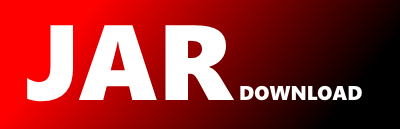
com.google.api.services.artifactregistry.v1.model.RemoteRepositoryConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.artifactregistry.v1.model;
/**
* Remote repository configuration.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Artifact Registry API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class RemoteRepositoryConfig extends com.google.api.client.json.GenericJson {
/**
* Specific settings for an Apt remote repository.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AptRepository aptRepository;
/**
* Common remote repository settings. Used as the remote repository upstream URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CommonRemoteRepository commonRepository;
/**
* The description of the remote source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Input only. A create/update remote repo option to avoid making a HEAD/GET request to validate a
* remote repo and any supplied upstream credentials.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean disableUpstreamValidation;
/**
* Specific settings for a Docker remote repository.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DockerRepository dockerRepository;
/**
* Specific settings for a Maven remote repository.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MavenRepository mavenRepository;
/**
* Specific settings for an Npm remote repository.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NpmRepository npmRepository;
/**
* Specific settings for a Python remote repository.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private PythonRepository pythonRepository;
/**
* Optional. The credentials used to access the remote repository.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private UpstreamCredentials upstreamCredentials;
/**
* Specific settings for a Yum remote repository.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private YumRepository yumRepository;
/**
* Specific settings for an Apt remote repository.
* @return value or {@code null} for none
*/
public AptRepository getAptRepository() {
return aptRepository;
}
/**
* Specific settings for an Apt remote repository.
* @param aptRepository aptRepository or {@code null} for none
*/
public RemoteRepositoryConfig setAptRepository(AptRepository aptRepository) {
this.aptRepository = aptRepository;
return this;
}
/**
* Common remote repository settings. Used as the remote repository upstream URL.
* @return value or {@code null} for none
*/
public CommonRemoteRepository getCommonRepository() {
return commonRepository;
}
/**
* Common remote repository settings. Used as the remote repository upstream URL.
* @param commonRepository commonRepository or {@code null} for none
*/
public RemoteRepositoryConfig setCommonRepository(CommonRemoteRepository commonRepository) {
this.commonRepository = commonRepository;
return this;
}
/**
* The description of the remote source.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* The description of the remote source.
* @param description description or {@code null} for none
*/
public RemoteRepositoryConfig setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Input only. A create/update remote repo option to avoid making a HEAD/GET request to validate a
* remote repo and any supplied upstream credentials.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDisableUpstreamValidation() {
return disableUpstreamValidation;
}
/**
* Input only. A create/update remote repo option to avoid making a HEAD/GET request to validate a
* remote repo and any supplied upstream credentials.
* @param disableUpstreamValidation disableUpstreamValidation or {@code null} for none
*/
public RemoteRepositoryConfig setDisableUpstreamValidation(java.lang.Boolean disableUpstreamValidation) {
this.disableUpstreamValidation = disableUpstreamValidation;
return this;
}
/**
* Specific settings for a Docker remote repository.
* @return value or {@code null} for none
*/
public DockerRepository getDockerRepository() {
return dockerRepository;
}
/**
* Specific settings for a Docker remote repository.
* @param dockerRepository dockerRepository or {@code null} for none
*/
public RemoteRepositoryConfig setDockerRepository(DockerRepository dockerRepository) {
this.dockerRepository = dockerRepository;
return this;
}
/**
* Specific settings for a Maven remote repository.
* @return value or {@code null} for none
*/
public MavenRepository getMavenRepository() {
return mavenRepository;
}
/**
* Specific settings for a Maven remote repository.
* @param mavenRepository mavenRepository or {@code null} for none
*/
public RemoteRepositoryConfig setMavenRepository(MavenRepository mavenRepository) {
this.mavenRepository = mavenRepository;
return this;
}
/**
* Specific settings for an Npm remote repository.
* @return value or {@code null} for none
*/
public NpmRepository getNpmRepository() {
return npmRepository;
}
/**
* Specific settings for an Npm remote repository.
* @param npmRepository npmRepository or {@code null} for none
*/
public RemoteRepositoryConfig setNpmRepository(NpmRepository npmRepository) {
this.npmRepository = npmRepository;
return this;
}
/**
* Specific settings for a Python remote repository.
* @return value or {@code null} for none
*/
public PythonRepository getPythonRepository() {
return pythonRepository;
}
/**
* Specific settings for a Python remote repository.
* @param pythonRepository pythonRepository or {@code null} for none
*/
public RemoteRepositoryConfig setPythonRepository(PythonRepository pythonRepository) {
this.pythonRepository = pythonRepository;
return this;
}
/**
* Optional. The credentials used to access the remote repository.
* @return value or {@code null} for none
*/
public UpstreamCredentials getUpstreamCredentials() {
return upstreamCredentials;
}
/**
* Optional. The credentials used to access the remote repository.
* @param upstreamCredentials upstreamCredentials or {@code null} for none
*/
public RemoteRepositoryConfig setUpstreamCredentials(UpstreamCredentials upstreamCredentials) {
this.upstreamCredentials = upstreamCredentials;
return this;
}
/**
* Specific settings for a Yum remote repository.
* @return value or {@code null} for none
*/
public YumRepository getYumRepository() {
return yumRepository;
}
/**
* Specific settings for a Yum remote repository.
* @param yumRepository yumRepository or {@code null} for none
*/
public RemoteRepositoryConfig setYumRepository(YumRepository yumRepository) {
this.yumRepository = yumRepository;
return this;
}
@Override
public RemoteRepositoryConfig set(String fieldName, Object value) {
return (RemoteRepositoryConfig) super.set(fieldName, value);
}
@Override
public RemoteRepositoryConfig clone() {
return (RemoteRepositoryConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy