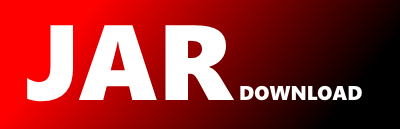
com.google.api.services.androidmanagement.v1.model.UsageLogEvent Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidmanagement.v1.model;
/**
* An event logged on the device.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Android Management API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class UsageLogEvent extends com.google.api.client.json.GenericJson {
/**
* A shell command was issued over ADB via “adb shell command”. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AdbShellCommandEvent adbShellCommandEvent;
/**
* An ADB interactive shell was opened via “adb shell”. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AdbShellInteractiveEvent adbShellInteractiveEvent;
/**
* An app process was started. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AppProcessStartEvent appProcessStartEvent;
/**
* A new root certificate was installed into the system's trusted credential storage. Part of
* SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CertAuthorityInstalledEvent certAuthorityInstalledEvent;
/**
* A root certificate was removed from the system's trusted credential storage. Part of
* SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CertAuthorityRemovedEvent certAuthorityRemovedEvent;
/**
* An X.509v3 certificate failed to validate, currently this validation is performed on the Wi-FI
* access point and failure may be due to a mismatch upon server certificate validation. However
* it may in the future include other validation events of an X.509v3 certificate. Part of
* SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CertValidationFailureEvent certValidationFailureEvent;
/**
* A TCP connect event was initiated through the standard network stack. Part of
* NETWORK_ACTIVITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ConnectEvent connectEvent;
/**
* Validates whether Android’s built-in cryptographic library (BoringSSL) is valid. Should always
* succeed on device boot, if it fails, the device should be considered untrusted. Part of
* SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CryptoSelfTestCompletedEvent cryptoSelfTestCompletedEvent;
/**
* A DNS lookup event was initiated through the standard network stack. Part of
* NETWORK_ACTIVITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DnsEvent dnsEvent;
/**
* Device has completed enrollment. Part of AMAPI_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private EnrollmentCompleteEvent enrollmentCompleteEvent;
/**
* Unique id of the event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long eventId;
/**
* Device timestamp when the event was logged.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String eventTime;
/**
* The particular usage log event type that was reported on the device. Use this to determine
* which event field to access.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String eventType;
/**
* A file was downloaded from the device. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FilePulledEvent filePulledEvent;
/**
* A file was uploaded onto the device. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FilePushedEvent filePushedEvent;
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is removed from the device either by the user or management. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private KeyDestructionEvent keyDestructionEvent;
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is installed on the device either by the user or management. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private KeyGeneratedEvent keyGeneratedEvent;
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is imported on the device either by the user or management. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private KeyImportEvent keyImportEvent;
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is determined to be corrupted due to storage corruption, hardware failure or some OS issue.
* Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private KeyIntegrityViolationEvent keyIntegrityViolationEvent;
/**
* An attempt was made to unlock the device. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private KeyguardDismissAuthAttemptEvent keyguardDismissAuthAttemptEvent;
/**
* The keyguard was dismissed. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private KeyguardDismissedEvent keyguardDismissedEvent;
/**
* The device was locked either by user or timeout. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private KeyguardSecuredEvent keyguardSecuredEvent;
/**
* The audit log buffer has reached 90% of its capacity, therefore older events may be dropped.
* Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LogBufferSizeCriticalEvent logBufferSizeCriticalEvent;
/**
* usageLog policy has been enabled. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LoggingStartedEvent loggingStartedEvent;
/**
* usageLog policy has been disabled. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LoggingStoppedEvent loggingStoppedEvent;
/**
* A lost mode location update when a device in lost mode.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LostModeLocationEvent lostModeLocationEvent;
/**
* An outgoing phone call has been made when a device in lost mode.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LostModeOutgoingPhoneCallEvent lostModeOutgoingPhoneCallEvent;
/**
* Removable media was mounted. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MediaMountEvent mediaMountEvent;
/**
* Removable media was unmounted. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MediaUnmountEvent mediaUnmountEvent;
/**
* Device was shutdown. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private OsShutdownEvent osShutdownEvent;
/**
* Device was started. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private OsStartupEvent osStartupEvent;
/**
* The device or profile has been remotely locked via the LOCK command. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RemoteLockEvent remoteLockEvent;
/**
* An attempt to take a device out of lost mode.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private StopLostModeUserAttemptEvent stopLostModeUserAttemptEvent;
/**
* The work profile or company-owned device failed to wipe when requested. This could be user
* initiated or admin initiated e.g. delete was received. Part of SECURITY_LOGS.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WipeFailureEvent wipeFailureEvent;
/**
* A shell command was issued over ADB via “adb shell command”. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public AdbShellCommandEvent getAdbShellCommandEvent() {
return adbShellCommandEvent;
}
/**
* A shell command was issued over ADB via “adb shell command”. Part of SECURITY_LOGS.
* @param adbShellCommandEvent adbShellCommandEvent or {@code null} for none
*/
public UsageLogEvent setAdbShellCommandEvent(AdbShellCommandEvent adbShellCommandEvent) {
this.adbShellCommandEvent = adbShellCommandEvent;
return this;
}
/**
* An ADB interactive shell was opened via “adb shell”. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public AdbShellInteractiveEvent getAdbShellInteractiveEvent() {
return adbShellInteractiveEvent;
}
/**
* An ADB interactive shell was opened via “adb shell”. Part of SECURITY_LOGS.
* @param adbShellInteractiveEvent adbShellInteractiveEvent or {@code null} for none
*/
public UsageLogEvent setAdbShellInteractiveEvent(AdbShellInteractiveEvent adbShellInteractiveEvent) {
this.adbShellInteractiveEvent = adbShellInteractiveEvent;
return this;
}
/**
* An app process was started. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public AppProcessStartEvent getAppProcessStartEvent() {
return appProcessStartEvent;
}
/**
* An app process was started. Part of SECURITY_LOGS.
* @param appProcessStartEvent appProcessStartEvent or {@code null} for none
*/
public UsageLogEvent setAppProcessStartEvent(AppProcessStartEvent appProcessStartEvent) {
this.appProcessStartEvent = appProcessStartEvent;
return this;
}
/**
* A new root certificate was installed into the system's trusted credential storage. Part of
* SECURITY_LOGS.
* @return value or {@code null} for none
*/
public CertAuthorityInstalledEvent getCertAuthorityInstalledEvent() {
return certAuthorityInstalledEvent;
}
/**
* A new root certificate was installed into the system's trusted credential storage. Part of
* SECURITY_LOGS.
* @param certAuthorityInstalledEvent certAuthorityInstalledEvent or {@code null} for none
*/
public UsageLogEvent setCertAuthorityInstalledEvent(CertAuthorityInstalledEvent certAuthorityInstalledEvent) {
this.certAuthorityInstalledEvent = certAuthorityInstalledEvent;
return this;
}
/**
* A root certificate was removed from the system's trusted credential storage. Part of
* SECURITY_LOGS.
* @return value or {@code null} for none
*/
public CertAuthorityRemovedEvent getCertAuthorityRemovedEvent() {
return certAuthorityRemovedEvent;
}
/**
* A root certificate was removed from the system's trusted credential storage. Part of
* SECURITY_LOGS.
* @param certAuthorityRemovedEvent certAuthorityRemovedEvent or {@code null} for none
*/
public UsageLogEvent setCertAuthorityRemovedEvent(CertAuthorityRemovedEvent certAuthorityRemovedEvent) {
this.certAuthorityRemovedEvent = certAuthorityRemovedEvent;
return this;
}
/**
* An X.509v3 certificate failed to validate, currently this validation is performed on the Wi-FI
* access point and failure may be due to a mismatch upon server certificate validation. However
* it may in the future include other validation events of an X.509v3 certificate. Part of
* SECURITY_LOGS.
* @return value or {@code null} for none
*/
public CertValidationFailureEvent getCertValidationFailureEvent() {
return certValidationFailureEvent;
}
/**
* An X.509v3 certificate failed to validate, currently this validation is performed on the Wi-FI
* access point and failure may be due to a mismatch upon server certificate validation. However
* it may in the future include other validation events of an X.509v3 certificate. Part of
* SECURITY_LOGS.
* @param certValidationFailureEvent certValidationFailureEvent or {@code null} for none
*/
public UsageLogEvent setCertValidationFailureEvent(CertValidationFailureEvent certValidationFailureEvent) {
this.certValidationFailureEvent = certValidationFailureEvent;
return this;
}
/**
* A TCP connect event was initiated through the standard network stack. Part of
* NETWORK_ACTIVITY_LOGS.
* @return value or {@code null} for none
*/
public ConnectEvent getConnectEvent() {
return connectEvent;
}
/**
* A TCP connect event was initiated through the standard network stack. Part of
* NETWORK_ACTIVITY_LOGS.
* @param connectEvent connectEvent or {@code null} for none
*/
public UsageLogEvent setConnectEvent(ConnectEvent connectEvent) {
this.connectEvent = connectEvent;
return this;
}
/**
* Validates whether Android’s built-in cryptographic library (BoringSSL) is valid. Should always
* succeed on device boot, if it fails, the device should be considered untrusted. Part of
* SECURITY_LOGS.
* @return value or {@code null} for none
*/
public CryptoSelfTestCompletedEvent getCryptoSelfTestCompletedEvent() {
return cryptoSelfTestCompletedEvent;
}
/**
* Validates whether Android’s built-in cryptographic library (BoringSSL) is valid. Should always
* succeed on device boot, if it fails, the device should be considered untrusted. Part of
* SECURITY_LOGS.
* @param cryptoSelfTestCompletedEvent cryptoSelfTestCompletedEvent or {@code null} for none
*/
public UsageLogEvent setCryptoSelfTestCompletedEvent(CryptoSelfTestCompletedEvent cryptoSelfTestCompletedEvent) {
this.cryptoSelfTestCompletedEvent = cryptoSelfTestCompletedEvent;
return this;
}
/**
* A DNS lookup event was initiated through the standard network stack. Part of
* NETWORK_ACTIVITY_LOGS.
* @return value or {@code null} for none
*/
public DnsEvent getDnsEvent() {
return dnsEvent;
}
/**
* A DNS lookup event was initiated through the standard network stack. Part of
* NETWORK_ACTIVITY_LOGS.
* @param dnsEvent dnsEvent or {@code null} for none
*/
public UsageLogEvent setDnsEvent(DnsEvent dnsEvent) {
this.dnsEvent = dnsEvent;
return this;
}
/**
* Device has completed enrollment. Part of AMAPI_LOGS.
* @return value or {@code null} for none
*/
public EnrollmentCompleteEvent getEnrollmentCompleteEvent() {
return enrollmentCompleteEvent;
}
/**
* Device has completed enrollment. Part of AMAPI_LOGS.
* @param enrollmentCompleteEvent enrollmentCompleteEvent or {@code null} for none
*/
public UsageLogEvent setEnrollmentCompleteEvent(EnrollmentCompleteEvent enrollmentCompleteEvent) {
this.enrollmentCompleteEvent = enrollmentCompleteEvent;
return this;
}
/**
* Unique id of the event.
* @return value or {@code null} for none
*/
public java.lang.Long getEventId() {
return eventId;
}
/**
* Unique id of the event.
* @param eventId eventId or {@code null} for none
*/
public UsageLogEvent setEventId(java.lang.Long eventId) {
this.eventId = eventId;
return this;
}
/**
* Device timestamp when the event was logged.
* @return value or {@code null} for none
*/
public String getEventTime() {
return eventTime;
}
/**
* Device timestamp when the event was logged.
* @param eventTime eventTime or {@code null} for none
*/
public UsageLogEvent setEventTime(String eventTime) {
this.eventTime = eventTime;
return this;
}
/**
* The particular usage log event type that was reported on the device. Use this to determine
* which event field to access.
* @return value or {@code null} for none
*/
public java.lang.String getEventType() {
return eventType;
}
/**
* The particular usage log event type that was reported on the device. Use this to determine
* which event field to access.
* @param eventType eventType or {@code null} for none
*/
public UsageLogEvent setEventType(java.lang.String eventType) {
this.eventType = eventType;
return this;
}
/**
* A file was downloaded from the device. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public FilePulledEvent getFilePulledEvent() {
return filePulledEvent;
}
/**
* A file was downloaded from the device. Part of SECURITY_LOGS.
* @param filePulledEvent filePulledEvent or {@code null} for none
*/
public UsageLogEvent setFilePulledEvent(FilePulledEvent filePulledEvent) {
this.filePulledEvent = filePulledEvent;
return this;
}
/**
* A file was uploaded onto the device. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public FilePushedEvent getFilePushedEvent() {
return filePushedEvent;
}
/**
* A file was uploaded onto the device. Part of SECURITY_LOGS.
* @param filePushedEvent filePushedEvent or {@code null} for none
*/
public UsageLogEvent setFilePushedEvent(FilePushedEvent filePushedEvent) {
this.filePushedEvent = filePushedEvent;
return this;
}
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is removed from the device either by the user or management. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public KeyDestructionEvent getKeyDestructionEvent() {
return keyDestructionEvent;
}
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is removed from the device either by the user or management. Part of SECURITY_LOGS.
* @param keyDestructionEvent keyDestructionEvent or {@code null} for none
*/
public UsageLogEvent setKeyDestructionEvent(KeyDestructionEvent keyDestructionEvent) {
this.keyDestructionEvent = keyDestructionEvent;
return this;
}
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is installed on the device either by the user or management. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public KeyGeneratedEvent getKeyGeneratedEvent() {
return keyGeneratedEvent;
}
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is installed on the device either by the user or management. Part of SECURITY_LOGS.
* @param keyGeneratedEvent keyGeneratedEvent or {@code null} for none
*/
public UsageLogEvent setKeyGeneratedEvent(KeyGeneratedEvent keyGeneratedEvent) {
this.keyGeneratedEvent = keyGeneratedEvent;
return this;
}
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is imported on the device either by the user or management. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public KeyImportEvent getKeyImportEvent() {
return keyImportEvent;
}
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is imported on the device either by the user or management. Part of SECURITY_LOGS.
* @param keyImportEvent keyImportEvent or {@code null} for none
*/
public UsageLogEvent setKeyImportEvent(KeyImportEvent keyImportEvent) {
this.keyImportEvent = keyImportEvent;
return this;
}
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is determined to be corrupted due to storage corruption, hardware failure or some OS issue.
* Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public KeyIntegrityViolationEvent getKeyIntegrityViolationEvent() {
return keyIntegrityViolationEvent;
}
/**
* A cryptographic key including user installed, admin installed and system maintained private key
* is determined to be corrupted due to storage corruption, hardware failure or some OS issue.
* Part of SECURITY_LOGS.
* @param keyIntegrityViolationEvent keyIntegrityViolationEvent or {@code null} for none
*/
public UsageLogEvent setKeyIntegrityViolationEvent(KeyIntegrityViolationEvent keyIntegrityViolationEvent) {
this.keyIntegrityViolationEvent = keyIntegrityViolationEvent;
return this;
}
/**
* An attempt was made to unlock the device. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public KeyguardDismissAuthAttemptEvent getKeyguardDismissAuthAttemptEvent() {
return keyguardDismissAuthAttemptEvent;
}
/**
* An attempt was made to unlock the device. Part of SECURITY_LOGS.
* @param keyguardDismissAuthAttemptEvent keyguardDismissAuthAttemptEvent or {@code null} for none
*/
public UsageLogEvent setKeyguardDismissAuthAttemptEvent(KeyguardDismissAuthAttemptEvent keyguardDismissAuthAttemptEvent) {
this.keyguardDismissAuthAttemptEvent = keyguardDismissAuthAttemptEvent;
return this;
}
/**
* The keyguard was dismissed. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public KeyguardDismissedEvent getKeyguardDismissedEvent() {
return keyguardDismissedEvent;
}
/**
* The keyguard was dismissed. Part of SECURITY_LOGS.
* @param keyguardDismissedEvent keyguardDismissedEvent or {@code null} for none
*/
public UsageLogEvent setKeyguardDismissedEvent(KeyguardDismissedEvent keyguardDismissedEvent) {
this.keyguardDismissedEvent = keyguardDismissedEvent;
return this;
}
/**
* The device was locked either by user or timeout. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public KeyguardSecuredEvent getKeyguardSecuredEvent() {
return keyguardSecuredEvent;
}
/**
* The device was locked either by user or timeout. Part of SECURITY_LOGS.
* @param keyguardSecuredEvent keyguardSecuredEvent or {@code null} for none
*/
public UsageLogEvent setKeyguardSecuredEvent(KeyguardSecuredEvent keyguardSecuredEvent) {
this.keyguardSecuredEvent = keyguardSecuredEvent;
return this;
}
/**
* The audit log buffer has reached 90% of its capacity, therefore older events may be dropped.
* Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public LogBufferSizeCriticalEvent getLogBufferSizeCriticalEvent() {
return logBufferSizeCriticalEvent;
}
/**
* The audit log buffer has reached 90% of its capacity, therefore older events may be dropped.
* Part of SECURITY_LOGS.
* @param logBufferSizeCriticalEvent logBufferSizeCriticalEvent or {@code null} for none
*/
public UsageLogEvent setLogBufferSizeCriticalEvent(LogBufferSizeCriticalEvent logBufferSizeCriticalEvent) {
this.logBufferSizeCriticalEvent = logBufferSizeCriticalEvent;
return this;
}
/**
* usageLog policy has been enabled. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public LoggingStartedEvent getLoggingStartedEvent() {
return loggingStartedEvent;
}
/**
* usageLog policy has been enabled. Part of SECURITY_LOGS.
* @param loggingStartedEvent loggingStartedEvent or {@code null} for none
*/
public UsageLogEvent setLoggingStartedEvent(LoggingStartedEvent loggingStartedEvent) {
this.loggingStartedEvent = loggingStartedEvent;
return this;
}
/**
* usageLog policy has been disabled. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public LoggingStoppedEvent getLoggingStoppedEvent() {
return loggingStoppedEvent;
}
/**
* usageLog policy has been disabled. Part of SECURITY_LOGS.
* @param loggingStoppedEvent loggingStoppedEvent or {@code null} for none
*/
public UsageLogEvent setLoggingStoppedEvent(LoggingStoppedEvent loggingStoppedEvent) {
this.loggingStoppedEvent = loggingStoppedEvent;
return this;
}
/**
* A lost mode location update when a device in lost mode.
* @return value or {@code null} for none
*/
public LostModeLocationEvent getLostModeLocationEvent() {
return lostModeLocationEvent;
}
/**
* A lost mode location update when a device in lost mode.
* @param lostModeLocationEvent lostModeLocationEvent or {@code null} for none
*/
public UsageLogEvent setLostModeLocationEvent(LostModeLocationEvent lostModeLocationEvent) {
this.lostModeLocationEvent = lostModeLocationEvent;
return this;
}
/**
* An outgoing phone call has been made when a device in lost mode.
* @return value or {@code null} for none
*/
public LostModeOutgoingPhoneCallEvent getLostModeOutgoingPhoneCallEvent() {
return lostModeOutgoingPhoneCallEvent;
}
/**
* An outgoing phone call has been made when a device in lost mode.
* @param lostModeOutgoingPhoneCallEvent lostModeOutgoingPhoneCallEvent or {@code null} for none
*/
public UsageLogEvent setLostModeOutgoingPhoneCallEvent(LostModeOutgoingPhoneCallEvent lostModeOutgoingPhoneCallEvent) {
this.lostModeOutgoingPhoneCallEvent = lostModeOutgoingPhoneCallEvent;
return this;
}
/**
* Removable media was mounted. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public MediaMountEvent getMediaMountEvent() {
return mediaMountEvent;
}
/**
* Removable media was mounted. Part of SECURITY_LOGS.
* @param mediaMountEvent mediaMountEvent or {@code null} for none
*/
public UsageLogEvent setMediaMountEvent(MediaMountEvent mediaMountEvent) {
this.mediaMountEvent = mediaMountEvent;
return this;
}
/**
* Removable media was unmounted. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public MediaUnmountEvent getMediaUnmountEvent() {
return mediaUnmountEvent;
}
/**
* Removable media was unmounted. Part of SECURITY_LOGS.
* @param mediaUnmountEvent mediaUnmountEvent or {@code null} for none
*/
public UsageLogEvent setMediaUnmountEvent(MediaUnmountEvent mediaUnmountEvent) {
this.mediaUnmountEvent = mediaUnmountEvent;
return this;
}
/**
* Device was shutdown. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public OsShutdownEvent getOsShutdownEvent() {
return osShutdownEvent;
}
/**
* Device was shutdown. Part of SECURITY_LOGS.
* @param osShutdownEvent osShutdownEvent or {@code null} for none
*/
public UsageLogEvent setOsShutdownEvent(OsShutdownEvent osShutdownEvent) {
this.osShutdownEvent = osShutdownEvent;
return this;
}
/**
* Device was started. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public OsStartupEvent getOsStartupEvent() {
return osStartupEvent;
}
/**
* Device was started. Part of SECURITY_LOGS.
* @param osStartupEvent osStartupEvent or {@code null} for none
*/
public UsageLogEvent setOsStartupEvent(OsStartupEvent osStartupEvent) {
this.osStartupEvent = osStartupEvent;
return this;
}
/**
* The device or profile has been remotely locked via the LOCK command. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public RemoteLockEvent getRemoteLockEvent() {
return remoteLockEvent;
}
/**
* The device or profile has been remotely locked via the LOCK command. Part of SECURITY_LOGS.
* @param remoteLockEvent remoteLockEvent or {@code null} for none
*/
public UsageLogEvent setRemoteLockEvent(RemoteLockEvent remoteLockEvent) {
this.remoteLockEvent = remoteLockEvent;
return this;
}
/**
* An attempt to take a device out of lost mode.
* @return value or {@code null} for none
*/
public StopLostModeUserAttemptEvent getStopLostModeUserAttemptEvent() {
return stopLostModeUserAttemptEvent;
}
/**
* An attempt to take a device out of lost mode.
* @param stopLostModeUserAttemptEvent stopLostModeUserAttemptEvent or {@code null} for none
*/
public UsageLogEvent setStopLostModeUserAttemptEvent(StopLostModeUserAttemptEvent stopLostModeUserAttemptEvent) {
this.stopLostModeUserAttemptEvent = stopLostModeUserAttemptEvent;
return this;
}
/**
* The work profile or company-owned device failed to wipe when requested. This could be user
* initiated or admin initiated e.g. delete was received. Part of SECURITY_LOGS.
* @return value or {@code null} for none
*/
public WipeFailureEvent getWipeFailureEvent() {
return wipeFailureEvent;
}
/**
* The work profile or company-owned device failed to wipe when requested. This could be user
* initiated or admin initiated e.g. delete was received. Part of SECURITY_LOGS.
* @param wipeFailureEvent wipeFailureEvent or {@code null} for none
*/
public UsageLogEvent setWipeFailureEvent(WipeFailureEvent wipeFailureEvent) {
this.wipeFailureEvent = wipeFailureEvent;
return this;
}
@Override
public UsageLogEvent set(String fieldName, Object value) {
return (UsageLogEvent) super.set(fieldName, value);
}
@Override
public UsageLogEvent clone() {
return (UsageLogEvent) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy