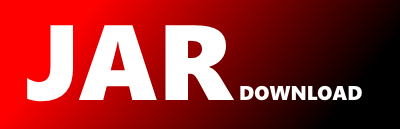
target.apidocs.com.google.api.services.androidpublisher.AndroidPublisher.html Maven / Gradle / Ivy
AndroidPublisher (Google Play Android Developer API v3-rev20240129-2.0.0)
com.google.api.services.androidpublisher
Class AndroidPublisher
- java.lang.Object
-
- com.google.api.client.googleapis.services.AbstractGoogleClient
-
- com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient
-
- com.google.api.services.androidpublisher.AndroidPublisher
-
public class AndroidPublisher
extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient
Service definition for AndroidPublisher (v3).
Lets Android application developers access their Google Play accounts. At a high level, the expected workflow is to "insert" an Edit, make changes as necessary, and then "commit" it.
For more information about this service, see the
API Documentation
This service uses AndroidPublisherRequestInitializer
to initialize global parameters via its
AndroidPublisher.Builder
.
- Since:
- 1.3
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
class
AndroidPublisher.Applications
The "applications" collection of methods.
class
AndroidPublisher.Apprecovery
The "apprecovery" collection of methods.
static class
AndroidPublisher.Builder
Builder for AndroidPublisher
.
class
AndroidPublisher.Edits
The "edits" collection of methods.
class
AndroidPublisher.Externaltransactions
The "externaltransactions" collection of methods.
class
AndroidPublisher.Generatedapks
The "generatedapks" collection of methods.
class
AndroidPublisher.Grants
The "grants" collection of methods.
class
AndroidPublisher.Inappproducts
The "inappproducts" collection of methods.
class
AndroidPublisher.Internalappsharingartifacts
The "internalappsharingartifacts" collection of methods.
class
AndroidPublisher.Monetization
The "monetization" collection of methods.
class
AndroidPublisher.Orders
The "orders" collection of methods.
class
AndroidPublisher.Purchases
The "purchases" collection of methods.
class
AndroidPublisher.Reviews
The "reviews" collection of methods.
class
AndroidPublisher.Systemapks
The "systemapks" collection of methods.
class
AndroidPublisher.Users
The "users" collection of methods.
-
Field Summary
Fields
Modifier and Type
Field and Description
static String
DEFAULT_BASE_URL
The default encoded base URL of the service.
static String
DEFAULT_BATCH_PATH
The default encoded batch path of the service.
static String
DEFAULT_MTLS_ROOT_URL
The default encoded mTLS root URL of the service.
static String
DEFAULT_ROOT_URL
The default encoded root URL of the service.
static String
DEFAULT_SERVICE_PATH
The default encoded service path of the service.
-
Constructor Summary
Constructors
Constructor and Description
AndroidPublisher(com.google.api.client.http.HttpTransport transport,
com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer)
Constructor.
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
AndroidPublisher.Applications
applications()
An accessor for creating requests from the Applications collection.
AndroidPublisher.Apprecovery
apprecovery()
An accessor for creating requests from the Apprecovery collection.
AndroidPublisher.Edits
edits()
An accessor for creating requests from the Edits collection.
AndroidPublisher.Externaltransactions
externaltransactions()
An accessor for creating requests from the Externaltransactions collection.
AndroidPublisher.Generatedapks
generatedapks()
An accessor for creating requests from the Generatedapks collection.
AndroidPublisher.Grants
grants()
An accessor for creating requests from the Grants collection.
AndroidPublisher.Inappproducts
inappproducts()
An accessor for creating requests from the Inappproducts collection.
protected void
initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest<?> httpClientRequest)
AndroidPublisher.Internalappsharingartifacts
internalappsharingartifacts()
An accessor for creating requests from the Internalappsharingartifacts collection.
AndroidPublisher.Monetization
monetization()
An accessor for creating requests from the Monetization collection.
AndroidPublisher.Orders
orders()
An accessor for creating requests from the Orders collection.
AndroidPublisher.Purchases
purchases()
An accessor for creating requests from the Purchases collection.
AndroidPublisher.Reviews
reviews()
An accessor for creating requests from the Reviews collection.
AndroidPublisher.Systemapks
systemapks()
An accessor for creating requests from the Systemapks collection.
AndroidPublisher.Users
users()
An accessor for creating requests from the Users collection.
-
Methods inherited from class com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient
getJsonFactory, getObjectParser
-
-
Field Detail
-
DEFAULT_ROOT_URL
public static final String DEFAULT_ROOT_URL
The default encoded root URL of the service. This is determined when the library is generated
and normally should not be changed.
- Since:
- 1.7
- See Also:
- Constant Field Values
-
DEFAULT_MTLS_ROOT_URL
public static final String DEFAULT_MTLS_ROOT_URL
The default encoded mTLS root URL of the service. This is determined when the library is generated
and normally should not be changed.
- Since:
- 1.31
- See Also:
- Constant Field Values
-
DEFAULT_SERVICE_PATH
public static final String DEFAULT_SERVICE_PATH
The default encoded service path of the service. This is determined when the library is
generated and normally should not be changed.
- Since:
- 1.7
- See Also:
- Constant Field Values
-
DEFAULT_BATCH_PATH
public static final String DEFAULT_BATCH_PATH
The default encoded batch path of the service. This is determined when the library is
generated and normally should not be changed.
- Since:
- 1.23
- See Also:
- Constant Field Values
-
DEFAULT_BASE_URL
public static final String DEFAULT_BASE_URL
The default encoded base URL of the service. This is determined when the library is generated
and normally should not be changed.
- See Also:
- Constant Field Values
-
Constructor Detail
-
AndroidPublisher
public AndroidPublisher(com.google.api.client.http.HttpTransport transport,
com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer)
Constructor.
Use AndroidPublisher.Builder
if you need to specify any of the optional parameters.
- Parameters:
transport
- HTTP transport, which should normally be:
- Google App Engine:
com.google.api.client.extensions.appengine.http.UrlFetchTransport
- Android:
newCompatibleTransport
from
com.google.api.client.extensions.android.http.AndroidHttp
- Java:
com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()
jsonFactory
- JSON factory, which may be:
- Jackson:
com.google.api.client.json.jackson2.JacksonFactory
- Google GSON:
com.google.api.client.json.gson.GsonFactory
- Android Honeycomb or higher:
com.google.api.client.extensions.android.json.AndroidJsonFactory
httpRequestInitializer
- HTTP request initializer or null
for none
- Since:
- 1.7
-
Method Detail
-
initialize
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest<?> httpClientRequest)
throws IOException
- Overrides:
initialize
in class com.google.api.client.googleapis.services.AbstractGoogleClient
- Throws:
IOException
-
applications
public AndroidPublisher.Applications applications()
An accessor for creating requests from the Applications collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Applications.List request = androidpublisher.applications().list(parameters ...)
- Returns:
- the resource collection
-
apprecovery
public AndroidPublisher.Apprecovery apprecovery()
An accessor for creating requests from the Apprecovery collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Apprecovery.List request = androidpublisher.apprecovery().list(parameters ...)
- Returns:
- the resource collection
-
edits
public AndroidPublisher.Edits edits()
An accessor for creating requests from the Edits collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Edits.List request = androidpublisher.edits().list(parameters ...)
- Returns:
- the resource collection
-
externaltransactions
public AndroidPublisher.Externaltransactions externaltransactions()
An accessor for creating requests from the Externaltransactions collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Externaltransactions.List request = androidpublisher.externaltransactions().list(parameters ...)
- Returns:
- the resource collection
-
generatedapks
public AndroidPublisher.Generatedapks generatedapks()
An accessor for creating requests from the Generatedapks collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Generatedapks.List request = androidpublisher.generatedapks().list(parameters ...)
- Returns:
- the resource collection
-
grants
public AndroidPublisher.Grants grants()
An accessor for creating requests from the Grants collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Grants.List request = androidpublisher.grants().list(parameters ...)
- Returns:
- the resource collection
-
inappproducts
public AndroidPublisher.Inappproducts inappproducts()
An accessor for creating requests from the Inappproducts collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Inappproducts.List request = androidpublisher.inappproducts().list(parameters ...)
- Returns:
- the resource collection
-
internalappsharingartifacts
public AndroidPublisher.Internalappsharingartifacts internalappsharingartifacts()
An accessor for creating requests from the Internalappsharingartifacts collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Internalappsharingartifacts.List request = androidpublisher.internalappsharingartifacts().list(parameters ...)
- Returns:
- the resource collection
-
monetization
public AndroidPublisher.Monetization monetization()
An accessor for creating requests from the Monetization collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Monetization.List request = androidpublisher.monetization().list(parameters ...)
- Returns:
- the resource collection
-
orders
public AndroidPublisher.Orders orders()
An accessor for creating requests from the Orders collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Orders.List request = androidpublisher.orders().list(parameters ...)
- Returns:
- the resource collection
-
purchases
public AndroidPublisher.Purchases purchases()
An accessor for creating requests from the Purchases collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Purchases.List request = androidpublisher.purchases().list(parameters ...)
- Returns:
- the resource collection
-
reviews
public AndroidPublisher.Reviews reviews()
An accessor for creating requests from the Reviews collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Reviews.List request = androidpublisher.reviews().list(parameters ...)
- Returns:
- the resource collection
-
systemapks
public AndroidPublisher.Systemapks systemapks()
An accessor for creating requests from the Systemapks collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Systemapks.List request = androidpublisher.systemapks().list(parameters ...)
- Returns:
- the resource collection
-
users
public AndroidPublisher.Users users()
An accessor for creating requests from the Users collection.
The typical use is:
AndroidPublisher androidpublisher = new AndroidPublisher(...);
AndroidPublisher.Users.List request = androidpublisher.users().list(parameters ...)
- Returns:
- the resource collection
Copyright © 2011–2024 Google. All rights reserved.