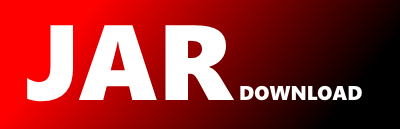
com.google.api.services.appengine.model.Application Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2017-11-07 19:12:12 UTC)
* on 2017-12-15 at 01:51:25 UTC
* Modify at your own risk.
*/
package com.google.api.services.appengine.model;
/**
* An Application resource contains the top-level configuration of an App Engine application.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google App Engine Admin API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Application extends com.google.api.client.json.GenericJson {
/**
* Google Apps authentication domain that controls which users can access this
* application.Defaults to open access for any Google Account.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String authDomain;
/**
* Google Cloud Storage bucket that can be used for storing files associated with this
* application. This bucket is associated with the application and can be used by the gcloud
* deployment commands.@OutputOnly
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String codeBucket;
/**
* Google Cloud Storage bucket that can be used by this application to store content.@OutputOnly
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultBucket;
/**
* Cookie expiration policy for this application.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String defaultCookieExpiration;
/**
* Hostname used to reach this application, as resolved by App Engine.@OutputOnly
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultHostname;
/**
* HTTP path dispatch rules for requests to the application that do not explicitly target a
* service or version. Rules are order-dependent. Up to 20 dispatch rules can be
* supported.@OutputOnly
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List dispatchRules;
static {
// hack to force ProGuard to consider UrlDispatchRule used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(UrlDispatchRule.class);
}
/**
* The feature specific settings to be used in the application.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FeatureSettings featureSettings;
/**
* The Google Container Registry domain used for storing managed build docker images for this
* application.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gcrDomain;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private IdentityAwareProxy iap;
/**
* Identifier of the Application resource. This identifier is equivalent to the project ID of the
* Google Cloud Platform project where you want to deploy your application. Example: myapp.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* Location from which this application will be run. Application instances will run out of data
* centers in the chosen location, which is also where all of the application's end user content
* is stored.Defaults to us-central.Options are:us-central - Central USeurope-west - Western
* Europeus-east1 - Eastern US
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String locationId;
/**
* Full path to the Application resource in the API. Example: apps/myapp.@OutputOnly
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Serving status of this application.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String servingStatus;
/**
* Google Apps authentication domain that controls which users can access this
* application.Defaults to open access for any Google Account.
* @return value or {@code null} for none
*/
public java.lang.String getAuthDomain() {
return authDomain;
}
/**
* Google Apps authentication domain that controls which users can access this
* application.Defaults to open access for any Google Account.
* @param authDomain authDomain or {@code null} for none
*/
public Application setAuthDomain(java.lang.String authDomain) {
this.authDomain = authDomain;
return this;
}
/**
* Google Cloud Storage bucket that can be used for storing files associated with this
* application. This bucket is associated with the application and can be used by the gcloud
* deployment commands.@OutputOnly
* @return value or {@code null} for none
*/
public java.lang.String getCodeBucket() {
return codeBucket;
}
/**
* Google Cloud Storage bucket that can be used for storing files associated with this
* application. This bucket is associated with the application and can be used by the gcloud
* deployment commands.@OutputOnly
* @param codeBucket codeBucket or {@code null} for none
*/
public Application setCodeBucket(java.lang.String codeBucket) {
this.codeBucket = codeBucket;
return this;
}
/**
* Google Cloud Storage bucket that can be used by this application to store content.@OutputOnly
* @return value or {@code null} for none
*/
public java.lang.String getDefaultBucket() {
return defaultBucket;
}
/**
* Google Cloud Storage bucket that can be used by this application to store content.@OutputOnly
* @param defaultBucket defaultBucket or {@code null} for none
*/
public Application setDefaultBucket(java.lang.String defaultBucket) {
this.defaultBucket = defaultBucket;
return this;
}
/**
* Cookie expiration policy for this application.
* @return value or {@code null} for none
*/
public String getDefaultCookieExpiration() {
return defaultCookieExpiration;
}
/**
* Cookie expiration policy for this application.
* @param defaultCookieExpiration defaultCookieExpiration or {@code null} for none
*/
public Application setDefaultCookieExpiration(String defaultCookieExpiration) {
this.defaultCookieExpiration = defaultCookieExpiration;
return this;
}
/**
* Hostname used to reach this application, as resolved by App Engine.@OutputOnly
* @return value or {@code null} for none
*/
public java.lang.String getDefaultHostname() {
return defaultHostname;
}
/**
* Hostname used to reach this application, as resolved by App Engine.@OutputOnly
* @param defaultHostname defaultHostname or {@code null} for none
*/
public Application setDefaultHostname(java.lang.String defaultHostname) {
this.defaultHostname = defaultHostname;
return this;
}
/**
* HTTP path dispatch rules for requests to the application that do not explicitly target a
* service or version. Rules are order-dependent. Up to 20 dispatch rules can be
* supported.@OutputOnly
* @return value or {@code null} for none
*/
public java.util.List getDispatchRules() {
return dispatchRules;
}
/**
* HTTP path dispatch rules for requests to the application that do not explicitly target a
* service or version. Rules are order-dependent. Up to 20 dispatch rules can be
* supported.@OutputOnly
* @param dispatchRules dispatchRules or {@code null} for none
*/
public Application setDispatchRules(java.util.List dispatchRules) {
this.dispatchRules = dispatchRules;
return this;
}
/**
* The feature specific settings to be used in the application.
* @return value or {@code null} for none
*/
public FeatureSettings getFeatureSettings() {
return featureSettings;
}
/**
* The feature specific settings to be used in the application.
* @param featureSettings featureSettings or {@code null} for none
*/
public Application setFeatureSettings(FeatureSettings featureSettings) {
this.featureSettings = featureSettings;
return this;
}
/**
* The Google Container Registry domain used for storing managed build docker images for this
* application.
* @return value or {@code null} for none
*/
public java.lang.String getGcrDomain() {
return gcrDomain;
}
/**
* The Google Container Registry domain used for storing managed build docker images for this
* application.
* @param gcrDomain gcrDomain or {@code null} for none
*/
public Application setGcrDomain(java.lang.String gcrDomain) {
this.gcrDomain = gcrDomain;
return this;
}
/**
* @return value or {@code null} for none
*/
public IdentityAwareProxy getIap() {
return iap;
}
/**
* @param iap iap or {@code null} for none
*/
public Application setIap(IdentityAwareProxy iap) {
this.iap = iap;
return this;
}
/**
* Identifier of the Application resource. This identifier is equivalent to the project ID of the
* Google Cloud Platform project where you want to deploy your application. Example: myapp.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* Identifier of the Application resource. This identifier is equivalent to the project ID of the
* Google Cloud Platform project where you want to deploy your application. Example: myapp.
* @param id id or {@code null} for none
*/
public Application setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Location from which this application will be run. Application instances will run out of data
* centers in the chosen location, which is also where all of the application's end user content
* is stored.Defaults to us-central.Options are:us-central - Central USeurope-west - Western
* Europeus-east1 - Eastern US
* @return value or {@code null} for none
*/
public java.lang.String getLocationId() {
return locationId;
}
/**
* Location from which this application will be run. Application instances will run out of data
* centers in the chosen location, which is also where all of the application's end user content
* is stored.Defaults to us-central.Options are:us-central - Central USeurope-west - Western
* Europeus-east1 - Eastern US
* @param locationId locationId or {@code null} for none
*/
public Application setLocationId(java.lang.String locationId) {
this.locationId = locationId;
return this;
}
/**
* Full path to the Application resource in the API. Example: apps/myapp.@OutputOnly
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Full path to the Application resource in the API. Example: apps/myapp.@OutputOnly
* @param name name or {@code null} for none
*/
public Application setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Serving status of this application.
* @return value or {@code null} for none
*/
public java.lang.String getServingStatus() {
return servingStatus;
}
/**
* Serving status of this application.
* @param servingStatus servingStatus or {@code null} for none
*/
public Application setServingStatus(java.lang.String servingStatus) {
this.servingStatus = servingStatus;
return this;
}
@Override
public Application set(String fieldName, Object value) {
return (Application) super.set(fieldName, value);
}
@Override
public Application clone() {
return (Application) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy