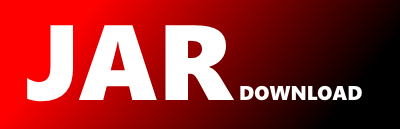
com.google.api.services.androidmanagement.v1.model.MigrationToken Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* A token to initiate the migration of a device from being managed by a third-party DPC to being
* managed by Android Management API. A migration token is valid only for a single device. See the
* guide (https://developers.google.com/android/management/dpc-migration) for more details.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class MigrationToken extends com.google.api.client.json.GenericJson {
/**
* Immutable. Optional EMM-specified additional data. Once the device is migrated this will be
* populated in the migrationAdditionalData field of the Device resource. This must be at most
* 1024 characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String additionalData;
/**
* Output only. Time when this migration token was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Output only. Once this migration token is used to migrate a device, the name of the resulting
* Device resource will be populated here, in the form enterprises/{enterprise}/devices/{device}.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String device;
/**
* Required. Immutable. The id of the device, as in the Play EMM API. This corresponds to the
* deviceId parameter in Play EMM API's Devices.get
* (https://developers.google.com/android/work/play/emm-api/v1/devices/get#parameters) call.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String deviceId;
/**
* Immutable. The time when this migration token expires. This can be at most seven days from the
* time of creation. The migration token is deleted seven days after it expires.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String expireTime;
/**
* Required. Immutable. The management mode of the device or profile being migrated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String managementMode;
/**
* Output only. The name of the migration token, which is generated by the server during creation,
* in the form enterprises/{enterprise}/migrationTokens/{migration_token}.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Required. Immutable. The name of the policy initially applied to the enrolled device, in the
* form enterprises/{enterprise}/policies/{policy}.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String policy;
/**
* Input only. The time that this migration token is valid for. This is input-only, and for
* returning a migration token the server will populate the expireTime field. This can be at most
* seven days. The default is seven days.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String ttl;
/**
* Required. Immutable. The user id of the Managed Google Play account on the device, as in the
* Play EMM API. This corresponds to the userId parameter in Play EMM API's Devices.get
* (https://developers.google.com/android/work/play/emm-api/v1/devices/get#parameters) call.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/**
* Output only. The value of the migration token.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String value;
/**
* Immutable. Optional EMM-specified additional data. Once the device is migrated this will be
* populated in the migrationAdditionalData field of the Device resource. This must be at most
* 1024 characters.
* @return value or {@code null} for none
*/
public java.lang.String getAdditionalData() {
return additionalData;
}
/**
* Immutable. Optional EMM-specified additional data. Once the device is migrated this will be
* populated in the migrationAdditionalData field of the Device resource. This must be at most
* 1024 characters.
* @param additionalData additionalData or {@code null} for none
*/
public MigrationToken setAdditionalData(java.lang.String additionalData) {
this.additionalData = additionalData;
return this;
}
/**
* Output only. Time when this migration token was created.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. Time when this migration token was created.
* @param createTime createTime or {@code null} for none
*/
public MigrationToken setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Output only. Once this migration token is used to migrate a device, the name of the resulting
* Device resource will be populated here, in the form enterprises/{enterprise}/devices/{device}.
* @return value or {@code null} for none
*/
public java.lang.String getDevice() {
return device;
}
/**
* Output only. Once this migration token is used to migrate a device, the name of the resulting
* Device resource will be populated here, in the form enterprises/{enterprise}/devices/{device}.
* @param device device or {@code null} for none
*/
public MigrationToken setDevice(java.lang.String device) {
this.device = device;
return this;
}
/**
* Required. Immutable. The id of the device, as in the Play EMM API. This corresponds to the
* deviceId parameter in Play EMM API's Devices.get
* (https://developers.google.com/android/work/play/emm-api/v1/devices/get#parameters) call.
* @return value or {@code null} for none
*/
public java.lang.String getDeviceId() {
return deviceId;
}
/**
* Required. Immutable. The id of the device, as in the Play EMM API. This corresponds to the
* deviceId parameter in Play EMM API's Devices.get
* (https://developers.google.com/android/work/play/emm-api/v1/devices/get#parameters) call.
* @param deviceId deviceId or {@code null} for none
*/
public MigrationToken setDeviceId(java.lang.String deviceId) {
this.deviceId = deviceId;
return this;
}
/**
* Immutable. The time when this migration token expires. This can be at most seven days from the
* time of creation. The migration token is deleted seven days after it expires.
* @return value or {@code null} for none
*/
public String getExpireTime() {
return expireTime;
}
/**
* Immutable. The time when this migration token expires. This can be at most seven days from the
* time of creation. The migration token is deleted seven days after it expires.
* @param expireTime expireTime or {@code null} for none
*/
public MigrationToken setExpireTime(String expireTime) {
this.expireTime = expireTime;
return this;
}
/**
* Required. Immutable. The management mode of the device or profile being migrated.
* @return value or {@code null} for none
*/
public java.lang.String getManagementMode() {
return managementMode;
}
/**
* Required. Immutable. The management mode of the device or profile being migrated.
* @param managementMode managementMode or {@code null} for none
*/
public MigrationToken setManagementMode(java.lang.String managementMode) {
this.managementMode = managementMode;
return this;
}
/**
* Output only. The name of the migration token, which is generated by the server during creation,
* in the form enterprises/{enterprise}/migrationTokens/{migration_token}.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. The name of the migration token, which is generated by the server during creation,
* in the form enterprises/{enterprise}/migrationTokens/{migration_token}.
* @param name name or {@code null} for none
*/
public MigrationToken setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Required. Immutable. The name of the policy initially applied to the enrolled device, in the
* form enterprises/{enterprise}/policies/{policy}.
* @return value or {@code null} for none
*/
public java.lang.String getPolicy() {
return policy;
}
/**
* Required. Immutable. The name of the policy initially applied to the enrolled device, in the
* form enterprises/{enterprise}/policies/{policy}.
* @param policy policy or {@code null} for none
*/
public MigrationToken setPolicy(java.lang.String policy) {
this.policy = policy;
return this;
}
/**
* Input only. The time that this migration token is valid for. This is input-only, and for
* returning a migration token the server will populate the expireTime field. This can be at most
* seven days. The default is seven days.
* @return value or {@code null} for none
*/
public String getTtl() {
return ttl;
}
/**
* Input only. The time that this migration token is valid for. This is input-only, and for
* returning a migration token the server will populate the expireTime field. This can be at most
* seven days. The default is seven days.
* @param ttl ttl or {@code null} for none
*/
public MigrationToken setTtl(String ttl) {
this.ttl = ttl;
return this;
}
/**
* Required. Immutable. The user id of the Managed Google Play account on the device, as in the
* Play EMM API. This corresponds to the userId parameter in Play EMM API's Devices.get
* (https://developers.google.com/android/work/play/emm-api/v1/devices/get#parameters) call.
* @return value or {@code null} for none
*/
public java.lang.String getUserId() {
return userId;
}
/**
* Required. Immutable. The user id of the Managed Google Play account on the device, as in the
* Play EMM API. This corresponds to the userId parameter in Play EMM API's Devices.get
* (https://developers.google.com/android/work/play/emm-api/v1/devices/get#parameters) call.
* @param userId userId or {@code null} for none
*/
public MigrationToken setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/**
* Output only. The value of the migration token.
* @return value or {@code null} for none
*/
public java.lang.String getValue() {
return value;
}
/**
* Output only. The value of the migration token.
* @param value value or {@code null} for none
*/
public MigrationToken setValue(java.lang.String value) {
this.value = value;
return this;
}
@Override
public MigrationToken set(String fieldName, Object value) {
return (MigrationToken) super.set(fieldName, value);
}
@Override
public MigrationToken clone() {
return (MigrationToken) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy