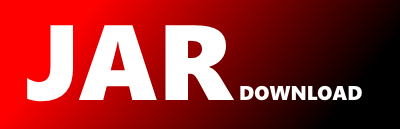
com.google.api.services.androidpublisher.model.SubscriptionOffer Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.androidpublisher.model;
/**
* A single, temporary offer
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Play Android Developer API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SubscriptionOffer extends com.google.api.client.json.GenericJson {
/**
* Required. Immutable. The ID of the base plan to which this offer is an extension.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String basePlanId;
/**
* Required. Immutable. Unique ID of this subscription offer. Must be unique within the base plan.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String offerId;
/**
* List of up to 20 custom tags specified for this offer, and returned to the app through the
* billing library.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List offerTags;
static {
// hack to force ProGuard to consider OfferTag used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(OfferTag.class);
}
/**
* The configuration for any new locations Play may launch in the future.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private OtherRegionsSubscriptionOfferConfig otherRegionsConfig;
/**
* Required. Immutable. The package name of the app the parent subscription belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String packageName;
/**
* Required. The phases of this subscription offer. Must contain at least one entry, and may
* contain at most five. Users will always receive all these phases in the specified order. Phases
* may not be added, removed, or reordered after initial creation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List phases;
/**
* Required. Immutable. The ID of the parent subscription this offer belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String productId;
/**
* Required. The region-specific configuration of this offer. Must contain at least one entry.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List regionalConfigs;
static {
// hack to force ProGuard to consider RegionalSubscriptionOfferConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(RegionalSubscriptionOfferConfig.class);
}
/**
* Output only. The current state of this offer. Can be changed using Activate and Deactivate
* actions. NB: the base plan state supersedes this state, so an active offer may not be available
* if the base plan is not active.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* The requirements that users need to fulfil to be eligible for this offer. Represents the
* requirements that Play will evaluate to decide whether an offer should be returned. Developers
* may further filter these offers themselves.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SubscriptionOfferTargeting targeting;
/**
* Required. Immutable. The ID of the base plan to which this offer is an extension.
* @return value or {@code null} for none
*/
public java.lang.String getBasePlanId() {
return basePlanId;
}
/**
* Required. Immutable. The ID of the base plan to which this offer is an extension.
* @param basePlanId basePlanId or {@code null} for none
*/
public SubscriptionOffer setBasePlanId(java.lang.String basePlanId) {
this.basePlanId = basePlanId;
return this;
}
/**
* Required. Immutable. Unique ID of this subscription offer. Must be unique within the base plan.
* @return value or {@code null} for none
*/
public java.lang.String getOfferId() {
return offerId;
}
/**
* Required. Immutable. Unique ID of this subscription offer. Must be unique within the base plan.
* @param offerId offerId or {@code null} for none
*/
public SubscriptionOffer setOfferId(java.lang.String offerId) {
this.offerId = offerId;
return this;
}
/**
* List of up to 20 custom tags specified for this offer, and returned to the app through the
* billing library.
* @return value or {@code null} for none
*/
public java.util.List getOfferTags() {
return offerTags;
}
/**
* List of up to 20 custom tags specified for this offer, and returned to the app through the
* billing library.
* @param offerTags offerTags or {@code null} for none
*/
public SubscriptionOffer setOfferTags(java.util.List offerTags) {
this.offerTags = offerTags;
return this;
}
/**
* The configuration for any new locations Play may launch in the future.
* @return value or {@code null} for none
*/
public OtherRegionsSubscriptionOfferConfig getOtherRegionsConfig() {
return otherRegionsConfig;
}
/**
* The configuration for any new locations Play may launch in the future.
* @param otherRegionsConfig otherRegionsConfig or {@code null} for none
*/
public SubscriptionOffer setOtherRegionsConfig(OtherRegionsSubscriptionOfferConfig otherRegionsConfig) {
this.otherRegionsConfig = otherRegionsConfig;
return this;
}
/**
* Required. Immutable. The package name of the app the parent subscription belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getPackageName() {
return packageName;
}
/**
* Required. Immutable. The package name of the app the parent subscription belongs to.
* @param packageName packageName or {@code null} for none
*/
public SubscriptionOffer setPackageName(java.lang.String packageName) {
this.packageName = packageName;
return this;
}
/**
* Required. The phases of this subscription offer. Must contain at least one entry, and may
* contain at most five. Users will always receive all these phases in the specified order. Phases
* may not be added, removed, or reordered after initial creation.
* @return value or {@code null} for none
*/
public java.util.List getPhases() {
return phases;
}
/**
* Required. The phases of this subscription offer. Must contain at least one entry, and may
* contain at most five. Users will always receive all these phases in the specified order. Phases
* may not be added, removed, or reordered after initial creation.
* @param phases phases or {@code null} for none
*/
public SubscriptionOffer setPhases(java.util.List phases) {
this.phases = phases;
return this;
}
/**
* Required. Immutable. The ID of the parent subscription this offer belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getProductId() {
return productId;
}
/**
* Required. Immutable. The ID of the parent subscription this offer belongs to.
* @param productId productId or {@code null} for none
*/
public SubscriptionOffer setProductId(java.lang.String productId) {
this.productId = productId;
return this;
}
/**
* Required. The region-specific configuration of this offer. Must contain at least one entry.
* @return value or {@code null} for none
*/
public java.util.List getRegionalConfigs() {
return regionalConfigs;
}
/**
* Required. The region-specific configuration of this offer. Must contain at least one entry.
* @param regionalConfigs regionalConfigs or {@code null} for none
*/
public SubscriptionOffer setRegionalConfigs(java.util.List regionalConfigs) {
this.regionalConfigs = regionalConfigs;
return this;
}
/**
* Output only. The current state of this offer. Can be changed using Activate and Deactivate
* actions. NB: the base plan state supersedes this state, so an active offer may not be available
* if the base plan is not active.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. The current state of this offer. Can be changed using Activate and Deactivate
* actions. NB: the base plan state supersedes this state, so an active offer may not be available
* if the base plan is not active.
* @param state state or {@code null} for none
*/
public SubscriptionOffer setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* The requirements that users need to fulfil to be eligible for this offer. Represents the
* requirements that Play will evaluate to decide whether an offer should be returned. Developers
* may further filter these offers themselves.
* @return value or {@code null} for none
*/
public SubscriptionOfferTargeting getTargeting() {
return targeting;
}
/**
* The requirements that users need to fulfil to be eligible for this offer. Represents the
* requirements that Play will evaluate to decide whether an offer should be returned. Developers
* may further filter these offers themselves.
* @param targeting targeting or {@code null} for none
*/
public SubscriptionOffer setTargeting(SubscriptionOfferTargeting targeting) {
this.targeting = targeting;
return this;
}
@Override
public SubscriptionOffer set(String fieldName, Object value) {
return (SubscriptionOffer) super.set(fieldName, value);
}
@Override
public SubscriptionOffer clone() {
return (SubscriptionOffer) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy