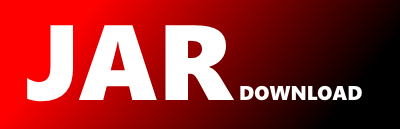
com.google.api.services.appengine.v1.model.StaticFilesHandler Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.appengine.v1.model;
/**
* Files served directly to the user for a given URL, such as images, CSS stylesheets, or JavaScript
* source files. Static file handlers describe which files in the application directory are static
* files, and which URLs serve them.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the App Engine Admin API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class StaticFilesHandler extends com.google.api.client.json.GenericJson {
/**
* Whether files should also be uploaded as code data. By default, files declared in static file
* handlers are uploaded as static data and are only served to end users; they cannot be read by
* the application. If enabled, uploads are charged against both your code and static data storage
* resource quotas.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean applicationReadable;
/**
* Time a static file served by this handler should be cached by web proxies and browsers.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String expiration;
/**
* HTTP headers to use for all responses from these URLs.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map httpHeaders;
/**
* MIME type used to serve all files served by this handler.Defaults to file-specific MIME types,
* which are derived from each file's filename extension.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mimeType;
/**
* Path to the static files matched by the URL pattern, from the application root directory. The
* path can refer to text matched in groupings in the URL pattern.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String path;
/**
* Whether this handler should match the request if the file referenced by the handler does not
* exist.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean requireMatchingFile;
/**
* Regular expression that matches the file paths for all files that should be referenced by this
* handler.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uploadPathRegex;
/**
* Whether files should also be uploaded as code data. By default, files declared in static file
* handlers are uploaded as static data and are only served to end users; they cannot be read by
* the application. If enabled, uploads are charged against both your code and static data storage
* resource quotas.
* @return value or {@code null} for none
*/
public java.lang.Boolean getApplicationReadable() {
return applicationReadable;
}
/**
* Whether files should also be uploaded as code data. By default, files declared in static file
* handlers are uploaded as static data and are only served to end users; they cannot be read by
* the application. If enabled, uploads are charged against both your code and static data storage
* resource quotas.
* @param applicationReadable applicationReadable or {@code null} for none
*/
public StaticFilesHandler setApplicationReadable(java.lang.Boolean applicationReadable) {
this.applicationReadable = applicationReadable;
return this;
}
/**
* Time a static file served by this handler should be cached by web proxies and browsers.
* @return value or {@code null} for none
*/
public String getExpiration() {
return expiration;
}
/**
* Time a static file served by this handler should be cached by web proxies and browsers.
* @param expiration expiration or {@code null} for none
*/
public StaticFilesHandler setExpiration(String expiration) {
this.expiration = expiration;
return this;
}
/**
* HTTP headers to use for all responses from these URLs.
* @return value or {@code null} for none
*/
public java.util.Map getHttpHeaders() {
return httpHeaders;
}
/**
* HTTP headers to use for all responses from these URLs.
* @param httpHeaders httpHeaders or {@code null} for none
*/
public StaticFilesHandler setHttpHeaders(java.util.Map httpHeaders) {
this.httpHeaders = httpHeaders;
return this;
}
/**
* MIME type used to serve all files served by this handler.Defaults to file-specific MIME types,
* which are derived from each file's filename extension.
* @return value or {@code null} for none
*/
public java.lang.String getMimeType() {
return mimeType;
}
/**
* MIME type used to serve all files served by this handler.Defaults to file-specific MIME types,
* which are derived from each file's filename extension.
* @param mimeType mimeType or {@code null} for none
*/
public StaticFilesHandler setMimeType(java.lang.String mimeType) {
this.mimeType = mimeType;
return this;
}
/**
* Path to the static files matched by the URL pattern, from the application root directory. The
* path can refer to text matched in groupings in the URL pattern.
* @return value or {@code null} for none
*/
public java.lang.String getPath() {
return path;
}
/**
* Path to the static files matched by the URL pattern, from the application root directory. The
* path can refer to text matched in groupings in the URL pattern.
* @param path path or {@code null} for none
*/
public StaticFilesHandler setPath(java.lang.String path) {
this.path = path;
return this;
}
/**
* Whether this handler should match the request if the file referenced by the handler does not
* exist.
* @return value or {@code null} for none
*/
public java.lang.Boolean getRequireMatchingFile() {
return requireMatchingFile;
}
/**
* Whether this handler should match the request if the file referenced by the handler does not
* exist.
* @param requireMatchingFile requireMatchingFile or {@code null} for none
*/
public StaticFilesHandler setRequireMatchingFile(java.lang.Boolean requireMatchingFile) {
this.requireMatchingFile = requireMatchingFile;
return this;
}
/**
* Regular expression that matches the file paths for all files that should be referenced by this
* handler.
* @return value or {@code null} for none
*/
public java.lang.String getUploadPathRegex() {
return uploadPathRegex;
}
/**
* Regular expression that matches the file paths for all files that should be referenced by this
* handler.
* @param uploadPathRegex uploadPathRegex or {@code null} for none
*/
public StaticFilesHandler setUploadPathRegex(java.lang.String uploadPathRegex) {
this.uploadPathRegex = uploadPathRegex;
return this;
}
@Override
public StaticFilesHandler set(String fieldName, Object value) {
return (StaticFilesHandler) super.set(fieldName, value);
}
@Override
public StaticFilesHandler clone() {
return (StaticFilesHandler) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy