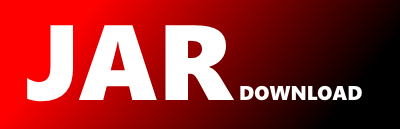
com.google.api.services.androidmanagement.v1.model.ScreenTimeoutSettings Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Controls the screen timeout settings.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ScreenTimeoutSettings extends com.google.api.client.json.GenericJson {
/**
* Optional. Controls the screen timeout duration. The screen timeout duration must be greater
* than 0, otherwise it is rejected. Additionally, it should not be greater than
* maximumTimeToLock, otherwise the screen timeout is set to maximumTimeToLock and a
* NonComplianceDetail with INVALID_VALUE reason and
* SCREEN_TIMEOUT_GREATER_THAN_MAXIMUM_TIME_TO_LOCK specific reason is reported. If the screen
* timeout is less than a certain lower bound, it is set to the lower bound. The lower bound may
* vary across devices. If this is set, screenTimeoutMode must be SCREEN_TIMEOUT_ENFORCED.
* Supported on Android 9 and above on fully managed devices. A NonComplianceDetail with API_LEVEL
* is reported if the Android version is less than 9. Supported on work profiles on company-owned
* devices on Android 15 and above.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String screenTimeout;
/**
* Optional. Controls whether the user is allowed to configure the screen timeout.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String screenTimeoutMode;
/**
* Optional. Controls the screen timeout duration. The screen timeout duration must be greater
* than 0, otherwise it is rejected. Additionally, it should not be greater than
* maximumTimeToLock, otherwise the screen timeout is set to maximumTimeToLock and a
* NonComplianceDetail with INVALID_VALUE reason and
* SCREEN_TIMEOUT_GREATER_THAN_MAXIMUM_TIME_TO_LOCK specific reason is reported. If the screen
* timeout is less than a certain lower bound, it is set to the lower bound. The lower bound may
* vary across devices. If this is set, screenTimeoutMode must be SCREEN_TIMEOUT_ENFORCED.
* Supported on Android 9 and above on fully managed devices. A NonComplianceDetail with API_LEVEL
* is reported if the Android version is less than 9. Supported on work profiles on company-owned
* devices on Android 15 and above.
* @return value or {@code null} for none
*/
public String getScreenTimeout() {
return screenTimeout;
}
/**
* Optional. Controls the screen timeout duration. The screen timeout duration must be greater
* than 0, otherwise it is rejected. Additionally, it should not be greater than
* maximumTimeToLock, otherwise the screen timeout is set to maximumTimeToLock and a
* NonComplianceDetail with INVALID_VALUE reason and
* SCREEN_TIMEOUT_GREATER_THAN_MAXIMUM_TIME_TO_LOCK specific reason is reported. If the screen
* timeout is less than a certain lower bound, it is set to the lower bound. The lower bound may
* vary across devices. If this is set, screenTimeoutMode must be SCREEN_TIMEOUT_ENFORCED.
* Supported on Android 9 and above on fully managed devices. A NonComplianceDetail with API_LEVEL
* is reported if the Android version is less than 9. Supported on work profiles on company-owned
* devices on Android 15 and above.
* @param screenTimeout screenTimeout or {@code null} for none
*/
public ScreenTimeoutSettings setScreenTimeout(String screenTimeout) {
this.screenTimeout = screenTimeout;
return this;
}
/**
* Optional. Controls whether the user is allowed to configure the screen timeout.
* @return value or {@code null} for none
*/
public java.lang.String getScreenTimeoutMode() {
return screenTimeoutMode;
}
/**
* Optional. Controls whether the user is allowed to configure the screen timeout.
* @param screenTimeoutMode screenTimeoutMode or {@code null} for none
*/
public ScreenTimeoutSettings setScreenTimeoutMode(java.lang.String screenTimeoutMode) {
this.screenTimeoutMode = screenTimeoutMode;
return this;
}
@Override
public ScreenTimeoutSettings set(String fieldName, Object value) {
return (ScreenTimeoutSettings) super.set(fieldName, value);
}
@Override
public ScreenTimeoutSettings clone() {
return (ScreenTimeoutSettings) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy