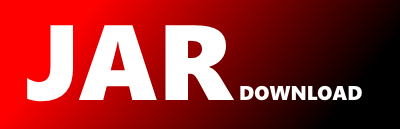
com.google.api.services.androidmanagement.v1.model.ApplicationReport Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* Information reported about an installed app.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ApplicationReport extends com.google.api.client.json.GenericJson {
/**
* The source of the package.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String applicationSource;
/**
* The display name of the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* The list of app events which have occurred in the last 30 hours.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List events;
static {
// hack to force ProGuard to consider ApplicationEvent used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ApplicationEvent.class);
}
/**
* The package name of the app that installed this app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String installerPackageName;
/**
* List of keyed app states reported by the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List keyedAppStates;
/**
* Package name of the app.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/**
* The SHA-256 hash of the app's APK file, which can be used to verify the app hasn't been
* modified. Each byte of the hash value is represented as a two-digit hexadecimal number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String packageSha256Hash;
/**
* The SHA-1 hash of each android.content.pm.Signature
* (https://developer.android.com/reference/android/content/pm/Signature.html) associated with the
* app package. Each byte of each hash value is represented as a two-digit hexadecimal number.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List signingKeyCertFingerprints;
/**
* Application state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Whether the app is user facing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String userFacingType;
/**
* The app version code, which can be used to determine whether one version is more recent than
* another.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer versionCode;
/**
* The app version as displayed to the user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String versionName;
/**
* The source of the package.
* @return value or {@code null} for none
*/
public java.lang.String getApplicationSource() {
return applicationSource;
}
/**
* The source of the package.
* @param applicationSource applicationSource or {@code null} for none
*/
public ApplicationReport setApplicationSource(java.lang.String applicationSource) {
this.applicationSource = applicationSource;
return this;
}
/**
* The display name of the app.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* The display name of the app.
* @param displayName displayName or {@code null} for none
*/
public ApplicationReport setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* The list of app events which have occurred in the last 30 hours.
* @return value or {@code null} for none
*/
public java.util.List getEvents() {
return events;
}
/**
* The list of app events which have occurred in the last 30 hours.
* @param events events or {@code null} for none
*/
public ApplicationReport setEvents(java.util.List events) {
this.events = events;
return this;
}
/**
* The package name of the app that installed this app.
* @return value or {@code null} for none
*/
public java.lang.String getInstallerPackageName() {
return installerPackageName;
}
/**
* The package name of the app that installed this app.
* @param installerPackageName installerPackageName or {@code null} for none
*/
public ApplicationReport setInstallerPackageName(java.lang.String installerPackageName) {
this.installerPackageName = installerPackageName;
return this;
}
/**
* List of keyed app states reported by the app.
* @return value or {@code null} for none
*/
public java.util.List getKeyedAppStates() {
return keyedAppStates;
}
/**
* List of keyed app states reported by the app.
* @param keyedAppStates keyedAppStates or {@code null} for none
*/
public ApplicationReport setKeyedAppStates(java.util.List keyedAppStates) {
this.keyedAppStates = keyedAppStates;
return this;
}
/**
* Package name of the app.
* @return value or {@code null} for none
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Package name of the app.
* @param packageName packageName or {@code null} for none
*/
public ApplicationReport setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/**
* The SHA-256 hash of the app's APK file, which can be used to verify the app hasn't been
* modified. Each byte of the hash value is represented as a two-digit hexadecimal number.
* @return value or {@code null} for none
*/
public java.lang.String getPackageSha256Hash() {
return packageSha256Hash;
}
/**
* The SHA-256 hash of the app's APK file, which can be used to verify the app hasn't been
* modified. Each byte of the hash value is represented as a two-digit hexadecimal number.
* @param packageSha256Hash packageSha256Hash or {@code null} for none
*/
public ApplicationReport setPackageSha256Hash(java.lang.String packageSha256Hash) {
this.packageSha256Hash = packageSha256Hash;
return this;
}
/**
* The SHA-1 hash of each android.content.pm.Signature
* (https://developer.android.com/reference/android/content/pm/Signature.html) associated with the
* app package. Each byte of each hash value is represented as a two-digit hexadecimal number.
* @return value or {@code null} for none
*/
public java.util.List getSigningKeyCertFingerprints() {
return signingKeyCertFingerprints;
}
/**
* The SHA-1 hash of each android.content.pm.Signature
* (https://developer.android.com/reference/android/content/pm/Signature.html) associated with the
* app package. Each byte of each hash value is represented as a two-digit hexadecimal number.
* @param signingKeyCertFingerprints signingKeyCertFingerprints or {@code null} for none
*/
public ApplicationReport setSigningKeyCertFingerprints(java.util.List signingKeyCertFingerprints) {
this.signingKeyCertFingerprints = signingKeyCertFingerprints;
return this;
}
/**
* Application state.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Application state.
* @param state state or {@code null} for none
*/
public ApplicationReport setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* Whether the app is user facing.
* @return value or {@code null} for none
*/
public java.lang.String getUserFacingType() {
return userFacingType;
}
/**
* Whether the app is user facing.
* @param userFacingType userFacingType or {@code null} for none
*/
public ApplicationReport setUserFacingType(java.lang.String userFacingType) {
this.userFacingType = userFacingType;
return this;
}
/**
* The app version code, which can be used to determine whether one version is more recent than
* another.
* @return value or {@code null} for none
*/
public java.lang.Integer getVersionCode() {
return versionCode;
}
/**
* The app version code, which can be used to determine whether one version is more recent than
* another.
* @param versionCode versionCode or {@code null} for none
*/
public ApplicationReport setVersionCode(java.lang.Integer versionCode) {
this.versionCode = versionCode;
return this;
}
/**
* The app version as displayed to the user.
* @return value or {@code null} for none
*/
public java.lang.String getVersionName() {
return versionName;
}
/**
* The app version as displayed to the user.
* @param versionName versionName or {@code null} for none
*/
public ApplicationReport setVersionName(java.lang.String versionName) {
this.versionName = versionName;
return this;
}
@Override
public ApplicationReport set(String fieldName, Object value) {
return (ApplicationReport) super.set(fieldName, value);
}
@Override
public ApplicationReport clone() {
return (ApplicationReport) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy