lang.String model) {
this.model = model;
return this;
}
/**
* The name of this resource in the form provisioningInfo/{provisioning_info}.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The name of this resource in the form provisioningInfo/{provisioning_info}.
* @param name name or {@code null} for none
*/
public ProvisioningInfo setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Ownership of the managed device.
* @return value or {@code null} for none
*/
public java.lang.String getOwnership() {
return ownership;
}
/**
* Ownership of the managed device.
* @param ownership ownership or {@code null} for none
*/
public ProvisioningInfo setOwnership(java.lang.String ownership) {
this.ownership = ownership;
return this;
}
/**
* For corporate-owned devices, The device serial number.
* @return value or {@code null} for none
*/
public java.lang.String getSerialNumber() {
return serialNumber;
}
/**
* For corporate-owned devices, The device serial number.
* @param serialNumber serialNumber or {@code null} for none
*/
public ProvisioningInfo setSerialNumber(java.lang.String serialNumber) {
this.serialNumber = serialNumber;
return this;
}
@Override
public ProvisioningInfo set(String fieldName, Object value) {
return (ProvisioningInfo) super.set(fieldName, value);
}
@Override
public ProvisioningInfo clone() {
return (ProvisioningInfo) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy