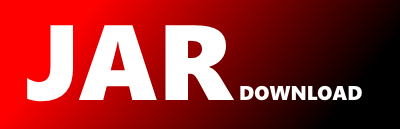
com.google.api.services.androidmanagement.v1.model.StatusReportingSettings Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Settings controlling the behavior of status reports.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class StatusReportingSettings extends com.google.api.client.json.GenericJson {
/**
* Application reporting settings. Only applicable if application_reports_enabled is true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ApplicationReportingSettings applicationReportingSettings;
/**
* Whether app reports are enabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean applicationReportsEnabled;
/**
* Whether Common Criteria Mode reporting is enabled. This is supported only on company-owned
* devices.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean commonCriteriaModeEnabled;
/**
* Whether device settings reporting is enabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean deviceSettingsEnabled;
/**
* Whether displays reporting is enabled. Report data is not available for personally owned
* devices with work profiles.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean displayInfoEnabled;
/**
* Whether hardware status reporting is enabled. Report data is not available for personally owned
* devices with work profiles.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean hardwareStatusEnabled;
/**
* Whether memory event reporting is enabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean memoryInfoEnabled;
/**
* Whether network info reporting is enabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean networkInfoEnabled;
/**
* Whether power management event reporting is enabled. Report data is not available for
* personally owned devices with work profiles.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean powerManagementEventsEnabled;
/**
* Whether software info reporting is enabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean softwareInfoEnabled;
/**
* Whether system properties reporting is enabled.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean systemPropertiesEnabled;
/**
* Application reporting settings. Only applicable if application_reports_enabled is true.
* @return value or {@code null} for none
*/
public ApplicationReportingSettings getApplicationReportingSettings() {
return applicationReportingSettings;
}
/**
* Application reporting settings. Only applicable if application_reports_enabled is true.
* @param applicationReportingSettings applicationReportingSettings or {@code null} for none
*/
public StatusReportingSettings setApplicationReportingSettings(ApplicationReportingSettings applicationReportingSettings) {
this.applicationReportingSettings = applicationReportingSettings;
return this;
}
/**
* Whether app reports are enabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getApplicationReportsEnabled() {
return applicationReportsEnabled;
}
/**
* Whether app reports are enabled.
* @param applicationReportsEnabled applicationReportsEnabled or {@code null} for none
*/
public StatusReportingSettings setApplicationReportsEnabled(java.lang.Boolean applicationReportsEnabled) {
this.applicationReportsEnabled = applicationReportsEnabled;
return this;
}
/**
* Whether Common Criteria Mode reporting is enabled. This is supported only on company-owned
* devices.
* @return value or {@code null} for none
*/
public java.lang.Boolean getCommonCriteriaModeEnabled() {
return commonCriteriaModeEnabled;
}
/**
* Whether Common Criteria Mode reporting is enabled. This is supported only on company-owned
* devices.
* @param commonCriteriaModeEnabled commonCriteriaModeEnabled or {@code null} for none
*/
public StatusReportingSettings setCommonCriteriaModeEnabled(java.lang.Boolean commonCriteriaModeEnabled) {
this.commonCriteriaModeEnabled = commonCriteriaModeEnabled;
return this;
}
/**
* Whether device settings reporting is enabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDeviceSettingsEnabled() {
return deviceSettingsEnabled;
}
/**
* Whether device settings reporting is enabled.
* @param deviceSettingsEnabled deviceSettingsEnabled or {@code null} for none
*/
public StatusReportingSettings setDeviceSettingsEnabled(java.lang.Boolean deviceSettingsEnabled) {
this.deviceSettingsEnabled = deviceSettingsEnabled;
return this;
}
/**
* Whether displays reporting is enabled. Report data is not available for personally owned
* devices with work profiles.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDisplayInfoEnabled() {
return displayInfoEnabled;
}
/**
* Whether displays reporting is enabled. Report data is not available for personally owned
* devices with work profiles.
* @param displayInfoEnabled displayInfoEnabled or {@code null} for none
*/
public StatusReportingSettings setDisplayInfoEnabled(java.lang.Boolean displayInfoEnabled) {
this.displayInfoEnabled = displayInfoEnabled;
return this;
}
/**
* Whether hardware status reporting is enabled. Report data is not available for personally owned
* devices with work profiles.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHardwareStatusEnabled() {
return hardwareStatusEnabled;
}
/**
* Whether hardware status reporting is enabled. Report data is not available for personally owned
* devices with work profiles.
* @param hardwareStatusEnabled hardwareStatusEnabled or {@code null} for none
*/
public StatusReportingSettings setHardwareStatusEnabled(java.lang.Boolean hardwareStatusEnabled) {
this.hardwareStatusEnabled = hardwareStatusEnabled;
return this;
}
/**
* Whether memory event reporting is enabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getMemoryInfoEnabled() {
return memoryInfoEnabled;
}
/**
* Whether memory event reporting is enabled.
* @param memoryInfoEnabled memoryInfoEnabled or {@code null} for none
*/
public StatusReportingSettings setMemoryInfoEnabled(java.lang.Boolean memoryInfoEnabled) {
this.memoryInfoEnabled = memoryInfoEnabled;
return this;
}
/**
* Whether network info reporting is enabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNetworkInfoEnabled() {
return networkInfoEnabled;
}
/**
* Whether network info reporting is enabled.
* @param networkInfoEnabled networkInfoEnabled or {@code null} for none
*/
public StatusReportingSettings setNetworkInfoEnabled(java.lang.Boolean networkInfoEnabled) {
this.networkInfoEnabled = networkInfoEnabled;
return this;
}
/**
* Whether power management event reporting is enabled. Report data is not available for
* personally owned devices with work profiles.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPowerManagementEventsEnabled() {
return powerManagementEventsEnabled;
}
/**
* Whether power management event reporting is enabled. Report data is not available for
* personally owned devices with work profiles.
* @param powerManagementEventsEnabled powerManagementEventsEnabled or {@code null} for none
*/
public StatusReportingSettings setPowerManagementEventsEnabled(java.lang.Boolean powerManagementEventsEnabled) {
this.powerManagementEventsEnabled = powerManagementEventsEnabled;
return this;
}
/**
* Whether software info reporting is enabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSoftwareInfoEnabled() {
return softwareInfoEnabled;
}
/**
* Whether software info reporting is enabled.
* @param softwareInfoEnabled softwareInfoEnabled or {@code null} for none
*/
public StatusReportingSettings setSoftwareInfoEnabled(java.lang.Boolean softwareInfoEnabled) {
this.softwareInfoEnabled = softwareInfoEnabled;
return this;
}
/**
* Whether system properties reporting is enabled.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSystemPropertiesEnabled() {
return systemPropertiesEnabled;
}
/**
* Whether system properties reporting is enabled.
* @param systemPropertiesEnabled systemPropertiesEnabled or {@code null} for none
*/
public StatusReportingSettings setSystemPropertiesEnabled(java.lang.Boolean systemPropertiesEnabled) {
this.systemPropertiesEnabled = systemPropertiesEnabled;
return this;
}
@Override
public StatusReportingSettings set(String fieldName, Object value) {
return (StatusReportingSettings) super.set(fieldName, value);
}
@Override
public StatusReportingSettings clone() {
return (StatusReportingSettings) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy