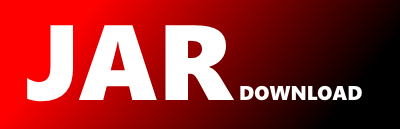
com.google.api.services.appengine.Appengine Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-05-04 17:28:03 UTC)
* on 2018-08-13 at 23:00:04 UTC
* Modify at your own risk.
*/
package com.google.api.services.appengine;
/**
* Service definition for Appengine (v1alpha).
*
*
* The App Engine Admin API enables developers to provision and manage their App Engine applications.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link AppengineRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Appengine extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.24.1 of the App Engine Admin API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://appengine.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Appengine(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Appengine(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Apps collection.
*
* The typical use is:
*
* {@code Appengine appengine = new Appengine(...);}
* {@code Appengine.Apps.List request = appengine.apps().list(parameters ...)}
*
*
* @return the resource collection
*/
public Apps apps() {
return new Apps();
}
/**
* The "apps" collection of methods.
*/
public class Apps {
/**
* An accessor for creating requests from the AuthorizedCertificates collection.
*
* The typical use is:
*
* {@code Appengine appengine = new Appengine(...);}
* {@code Appengine.AuthorizedCertificates.List request = appengine.authorizedCertificates().list(parameters ...)}
*
*
* @return the resource collection
*/
public AuthorizedCertificates authorizedCertificates() {
return new AuthorizedCertificates();
}
/**
* The "authorizedCertificates" collection of methods.
*/
public class AuthorizedCertificates {
/**
* Uploads the specified SSL certificate.
*
* Create a request for the method "authorizedCertificates.create".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @param content the {@link com.google.api.services.appengine.model.AuthorizedCertificate}
* @return the request
*/
public Create create(java.lang.String appsId, com.google.api.services.appengine.model.AuthorizedCertificate content) throws java.io.IOException {
Create result = new Create(appsId, content);
initialize(result);
return result;
}
public class Create extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/authorizedCertificates";
/**
* Uploads the specified SSL certificate.
*
* Create a request for the method "authorizedCertificates.create".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @param content the {@link com.google.api.services.appengine.model.AuthorizedCertificate}
* @since 1.13
*/
protected Create(java.lang.String appsId, com.google.api.services.appengine.model.AuthorizedCertificate content) {
super(Appengine.this, "POST", REST_PATH, content, com.google.api.services.appengine.model.AuthorizedCertificate.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
public Create setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified SSL certificate.
*
* Create a request for the method "authorizedCertificates.delete".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. Name of the resource to delete. Example: apps/myapp/authorizedCertificates/12345.
* @param authorizedCertificatesId Part of `name`. See documentation of `appsId`.
* @return the request
*/
public Delete delete(java.lang.String appsId, java.lang.String authorizedCertificatesId) throws java.io.IOException {
Delete result = new Delete(appsId, authorizedCertificatesId);
initialize(result);
return result;
}
public class Delete extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/authorizedCertificates/{authorizedCertificatesId}";
/**
* Deletes the specified SSL certificate.
*
* Create a request for the method "authorizedCertificates.delete".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. Name of the resource to delete. Example: apps/myapp/authorizedCertificates/12345.
* @param authorizedCertificatesId Part of `name`. See documentation of `appsId`.
* @since 1.13
*/
protected Delete(java.lang.String appsId, java.lang.String authorizedCertificatesId) {
super(Appengine.this, "DELETE", REST_PATH, null, com.google.api.services.appengine.model.Empty.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
this.authorizedCertificatesId = com.google.api.client.util.Preconditions.checkNotNull(authorizedCertificatesId, "Required parameter authorizedCertificatesId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Part of `name`. Name of the resource to delete. Example:
* apps/myapp/authorizedCertificates/12345.
*/
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. Name of the resource to delete. Example: apps/myapp/authorizedCertificates/12345.
*/
public java.lang.String getAppsId() {
return appsId;
}
/**
* Part of `name`. Name of the resource to delete. Example:
* apps/myapp/authorizedCertificates/12345.
*/
public Delete setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Part of `name`. See documentation of `appsId`. */
@com.google.api.client.util.Key
private java.lang.String authorizedCertificatesId;
/** Part of `name`. See documentation of `appsId`.
*/
public java.lang.String getAuthorizedCertificatesId() {
return authorizedCertificatesId;
}
/** Part of `name`. See documentation of `appsId`. */
public Delete setAuthorizedCertificatesId(java.lang.String authorizedCertificatesId) {
this.authorizedCertificatesId = authorizedCertificatesId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the specified SSL certificate.
*
* Create a request for the method "authorizedCertificates.get".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. Name of the resource requested. Example: apps/myapp/authorizedCertificates/12345.
* @param authorizedCertificatesId Part of `name`. See documentation of `appsId`.
* @return the request
*/
public Get get(java.lang.String appsId, java.lang.String authorizedCertificatesId) throws java.io.IOException {
Get result = new Get(appsId, authorizedCertificatesId);
initialize(result);
return result;
}
public class Get extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/authorizedCertificates/{authorizedCertificatesId}";
/**
* Gets the specified SSL certificate.
*
* Create a request for the method "authorizedCertificates.get".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. Name of the resource requested. Example: apps/myapp/authorizedCertificates/12345.
* @param authorizedCertificatesId Part of `name`. See documentation of `appsId`.
* @since 1.13
*/
protected Get(java.lang.String appsId, java.lang.String authorizedCertificatesId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.AuthorizedCertificate.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
this.authorizedCertificatesId = com.google.api.client.util.Preconditions.checkNotNull(authorizedCertificatesId, "Required parameter authorizedCertificatesId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Part of `name`. Name of the resource requested. Example:
* apps/myapp/authorizedCertificates/12345.
*/
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. Name of the resource requested. Example: apps/myapp/authorizedCertificates/12345.
*/
public java.lang.String getAppsId() {
return appsId;
}
/**
* Part of `name`. Name of the resource requested. Example:
* apps/myapp/authorizedCertificates/12345.
*/
public Get setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Part of `name`. See documentation of `appsId`. */
@com.google.api.client.util.Key
private java.lang.String authorizedCertificatesId;
/** Part of `name`. See documentation of `appsId`.
*/
public java.lang.String getAuthorizedCertificatesId() {
return authorizedCertificatesId;
}
/** Part of `name`. See documentation of `appsId`. */
public Get setAuthorizedCertificatesId(java.lang.String authorizedCertificatesId) {
this.authorizedCertificatesId = authorizedCertificatesId;
return this;
}
/** Controls the set of fields returned in the GET response. */
@com.google.api.client.util.Key
private java.lang.String view;
/** Controls the set of fields returned in the GET response.
*/
public java.lang.String getView() {
return view;
}
/** Controls the set of fields returned in the GET response. */
public Get setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all SSL certificates the user is authorized to administer.
*
* Create a request for the method "authorizedCertificates.list".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @return the request
*/
public List list(java.lang.String appsId) throws java.io.IOException {
List result = new List(appsId);
initialize(result);
return result;
}
public class List extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/authorizedCertificates";
/**
* Lists all SSL certificates the user is authorized to administer.
*
* Create a request for the method "authorizedCertificates.list".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @since 1.13
*/
protected List(java.lang.String appsId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.ListAuthorizedCertificatesResponse.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
public List setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Continuation token for fetching the next page of results. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Continuation token for fetching the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Continuation token for fetching the next page of results. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Maximum results to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum results to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum results to return per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Controls the set of fields returned in the LIST response. */
@com.google.api.client.util.Key
private java.lang.String view;
/** Controls the set of fields returned in the LIST response.
*/
public java.lang.String getView() {
return view;
}
/** Controls the set of fields returned in the LIST response. */
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified SSL certificate. To renew a certificate and maintain its existing domain
* mappings, update certificate_data with a new certificate. The new certificate must be applicable
* to the same domains as the original certificate. The certificate display_name may also be
* updated.
*
* Create a request for the method "authorizedCertificates.patch".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. Name of the resource to update. Example: apps/myapp/authorizedCertificates/12345.
* @param authorizedCertificatesId Part of `name`. See documentation of `appsId`.
* @param content the {@link com.google.api.services.appengine.model.AuthorizedCertificate}
* @return the request
*/
public Patch patch(java.lang.String appsId, java.lang.String authorizedCertificatesId, com.google.api.services.appengine.model.AuthorizedCertificate content) throws java.io.IOException {
Patch result = new Patch(appsId, authorizedCertificatesId, content);
initialize(result);
return result;
}
public class Patch extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/authorizedCertificates/{authorizedCertificatesId}";
/**
* Updates the specified SSL certificate. To renew a certificate and maintain its existing domain
* mappings, update certificate_data with a new certificate. The new certificate must be
* applicable to the same domains as the original certificate. The certificate display_name may
* also be updated.
*
* Create a request for the method "authorizedCertificates.patch".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. Name of the resource to update. Example: apps/myapp/authorizedCertificates/12345.
* @param authorizedCertificatesId Part of `name`. See documentation of `appsId`.
* @param content the {@link com.google.api.services.appengine.model.AuthorizedCertificate}
* @since 1.13
*/
protected Patch(java.lang.String appsId, java.lang.String authorizedCertificatesId, com.google.api.services.appengine.model.AuthorizedCertificate content) {
super(Appengine.this, "PATCH", REST_PATH, content, com.google.api.services.appengine.model.AuthorizedCertificate.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
this.authorizedCertificatesId = com.google.api.client.util.Preconditions.checkNotNull(authorizedCertificatesId, "Required parameter authorizedCertificatesId must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Part of `name`. Name of the resource to update. Example:
* apps/myapp/authorizedCertificates/12345.
*/
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. Name of the resource to update. Example: apps/myapp/authorizedCertificates/12345.
*/
public java.lang.String getAppsId() {
return appsId;
}
/**
* Part of `name`. Name of the resource to update. Example:
* apps/myapp/authorizedCertificates/12345.
*/
public Patch setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Part of `name`. See documentation of `appsId`. */
@com.google.api.client.util.Key
private java.lang.String authorizedCertificatesId;
/** Part of `name`. See documentation of `appsId`.
*/
public java.lang.String getAuthorizedCertificatesId() {
return authorizedCertificatesId;
}
/** Part of `name`. See documentation of `appsId`. */
public Patch setAuthorizedCertificatesId(java.lang.String authorizedCertificatesId) {
this.authorizedCertificatesId = authorizedCertificatesId;
return this;
}
/**
* Standard field mask for the set of fields to be updated. Updates are only supported on
* the certificate_raw_data and display_name fields.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Standard field mask for the set of fields to be updated. Updates are only supported on the
certificate_raw_data and display_name fields.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Standard field mask for the set of fields to be updated. Updates are only supported on
* the certificate_raw_data and display_name fields.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the AuthorizedDomains collection.
*
* The typical use is:
*
* {@code Appengine appengine = new Appengine(...);}
* {@code Appengine.AuthorizedDomains.List request = appengine.authorizedDomains().list(parameters ...)}
*
*
* @return the resource collection
*/
public AuthorizedDomains authorizedDomains() {
return new AuthorizedDomains();
}
/**
* The "authorizedDomains" collection of methods.
*/
public class AuthorizedDomains {
/**
* Lists all domains the user is authorized to administer.
*
* Create a request for the method "authorizedDomains.list".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @return the request
*/
public List list(java.lang.String appsId) throws java.io.IOException {
List result = new List(appsId);
initialize(result);
return result;
}
public class List extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/authorizedDomains";
/**
* Lists all domains the user is authorized to administer.
*
* Create a request for the method "authorizedDomains.list".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @since 1.13
*/
protected List(java.lang.String appsId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.ListAuthorizedDomainsResponse.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
public List setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Continuation token for fetching the next page of results. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Continuation token for fetching the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Continuation token for fetching the next page of results. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Maximum results to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum results to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum results to return per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the DomainMappings collection.
*
* The typical use is:
*
* {@code Appengine appengine = new Appengine(...);}
* {@code Appengine.DomainMappings.List request = appengine.domainMappings().list(parameters ...)}
*
*
* @return the resource collection
*/
public DomainMappings domainMappings() {
return new DomainMappings();
}
/**
* The "domainMappings" collection of methods.
*/
public class DomainMappings {
/**
* Maps a domain to an application. A user must be authorized to administer a domain in order to map
* it to an application. For a list of available authorized domains, see
* AuthorizedDomains.ListAuthorizedDomains.
*
* Create a request for the method "domainMappings.create".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @param content the {@link com.google.api.services.appengine.model.DomainMapping}
* @return the request
*/
public Create create(java.lang.String appsId, com.google.api.services.appengine.model.DomainMapping content) throws java.io.IOException {
Create result = new Create(appsId, content);
initialize(result);
return result;
}
public class Create extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/domainMappings";
/**
* Maps a domain to an application. A user must be authorized to administer a domain in order to
* map it to an application. For a list of available authorized domains, see
* AuthorizedDomains.ListAuthorizedDomains.
*
* Create a request for the method "domainMappings.create".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @param content the {@link com.google.api.services.appengine.model.DomainMapping}
* @since 1.13
*/
protected Create(java.lang.String appsId, com.google.api.services.appengine.model.DomainMapping content) {
super(Appengine.this, "POST", REST_PATH, content, com.google.api.services.appengine.model.Operation.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
public Create setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/**
* Whether the domain creation should override any existing mappings for this domain. By
* default, overrides are rejected.
*/
@com.google.api.client.util.Key
private java.lang.String overrideStrategy;
/** Whether the domain creation should override any existing mappings for this domain. By default,
overrides are rejected.
*/
public java.lang.String getOverrideStrategy() {
return overrideStrategy;
}
/**
* Whether the domain creation should override any existing mappings for this domain. By
* default, overrides are rejected.
*/
public Create setOverrideStrategy(java.lang.String overrideStrategy) {
this.overrideStrategy = overrideStrategy;
return this;
}
/**
* Whether a managed certificate should be provided by App Engine. If true, a certificate ID
* must be manaually set in the DomainMapping resource to configure SSL for this domain. If
* false, a managed certificate will be provisioned and a certificate ID will be
* automatically populated.
*/
@com.google.api.client.util.Key
private java.lang.Boolean noManagedCertificate;
/** Whether a managed certificate should be provided by App Engine. If true, a certificate ID must be
manaually set in the DomainMapping resource to configure SSL for this domain. If false, a managed
certificate will be provisioned and a certificate ID will be automatically populated.
*/
public java.lang.Boolean getNoManagedCertificate() {
return noManagedCertificate;
}
/**
* Whether a managed certificate should be provided by App Engine. If true, a certificate ID
* must be manaually set in the DomainMapping resource to configure SSL for this domain. If
* false, a managed certificate will be provisioned and a certificate ID will be
* automatically populated.
*/
public Create setNoManagedCertificate(java.lang.Boolean noManagedCertificate) {
this.noManagedCertificate = noManagedCertificate;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified domain mapping. A user must be authorized to administer the associated
* domain in order to delete a DomainMapping resource.
*
* Create a request for the method "domainMappings.delete".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. Name of the resource to delete. Example: apps/myapp/domainMappings/example.com.
* @param domainMappingsId Part of `name`. See documentation of `appsId`.
* @return the request
*/
public Delete delete(java.lang.String appsId, java.lang.String domainMappingsId) throws java.io.IOException {
Delete result = new Delete(appsId, domainMappingsId);
initialize(result);
return result;
}
public class Delete extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/domainMappings/{domainMappingsId}";
/**
* Deletes the specified domain mapping. A user must be authorized to administer the associated
* domain in order to delete a DomainMapping resource.
*
* Create a request for the method "domainMappings.delete".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. Name of the resource to delete. Example: apps/myapp/domainMappings/example.com.
* @param domainMappingsId Part of `name`. See documentation of `appsId`.
* @since 1.13
*/
protected Delete(java.lang.String appsId, java.lang.String domainMappingsId) {
super(Appengine.this, "DELETE", REST_PATH, null, com.google.api.services.appengine.model.Operation.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
this.domainMappingsId = com.google.api.client.util.Preconditions.checkNotNull(domainMappingsId, "Required parameter domainMappingsId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Part of `name`. Name of the resource to delete. Example:
* apps/myapp/domainMappings/example.com.
*/
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. Name of the resource to delete. Example: apps/myapp/domainMappings/example.com.
*/
public java.lang.String getAppsId() {
return appsId;
}
/**
* Part of `name`. Name of the resource to delete. Example:
* apps/myapp/domainMappings/example.com.
*/
public Delete setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Part of `name`. See documentation of `appsId`. */
@com.google.api.client.util.Key
private java.lang.String domainMappingsId;
/** Part of `name`. See documentation of `appsId`.
*/
public java.lang.String getDomainMappingsId() {
return domainMappingsId;
}
/** Part of `name`. See documentation of `appsId`. */
public Delete setDomainMappingsId(java.lang.String domainMappingsId) {
this.domainMappingsId = domainMappingsId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the specified domain mapping.
*
* Create a request for the method "domainMappings.get".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. Name of the resource requested. Example: apps/myapp/domainMappings/example.com.
* @param domainMappingsId Part of `name`. See documentation of `appsId`.
* @return the request
*/
public Get get(java.lang.String appsId, java.lang.String domainMappingsId) throws java.io.IOException {
Get result = new Get(appsId, domainMappingsId);
initialize(result);
return result;
}
public class Get extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/domainMappings/{domainMappingsId}";
/**
* Gets the specified domain mapping.
*
* Create a request for the method "domainMappings.get".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. Name of the resource requested. Example: apps/myapp/domainMappings/example.com.
* @param domainMappingsId Part of `name`. See documentation of `appsId`.
* @since 1.13
*/
protected Get(java.lang.String appsId, java.lang.String domainMappingsId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.DomainMapping.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
this.domainMappingsId = com.google.api.client.util.Preconditions.checkNotNull(domainMappingsId, "Required parameter domainMappingsId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Part of `name`. Name of the resource requested. Example:
* apps/myapp/domainMappings/example.com.
*/
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. Name of the resource requested. Example: apps/myapp/domainMappings/example.com.
*/
public java.lang.String getAppsId() {
return appsId;
}
/**
* Part of `name`. Name of the resource requested. Example:
* apps/myapp/domainMappings/example.com.
*/
public Get setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Part of `name`. See documentation of `appsId`. */
@com.google.api.client.util.Key
private java.lang.String domainMappingsId;
/** Part of `name`. See documentation of `appsId`.
*/
public java.lang.String getDomainMappingsId() {
return domainMappingsId;
}
/** Part of `name`. See documentation of `appsId`. */
public Get setDomainMappingsId(java.lang.String domainMappingsId) {
this.domainMappingsId = domainMappingsId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the domain mappings on an application.
*
* Create a request for the method "domainMappings.list".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @return the request
*/
public List list(java.lang.String appsId) throws java.io.IOException {
List result = new List(appsId);
initialize(result);
return result;
}
public class List extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/domainMappings";
/**
* Lists the domain mappings on an application.
*
* Create a request for the method "domainMappings.list".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
* @since 1.13
*/
protected List(java.lang.String appsId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.ListDomainMappingsResponse.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `parent`. Name of the parent Application resource. Example: apps/myapp. */
public List setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Continuation token for fetching the next page of results. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Continuation token for fetching the next page of results.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Continuation token for fetching the next page of results. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Maximum results to return per page. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Maximum results to return per page.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** Maximum results to return per page. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified domain mapping. To map an SSL certificate to a domain mapping, update
* certificate_id to point to an AuthorizedCertificate resource. A user must be authorized to
* administer the associated domain in order to update a DomainMapping resource.
*
* Create a request for the method "domainMappings.patch".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. Name of the resource to update. Example: apps/myapp/domainMappings/example.com.
* @param domainMappingsId Part of `name`. See documentation of `appsId`.
* @param content the {@link com.google.api.services.appengine.model.DomainMapping}
* @return the request
*/
public Patch patch(java.lang.String appsId, java.lang.String domainMappingsId, com.google.api.services.appengine.model.DomainMapping content) throws java.io.IOException {
Patch result = new Patch(appsId, domainMappingsId, content);
initialize(result);
return result;
}
public class Patch extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/domainMappings/{domainMappingsId}";
/**
* Updates the specified domain mapping. To map an SSL certificate to a domain mapping, update
* certificate_id to point to an AuthorizedCertificate resource. A user must be authorized to
* administer the associated domain in order to update a DomainMapping resource.
*
* Create a request for the method "domainMappings.patch".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. Name of the resource to update. Example: apps/myapp/domainMappings/example.com.
* @param domainMappingsId Part of `name`. See documentation of `appsId`.
* @param content the {@link com.google.api.services.appengine.model.DomainMapping}
* @since 1.13
*/
protected Patch(java.lang.String appsId, java.lang.String domainMappingsId, com.google.api.services.appengine.model.DomainMapping content) {
super(Appengine.this, "PATCH", REST_PATH, content, com.google.api.services.appengine.model.Operation.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
this.domainMappingsId = com.google.api.client.util.Preconditions.checkNotNull(domainMappingsId, "Required parameter domainMappingsId must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Part of `name`. Name of the resource to update. Example:
* apps/myapp/domainMappings/example.com.
*/
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. Name of the resource to update. Example: apps/myapp/domainMappings/example.com.
*/
public java.lang.String getAppsId() {
return appsId;
}
/**
* Part of `name`. Name of the resource to update. Example:
* apps/myapp/domainMappings/example.com.
*/
public Patch setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Part of `name`. See documentation of `appsId`. */
@com.google.api.client.util.Key
private java.lang.String domainMappingsId;
/** Part of `name`. See documentation of `appsId`.
*/
public java.lang.String getDomainMappingsId() {
return domainMappingsId;
}
/** Part of `name`. See documentation of `appsId`. */
public Patch setDomainMappingsId(java.lang.String domainMappingsId) {
this.domainMappingsId = domainMappingsId;
return this;
}
/** Standard field mask for the set of fields to be updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Standard field mask for the set of fields to be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Standard field mask for the set of fields to be updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
/**
* Whether a managed certificate should be provided by App Engine. If true, a certificate ID
* must be manually set in the DomainMapping resource to configure SSL for this domain. If
* false, a managed certificate will be provisioned and a certificate ID will be
* automatically populated. Only applicable if ssl_settings.certificate_id is specified in
* the update mask.
*/
@com.google.api.client.util.Key
private java.lang.Boolean noManagedCertificate;
/** Whether a managed certificate should be provided by App Engine. If true, a certificate ID must be
manually set in the DomainMapping resource to configure SSL for this domain. If false, a managed
certificate will be provisioned and a certificate ID will be automatically populated. Only
applicable if ssl_settings.certificate_id is specified in the update mask.
*/
public java.lang.Boolean getNoManagedCertificate() {
return noManagedCertificate;
}
/**
* Whether a managed certificate should be provided by App Engine. If true, a certificate ID
* must be manually set in the DomainMapping resource to configure SSL for this domain. If
* false, a managed certificate will be provisioned and a certificate ID will be
* automatically populated. Only applicable if ssl_settings.certificate_id is specified in
* the update mask.
*/
public Patch setNoManagedCertificate(java.lang.Boolean noManagedCertificate) {
this.noManagedCertificate = noManagedCertificate;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Appengine appengine = new Appengine(...);}
* {@code Appengine.Locations.List request = appengine.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. Resource name for the location.
* @param locationsId Part of `name`. See documentation of `appsId`.
* @return the request
*/
public Get get(java.lang.String appsId, java.lang.String locationsId) throws java.io.IOException {
Get result = new Get(appsId, locationsId);
initialize(result);
return result;
}
public class Get extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/locations/{locationsId}";
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. Resource name for the location.
* @param locationsId Part of `name`. See documentation of `appsId`.
* @since 1.13
*/
protected Get(java.lang.String appsId, java.lang.String locationsId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.Location.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
this.locationsId = com.google.api.client.util.Preconditions.checkNotNull(locationsId, "Required parameter locationsId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Part of `name`. Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. Resource name for the location.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `name`. Resource name for the location. */
public Get setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Part of `name`. See documentation of `appsId`. */
@com.google.api.client.util.Key
private java.lang.String locationsId;
/** Part of `name`. See documentation of `appsId`.
*/
public java.lang.String getLocationsId() {
return locationsId;
}
/** Part of `name`. See documentation of `appsId`. */
public Get setLocationsId(java.lang.String locationsId) {
this.locationsId = locationsId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String appsId) throws java.io.IOException {
List result = new List(appsId);
initialize(result);
return result;
}
public class List extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/locations";
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String appsId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.ListLocationsResponse.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Part of `name`. The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. The resource that owns the locations collection, if applicable.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `name`. The resource that owns the locations collection, if applicable. */
public List setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Appengine appengine = new Appengine(...);}
* {@code Appengine.Operations.List request = appengine.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. The name of the operation resource.
* @param operationsId Part of `name`. See documentation of `appsId`.
* @return the request
*/
public Get get(java.lang.String appsId, java.lang.String operationsId) throws java.io.IOException {
Get result = new Get(appsId, operationsId);
initialize(result);
return result;
}
public class Get extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/operations/{operationsId}";
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. The name of the operation resource.
* @param operationsId Part of `name`. See documentation of `appsId`.
* @since 1.13
*/
protected Get(java.lang.String appsId, java.lang.String operationsId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.Operation.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
this.operationsId = com.google.api.client.util.Preconditions.checkNotNull(operationsId, "Required parameter operationsId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Part of `name`. The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. The name of the operation resource.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `name`. The name of the operation resource. */
public Get setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** Part of `name`. See documentation of `appsId`. */
@com.google.api.client.util.Key
private java.lang.String operationsId;
/** Part of `name`. See documentation of `appsId`.
*/
public java.lang.String getOperationsId() {
return operationsId;
}
/** Part of `name`. See documentation of `appsId`. */
public Get setOperationsId(java.lang.String operationsId) {
this.operationsId = operationsId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.NOTE: the name binding allows API services to override the
* binding to use different resource name schemes, such as users/operations. To override the
* binding, API services can add a binding such as "/v1/{name=users}/operations" to their service
* configuration. For backwards compatibility, the default name includes the operations collection
* id, however overriding users must ensure the name binding is the parent resource, without the
* operations collection id.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the appengine server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param appsId Part of `name`. The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String appsId) throws java.io.IOException {
List result = new List(appsId);
initialize(result);
return result;
}
public class List extends AppengineRequest {
private static final String REST_PATH = "v1alpha/apps/{appsId}/operations";
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns UNIMPLEMENTED.NOTE: the name binding allows API services to override
* the binding to use different resource name schemes, such as users/operations. To override the
* binding, API services can add a binding such as "/v1/{name=users}/operations" to their service
* configuration. For backwards compatibility, the default name includes the operations collection
* id, however overriding users must ensure the name binding is the parent resource, without the
* operations collection id.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the appengine server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param appsId Part of `name`. The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String appsId) {
super(Appengine.this, "GET", REST_PATH, null, com.google.api.services.appengine.model.ListOperationsResponse.class);
this.appsId = com.google.api.client.util.Preconditions.checkNotNull(appsId, "Required parameter appsId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Part of `name`. The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String appsId;
/** Part of `name`. The name of the operation's parent resource.
*/
public java.lang.String getAppsId() {
return appsId;
}
/** Part of `name`. The name of the operation's parent resource. */
public List setAppsId(java.lang.String appsId) {
this.appsId = appsId;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link Appengine}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Appengine}. */
@Override
public Appengine build() {
return new Appengine(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link AppengineRequestInitializer}.
*
* @since 1.12
*/
public Builder setAppengineRequestInitializer(
AppengineRequestInitializer appengineRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(appengineRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}