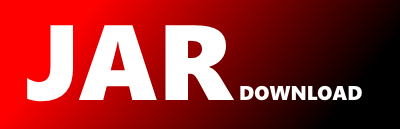
com.google.api.services.androidpublisher.AndroidPublisher Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-02-26 17:53:51 UTC)
* on 2018-03-07 at 01:11:10 UTC
* Modify at your own risk.
*/
package com.google.api.services.androidpublisher;
/**
* Service definition for AndroidPublisher (v2).
*
*
* Lets Android application developers access their Google Play accounts.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AndroidPublisherRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class AndroidPublisher extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.23.0 of the Google Play Developer API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "androidpublisher/v2/applications/";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch/androidpublisher/v2";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public AndroidPublisher(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
AndroidPublisher(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Edits collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Edits.List request = androidpublisher.edits().list(parameters ...)}
*
*
* @return the resource collection
*/
public Edits edits() {
return new Edits();
}
/**
* The "edits" collection of methods.
*/
public class Edits {
/**
* Commits/applies the changes made in this edit back to the app.
*
* Create a request for the method "edits.commit".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Commit#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public Commit commit(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
Commit result = new Commit(packageName, editId);
initialize(result);
return result;
}
public class Commit extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}:commit";
/**
* Commits/applies the changes made in this edit back to the app.
*
* Create a request for the method "edits.commit".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Commit#execute()} method to invoke the remote operation.
* {@link
* Commit#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected Commit(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "POST", REST_PATH, null, com.google.api.services.androidpublisher.model.AppEdit.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public Commit setAlt(java.lang.String alt) {
return (Commit) super.setAlt(alt);
}
@Override
public Commit setFields(java.lang.String fields) {
return (Commit) super.setFields(fields);
}
@Override
public Commit setKey(java.lang.String key) {
return (Commit) super.setKey(key);
}
@Override
public Commit setOauthToken(java.lang.String oauthToken) {
return (Commit) super.setOauthToken(oauthToken);
}
@Override
public Commit setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Commit) super.setPrettyPrint(prettyPrint);
}
@Override
public Commit setQuotaUser(java.lang.String quotaUser) {
return (Commit) super.setQuotaUser(quotaUser);
}
@Override
public Commit setUserIp(java.lang.String userIp) {
return (Commit) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public Commit setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Commit setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Commit set(String parameterName, Object value) {
return (Commit) super.set(parameterName, value);
}
}
/**
* Deletes an edit for an app. Creating a new edit will automatically delete any of your previous
* edits so this method need only be called if you want to preemptively abandon an edit.
*
* Create a request for the method "edits.delete".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public Delete delete(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
Delete result = new Delete(packageName, editId);
initialize(result);
return result;
}
public class Delete extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}";
/**
* Deletes an edit for an app. Creating a new edit will automatically delete any of your previous
* edits so this method need only be called if you want to preemptively abandon an edit.
*
* Create a request for the method "edits.delete".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected Delete(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "DELETE", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public Delete setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Delete setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns information about the edit specified. Calls will fail if the edit is no long active (e.g.
* has been deleted, superseded or expired).
*
* Create a request for the method "edits.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
Get result = new Get(packageName, editId);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}";
/**
* Returns information about the edit specified. Calls will fail if the edit is no long active
* (e.g. has been deleted, superseded or expired).
*
* Create a request for the method "edits.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.AppEdit.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Get setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a new edit for an app, populated with the app's current state.
*
* Create a request for the method "edits.insert".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param content the {@link com.google.api.services.androidpublisher.model.AppEdit}
* @return the request
*/
public Insert insert(java.lang.String packageName, com.google.api.services.androidpublisher.model.AppEdit content) throws java.io.IOException {
Insert result = new Insert(packageName, content);
initialize(result);
return result;
}
public class Insert extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits";
/**
* Creates a new edit for an app, populated with the app's current state.
*
* Create a request for the method "edits.insert".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param content the {@link com.google.api.services.androidpublisher.model.AppEdit}
* @since 1.13
*/
protected Insert(java.lang.String packageName, com.google.api.services.androidpublisher.model.AppEdit content) {
super(AndroidPublisher.this, "POST", REST_PATH, content, com.google.api.services.androidpublisher.model.AppEdit.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public Insert setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Checks that the edit can be successfully committed. The edit's changes are not applied to the
* live app.
*
* Create a request for the method "edits.validate".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Validate#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public Validate validate(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
Validate result = new Validate(packageName, editId);
initialize(result);
return result;
}
public class Validate extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}:validate";
/**
* Checks that the edit can be successfully committed. The edit's changes are not applied to the
* live app.
*
* Create a request for the method "edits.validate".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Validate#execute()} method to invoke the remote operation.
* {@link
* Validate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected Validate(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "POST", REST_PATH, null, com.google.api.services.androidpublisher.model.AppEdit.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public Validate setAlt(java.lang.String alt) {
return (Validate) super.setAlt(alt);
}
@Override
public Validate setFields(java.lang.String fields) {
return (Validate) super.setFields(fields);
}
@Override
public Validate setKey(java.lang.String key) {
return (Validate) super.setKey(key);
}
@Override
public Validate setOauthToken(java.lang.String oauthToken) {
return (Validate) super.setOauthToken(oauthToken);
}
@Override
public Validate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Validate) super.setPrettyPrint(prettyPrint);
}
@Override
public Validate setQuotaUser(java.lang.String quotaUser) {
return (Validate) super.setQuotaUser(quotaUser);
}
@Override
public Validate setUserIp(java.lang.String userIp) {
return (Validate) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public Validate setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Validate setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Validate set(String parameterName, Object value) {
return (Validate) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Apklistings collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Apklistings.List request = androidpublisher.apklistings().list(parameters ...)}
*
*
* @return the resource collection
*/
public Apklistings apklistings() {
return new Apklistings();
}
/**
* The "apklistings" collection of methods.
*/
public class Apklistings {
/**
* Deletes the APK-specific localized listing for a specified APK and language code.
*
* Create a request for the method "apklistings.delete".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @param language The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
* For example, to select Austrian German, pass "de-AT".
* @return the request
*/
public Delete delete(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String language) throws java.io.IOException {
Delete result = new Delete(packageName, editId, apkVersionCode, language);
initialize(result);
return result;
}
public class Delete extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/listings/{language}";
/**
* Deletes the APK-specific localized listing for a specified APK and language code.
*
* Create a request for the method "apklistings.delete".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @param language The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
* For example, to select Austrian German, pass "de-AT".
* @since 1.13
*/
protected Delete(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String language) {
super(AndroidPublisher.this, "DELETE", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Delete setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Delete setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/** The APK version code whose APK-specific listings should be read or modified. */
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The APK version code whose APK-specific listings should be read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/** The APK version code whose APK-specific listings should be read or modified. */
public Delete setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
/**
* The language code (a BCP-47 language tag) of the APK-specific localized listing to read
* or modify. For example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
For example, to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the APK-specific localized listing to read
* or modify. For example, to select Austrian German, pass "de-AT".
*/
public Delete setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Deletes all the APK-specific localized listings for a specified APK.
*
* Create a request for the method "apklistings.deleteall".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Deleteall#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @return the request
*/
public Deleteall deleteall(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode) throws java.io.IOException {
Deleteall result = new Deleteall(packageName, editId, apkVersionCode);
initialize(result);
return result;
}
public class Deleteall extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/listings";
/**
* Deletes all the APK-specific localized listings for a specified APK.
*
* Create a request for the method "apklistings.deleteall".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Deleteall#execute()} method to invoke the remote
* operation. {@link
* Deleteall#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @since 1.13
*/
protected Deleteall(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode) {
super(AndroidPublisher.this, "DELETE", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
}
@Override
public Deleteall setAlt(java.lang.String alt) {
return (Deleteall) super.setAlt(alt);
}
@Override
public Deleteall setFields(java.lang.String fields) {
return (Deleteall) super.setFields(fields);
}
@Override
public Deleteall setKey(java.lang.String key) {
return (Deleteall) super.setKey(key);
}
@Override
public Deleteall setOauthToken(java.lang.String oauthToken) {
return (Deleteall) super.setOauthToken(oauthToken);
}
@Override
public Deleteall setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Deleteall) super.setPrettyPrint(prettyPrint);
}
@Override
public Deleteall setQuotaUser(java.lang.String quotaUser) {
return (Deleteall) super.setQuotaUser(quotaUser);
}
@Override
public Deleteall setUserIp(java.lang.String userIp) {
return (Deleteall) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Deleteall setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Deleteall setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/** The APK version code whose APK-specific listings should be read or modified. */
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The APK version code whose APK-specific listings should be read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/** The APK version code whose APK-specific listings should be read or modified. */
public Deleteall setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
@Override
public Deleteall set(String parameterName, Object value) {
return (Deleteall) super.set(parameterName, value);
}
}
/**
* Fetches the APK-specific localized listing for a specified APK and language code.
*
* Create a request for the method "apklistings.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @param language The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
* For example, to select Austrian German, pass "de-AT".
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String language) throws java.io.IOException {
Get result = new Get(packageName, editId, apkVersionCode, language);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/listings/{language}";
/**
* Fetches the APK-specific localized listing for a specified APK and language code.
*
* Create a request for the method "apklistings.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @param language The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
* For example, to select Austrian German, pass "de-AT".
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String language) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.ApkListing.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Get setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/** The APK version code whose APK-specific listings should be read or modified. */
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The APK version code whose APK-specific listings should be read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/** The APK version code whose APK-specific listings should be read or modified. */
public Get setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
/**
* The language code (a BCP-47 language tag) of the APK-specific localized listing to read
* or modify. For example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
For example, to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the APK-specific localized listing to read
* or modify. For example, to select Austrian German, pass "de-AT".
*/
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the APK-specific localized listings for a specified APK.
*
* Create a request for the method "apklistings.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @return the request
*/
public List list(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode) throws java.io.IOException {
List result = new List(packageName, editId, apkVersionCode);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/listings";
/**
* Lists all the APK-specific localized listings for a specified APK.
*
* Create a request for the method "apklistings.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @since 1.13
*/
protected List(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.ApkListingsListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public List setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/** The APK version code whose APK-specific listings should be read or modified. */
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The APK version code whose APK-specific listings should be read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/** The APK version code whose APK-specific listings should be read or modified. */
public List setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates or creates the APK-specific localized listing for a specified APK and language code. This
* method supports patch semantics.
*
* Create a request for the method "apklistings.patch".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @param language The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
* For example, to select Austrian German, pass "de-AT".
* @param content the {@link com.google.api.services.androidpublisher.model.ApkListing}
* @return the request
*/
public Patch patch(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String language, com.google.api.services.androidpublisher.model.ApkListing content) throws java.io.IOException {
Patch result = new Patch(packageName, editId, apkVersionCode, language, content);
initialize(result);
return result;
}
public class Patch extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/listings/{language}";
/**
* Updates or creates the APK-specific localized listing for a specified APK and language code.
* This method supports patch semantics.
*
* Create a request for the method "apklistings.patch".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @param language The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
* For example, to select Austrian German, pass "de-AT".
* @param content the {@link com.google.api.services.androidpublisher.model.ApkListing}
* @since 1.13
*/
protected Patch(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String language, com.google.api.services.androidpublisher.model.ApkListing content) {
super(AndroidPublisher.this, "PATCH", REST_PATH, content, com.google.api.services.androidpublisher.model.ApkListing.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Patch setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Patch setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/** The APK version code whose APK-specific listings should be read or modified. */
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The APK version code whose APK-specific listings should be read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/** The APK version code whose APK-specific listings should be read or modified. */
public Patch setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
/**
* The language code (a BCP-47 language tag) of the APK-specific localized listing to read
* or modify. For example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
For example, to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the APK-specific localized listing to read
* or modify. For example, to select Austrian German, pass "de-AT".
*/
public Patch setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates or creates the APK-specific localized listing for a specified APK and language code.
*
* Create a request for the method "apklistings.update".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @param language The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
* For example, to select Austrian German, pass "de-AT".
* @param content the {@link com.google.api.services.androidpublisher.model.ApkListing}
* @return the request
*/
public Update update(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String language, com.google.api.services.androidpublisher.model.ApkListing content) throws java.io.IOException {
Update result = new Update(packageName, editId, apkVersionCode, language, content);
initialize(result);
return result;
}
public class Update extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/listings/{language}";
/**
* Updates or creates the APK-specific localized listing for a specified APK and language code.
*
* Create a request for the method "apklistings.update".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The APK version code whose APK-specific listings should be read or modified.
* @param language The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
* For example, to select Austrian German, pass "de-AT".
* @param content the {@link com.google.api.services.androidpublisher.model.ApkListing}
* @since 1.13
*/
protected Update(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String language, com.google.api.services.androidpublisher.model.ApkListing content) {
super(AndroidPublisher.this, "PUT", REST_PATH, content, com.google.api.services.androidpublisher.model.ApkListing.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Update setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Update setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/** The APK version code whose APK-specific listings should be read or modified. */
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The APK version code whose APK-specific listings should be read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/** The APK version code whose APK-specific listings should be read or modified. */
public Update setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
/**
* The language code (a BCP-47 language tag) of the APK-specific localized listing to read
* or modify. For example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the APK-specific localized listing to read or modify.
For example, to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the APK-specific localized listing to read
* or modify. For example, to select Austrian German, pass "de-AT".
*/
public Update setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Apks collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Apks.List request = androidpublisher.apks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Apks apks() {
return new Apks();
}
/**
* The "apks" collection of methods.
*/
public class Apks {
/**
* Creates a new APK without uploading the APK itself to Google Play, instead hosting the APK at a
* specified URL. This function is only available to enterprises using Google Play for Work whose
* application is configured to restrict distribution to the enterprise domain.
*
* Create a request for the method "apks.addexternallyhosted".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Addexternallyhosted#execute()} method to invoke the remote
* operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param content the {@link com.google.api.services.androidpublisher.model.ApksAddExternallyHostedRequest}
* @return the request
*/
public Addexternallyhosted addexternallyhosted(java.lang.String packageName, java.lang.String editId, com.google.api.services.androidpublisher.model.ApksAddExternallyHostedRequest content) throws java.io.IOException {
Addexternallyhosted result = new Addexternallyhosted(packageName, editId, content);
initialize(result);
return result;
}
public class Addexternallyhosted extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/externallyHosted";
/**
* Creates a new APK without uploading the APK itself to Google Play, instead hosting the APK at a
* specified URL. This function is only available to enterprises using Google Play for Work whose
* application is configured to restrict distribution to the enterprise domain.
*
* Create a request for the method "apks.addexternallyhosted".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Addexternallyhosted#execute()} method to invoke the remote
* operation. {@link Addexternallyhosted#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param content the {@link com.google.api.services.androidpublisher.model.ApksAddExternallyHostedRequest}
* @since 1.13
*/
protected Addexternallyhosted(java.lang.String packageName, java.lang.String editId, com.google.api.services.androidpublisher.model.ApksAddExternallyHostedRequest content) {
super(AndroidPublisher.this, "POST", REST_PATH, content, com.google.api.services.androidpublisher.model.ApksAddExternallyHostedResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public Addexternallyhosted setAlt(java.lang.String alt) {
return (Addexternallyhosted) super.setAlt(alt);
}
@Override
public Addexternallyhosted setFields(java.lang.String fields) {
return (Addexternallyhosted) super.setFields(fields);
}
@Override
public Addexternallyhosted setKey(java.lang.String key) {
return (Addexternallyhosted) super.setKey(key);
}
@Override
public Addexternallyhosted setOauthToken(java.lang.String oauthToken) {
return (Addexternallyhosted) super.setOauthToken(oauthToken);
}
@Override
public Addexternallyhosted setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Addexternallyhosted) super.setPrettyPrint(prettyPrint);
}
@Override
public Addexternallyhosted setQuotaUser(java.lang.String quotaUser) {
return (Addexternallyhosted) super.setQuotaUser(quotaUser);
}
@Override
public Addexternallyhosted setUserIp(java.lang.String userIp) {
return (Addexternallyhosted) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Addexternallyhosted setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Addexternallyhosted setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Addexternallyhosted set(String parameterName, Object value) {
return (Addexternallyhosted) super.set(parameterName, value);
}
}
/**
* Create a request for the method "apks.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public List list(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
List result = new List(packageName, editId);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks";
/**
* Create a request for the method "apks.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected List(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.ApksListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public List setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Create a request for the method "apks.upload".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public Upload upload(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
Upload result = new Upload(packageName, editId);
initialize(result);
return result;
}
/**
* Create a request for the method "apks.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".@param editId Unique identifier for this edit.
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Upload upload(java.lang.String packageName, java.lang.String editId, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Upload result = new Upload(packageName, editId, mediaContent);
initialize(result);
return result;
}
public class Upload extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks";
/**
* Create a request for the method "apks.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected Upload(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "POST", REST_PATH, null, com.google.api.services.androidpublisher.model.Apk.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
/**
* Create a request for the method "apks.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".@param editId Unique identifier for this edit.
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Upload(java.lang.String packageName, java.lang.String editId, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(AndroidPublisher.this, "POST", "/upload/" + getServicePath() + REST_PATH, null, com.google.api.services.androidpublisher.model.Apk.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Upload setAlt(java.lang.String alt) {
return (Upload) super.setAlt(alt);
}
@Override
public Upload setFields(java.lang.String fields) {
return (Upload) super.setFields(fields);
}
@Override
public Upload setKey(java.lang.String key) {
return (Upload) super.setKey(key);
}
@Override
public Upload setOauthToken(java.lang.String oauthToken) {
return (Upload) super.setOauthToken(oauthToken);
}
@Override
public Upload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Upload) super.setPrettyPrint(prettyPrint);
}
@Override
public Upload setQuotaUser(java.lang.String quotaUser) {
return (Upload) super.setQuotaUser(quotaUser);
}
@Override
public Upload setUserIp(java.lang.String userIp) {
return (Upload) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Upload setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Upload setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Upload set(String parameterName, Object value) {
return (Upload) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Deobfuscationfiles collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Deobfuscationfiles.List request = androidpublisher.deobfuscationfiles().list(parameters ...)}
*
*
* @return the resource collection
*/
public Deobfuscationfiles deobfuscationfiles() {
return new Deobfuscationfiles();
}
/**
* The "deobfuscationfiles" collection of methods.
*/
public class Deobfuscationfiles {
/**
* Uploads the deobfuscation file of the specified APK. If a deobfuscation file already exists, it
* will be replaced.
*
* Create a request for the method "deobfuscationfiles.upload".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier of the Android app for which the deobfuscatiuon files are being uploaded; for
* example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose deobfuscation file is being uploaded.
* @param deobfuscationFileType
* @return the request
*/
public Upload upload(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String deobfuscationFileType) throws java.io.IOException {
Upload result = new Upload(packageName, editId, apkVersionCode, deobfuscationFileType);
initialize(result);
return result;
}
/**
* Uploads the deobfuscation file of the specified APK. If a deobfuscation file already exists, it
* will be replaced.
*
* Create a request for the method "deobfuscationfiles.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param packageName Unique identifier of the Android app for which the deobfuscatiuon files are being uploaded; for
* example, "com.spiffygame".@param editId Unique identifier for this edit.@param apkVersionCode The version code of the APK whose deobfuscation file is being uploaded.@param deobfuscationFileType
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Upload upload(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String deobfuscationFileType, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Upload result = new Upload(packageName, editId, apkVersionCode, deobfuscationFileType, mediaContent);
initialize(result);
return result;
}
public class Upload extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/deobfuscationFiles/{deobfuscationFileType}";
/**
* Uploads the deobfuscation file of the specified APK. If a deobfuscation file already exists, it
* will be replaced.
*
* Create a request for the method "deobfuscationfiles.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier of the Android app for which the deobfuscatiuon files are being uploaded; for
* example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose deobfuscation file is being uploaded.
* @param deobfuscationFileType
* @since 1.13
*/
protected Upload(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String deobfuscationFileType) {
super(AndroidPublisher.this, "POST", REST_PATH, null, com.google.api.services.androidpublisher.model.DeobfuscationFilesUploadResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.deobfuscationFileType = com.google.api.client.util.Preconditions.checkNotNull(deobfuscationFileType, "Required parameter deobfuscationFileType must be specified.");
}
/**
* Uploads the deobfuscation file of the specified APK. If a deobfuscation file already exists, it
* will be replaced.
*
* Create a request for the method "deobfuscationfiles.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param packageName Unique identifier of the Android app for which the deobfuscatiuon files are being uploaded; for
* example, "com.spiffygame".@param editId Unique identifier for this edit.@param apkVersionCode The version code of the APK whose deobfuscation file is being uploaded.@param deobfuscationFileType
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Upload(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String deobfuscationFileType, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(AndroidPublisher.this, "POST", "/upload/" + getServicePath() + REST_PATH, null, com.google.api.services.androidpublisher.model.DeobfuscationFilesUploadResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.deobfuscationFileType = com.google.api.client.util.Preconditions.checkNotNull(deobfuscationFileType, "Required parameter deobfuscationFileType must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Upload setAlt(java.lang.String alt) {
return (Upload) super.setAlt(alt);
}
@Override
public Upload setFields(java.lang.String fields) {
return (Upload) super.setFields(fields);
}
@Override
public Upload setKey(java.lang.String key) {
return (Upload) super.setKey(key);
}
@Override
public Upload setOauthToken(java.lang.String oauthToken) {
return (Upload) super.setOauthToken(oauthToken);
}
@Override
public Upload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Upload) super.setPrettyPrint(prettyPrint);
}
@Override
public Upload setQuotaUser(java.lang.String quotaUser) {
return (Upload) super.setQuotaUser(quotaUser);
}
@Override
public Upload setUserIp(java.lang.String userIp) {
return (Upload) super.setUserIp(userIp);
}
/**
* Unique identifier of the Android app for which the deobfuscatiuon files are being
* uploaded; for example, "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier of the Android app for which the deobfuscatiuon files are being uploaded; for
example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier of the Android app for which the deobfuscatiuon files are being
* uploaded; for example, "com.spiffygame".
*/
public Upload setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Upload setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/** The version code of the APK whose deobfuscation file is being uploaded. */
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The version code of the APK whose deobfuscation file is being uploaded.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/** The version code of the APK whose deobfuscation file is being uploaded. */
public Upload setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
@com.google.api.client.util.Key
private java.lang.String deobfuscationFileType;
/**
*/
public java.lang.String getDeobfuscationFileType() {
return deobfuscationFileType;
}
public Upload setDeobfuscationFileType(java.lang.String deobfuscationFileType) {
this.deobfuscationFileType = deobfuscationFileType;
return this;
}
@Override
public Upload set(String parameterName, Object value) {
return (Upload) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Details collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Details.List request = androidpublisher.details().list(parameters ...)}
*
*
* @return the resource collection
*/
public Details details() {
return new Details();
}
/**
* The "details" collection of methods.
*/
public class Details {
/**
* Fetches app details for this edit. This includes the default language and developer support
* contact information.
*
* Create a request for the method "details.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
Get result = new Get(packageName, editId);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/details";
/**
* Fetches app details for this edit. This includes the default language and developer support
* contact information.
*
* Create a request for the method "details.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.AppDetails.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Get setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Updates app details for this edit. This method supports patch semantics.
*
* Create a request for the method "details.patch".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param content the {@link com.google.api.services.androidpublisher.model.AppDetails}
* @return the request
*/
public Patch patch(java.lang.String packageName, java.lang.String editId, com.google.api.services.androidpublisher.model.AppDetails content) throws java.io.IOException {
Patch result = new Patch(packageName, editId, content);
initialize(result);
return result;
}
public class Patch extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/details";
/**
* Updates app details for this edit. This method supports patch semantics.
*
* Create a request for the method "details.patch".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param content the {@link com.google.api.services.androidpublisher.model.AppDetails}
* @since 1.13
*/
protected Patch(java.lang.String packageName, java.lang.String editId, com.google.api.services.androidpublisher.model.AppDetails content) {
super(AndroidPublisher.this, "PATCH", REST_PATH, content, com.google.api.services.androidpublisher.model.AppDetails.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Patch setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Patch setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates app details for this edit.
*
* Create a request for the method "details.update".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param content the {@link com.google.api.services.androidpublisher.model.AppDetails}
* @return the request
*/
public Update update(java.lang.String packageName, java.lang.String editId, com.google.api.services.androidpublisher.model.AppDetails content) throws java.io.IOException {
Update result = new Update(packageName, editId, content);
initialize(result);
return result;
}
public class Update extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/details";
/**
* Updates app details for this edit.
*
* Create a request for the method "details.update".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param content the {@link com.google.api.services.androidpublisher.model.AppDetails}
* @since 1.13
*/
protected Update(java.lang.String packageName, java.lang.String editId, com.google.api.services.androidpublisher.model.AppDetails content) {
super(AndroidPublisher.this, "PUT", REST_PATH, content, com.google.api.services.androidpublisher.model.AppDetails.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Update setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Update setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Expansionfiles collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Expansionfiles.List request = androidpublisher.expansionfiles().list(parameters ...)}
*
*
* @return the resource collection
*/
public Expansionfiles expansionfiles() {
return new Expansionfiles();
}
/**
* The "expansionfiles" collection of methods.
*/
public class Expansionfiles {
/**
* Fetches the Expansion File configuration for the APK specified.
*
* Create a request for the method "expansionfiles.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.
* @param expansionFileType
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType) throws java.io.IOException {
Get result = new Get(packageName, editId, apkVersionCode, expansionFileType);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/expansionFiles/{expansionFileType}";
/**
* Fetches the Expansion File configuration for the APK specified.
*
* Create a request for the method "expansionfiles.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.
* @param expansionFileType
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.ExpansionFile.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.expansionFileType = com.google.api.client.util.Preconditions.checkNotNull(expansionFileType, "Required parameter expansionFileType must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Get setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The version code of the APK whose Expansion File configuration is being read or modified.
*/
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The version code of the APK whose Expansion File configuration is being read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/**
* The version code of the APK whose Expansion File configuration is being read or modified.
*/
public Get setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
@com.google.api.client.util.Key
private java.lang.String expansionFileType;
/**
*/
public java.lang.String getExpansionFileType() {
return expansionFileType;
}
public Get setExpansionFileType(java.lang.String expansionFileType) {
this.expansionFileType = expansionFileType;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Updates the APK's Expansion File configuration to reference another APK's Expansion Files. To add
* a new Expansion File use the Upload method. This method supports patch semantics.
*
* Create a request for the method "expansionfiles.patch".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.
* @param expansionFileType
* @param content the {@link com.google.api.services.androidpublisher.model.ExpansionFile}
* @return the request
*/
public Patch patch(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType, com.google.api.services.androidpublisher.model.ExpansionFile content) throws java.io.IOException {
Patch result = new Patch(packageName, editId, apkVersionCode, expansionFileType, content);
initialize(result);
return result;
}
public class Patch extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/expansionFiles/{expansionFileType}";
/**
* Updates the APK's Expansion File configuration to reference another APK's Expansion Files. To
* add a new Expansion File use the Upload method. This method supports patch semantics.
*
* Create a request for the method "expansionfiles.patch".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.
* @param expansionFileType
* @param content the {@link com.google.api.services.androidpublisher.model.ExpansionFile}
* @since 1.13
*/
protected Patch(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType, com.google.api.services.androidpublisher.model.ExpansionFile content) {
super(AndroidPublisher.this, "PATCH", REST_PATH, content, com.google.api.services.androidpublisher.model.ExpansionFile.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.expansionFileType = com.google.api.client.util.Preconditions.checkNotNull(expansionFileType, "Required parameter expansionFileType must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Patch setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Patch setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The version code of the APK whose Expansion File configuration is being read or modified.
*/
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The version code of the APK whose Expansion File configuration is being read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/**
* The version code of the APK whose Expansion File configuration is being read or modified.
*/
public Patch setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
@com.google.api.client.util.Key
private java.lang.String expansionFileType;
/**
*/
public java.lang.String getExpansionFileType() {
return expansionFileType;
}
public Patch setExpansionFileType(java.lang.String expansionFileType) {
this.expansionFileType = expansionFileType;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates the APK's Expansion File configuration to reference another APK's Expansion Files. To add
* a new Expansion File use the Upload method.
*
* Create a request for the method "expansionfiles.update".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.
* @param expansionFileType
* @param content the {@link com.google.api.services.androidpublisher.model.ExpansionFile}
* @return the request
*/
public Update update(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType, com.google.api.services.androidpublisher.model.ExpansionFile content) throws java.io.IOException {
Update result = new Update(packageName, editId, apkVersionCode, expansionFileType, content);
initialize(result);
return result;
}
public class Update extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/expansionFiles/{expansionFileType}";
/**
* Updates the APK's Expansion File configuration to reference another APK's Expansion Files. To
* add a new Expansion File use the Upload method.
*
* Create a request for the method "expansionfiles.update".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.
* @param expansionFileType
* @param content the {@link com.google.api.services.androidpublisher.model.ExpansionFile}
* @since 1.13
*/
protected Update(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType, com.google.api.services.androidpublisher.model.ExpansionFile content) {
super(AndroidPublisher.this, "PUT", REST_PATH, content, com.google.api.services.androidpublisher.model.ExpansionFile.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.expansionFileType = com.google.api.client.util.Preconditions.checkNotNull(expansionFileType, "Required parameter expansionFileType must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Update setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Update setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The version code of the APK whose Expansion File configuration is being read or modified.
*/
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The version code of the APK whose Expansion File configuration is being read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/**
* The version code of the APK whose Expansion File configuration is being read or modified.
*/
public Update setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
@com.google.api.client.util.Key
private java.lang.String expansionFileType;
/**
*/
public java.lang.String getExpansionFileType() {
return expansionFileType;
}
public Update setExpansionFileType(java.lang.String expansionFileType) {
this.expansionFileType = expansionFileType;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
/**
* Uploads and attaches a new Expansion File to the APK specified.
*
* Create a request for the method "expansionfiles.upload".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.
* @param expansionFileType
* @return the request
*/
public Upload upload(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType) throws java.io.IOException {
Upload result = new Upload(packageName, editId, apkVersionCode, expansionFileType);
initialize(result);
return result;
}
/**
* Uploads and attaches a new Expansion File to the APK specified.
*
* Create a request for the method "expansionfiles.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".@param editId Unique identifier for this edit.@param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.@param expansionFileType
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Upload upload(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Upload result = new Upload(packageName, editId, apkVersionCode, expansionFileType, mediaContent);
initialize(result);
return result;
}
public class Upload extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/apks/{apkVersionCode}/expansionFiles/{expansionFileType}";
/**
* Uploads and attaches a new Expansion File to the APK specified.
*
* Create a request for the method "expansionfiles.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.
* @param expansionFileType
* @since 1.13
*/
protected Upload(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType) {
super(AndroidPublisher.this, "POST", REST_PATH, null, com.google.api.services.androidpublisher.model.ExpansionFilesUploadResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.expansionFileType = com.google.api.client.util.Preconditions.checkNotNull(expansionFileType, "Required parameter expansionFileType must be specified.");
}
/**
* Uploads and attaches a new Expansion File to the APK specified.
*
* Create a request for the method "expansionfiles.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".@param editId Unique identifier for this edit.@param apkVersionCode The version code of the APK whose Expansion File configuration is being read or modified.@param expansionFileType
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Upload(java.lang.String packageName, java.lang.String editId, java.lang.Integer apkVersionCode, java.lang.String expansionFileType, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(AndroidPublisher.this, "POST", "/upload/" + getServicePath() + REST_PATH, null, com.google.api.services.androidpublisher.model.ExpansionFilesUploadResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.apkVersionCode = com.google.api.client.util.Preconditions.checkNotNull(apkVersionCode, "Required parameter apkVersionCode must be specified.");
this.expansionFileType = com.google.api.client.util.Preconditions.checkNotNull(expansionFileType, "Required parameter expansionFileType must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Upload setAlt(java.lang.String alt) {
return (Upload) super.setAlt(alt);
}
@Override
public Upload setFields(java.lang.String fields) {
return (Upload) super.setFields(fields);
}
@Override
public Upload setKey(java.lang.String key) {
return (Upload) super.setKey(key);
}
@Override
public Upload setOauthToken(java.lang.String oauthToken) {
return (Upload) super.setOauthToken(oauthToken);
}
@Override
public Upload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Upload) super.setPrettyPrint(prettyPrint);
}
@Override
public Upload setQuotaUser(java.lang.String quotaUser) {
return (Upload) super.setQuotaUser(quotaUser);
}
@Override
public Upload setUserIp(java.lang.String userIp) {
return (Upload) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Upload setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Upload setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The version code of the APK whose Expansion File configuration is being read or modified.
*/
@com.google.api.client.util.Key
private java.lang.Integer apkVersionCode;
/** The version code of the APK whose Expansion File configuration is being read or modified.
*/
public java.lang.Integer getApkVersionCode() {
return apkVersionCode;
}
/**
* The version code of the APK whose Expansion File configuration is being read or modified.
*/
public Upload setApkVersionCode(java.lang.Integer apkVersionCode) {
this.apkVersionCode = apkVersionCode;
return this;
}
@com.google.api.client.util.Key
private java.lang.String expansionFileType;
/**
*/
public java.lang.String getExpansionFileType() {
return expansionFileType;
}
public Upload setExpansionFileType(java.lang.String expansionFileType) {
this.expansionFileType = expansionFileType;
return this;
}
@Override
public Upload set(String parameterName, Object value) {
return (Upload) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Images collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Images.List request = androidpublisher.images().list(parameters ...)}
*
*
* @return the resource collection
*/
public Images images() {
return new Images();
}
/**
* The "images" collection of methods.
*/
public class Images {
/**
* Deletes the image (specified by id) from the edit.
*
* Create a request for the method "images.delete".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".
* @param imageType
* @param imageId Unique identifier an image within the set of images attached to this edit.
* @return the request
*/
public Delete delete(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType, java.lang.String imageId) throws java.io.IOException {
Delete result = new Delete(packageName, editId, language, imageType, imageId);
initialize(result);
return result;
}
public class Delete extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings/{language}/{imageType}/{imageId}";
/**
* Deletes the image (specified by id) from the edit.
*
* Create a request for the method "images.delete".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".
* @param imageType
* @param imageId Unique identifier an image within the set of images attached to this edit.
* @since 1.13
*/
protected Delete(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType, java.lang.String imageId) {
super(AndroidPublisher.this, "DELETE", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
this.imageType = com.google.api.client.util.Preconditions.checkNotNull(imageType, "Required parameter imageType must be specified.");
this.imageId = com.google.api.client.util.Preconditions.checkNotNull(imageId, "Required parameter imageId must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Delete setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Delete setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The language code (a BCP-47 language tag) of the localized listing whose images are to
* read or modified. For example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the localized listing whose images are to read or
modified. For example, to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the localized listing whose images are to
* read or modified. For example, to select Austrian German, pass "de-AT".
*/
public Delete setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@com.google.api.client.util.Key
private java.lang.String imageType;
/**
*/
public java.lang.String getImageType() {
return imageType;
}
public Delete setImageType(java.lang.String imageType) {
this.imageType = imageType;
return this;
}
/** Unique identifier an image within the set of images attached to this edit. */
@com.google.api.client.util.Key
private java.lang.String imageId;
/** Unique identifier an image within the set of images attached to this edit.
*/
public java.lang.String getImageId() {
return imageId;
}
/** Unique identifier an image within the set of images attached to this edit. */
public Delete setImageId(java.lang.String imageId) {
this.imageId = imageId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Deletes all images for the specified language and image type.
*
* Create a request for the method "images.deleteall".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Deleteall#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".
* @param imageType
* @return the request
*/
public Deleteall deleteall(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType) throws java.io.IOException {
Deleteall result = new Deleteall(packageName, editId, language, imageType);
initialize(result);
return result;
}
public class Deleteall extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings/{language}/{imageType}";
/**
* Deletes all images for the specified language and image type.
*
* Create a request for the method "images.deleteall".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Deleteall#execute()} method to invoke the remote
* operation. {@link
* Deleteall#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".
* @param imageType
* @since 1.13
*/
protected Deleteall(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType) {
super(AndroidPublisher.this, "DELETE", REST_PATH, null, com.google.api.services.androidpublisher.model.ImagesDeleteAllResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
this.imageType = com.google.api.client.util.Preconditions.checkNotNull(imageType, "Required parameter imageType must be specified.");
}
@Override
public Deleteall setAlt(java.lang.String alt) {
return (Deleteall) super.setAlt(alt);
}
@Override
public Deleteall setFields(java.lang.String fields) {
return (Deleteall) super.setFields(fields);
}
@Override
public Deleteall setKey(java.lang.String key) {
return (Deleteall) super.setKey(key);
}
@Override
public Deleteall setOauthToken(java.lang.String oauthToken) {
return (Deleteall) super.setOauthToken(oauthToken);
}
@Override
public Deleteall setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Deleteall) super.setPrettyPrint(prettyPrint);
}
@Override
public Deleteall setQuotaUser(java.lang.String quotaUser) {
return (Deleteall) super.setQuotaUser(quotaUser);
}
@Override
public Deleteall setUserIp(java.lang.String userIp) {
return (Deleteall) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Deleteall setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Deleteall setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The language code (a BCP-47 language tag) of the localized listing whose images are to
* read or modified. For example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the localized listing whose images are to read or
modified. For example, to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the localized listing whose images are to
* read or modified. For example, to select Austrian German, pass "de-AT".
*/
public Deleteall setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@com.google.api.client.util.Key
private java.lang.String imageType;
/**
*/
public java.lang.String getImageType() {
return imageType;
}
public Deleteall setImageType(java.lang.String imageType) {
this.imageType = imageType;
return this;
}
@Override
public Deleteall set(String parameterName, Object value) {
return (Deleteall) super.set(parameterName, value);
}
}
/**
* Lists all images for the specified language and image type.
*
* Create a request for the method "images.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".
* @param imageType
* @return the request
*/
public List list(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType) throws java.io.IOException {
List result = new List(packageName, editId, language, imageType);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings/{language}/{imageType}";
/**
* Lists all images for the specified language and image type.
*
* Create a request for the method "images.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".
* @param imageType
* @since 1.13
*/
protected List(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.ImagesListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
this.imageType = com.google.api.client.util.Preconditions.checkNotNull(imageType, "Required parameter imageType must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public List setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The language code (a BCP-47 language tag) of the localized listing whose images are to
* read or modified. For example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the localized listing whose images are to read or
modified. For example, to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the localized listing whose images are to
* read or modified. For example, to select Austrian German, pass "de-AT".
*/
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@com.google.api.client.util.Key
private java.lang.String imageType;
/**
*/
public java.lang.String getImageType() {
return imageType;
}
public List setImageType(java.lang.String imageType) {
this.imageType = imageType;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Uploads a new image and adds it to the list of images for the specified language and image type.
*
* Create a request for the method "images.upload".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".
* @param imageType
* @return the request
*/
public Upload upload(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType) throws java.io.IOException {
Upload result = new Upload(packageName, editId, language, imageType);
initialize(result);
return result;
}
/**
* Uploads a new image and adds it to the list of images for the specified language and image type.
*
* Create a request for the method "images.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".@param editId Unique identifier for this edit.@param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".@param imageType
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Upload upload(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Upload result = new Upload(packageName, editId, language, imageType, mediaContent);
initialize(result);
return result;
}
public class Upload extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings/{language}/{imageType}";
/**
* Uploads a new image and adds it to the list of images for the specified language and image
* type.
*
* Create a request for the method "images.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".
* @param imageType
* @since 1.13
*/
protected Upload(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType) {
super(AndroidPublisher.this, "POST", REST_PATH, null, com.google.api.services.androidpublisher.model.ImagesUploadResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
this.imageType = com.google.api.client.util.Preconditions.checkNotNull(imageType, "Required parameter imageType must be specified.");
}
/**
* Uploads a new image and adds it to the list of images for the specified language and image
* type.
*
* Create a request for the method "images.upload".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Upload#execute()} method to invoke the remote operation.
* {@link
* Upload#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".@param editId Unique identifier for this edit.@param language The language code (a BCP-47 language tag) of the localized listing whose images are to read or
* modified. For example, to select Austrian German, pass "de-AT".@param imageType
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Upload(java.lang.String packageName, java.lang.String editId, java.lang.String language, java.lang.String imageType, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(AndroidPublisher.this, "POST", "/upload/" + getServicePath() + REST_PATH, null, com.google.api.services.androidpublisher.model.ImagesUploadResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
this.imageType = com.google.api.client.util.Preconditions.checkNotNull(imageType, "Required parameter imageType must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Upload setAlt(java.lang.String alt) {
return (Upload) super.setAlt(alt);
}
@Override
public Upload setFields(java.lang.String fields) {
return (Upload) super.setFields(fields);
}
@Override
public Upload setKey(java.lang.String key) {
return (Upload) super.setKey(key);
}
@Override
public Upload setOauthToken(java.lang.String oauthToken) {
return (Upload) super.setOauthToken(oauthToken);
}
@Override
public Upload setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Upload) super.setPrettyPrint(prettyPrint);
}
@Override
public Upload setQuotaUser(java.lang.String quotaUser) {
return (Upload) super.setQuotaUser(quotaUser);
}
@Override
public Upload setUserIp(java.lang.String userIp) {
return (Upload) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Upload setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Upload setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The language code (a BCP-47 language tag) of the localized listing whose images are to
* read or modified. For example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the localized listing whose images are to read or
modified. For example, to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the localized listing whose images are to
* read or modified. For example, to select Austrian German, pass "de-AT".
*/
public Upload setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@com.google.api.client.util.Key
private java.lang.String imageType;
/**
*/
public java.lang.String getImageType() {
return imageType;
}
public Upload setImageType(java.lang.String imageType) {
this.imageType = imageType;
return this;
}
@Override
public Upload set(String parameterName, Object value) {
return (Upload) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Listings collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Listings.List request = androidpublisher.listings().list(parameters ...)}
*
*
* @return the resource collection
*/
public Listings listings() {
return new Listings();
}
/**
* The "listings" collection of methods.
*/
public class Listings {
/**
* Deletes the specified localized store listing from an edit.
*
* Create a request for the method "listings.delete".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
* to select Austrian German, pass "de-AT".
* @return the request
*/
public Delete delete(java.lang.String packageName, java.lang.String editId, java.lang.String language) throws java.io.IOException {
Delete result = new Delete(packageName, editId, language);
initialize(result);
return result;
}
public class Delete extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings/{language}";
/**
* Deletes the specified localized store listing from an edit.
*
* Create a request for the method "listings.delete".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
* to select Austrian German, pass "de-AT".
* @since 1.13
*/
protected Delete(java.lang.String packageName, java.lang.String editId, java.lang.String language) {
super(AndroidPublisher.this, "DELETE", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Delete setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Delete setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The language code (a BCP-47 language tag) of the localized listing to read or modify. For
* example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the localized listing to read or modify. For
* example, to select Austrian German, pass "de-AT".
*/
public Delete setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Deletes all localized listings from an edit.
*
* Create a request for the method "listings.deleteall".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Deleteall#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public Deleteall deleteall(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
Deleteall result = new Deleteall(packageName, editId);
initialize(result);
return result;
}
public class Deleteall extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings";
/**
* Deletes all localized listings from an edit.
*
* Create a request for the method "listings.deleteall".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Deleteall#execute()} method to invoke the remote
* operation. {@link
* Deleteall#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected Deleteall(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "DELETE", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public Deleteall setAlt(java.lang.String alt) {
return (Deleteall) super.setAlt(alt);
}
@Override
public Deleteall setFields(java.lang.String fields) {
return (Deleteall) super.setFields(fields);
}
@Override
public Deleteall setKey(java.lang.String key) {
return (Deleteall) super.setKey(key);
}
@Override
public Deleteall setOauthToken(java.lang.String oauthToken) {
return (Deleteall) super.setOauthToken(oauthToken);
}
@Override
public Deleteall setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Deleteall) super.setPrettyPrint(prettyPrint);
}
@Override
public Deleteall setQuotaUser(java.lang.String quotaUser) {
return (Deleteall) super.setQuotaUser(quotaUser);
}
@Override
public Deleteall setUserIp(java.lang.String userIp) {
return (Deleteall) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Deleteall setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Deleteall setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public Deleteall set(String parameterName, Object value) {
return (Deleteall) super.set(parameterName, value);
}
}
/**
* Fetches information about a localized store listing.
*
* Create a request for the method "listings.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
* to select Austrian German, pass "de-AT".
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String editId, java.lang.String language) throws java.io.IOException {
Get result = new Get(packageName, editId, language);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings/{language}";
/**
* Fetches information about a localized store listing.
*
* Create a request for the method "listings.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
* to select Austrian German, pass "de-AT".
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String editId, java.lang.String language) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.Listing.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Get setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The language code (a BCP-47 language tag) of the localized listing to read or modify. For
* example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the localized listing to read or modify. For
* example, to select Austrian German, pass "de-AT".
*/
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns all of the localized store listings attached to this edit.
*
* Create a request for the method "listings.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public List list(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
List result = new List(packageName, editId);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings";
/**
* Returns all of the localized store listings attached to this edit.
*
* Create a request for the method "listings.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected List(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.ListingsListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public List setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Creates or updates a localized store listing. This method supports patch semantics.
*
* Create a request for the method "listings.patch".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
* to select Austrian German, pass "de-AT".
* @param content the {@link com.google.api.services.androidpublisher.model.Listing}
* @return the request
*/
public Patch patch(java.lang.String packageName, java.lang.String editId, java.lang.String language, com.google.api.services.androidpublisher.model.Listing content) throws java.io.IOException {
Patch result = new Patch(packageName, editId, language, content);
initialize(result);
return result;
}
public class Patch extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings/{language}";
/**
* Creates or updates a localized store listing. This method supports patch semantics.
*
* Create a request for the method "listings.patch".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
* to select Austrian German, pass "de-AT".
* @param content the {@link com.google.api.services.androidpublisher.model.Listing}
* @since 1.13
*/
protected Patch(java.lang.String packageName, java.lang.String editId, java.lang.String language, com.google.api.services.androidpublisher.model.Listing content) {
super(AndroidPublisher.this, "PATCH", REST_PATH, content, com.google.api.services.androidpublisher.model.Listing.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Patch setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Patch setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The language code (a BCP-47 language tag) of the localized listing to read or modify. For
* example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the localized listing to read or modify. For
* example, to select Austrian German, pass "de-AT".
*/
public Patch setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Creates or updates a localized store listing.
*
* Create a request for the method "listings.update".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
* to select Austrian German, pass "de-AT".
* @param content the {@link com.google.api.services.androidpublisher.model.Listing}
* @return the request
*/
public Update update(java.lang.String packageName, java.lang.String editId, java.lang.String language, com.google.api.services.androidpublisher.model.Listing content) throws java.io.IOException {
Update result = new Update(packageName, editId, language, content);
initialize(result);
return result;
}
public class Update extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/listings/{language}";
/**
* Creates or updates a localized store listing.
*
* Create a request for the method "listings.update".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param language The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
* to select Austrian German, pass "de-AT".
* @param content the {@link com.google.api.services.androidpublisher.model.Listing}
* @since 1.13
*/
protected Update(java.lang.String packageName, java.lang.String editId, java.lang.String language, com.google.api.services.androidpublisher.model.Listing content) {
super(AndroidPublisher.this, "PUT", REST_PATH, content, com.google.api.services.androidpublisher.model.Listing.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.language = com.google.api.client.util.Preconditions.checkNotNull(language, "Required parameter language must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Update setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Update setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The language code (a BCP-47 language tag) of the localized listing to read or modify. For
* example, to select Austrian German, pass "de-AT".
*/
@com.google.api.client.util.Key
private java.lang.String language;
/** The language code (a BCP-47 language tag) of the localized listing to read or modify. For example,
to select Austrian German, pass "de-AT".
*/
public java.lang.String getLanguage() {
return language;
}
/**
* The language code (a BCP-47 language tag) of the localized listing to read or modify. For
* example, to select Austrian German, pass "de-AT".
*/
public Update setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Testers collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Testers.List request = androidpublisher.testers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Testers testers() {
return new Testers();
}
/**
* The "testers" collection of methods.
*/
public class Testers {
/**
* Create a request for the method "testers.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String editId, java.lang.String track) throws java.io.IOException {
Get result = new Get(packageName, editId, track);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/testers/{track}";
private final java.util.regex.Pattern TRACK_PATTERN =
java.util.regex.Pattern.compile("(alpha|beta|production|rollout)");
/**
* Create a request for the method "testers.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String editId, java.lang.String track) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.Testers.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.track = com.google.api.client.util.Preconditions.checkNotNull(track, "Required parameter track must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Get setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
@com.google.api.client.util.Key
private java.lang.String track;
/** The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
*/
public java.lang.String getTrack() {
return track;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
public Get setTrack(java.lang.String track) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
this.track = track;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Create a request for the method "testers.patch".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @param content the {@link com.google.api.services.androidpublisher.model.Testers}
* @return the request
*/
public Patch patch(java.lang.String packageName, java.lang.String editId, java.lang.String track, com.google.api.services.androidpublisher.model.Testers content) throws java.io.IOException {
Patch result = new Patch(packageName, editId, track, content);
initialize(result);
return result;
}
public class Patch extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/testers/{track}";
private final java.util.regex.Pattern TRACK_PATTERN =
java.util.regex.Pattern.compile("(alpha|beta|production|rollout)");
/**
* Create a request for the method "testers.patch".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @param content the {@link com.google.api.services.androidpublisher.model.Testers}
* @since 1.13
*/
protected Patch(java.lang.String packageName, java.lang.String editId, java.lang.String track, com.google.api.services.androidpublisher.model.Testers content) {
super(AndroidPublisher.this, "PATCH", REST_PATH, content, com.google.api.services.androidpublisher.model.Testers.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.track = com.google.api.client.util.Preconditions.checkNotNull(track, "Required parameter track must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Patch setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Patch setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
@com.google.api.client.util.Key
private java.lang.String track;
/** The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
*/
public java.lang.String getTrack() {
return track;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
public Patch setTrack(java.lang.String track) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
this.track = track;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Create a request for the method "testers.update".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @param content the {@link com.google.api.services.androidpublisher.model.Testers}
* @return the request
*/
public Update update(java.lang.String packageName, java.lang.String editId, java.lang.String track, com.google.api.services.androidpublisher.model.Testers content) throws java.io.IOException {
Update result = new Update(packageName, editId, track, content);
initialize(result);
return result;
}
public class Update extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/testers/{track}";
private final java.util.regex.Pattern TRACK_PATTERN =
java.util.regex.Pattern.compile("(alpha|beta|production|rollout)");
/**
* Create a request for the method "testers.update".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @param content the {@link com.google.api.services.androidpublisher.model.Testers}
* @since 1.13
*/
protected Update(java.lang.String packageName, java.lang.String editId, java.lang.String track, com.google.api.services.androidpublisher.model.Testers content) {
super(AndroidPublisher.this, "PUT", REST_PATH, content, com.google.api.services.androidpublisher.model.Testers.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.track = com.google.api.client.util.Preconditions.checkNotNull(track, "Required parameter track must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Update setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Update setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
@com.google.api.client.util.Key
private java.lang.String track;
/** The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
*/
public java.lang.String getTrack() {
return track;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
public Update setTrack(java.lang.String track) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
this.track = track;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Tracks collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Tracks.List request = androidpublisher.tracks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tracks tracks() {
return new Tracks();
}
/**
* The "tracks" collection of methods.
*/
public class Tracks {
/**
* Fetches the track configuration for the specified track type. Includes the APK version codes that
* are in this track.
*
* Create a request for the method "tracks.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String editId, java.lang.String track) throws java.io.IOException {
Get result = new Get(packageName, editId, track);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/tracks/{track}";
private final java.util.regex.Pattern TRACK_PATTERN =
java.util.regex.Pattern.compile("(alpha|beta|production|rollout)");
/**
* Fetches the track configuration for the specified track type. Includes the APK version codes
* that are in this track.
*
* Create a request for the method "tracks.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String editId, java.lang.String track) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.Track.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.track = com.google.api.client.util.Preconditions.checkNotNull(track, "Required parameter track must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Get setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
@com.google.api.client.util.Key
private java.lang.String track;
/** The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
*/
public java.lang.String getTrack() {
return track;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
public Get setTrack(java.lang.String track) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
this.track = track;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the track configurations for this edit.
*
* Create a request for the method "tracks.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @return the request
*/
public List list(java.lang.String packageName, java.lang.String editId) throws java.io.IOException {
List result = new List(packageName, editId);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/tracks";
/**
* Lists all the track configurations for this edit.
*
* Create a request for the method "tracks.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @since 1.13
*/
protected List(java.lang.String packageName, java.lang.String editId) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.TracksListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public List setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the track configuration for the specified track type. When halted, the rollout track
* cannot be updated without adding new APKs, and adding new APKs will cause it to resume. This
* method supports patch semantics.
*
* Create a request for the method "tracks.patch".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @param content the {@link com.google.api.services.androidpublisher.model.Track}
* @return the request
*/
public Patch patch(java.lang.String packageName, java.lang.String editId, java.lang.String track, com.google.api.services.androidpublisher.model.Track content) throws java.io.IOException {
Patch result = new Patch(packageName, editId, track, content);
initialize(result);
return result;
}
public class Patch extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/tracks/{track}";
private final java.util.regex.Pattern TRACK_PATTERN =
java.util.regex.Pattern.compile("(alpha|beta|production|rollout)");
/**
* Updates the track configuration for the specified track type. When halted, the rollout track
* cannot be updated without adding new APKs, and adding new APKs will cause it to resume. This
* method supports patch semantics.
*
* Create a request for the method "tracks.patch".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @param content the {@link com.google.api.services.androidpublisher.model.Track}
* @since 1.13
*/
protected Patch(java.lang.String packageName, java.lang.String editId, java.lang.String track, com.google.api.services.androidpublisher.model.Track content) {
super(AndroidPublisher.this, "PATCH", REST_PATH, content, com.google.api.services.androidpublisher.model.Track.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.track = com.google.api.client.util.Preconditions.checkNotNull(track, "Required parameter track must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Patch setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Patch setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
@com.google.api.client.util.Key
private java.lang.String track;
/** The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
*/
public java.lang.String getTrack() {
return track;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
public Patch setTrack(java.lang.String track) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
this.track = track;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates the track configuration for the specified track type. When halted, the rollout track
* cannot be updated without adding new APKs, and adding new APKs will cause it to resume.
*
* Create a request for the method "tracks.update".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @param content the {@link com.google.api.services.androidpublisher.model.Track}
* @return the request
*/
public Update update(java.lang.String packageName, java.lang.String editId, java.lang.String track, com.google.api.services.androidpublisher.model.Track content) throws java.io.IOException {
Update result = new Update(packageName, editId, track, content);
initialize(result);
return result;
}
public class Update extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/edits/{editId}/tracks/{track}";
private final java.util.regex.Pattern TRACK_PATTERN =
java.util.regex.Pattern.compile("(alpha|beta|production|rollout)");
/**
* Updates the track configuration for the specified track type. When halted, the rollout track
* cannot be updated without adding new APKs, and adding new APKs will cause it to resume.
*
* Create a request for the method "tracks.update".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
* @param editId Unique identifier for this edit.
* @param track The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
* @param content the {@link com.google.api.services.androidpublisher.model.Track}
* @since 1.13
*/
protected Update(java.lang.String packageName, java.lang.String editId, java.lang.String track, com.google.api.services.androidpublisher.model.Track content) {
super(AndroidPublisher.this, "PUT", REST_PATH, content, com.google.api.services.androidpublisher.model.Track.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.editId = com.google.api.client.util.Preconditions.checkNotNull(editId, "Required parameter editId must be specified.");
this.track = com.google.api.client.util.Preconditions.checkNotNull(track, "Required parameter track must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app that is being updated; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app that is being updated; for example,
* "com.spiffygame".
*/
public Update setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for this edit. */
@com.google.api.client.util.Key
private java.lang.String editId;
/** Unique identifier for this edit.
*/
public java.lang.String getEditId() {
return editId;
}
/** Unique identifier for this edit. */
public Update setEditId(java.lang.String editId) {
this.editId = editId;
return this;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
@com.google.api.client.util.Key
private java.lang.String track;
/** The track to read or modify. Acceptable values are: "alpha", "beta", "production" or "rollout".
*/
public java.lang.String getTrack() {
return track;
}
/**
* The track to read or modify. Acceptable values are: "alpha", "beta", "production" or
* "rollout".
*/
public Update setTrack(java.lang.String track) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(TRACK_PATTERN.matcher(track).matches(),
"Parameter track must conform to the pattern " +
"(alpha|beta|production|rollout)");
}
this.track = track;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Entitlements collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Entitlements.List request = androidpublisher.entitlements().list(parameters ...)}
*
*
* @return the resource collection
*/
public Entitlements entitlements() {
return new Entitlements();
}
/**
* The "entitlements" collection of methods.
*/
public class Entitlements {
/**
* Lists the user's current inapp item or subscription entitlements
*
* Create a request for the method "entitlements.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName The package name of the application the inapp product was sold in (for example, 'com.some.thing').
* @return the request
*/
public List list(java.lang.String packageName) throws java.io.IOException {
List result = new List(packageName);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/entitlements";
/**
* Lists the user's current inapp item or subscription entitlements
*
* Create a request for the method "entitlements.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName The package name of the application the inapp product was sold in (for example, 'com.some.thing').
* @since 1.13
*/
protected List(java.lang.String packageName) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.EntitlementsListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The package name of the application the inapp product was sold in (for example,
* 'com.some.thing').
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** The package name of the application the inapp product was sold in (for example, 'com.some.thing').
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the application the inapp product was sold in (for example,
* 'com.some.thing').
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/**
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* The product id of the inapp product (for example, 'sku1'). This can be used to restrict the
* result set.
*/
@com.google.api.client.util.Key
private java.lang.String productId;
/** The product id of the inapp product (for example, 'sku1'). This can be used to restrict the result
set.
*/
public java.lang.String getProductId() {
return productId;
}
/**
* The product id of the inapp product (for example, 'sku1'). This can be used to restrict the
* result set.
*/
public List setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/**
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
@com.google.api.client.util.Key
private java.lang.String token;
/**
*/
public java.lang.String getToken() {
return token;
}
public List setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Inappproducts collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Inappproducts.List request = androidpublisher.inappproducts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Inappproducts inappproducts() {
return new Inappproducts();
}
/**
* The "inappproducts" collection of methods.
*/
public class Inappproducts {
/**
* Delete an in-app product for an app.
*
* Create a request for the method "inappproducts.delete".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
* @param sku Unique identifier for the in-app product.
* @return the request
*/
public Delete delete(java.lang.String packageName, java.lang.String sku) throws java.io.IOException {
Delete result = new Delete(packageName, sku);
initialize(result);
return result;
}
public class Delete extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/inappproducts/{sku}";
/**
* Delete an in-app product for an app.
*
* Create a request for the method "inappproducts.delete".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
* @param sku Unique identifier for the in-app product.
* @since 1.13
*/
protected Delete(java.lang.String packageName, java.lang.String sku) {
super(AndroidPublisher.this, "DELETE", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.sku = com.google.api.client.util.Preconditions.checkNotNull(sku, "Required parameter sku must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app with the in-app product; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app with the in-app product; for example,
* "com.spiffygame".
*/
public Delete setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for the in-app product. */
@com.google.api.client.util.Key
private java.lang.String sku;
/** Unique identifier for the in-app product.
*/
public java.lang.String getSku() {
return sku;
}
/** Unique identifier for the in-app product. */
public Delete setSku(java.lang.String sku) {
this.sku = sku;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Returns information about the in-app product specified.
*
* Create a request for the method "inappproducts.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName
* @param sku Unique identifier for the in-app product.
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String sku) throws java.io.IOException {
Get result = new Get(packageName, sku);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/inappproducts/{sku}";
/**
* Returns information about the in-app product specified.
*
* Create a request for the method "inappproducts.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName
* @param sku Unique identifier for the in-app product.
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String sku) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.InAppProduct.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.sku = com.google.api.client.util.Preconditions.checkNotNull(sku, "Required parameter sku must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
@com.google.api.client.util.Key
private java.lang.String packageName;
/**
*/
public java.lang.String getPackageName() {
return packageName;
}
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for the in-app product. */
@com.google.api.client.util.Key
private java.lang.String sku;
/** Unique identifier for the in-app product.
*/
public java.lang.String getSku() {
return sku;
}
/** Unique identifier for the in-app product. */
public Get setSku(java.lang.String sku) {
this.sku = sku;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates a new in-app product for an app.
*
* Create a request for the method "inappproducts.insert".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app; for example, "com.spiffygame".
* @param content the {@link com.google.api.services.androidpublisher.model.InAppProduct}
* @return the request
*/
public Insert insert(java.lang.String packageName, com.google.api.services.androidpublisher.model.InAppProduct content) throws java.io.IOException {
Insert result = new Insert(packageName, content);
initialize(result);
return result;
}
public class Insert extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/inappproducts";
/**
* Creates a new in-app product for an app.
*
* Create a request for the method "inappproducts.insert".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Insert#execute()} method to invoke the remote operation.
* {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app; for example, "com.spiffygame".
* @param content the {@link com.google.api.services.androidpublisher.model.InAppProduct}
* @since 1.13
*/
protected Insert(java.lang.String packageName, com.google.api.services.androidpublisher.model.InAppProduct content) {
super(AndroidPublisher.this, "POST", REST_PATH, content, com.google.api.services.androidpublisher.model.InAppProduct.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/** Unique identifier for the Android app; for example, "com.spiffygame". */
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/** Unique identifier for the Android app; for example, "com.spiffygame". */
public Insert setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/**
* If true the prices for all regions targeted by the parent app that don't have a price
* specified for this in-app product will be auto converted to the target currency based on
* the default price. Defaults to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean autoConvertMissingPrices;
/** If true the prices for all regions targeted by the parent app that don't have a price specified for
this in-app product will be auto converted to the target currency based on the default price.
Defaults to false.
*/
public java.lang.Boolean getAutoConvertMissingPrices() {
return autoConvertMissingPrices;
}
/**
* If true the prices for all regions targeted by the parent app that don't have a price
* specified for this in-app product will be auto converted to the target currency based on
* the default price. Defaults to false.
*/
public Insert setAutoConvertMissingPrices(java.lang.Boolean autoConvertMissingPrices) {
this.autoConvertMissingPrices = autoConvertMissingPrices;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* List all the in-app products for an Android app, both subscriptions and managed in-app products..
*
* Create a request for the method "inappproducts.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app with in-app products; for example, "com.spiffygame".
* @return the request
*/
public List list(java.lang.String packageName) throws java.io.IOException {
List result = new List(packageName);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/inappproducts";
/**
* List all the in-app products for an Android app, both subscriptions and managed in-app
* products..
*
* Create a request for the method "inappproducts.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app with in-app products; for example, "com.spiffygame".
* @since 1.13
*/
protected List(java.lang.String packageName) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.InappproductsListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app with in-app products; for example, "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app with in-app products; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app with in-app products; for example, "com.spiffygame".
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/**
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/**
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
@com.google.api.client.util.Key
private java.lang.String token;
/**
*/
public java.lang.String getToken() {
return token;
}
public List setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the details of an in-app product. This method supports patch semantics.
*
* Create a request for the method "inappproducts.patch".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
* @param sku Unique identifier for the in-app product.
* @param content the {@link com.google.api.services.androidpublisher.model.InAppProduct}
* @return the request
*/
public Patch patch(java.lang.String packageName, java.lang.String sku, com.google.api.services.androidpublisher.model.InAppProduct content) throws java.io.IOException {
Patch result = new Patch(packageName, sku, content);
initialize(result);
return result;
}
public class Patch extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/inappproducts/{sku}";
/**
* Updates the details of an in-app product. This method supports patch semantics.
*
* Create a request for the method "inappproducts.patch".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
* @param sku Unique identifier for the in-app product.
* @param content the {@link com.google.api.services.androidpublisher.model.InAppProduct}
* @since 1.13
*/
protected Patch(java.lang.String packageName, java.lang.String sku, com.google.api.services.androidpublisher.model.InAppProduct content) {
super(AndroidPublisher.this, "PATCH", REST_PATH, content, com.google.api.services.androidpublisher.model.InAppProduct.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.sku = com.google.api.client.util.Preconditions.checkNotNull(sku, "Required parameter sku must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app with the in-app product; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app with the in-app product; for example,
* "com.spiffygame".
*/
public Patch setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for the in-app product. */
@com.google.api.client.util.Key
private java.lang.String sku;
/** Unique identifier for the in-app product.
*/
public java.lang.String getSku() {
return sku;
}
/** Unique identifier for the in-app product. */
public Patch setSku(java.lang.String sku) {
this.sku = sku;
return this;
}
/**
* If true the prices for all regions targeted by the parent app that don't have a price
* specified for this in-app product will be auto converted to the target currency based on
* the default price. Defaults to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean autoConvertMissingPrices;
/** If true the prices for all regions targeted by the parent app that don't have a price specified for
this in-app product will be auto converted to the target currency based on the default price.
Defaults to false.
*/
public java.lang.Boolean getAutoConvertMissingPrices() {
return autoConvertMissingPrices;
}
/**
* If true the prices for all regions targeted by the parent app that don't have a price
* specified for this in-app product will be auto converted to the target currency based on
* the default price. Defaults to false.
*/
public Patch setAutoConvertMissingPrices(java.lang.Boolean autoConvertMissingPrices) {
this.autoConvertMissingPrices = autoConvertMissingPrices;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates the details of an in-app product.
*
* Create a request for the method "inappproducts.update".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
* @param sku Unique identifier for the in-app product.
* @param content the {@link com.google.api.services.androidpublisher.model.InAppProduct}
* @return the request
*/
public Update update(java.lang.String packageName, java.lang.String sku, com.google.api.services.androidpublisher.model.InAppProduct content) throws java.io.IOException {
Update result = new Update(packageName, sku, content);
initialize(result);
return result;
}
public class Update extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/inappproducts/{sku}";
/**
* Updates the details of an in-app product.
*
* Create a request for the method "inappproducts.update".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
* @param sku Unique identifier for the in-app product.
* @param content the {@link com.google.api.services.androidpublisher.model.InAppProduct}
* @since 1.13
*/
protected Update(java.lang.String packageName, java.lang.String sku, com.google.api.services.androidpublisher.model.InAppProduct content) {
super(AndroidPublisher.this, "PUT", REST_PATH, content, com.google.api.services.androidpublisher.model.InAppProduct.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.sku = com.google.api.client.util.Preconditions.checkNotNull(sku, "Required parameter sku must be specified.");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app with the in-app product; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app with the in-app product; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app with the in-app product; for example,
* "com.spiffygame".
*/
public Update setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** Unique identifier for the in-app product. */
@com.google.api.client.util.Key
private java.lang.String sku;
/** Unique identifier for the in-app product.
*/
public java.lang.String getSku() {
return sku;
}
/** Unique identifier for the in-app product. */
public Update setSku(java.lang.String sku) {
this.sku = sku;
return this;
}
/**
* If true the prices for all regions targeted by the parent app that don't have a price
* specified for this in-app product will be auto converted to the target currency based on
* the default price. Defaults to false.
*/
@com.google.api.client.util.Key
private java.lang.Boolean autoConvertMissingPrices;
/** If true the prices for all regions targeted by the parent app that don't have a price specified for
this in-app product will be auto converted to the target currency based on the default price.
Defaults to false.
*/
public java.lang.Boolean getAutoConvertMissingPrices() {
return autoConvertMissingPrices;
}
/**
* If true the prices for all regions targeted by the parent app that don't have a price
* specified for this in-app product will be auto converted to the target currency based on
* the default price. Defaults to false.
*/
public Update setAutoConvertMissingPrices(java.lang.Boolean autoConvertMissingPrices) {
this.autoConvertMissingPrices = autoConvertMissingPrices;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Purchases collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Purchases.List request = androidpublisher.purchases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Purchases purchases() {
return new Purchases();
}
/**
* The "purchases" collection of methods.
*/
public class Purchases {
/**
* An accessor for creating requests from the Products collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Products.List request = androidpublisher.products().list(parameters ...)}
*
*
* @return the resource collection
*/
public Products products() {
return new Products();
}
/**
* The "products" collection of methods.
*/
public class Products {
/**
* Checks the purchase and consumption status of an inapp item.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName The package name of the application the inapp product was sold in (for example, 'com.some.thing').
* @param productId The inapp product SKU (for example, 'com.some.thing.inapp1').
* @param token The token provided to the user's device when the inapp product was purchased.
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String productId, java.lang.String token) throws java.io.IOException {
Get result = new Get(packageName, productId, token);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/purchases/products/{productId}/tokens/{token}";
/**
* Checks the purchase and consumption status of an inapp item.
*
* Create a request for the method "products.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName The package name of the application the inapp product was sold in (for example, 'com.some.thing').
* @param productId The inapp product SKU (for example, 'com.some.thing.inapp1').
* @param token The token provided to the user's device when the inapp product was purchased.
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String productId, java.lang.String token) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.ProductPurchase.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.productId = com.google.api.client.util.Preconditions.checkNotNull(productId, "Required parameter productId must be specified.");
this.token = com.google.api.client.util.Preconditions.checkNotNull(token, "Required parameter token must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The package name of the application the inapp product was sold in (for example,
* 'com.some.thing').
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** The package name of the application the inapp product was sold in (for example, 'com.some.thing').
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the application the inapp product was sold in (for example,
* 'com.some.thing').
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** The inapp product SKU (for example, 'com.some.thing.inapp1'). */
@com.google.api.client.util.Key
private java.lang.String productId;
/** The inapp product SKU (for example, 'com.some.thing.inapp1').
*/
public java.lang.String getProductId() {
return productId;
}
/** The inapp product SKU (for example, 'com.some.thing.inapp1'). */
public Get setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
/** The token provided to the user's device when the inapp product was purchased. */
@com.google.api.client.util.Key
private java.lang.String token;
/** The token provided to the user's device when the inapp product was purchased.
*/
public java.lang.String getToken() {
return token;
}
/** The token provided to the user's device when the inapp product was purchased. */
public Get setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Subscriptions collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Subscriptions.List request = androidpublisher.subscriptions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Subscriptions subscriptions() {
return new Subscriptions();
}
/**
* The "subscriptions" collection of methods.
*/
public class Subscriptions {
/**
* Cancels a user's subscription purchase. The subscription remains valid until its expiration time.
*
* Create a request for the method "subscriptions.cancel".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @return the request
*/
public Cancel cancel(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token) throws java.io.IOException {
Cancel result = new Cancel(packageName, subscriptionId, token);
initialize(result);
return result;
}
public class Cancel extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/purchases/subscriptions/{subscriptionId}/tokens/{token}:cancel";
/**
* Cancels a user's subscription purchase. The subscription remains valid until its expiration
* time.
*
* Create a request for the method "subscriptions.cancel".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @since 1.13
*/
protected Cancel(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token) {
super(AndroidPublisher.this, "POST", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.subscriptionId = com.google.api.client.util.Preconditions.checkNotNull(subscriptionId, "Required parameter subscriptionId must be specified.");
this.token = com.google.api.client.util.Preconditions.checkNotNull(token, "Required parameter token must be specified.");
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUserIp(java.lang.String userIp) {
return (Cancel) super.setUserIp(userIp);
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** The package name of the application for which this subscription was purchased (for example,
'com.some.thing').
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
public Cancel setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** The purchased subscription ID (for example, 'monthly001'). */
@com.google.api.client.util.Key
private java.lang.String subscriptionId;
/** The purchased subscription ID (for example, 'monthly001').
*/
public java.lang.String getSubscriptionId() {
return subscriptionId;
}
/** The purchased subscription ID (for example, 'monthly001'). */
public Cancel setSubscriptionId(java.lang.String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/** The token provided to the user's device when the subscription was purchased. */
@com.google.api.client.util.Key
private java.lang.String token;
/** The token provided to the user's device when the subscription was purchased.
*/
public java.lang.String getToken() {
return token;
}
/** The token provided to the user's device when the subscription was purchased. */
public Cancel setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Defers a user's subscription purchase until a specified future expiration time.
*
* Create a request for the method "subscriptions.defer".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Defer#execute()} method to invoke the remote operation.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @param content the {@link com.google.api.services.androidpublisher.model.SubscriptionPurchasesDeferRequest}
* @return the request
*/
public Defer defer(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token, com.google.api.services.androidpublisher.model.SubscriptionPurchasesDeferRequest content) throws java.io.IOException {
Defer result = new Defer(packageName, subscriptionId, token, content);
initialize(result);
return result;
}
public class Defer extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/purchases/subscriptions/{subscriptionId}/tokens/{token}:defer";
/**
* Defers a user's subscription purchase until a specified future expiration time.
*
* Create a request for the method "subscriptions.defer".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Defer#execute()} method to invoke the remote operation.
* {@link
* Defer#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @param content the {@link com.google.api.services.androidpublisher.model.SubscriptionPurchasesDeferRequest}
* @since 1.13
*/
protected Defer(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token, com.google.api.services.androidpublisher.model.SubscriptionPurchasesDeferRequest content) {
super(AndroidPublisher.this, "POST", REST_PATH, content, com.google.api.services.androidpublisher.model.SubscriptionPurchasesDeferResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.subscriptionId = com.google.api.client.util.Preconditions.checkNotNull(subscriptionId, "Required parameter subscriptionId must be specified.");
this.token = com.google.api.client.util.Preconditions.checkNotNull(token, "Required parameter token must be specified.");
}
@Override
public Defer setAlt(java.lang.String alt) {
return (Defer) super.setAlt(alt);
}
@Override
public Defer setFields(java.lang.String fields) {
return (Defer) super.setFields(fields);
}
@Override
public Defer setKey(java.lang.String key) {
return (Defer) super.setKey(key);
}
@Override
public Defer setOauthToken(java.lang.String oauthToken) {
return (Defer) super.setOauthToken(oauthToken);
}
@Override
public Defer setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Defer) super.setPrettyPrint(prettyPrint);
}
@Override
public Defer setQuotaUser(java.lang.String quotaUser) {
return (Defer) super.setQuotaUser(quotaUser);
}
@Override
public Defer setUserIp(java.lang.String userIp) {
return (Defer) super.setUserIp(userIp);
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** The package name of the application for which this subscription was purchased (for example,
'com.some.thing').
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
public Defer setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** The purchased subscription ID (for example, 'monthly001'). */
@com.google.api.client.util.Key
private java.lang.String subscriptionId;
/** The purchased subscription ID (for example, 'monthly001').
*/
public java.lang.String getSubscriptionId() {
return subscriptionId;
}
/** The purchased subscription ID (for example, 'monthly001'). */
public Defer setSubscriptionId(java.lang.String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/** The token provided to the user's device when the subscription was purchased. */
@com.google.api.client.util.Key
private java.lang.String token;
/** The token provided to the user's device when the subscription was purchased.
*/
public java.lang.String getToken() {
return token;
}
/** The token provided to the user's device when the subscription was purchased. */
public Defer setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public Defer set(String parameterName, Object value) {
return (Defer) super.set(parameterName, value);
}
}
/**
* Checks whether a user's subscription purchase is valid and returns its expiry time.
*
* Create a request for the method "subscriptions.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token) throws java.io.IOException {
Get result = new Get(packageName, subscriptionId, token);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/purchases/subscriptions/{subscriptionId}/tokens/{token}";
/**
* Checks whether a user's subscription purchase is valid and returns its expiry time.
*
* Create a request for the method "subscriptions.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.SubscriptionPurchase.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.subscriptionId = com.google.api.client.util.Preconditions.checkNotNull(subscriptionId, "Required parameter subscriptionId must be specified.");
this.token = com.google.api.client.util.Preconditions.checkNotNull(token, "Required parameter token must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** The package name of the application for which this subscription was purchased (for example,
'com.some.thing').
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** The purchased subscription ID (for example, 'monthly001'). */
@com.google.api.client.util.Key
private java.lang.String subscriptionId;
/** The purchased subscription ID (for example, 'monthly001').
*/
public java.lang.String getSubscriptionId() {
return subscriptionId;
}
/** The purchased subscription ID (for example, 'monthly001'). */
public Get setSubscriptionId(java.lang.String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/** The token provided to the user's device when the subscription was purchased. */
@com.google.api.client.util.Key
private java.lang.String token;
/** The token provided to the user's device when the subscription was purchased.
*/
public java.lang.String getToken() {
return token;
}
/** The token provided to the user's device when the subscription was purchased. */
public Get setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Refunds a user's subscription purchase, but the subscription remains valid until its expiration
* time and it will continue to recur.
*
* Create a request for the method "subscriptions.refund".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Refund#execute()} method to invoke the remote operation.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @return the request
*/
public Refund refund(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token) throws java.io.IOException {
Refund result = new Refund(packageName, subscriptionId, token);
initialize(result);
return result;
}
public class Refund extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/purchases/subscriptions/{subscriptionId}/tokens/{token}:refund";
/**
* Refunds a user's subscription purchase, but the subscription remains valid until its expiration
* time and it will continue to recur.
*
* Create a request for the method "subscriptions.refund".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Refund#execute()} method to invoke the remote operation.
* {@link
* Refund#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @since 1.13
*/
protected Refund(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token) {
super(AndroidPublisher.this, "POST", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.subscriptionId = com.google.api.client.util.Preconditions.checkNotNull(subscriptionId, "Required parameter subscriptionId must be specified.");
this.token = com.google.api.client.util.Preconditions.checkNotNull(token, "Required parameter token must be specified.");
}
@Override
public Refund setAlt(java.lang.String alt) {
return (Refund) super.setAlt(alt);
}
@Override
public Refund setFields(java.lang.String fields) {
return (Refund) super.setFields(fields);
}
@Override
public Refund setKey(java.lang.String key) {
return (Refund) super.setKey(key);
}
@Override
public Refund setOauthToken(java.lang.String oauthToken) {
return (Refund) super.setOauthToken(oauthToken);
}
@Override
public Refund setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Refund) super.setPrettyPrint(prettyPrint);
}
@Override
public Refund setQuotaUser(java.lang.String quotaUser) {
return (Refund) super.setQuotaUser(quotaUser);
}
@Override
public Refund setUserIp(java.lang.String userIp) {
return (Refund) super.setUserIp(userIp);
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** The package name of the application for which this subscription was purchased (for example,
'com.some.thing').
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
public Refund setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** The purchased subscription ID (for example, 'monthly001'). */
@com.google.api.client.util.Key
private java.lang.String subscriptionId;
/** The purchased subscription ID (for example, 'monthly001').
*/
public java.lang.String getSubscriptionId() {
return subscriptionId;
}
/** The purchased subscription ID (for example, 'monthly001'). */
public Refund setSubscriptionId(java.lang.String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/** The token provided to the user's device when the subscription was purchased. */
@com.google.api.client.util.Key
private java.lang.String token;
/** The token provided to the user's device when the subscription was purchased.
*/
public java.lang.String getToken() {
return token;
}
/** The token provided to the user's device when the subscription was purchased. */
public Refund setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public Refund set(String parameterName, Object value) {
return (Refund) super.set(parameterName, value);
}
}
/**
* Refunds and immediately revokes a user's subscription purchase. Access to the subscription will
* be terminated immediately and it will stop recurring.
*
* Create a request for the method "subscriptions.revoke".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Revoke#execute()} method to invoke the remote operation.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @return the request
*/
public Revoke revoke(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token) throws java.io.IOException {
Revoke result = new Revoke(packageName, subscriptionId, token);
initialize(result);
return result;
}
public class Revoke extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/purchases/subscriptions/{subscriptionId}/tokens/{token}:revoke";
/**
* Refunds and immediately revokes a user's subscription purchase. Access to the subscription will
* be terminated immediately and it will stop recurring.
*
* Create a request for the method "subscriptions.revoke".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Revoke#execute()} method to invoke the remote operation.
* {@link
* Revoke#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName The package name of the application for which this subscription was purchased (for example,
* 'com.some.thing').
* @param subscriptionId The purchased subscription ID (for example, 'monthly001').
* @param token The token provided to the user's device when the subscription was purchased.
* @since 1.13
*/
protected Revoke(java.lang.String packageName, java.lang.String subscriptionId, java.lang.String token) {
super(AndroidPublisher.this, "POST", REST_PATH, null, Void.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.subscriptionId = com.google.api.client.util.Preconditions.checkNotNull(subscriptionId, "Required parameter subscriptionId must be specified.");
this.token = com.google.api.client.util.Preconditions.checkNotNull(token, "Required parameter token must be specified.");
}
@Override
public Revoke setAlt(java.lang.String alt) {
return (Revoke) super.setAlt(alt);
}
@Override
public Revoke setFields(java.lang.String fields) {
return (Revoke) super.setFields(fields);
}
@Override
public Revoke setKey(java.lang.String key) {
return (Revoke) super.setKey(key);
}
@Override
public Revoke setOauthToken(java.lang.String oauthToken) {
return (Revoke) super.setOauthToken(oauthToken);
}
@Override
public Revoke setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Revoke) super.setPrettyPrint(prettyPrint);
}
@Override
public Revoke setQuotaUser(java.lang.String quotaUser) {
return (Revoke) super.setQuotaUser(quotaUser);
}
@Override
public Revoke setUserIp(java.lang.String userIp) {
return (Revoke) super.setUserIp(userIp);
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** The package name of the application for which this subscription was purchased (for example,
'com.some.thing').
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the application for which this subscription was purchased (for
* example, 'com.some.thing').
*/
public Revoke setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/** The purchased subscription ID (for example, 'monthly001'). */
@com.google.api.client.util.Key
private java.lang.String subscriptionId;
/** The purchased subscription ID (for example, 'monthly001').
*/
public java.lang.String getSubscriptionId() {
return subscriptionId;
}
/** The purchased subscription ID (for example, 'monthly001'). */
public Revoke setSubscriptionId(java.lang.String subscriptionId) {
this.subscriptionId = subscriptionId;
return this;
}
/** The token provided to the user's device when the subscription was purchased. */
@com.google.api.client.util.Key
private java.lang.String token;
/** The token provided to the user's device when the subscription was purchased.
*/
public java.lang.String getToken() {
return token;
}
/** The token provided to the user's device when the subscription was purchased. */
public Revoke setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public Revoke set(String parameterName, Object value) {
return (Revoke) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Voidedpurchases collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Voidedpurchases.List request = androidpublisher.voidedpurchases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Voidedpurchases voidedpurchases() {
return new Voidedpurchases();
}
/**
* The "voidedpurchases" collection of methods.
*/
public class Voidedpurchases {
/**
* Lists the purchases that were canceled, refunded or charged-back.
*
* Create a request for the method "voidedpurchases.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName The package name of the application for which voided purchases need to be returned (for example,
* 'com.some.thing').
* @return the request
*/
public List list(java.lang.String packageName) throws java.io.IOException {
List result = new List(packageName);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/purchases/voidedpurchases";
/**
* Lists the purchases that were canceled, refunded or charged-back.
*
* Create a request for the method "voidedpurchases.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName The package name of the application for which voided purchases need to be returned (for example,
* 'com.some.thing').
* @since 1.13
*/
protected List(java.lang.String packageName) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.VoidedPurchasesListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The package name of the application for which voided purchases need to be returned (for
* example, 'com.some.thing').
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** The package name of the application for which voided purchases need to be returned (for example,
'com.some.thing').
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* The package name of the application for which voided purchases need to be returned (for
* example, 'com.some.thing').
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/**
* The time, in milliseconds since the Epoch, of the newest voided in-app product purchase
* that you want to see in the response. The value of this parameter cannot be greater than
* the current time and is ignored if a pagination token is set. Default value is current
* time. Note: This filter is applied on the time at which the record is seen as voided by
* our systems and not the actual voided time returned in the response.
*/
@com.google.api.client.util.Key
private java.lang.Long endTime;
/** The time, in milliseconds since the Epoch, of the newest voided in-app product purchase that you
want to see in the response. The value of this parameter cannot be greater than the current time
and is ignored if a pagination token is set. Default value is current time. Note: This filter is
applied on the time at which the record is seen as voided by our systems and not the actual voided
time returned in the response.
*/
public java.lang.Long getEndTime() {
return endTime;
}
/**
* The time, in milliseconds since the Epoch, of the newest voided in-app product purchase
* that you want to see in the response. The value of this parameter cannot be greater than
* the current time and is ignored if a pagination token is set. Default value is current
* time. Note: This filter is applied on the time at which the record is seen as voided by
* our systems and not the actual voided time returned in the response.
*/
public List setEndTime(java.lang.Long endTime) {
this.endTime = endTime;
return this;
}
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/**
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/**
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
/**
* The time, in milliseconds since the Epoch, of the oldest voided in-app product purchase
* that you want to see in the response. The value of this parameter cannot be older than 30
* days and is ignored if a pagination token is set. Default value is current time minus 30
* days. Note: This filter is applied on the time at which the record is seen as voided by
* our systems and not the actual voided time returned in the response.
*/
@com.google.api.client.util.Key
private java.lang.Long startTime;
/** The time, in milliseconds since the Epoch, of the oldest voided in-app product purchase that you
want to see in the response. The value of this parameter cannot be older than 30 days and is
ignored if a pagination token is set. Default value is current time minus 30 days. Note: This
filter is applied on the time at which the record is seen as voided by our systems and not the
actual voided time returned in the response.
*/
public java.lang.Long getStartTime() {
return startTime;
}
/**
* The time, in milliseconds since the Epoch, of the oldest voided in-app product purchase
* that you want to see in the response. The value of this parameter cannot be older than 30
* days and is ignored if a pagination token is set. Default value is current time minus 30
* days. Note: This filter is applied on the time at which the record is seen as voided by
* our systems and not the actual voided time returned in the response.
*/
public List setStartTime(java.lang.Long startTime) {
this.startTime = startTime;
return this;
}
@com.google.api.client.util.Key
private java.lang.String token;
/**
*/
public java.lang.String getToken() {
return token;
}
public List setToken(java.lang.String token) {
this.token = token;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Reviews collection.
*
* The typical use is:
*
* {@code AndroidPublisher androidpublisher = new AndroidPublisher(...);}
* {@code AndroidPublisher.Reviews.List request = androidpublisher.reviews().list(parameters ...)}
*
*
* @return the resource collection
*/
public Reviews reviews() {
return new Reviews();
}
/**
* The "reviews" collection of methods.
*/
public class Reviews {
/**
* Returns a single review.
*
* Create a request for the method "reviews.get".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
* @param reviewId
* @return the request
*/
public Get get(java.lang.String packageName, java.lang.String reviewId) throws java.io.IOException {
Get result = new Get(packageName, reviewId);
initialize(result);
return result;
}
public class Get extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/reviews/{reviewId}";
/**
* Returns a single review.
*
* Create a request for the method "reviews.get".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
* @param reviewId
* @since 1.13
*/
protected Get(java.lang.String packageName, java.lang.String reviewId) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.Review.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.reviewId = com.google.api.client.util.Preconditions.checkNotNull(reviewId, "Required parameter reviewId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app for which we want reviews; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app for which we want reviews; for example,
* "com.spiffygame".
*/
public Get setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
@com.google.api.client.util.Key
private java.lang.String reviewId;
/**
*/
public java.lang.String getReviewId() {
return reviewId;
}
public Get setReviewId(java.lang.String reviewId) {
this.reviewId = reviewId;
return this;
}
@com.google.api.client.util.Key
private java.lang.String translationLanguage;
/**
*/
public java.lang.String getTranslationLanguage() {
return translationLanguage;
}
public Get setTranslationLanguage(java.lang.String translationLanguage) {
this.translationLanguage = translationLanguage;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns a list of reviews. Only reviews from last week will be returned.
*
* Create a request for the method "reviews.list".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
* @return the request
*/
public List list(java.lang.String packageName) throws java.io.IOException {
List result = new List(packageName);
initialize(result);
return result;
}
public class List extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/reviews";
/**
* Returns a list of reviews. Only reviews from last week will be returned.
*
* Create a request for the method "reviews.list".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
* @since 1.13
*/
protected List(java.lang.String packageName) {
super(AndroidPublisher.this, "GET", REST_PATH, null, com.google.api.services.androidpublisher.model.ReviewsListResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app for which we want reviews; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app for which we want reviews; for example,
* "com.spiffygame".
*/
public List setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/**
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
@com.google.api.client.util.Key
private java.lang.Long startIndex;
/**
*/
public java.lang.Long getStartIndex() {
return startIndex;
}
public List setStartIndex(java.lang.Long startIndex) {
this.startIndex = startIndex;
return this;
}
@com.google.api.client.util.Key
private java.lang.String token;
/**
*/
public java.lang.String getToken() {
return token;
}
public List setToken(java.lang.String token) {
this.token = token;
return this;
}
@com.google.api.client.util.Key
private java.lang.String translationLanguage;
/**
*/
public java.lang.String getTranslationLanguage() {
return translationLanguage;
}
public List setTranslationLanguage(java.lang.String translationLanguage) {
this.translationLanguage = translationLanguage;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Reply to a single review, or update an existing reply.
*
* Create a request for the method "reviews.reply".
*
* This request holds the parameters needed by the androidpublisher server. After setting any
* optional parameters, call the {@link Reply#execute()} method to invoke the remote operation.
*
* @param packageName Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
* @param reviewId
* @param content the {@link com.google.api.services.androidpublisher.model.ReviewsReplyRequest}
* @return the request
*/
public Reply reply(java.lang.String packageName, java.lang.String reviewId, com.google.api.services.androidpublisher.model.ReviewsReplyRequest content) throws java.io.IOException {
Reply result = new Reply(packageName, reviewId, content);
initialize(result);
return result;
}
public class Reply extends AndroidPublisherRequest {
private static final String REST_PATH = "{packageName}/reviews/{reviewId}:reply";
/**
* Reply to a single review, or update an existing reply.
*
* Create a request for the method "reviews.reply".
*
* This request holds the parameters needed by the the androidpublisher server. After setting any
* optional parameters, call the {@link Reply#execute()} method to invoke the remote operation.
* {@link
* Reply#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param packageName Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
* @param reviewId
* @param content the {@link com.google.api.services.androidpublisher.model.ReviewsReplyRequest}
* @since 1.13
*/
protected Reply(java.lang.String packageName, java.lang.String reviewId, com.google.api.services.androidpublisher.model.ReviewsReplyRequest content) {
super(AndroidPublisher.this, "POST", REST_PATH, content, com.google.api.services.androidpublisher.model.ReviewsReplyResponse.class);
this.packageName = com.google.api.client.util.Preconditions.checkNotNull(packageName, "Required parameter packageName must be specified.");
this.reviewId = com.google.api.client.util.Preconditions.checkNotNull(reviewId, "Required parameter reviewId must be specified.");
}
@Override
public Reply setAlt(java.lang.String alt) {
return (Reply) super.setAlt(alt);
}
@Override
public Reply setFields(java.lang.String fields) {
return (Reply) super.setFields(fields);
}
@Override
public Reply setKey(java.lang.String key) {
return (Reply) super.setKey(key);
}
@Override
public Reply setOauthToken(java.lang.String oauthToken) {
return (Reply) super.setOauthToken(oauthToken);
}
@Override
public Reply setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Reply) super.setPrettyPrint(prettyPrint);
}
@Override
public Reply setQuotaUser(java.lang.String quotaUser) {
return (Reply) super.setQuotaUser(quotaUser);
}
@Override
public Reply setUserIp(java.lang.String userIp) {
return (Reply) super.setUserIp(userIp);
}
/**
* Unique identifier for the Android app for which we want reviews; for example,
* "com.spiffygame".
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/** Unique identifier for the Android app for which we want reviews; for example, "com.spiffygame".
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Unique identifier for the Android app for which we want reviews; for example,
* "com.spiffygame".
*/
public Reply setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
@com.google.api.client.util.Key
private java.lang.String reviewId;
/**
*/
public java.lang.String getReviewId() {
return reviewId;
}
public Reply setReviewId(java.lang.String reviewId) {
this.reviewId = reviewId;
return this;
}
@Override
public Reply set(String parameterName, Object value) {
return (Reply) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link AndroidPublisher}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link AndroidPublisher}. */
@Override
public AndroidPublisher build() {
return new AndroidPublisher(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AndroidPublisherRequestInitializer}.
*
* @since 1.12
*/
public Builder setAndroidPublisherRequestInitializer(
AndroidPublisherRequestInitializer androidpublisherRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(androidpublisherRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}