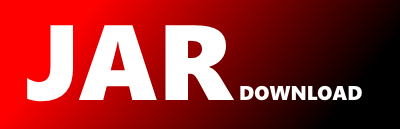
com.google.api.services.backupdr.v1.Backupdr Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1;
/**
* Service definition for Backupdr (v1).
*
*
*
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link BackupdrRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Backupdr extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Backup and DR Service API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://backupdr.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://backupdr.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Backupdr(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Backupdr(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.Projects.List request = backupdr.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.Locations.List request = backupdr.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.Location.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
`"displayName=tokyo"`, and is documented in more detail in [AIP-160](https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the `next_page_token` field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the BackupPlanAssociations collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.BackupPlanAssociations.List request = backupdr.backupPlanAssociations().list(parameters ...)}
*
*
* @return the resource collection
*/
public BackupPlanAssociations backupPlanAssociations() {
return new BackupPlanAssociations();
}
/**
* The "backupPlanAssociations" collection of methods.
*/
public class BackupPlanAssociations {
/**
* Create a BackupPlanAssociation
*
* Create a request for the method "backupPlanAssociations.create".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The backup plan association project and location in the format
* `projects/{project_id}/locations/{location}`. In Cloud BackupDR locations map to GCP
* regions, for example **us-central1**.
* @param content the {@link com.google.api.services.backupdr.v1.model.BackupPlanAssociation}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.backupdr.v1.model.BackupPlanAssociation content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/backupPlanAssociations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Create a BackupPlanAssociation
*
* Create a request for the method "backupPlanAssociations.create".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The backup plan association project and location in the format
* `projects/{project_id}/locations/{location}`. In Cloud BackupDR locations map to GCP
* regions, for example **us-central1**.
* @param content the {@link com.google.api.services.backupdr.v1.model.BackupPlanAssociation}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.backupdr.v1.model.BackupPlanAssociation content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The backup plan association project and location in the format
* `projects/{project_id}/locations/{location}`. In Cloud BackupDR locations map to GCP
* regions, for example **us-central1**.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The backup plan association project and location in the format
`projects/{project_id}/locations/{location}`. In Cloud BackupDR locations map to GCP regions, for
example **us-central1**.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The backup plan association project and location in the format
* `projects/{project_id}/locations/{location}`. In Cloud BackupDR locations map to GCP
* regions, for example **us-central1**.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The name of the backup plan association to create. The name must be unique
* for the specified project and location.
*/
@com.google.api.client.util.Key
private java.lang.String backupPlanAssociationId;
/** Required. The name of the backup plan association to create. The name must be unique for the
specified project and location.
*/
public java.lang.String getBackupPlanAssociationId() {
return backupPlanAssociationId;
}
/**
* Required. The name of the backup plan association to create. The name must be unique
* for the specified project and location.
*/
public Create setBackupPlanAssociationId(java.lang.String backupPlanAssociationId) {
this.backupPlanAssociationId = backupPlanAssociationId;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and t he request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes since the first request. For
example, consider a situation where you make an initial request and t he request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and t he request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single BackupPlanAssociation.
*
* Create a request for the method "backupPlanAssociations.delete".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the backup plan association resource, in the format
* `projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
/**
* Deletes a single BackupPlanAssociation.
*
* Create a request for the method "backupPlanAssociations.delete".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the backup plan association resource, in the format
* `projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Backupdr.this, "DELETE", REST_PATH, null, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the backup plan association resource, in the format `projects/{projec
* t}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the backup plan association resource, in the format
`projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the backup plan association resource, in the format `projects/{projec
* t}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* after the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes after the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* after the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single BackupPlanAssociation.
*
* Create a request for the method "backupPlanAssociations.get".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the backup plan association resource, in the format
* `projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
/**
* Gets details of a single BackupPlanAssociation.
*
* Create a request for the method "backupPlanAssociations.get".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the backup plan association resource, in the format
* `projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.BackupPlanAssociation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the backup plan association resource, in the format `projects/{projec
* t}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the backup plan association resource, in the format
`projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the backup plan association resource, in the format `projects/{projec
* t}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists BackupPlanAssociations in a given project and location.
*
* Create a request for the method "backupPlanAssociations.list".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The project and location for which to retrieve backup Plan Associations information, in
* the format `projects/{project_id}/locations/{location}`. In Cloud BackupDR, locations map
* to GCP regions, for example **us-central1**. To retrieve backup plan associations for all
* locations, use "-" for the `{location}` value.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/backupPlanAssociations";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists BackupPlanAssociations in a given project and location.
*
* Create a request for the method "backupPlanAssociations.list".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project and location for which to retrieve backup Plan Associations information, in
* the format `projects/{project_id}/locations/{location}`. In Cloud BackupDR, locations map
* to GCP regions, for example **us-central1**. To retrieve backup plan associations for all
* locations, use "-" for the `{location}` value.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ListBackupPlanAssociationsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project and location for which to retrieve backup Plan Associations
* information, in the format `projects/{project_id}/locations/{location}`. In Cloud
* BackupDR, locations map to GCP regions, for example **us-central1**. To retrieve backup
* plan associations for all locations, use "-" for the `{location}` value.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project and location for which to retrieve backup Plan Associations information, in
the format `projects/{project_id}/locations/{location}`. In Cloud BackupDR, locations map to GCP
regions, for example **us-central1**. To retrieve backup plan associations for all locations, use
"-" for the `{location}` value.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project and location for which to retrieve backup Plan Associations
* information, in the format `projects/{project_id}/locations/{location}`. In Cloud
* BackupDR, locations map to GCP regions, for example **us-central1**. To retrieve backup
* plan associations for all locations, use "-" for the `{location}` value.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Filtering results */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filtering results
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filtering results */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server may return fewer items than requested. If unspecified, server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token identifying a page of results the server should return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results the server should return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token identifying a page of results the server should return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Triggers a new Backup.
*
* Create a request for the method "backupPlanAssociations.triggerBackup".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link TriggerBackup#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the backup plan association resource, in the format
* `projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
* @param content the {@link com.google.api.services.backupdr.v1.model.TriggerBackupRequest}
* @return the request
*/
public TriggerBackup triggerBackup(java.lang.String name, com.google.api.services.backupdr.v1.model.TriggerBackupRequest content) throws java.io.IOException {
TriggerBackup result = new TriggerBackup(name, content);
initialize(result);
return result;
}
public class TriggerBackup extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}:triggerBackup";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
/**
* Triggers a new Backup.
*
* Create a request for the method "backupPlanAssociations.triggerBackup".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link TriggerBackup#execute()} method to invoke the remote
* operation. {@link TriggerBackup#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param name Required. Name of the backup plan association resource, in the format
* `projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
* @param content the {@link com.google.api.services.backupdr.v1.model.TriggerBackupRequest}
* @since 1.13
*/
protected TriggerBackup(java.lang.String name, com.google.api.services.backupdr.v1.model.TriggerBackupRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
}
}
@Override
public TriggerBackup set$Xgafv(java.lang.String $Xgafv) {
return (TriggerBackup) super.set$Xgafv($Xgafv);
}
@Override
public TriggerBackup setAccessToken(java.lang.String accessToken) {
return (TriggerBackup) super.setAccessToken(accessToken);
}
@Override
public TriggerBackup setAlt(java.lang.String alt) {
return (TriggerBackup) super.setAlt(alt);
}
@Override
public TriggerBackup setCallback(java.lang.String callback) {
return (TriggerBackup) super.setCallback(callback);
}
@Override
public TriggerBackup setFields(java.lang.String fields) {
return (TriggerBackup) super.setFields(fields);
}
@Override
public TriggerBackup setKey(java.lang.String key) {
return (TriggerBackup) super.setKey(key);
}
@Override
public TriggerBackup setOauthToken(java.lang.String oauthToken) {
return (TriggerBackup) super.setOauthToken(oauthToken);
}
@Override
public TriggerBackup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TriggerBackup) super.setPrettyPrint(prettyPrint);
}
@Override
public TriggerBackup setQuotaUser(java.lang.String quotaUser) {
return (TriggerBackup) super.setQuotaUser(quotaUser);
}
@Override
public TriggerBackup setUploadType(java.lang.String uploadType) {
return (TriggerBackup) super.setUploadType(uploadType);
}
@Override
public TriggerBackup setUploadProtocol(java.lang.String uploadProtocol) {
return (TriggerBackup) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the backup plan association resource, in the format `projects/{projec
* t}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the backup plan association resource, in the format
`projects/{project}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the backup plan association resource, in the format `projects/{projec
* t}/locations/{location}/backupPlanAssociations/{backupPlanAssociationId}`
*/
public TriggerBackup setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlanAssociations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public TriggerBackup set(String parameterName, Object value) {
return (TriggerBackup) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BackupPlans collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.BackupPlans.List request = backupdr.backupPlans().list(parameters ...)}
*
*
* @return the resource collection
*/
public BackupPlans backupPlans() {
return new BackupPlans();
}
/**
* The "backupPlans" collection of methods.
*/
public class BackupPlans {
/**
* Create a BackupPlan
*
* Create a request for the method "backupPlans.create".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The `BackupPlan` project and location in the format
* `projects/{project}/locations/{location}`. In Cloud BackupDR locations map to GCP regions,
* for example **us-central1**.
* @param content the {@link com.google.api.services.backupdr.v1.model.BackupPlan}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.backupdr.v1.model.BackupPlan content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/backupPlans";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Create a BackupPlan
*
* Create a request for the method "backupPlans.create".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The `BackupPlan` project and location in the format
* `projects/{project}/locations/{location}`. In Cloud BackupDR locations map to GCP regions,
* for example **us-central1**.
* @param content the {@link com.google.api.services.backupdr.v1.model.BackupPlan}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.backupdr.v1.model.BackupPlan content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The `BackupPlan` project and location in the format
* `projects/{project}/locations/{location}`. In Cloud BackupDR locations map to GCP
* regions, for example **us-central1**.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The `BackupPlan` project and location in the format
`projects/{project}/locations/{location}`. In Cloud BackupDR locations map to GCP regions, for
example **us-central1**.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The `BackupPlan` project and location in the format
* `projects/{project}/locations/{location}`. In Cloud BackupDR locations map to GCP
* regions, for example **us-central1**.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The name of the `BackupPlan` to create. The name must be unique for the
* specified project and location.The name must start with a lowercase letter followed by
* up to 62 lowercase letters, numbers, or hyphens. Pattern, /a-z{,62}/.
*/
@com.google.api.client.util.Key
private java.lang.String backupPlanId;
/** Required. The name of the `BackupPlan` to create. The name must be unique for the specified project
and location.The name must start with a lowercase letter followed by up to 62 lowercase letters,
numbers, or hyphens. Pattern, /a-z{,62}/.
*/
public java.lang.String getBackupPlanId() {
return backupPlanId;
}
/**
* Required. The name of the `BackupPlan` to create. The name must be unique for the
* specified project and location.The name must start with a lowercase letter followed by
* up to 62 lowercase letters, numbers, or hyphens. Pattern, /a-z{,62}/.
*/
public Create setBackupPlanId(java.lang.String backupPlanId) {
this.backupPlanId = backupPlanId;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and t he request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes since the first request. For
example, consider a situation where you make an initial request and t he request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and t he request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single BackupPlan.
*
* Create a request for the method "backupPlans.delete".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the `BackupPlan` to delete. Format:
* `projects/{project}/locations/{location}/backupPlans/{backup_plan}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupPlans/[^/]+$");
/**
* Deletes a single BackupPlan.
*
* Create a request for the method "backupPlans.delete".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the `BackupPlan` to delete. Format:
* `projects/{project}/locations/{location}/backupPlans/{backup_plan}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Backupdr.this, "DELETE", REST_PATH, null, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlans/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the `BackupPlan` to delete. Format:
* `projects/{project}/locations/{location}/backupPlans/{backup_plan}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the `BackupPlan` to delete. Format:
`projects/{project}/locations/{location}/backupPlans/{backup_plan}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the `BackupPlan` to delete. Format:
* `projects/{project}/locations/{location}/backupPlans/{backup_plan}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlans/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* after the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes after the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* after the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single BackupPlan.
*
* Create a request for the method "backupPlans.get".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the `BackupPlan` to retrieve. Format:
* `projects/{project}/locations/{location}/backupPlans/{backup_plan}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupPlans/[^/]+$");
/**
* Gets details of a single BackupPlan.
*
* Create a request for the method "backupPlans.get".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the `BackupPlan` to retrieve. Format:
* `projects/{project}/locations/{location}/backupPlans/{backup_plan}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.BackupPlan.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlans/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the `BackupPlan` to retrieve. Format:
* `projects/{project}/locations/{location}/backupPlans/{backup_plan}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the `BackupPlan` to retrieve. Format:
`projects/{project}/locations/{location}/backupPlans/{backup_plan}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the `BackupPlan` to retrieve. Format:
* `projects/{project}/locations/{location}/backupPlans/{backup_plan}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupPlans/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists BackupPlans in a given project and location.
*
* Create a request for the method "backupPlans.list".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The project and location for which to retrieve `BackupPlans` information. Format:
* `projects/{project}/locations/{location}`. In Cloud BackupDR, locations map to GCP
* regions, for e.g. **us-central1**. To retrieve backup plans for all locations, use "-" for
* the `{location}` value.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/backupPlans";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists BackupPlans in a given project and location.
*
* Create a request for the method "backupPlans.list".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project and location for which to retrieve `BackupPlans` information. Format:
* `projects/{project}/locations/{location}`. In Cloud BackupDR, locations map to GCP
* regions, for e.g. **us-central1**. To retrieve backup plans for all locations, use "-" for
* the `{location}` value.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ListBackupPlansResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project and location for which to retrieve `BackupPlans` information.
* Format: `projects/{project}/locations/{location}`. In Cloud BackupDR, locations map to
* GCP regions, for e.g. **us-central1**. To retrieve backup plans for all locations, use
* "-" for the `{location}` value.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project and location for which to retrieve `BackupPlans` information. Format:
`projects/{project}/locations/{location}`. In Cloud BackupDR, locations map to GCP regions, for
e.g. **us-central1**. To retrieve backup plans for all locations, use "-" for the `{location}`
value.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project and location for which to retrieve `BackupPlans` information.
* Format: `projects/{project}/locations/{location}`. In Cloud BackupDR, locations map to
* GCP regions, for e.g. **us-central1**. To retrieve backup plans for all locations, use
* "-" for the `{location}` value.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Field match expression used to filter the results. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Field match expression used to filter the results.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Field match expression used to filter the results. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Optional. Field by which to sort the results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Field by which to sort the results.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Optional. Field by which to sort the results. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. The maximum number of `BackupPlans` to return in a single response. If not
* specified, a default value will be chosen by the service. Note that the response may
* include a partial list and a caller should only rely on the response's next_page_token
* to determine if there are more instances left to be queried.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of `BackupPlans` to return in a single response. If not specified, a
default value will be chosen by the service. Note that the response may include a partial list and
a caller should only rely on the response's next_page_token to determine if there are more
instances left to be queried.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of `BackupPlans` to return in a single response. If not
* specified, a default value will be chosen by the service. Note that the response may
* include a partial list and a caller should only rely on the response's next_page_token
* to determine if there are more instances left to be queried.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. The value of next_page_token received from a previous `ListBackupPlans` call.
* Provide this to retrieve the subsequent page in a multi-page list of results. When
* paginating, all other parameters provided to `ListBackupPlans` must match the call that
* provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. The value of next_page_token received from a previous `ListBackupPlans` call. Provide
this to retrieve the subsequent page in a multi-page list of results. When paginating, all other
parameters provided to `ListBackupPlans` must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. The value of next_page_token received from a previous `ListBackupPlans` call.
* Provide this to retrieve the subsequent page in a multi-page list of results. When
* paginating, all other parameters provided to `ListBackupPlans` must match the call that
* provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the BackupVaults collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.BackupVaults.List request = backupdr.backupVaults().list(parameters ...)}
*
*
* @return the resource collection
*/
public BackupVaults backupVaults() {
return new BackupVaults();
}
/**
* The "backupVaults" collection of methods.
*/
public class BackupVaults {
/**
* Create a request for the method "backupVaults.create".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Value for parent.
* @param content the {@link com.google.api.services.backupdr.v1.model.BackupVault}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.backupdr.v1.model.BackupVault content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/backupVaults";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Create a request for the method "backupVaults.create".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Value for parent.
* @param content the {@link com.google.api.services.backupdr.v1.model.BackupVault}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.backupdr.v1.model.BackupVault content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. Value for parent. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Value for parent.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Value for parent. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. ID of the requesting object If auto-generating ID server-side, remove this
* field and backup_vault_id from the method_signature of Create RPC
*/
@com.google.api.client.util.Key
private java.lang.String backupVaultId;
/** Required. ID of the requesting object If auto-generating ID server-side, remove this field and
backup_vault_id from the method_signature of Create RPC
*/
public java.lang.String getBackupVaultId() {
return backupVaultId;
}
/**
* Required. ID of the requesting object If auto-generating ID server-side, remove this
* field and backup_vault_id from the method_signature of Create RPC
*/
public Create setBackupVaultId(java.lang.String backupVaultId) {
this.backupVaultId = backupVaultId;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes since the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Optional. Only validate the request, but do not perform mutations. The default is
* 'false'.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Optional. Only validate the request, but do not perform mutations. The default is 'false'.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Optional. Only validate the request, but do not perform mutations. The default is
* 'false'.
*/
public Create setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a BackupVault.
*
* Create a request for the method "backupVaults.delete".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the resource.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
/**
* Deletes a BackupVault.
*
* Create a request for the method "backupVaults.delete".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the resource.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Backupdr.this, "DELETE", REST_PATH, null, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the resource.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the resource. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. If true and the BackupVault is not found, the request will succeed but no
* action will be taken.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowMissing;
/** Optional. If true and the BackupVault is not found, the request will succeed but no action will be
taken.
*/
public java.lang.Boolean getAllowMissing() {
return allowMissing;
}
/**
* Optional. If true and the BackupVault is not found, the request will succeed but no
* action will be taken.
*/
public Delete setAllowMissing(java.lang.Boolean allowMissing) {
this.allowMissing = allowMissing;
return this;
}
/**
* The current etag of the backup vault. If an etag is provided and does not match the
* current etag of the connection, deletion will be blocked.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** The current etag of the backup vault. If an etag is provided and does not match the current etag of
the connection, deletion will be blocked.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* The current etag of the backup vault. If an etag is provided and does not match the
* current etag of the connection, deletion will be blocked.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Optional. If set to true, any data source from this backup vault will also be deleted.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Optional. If set to true, any data source from this backup vault will also be deleted.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Optional. If set to true, any data source from this backup vault will also be deleted.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* after the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes after the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* after the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Optional. Only validate the request, but do not perform mutations. The default is
* 'false'.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Optional. Only validate the request, but do not perform mutations. The default is 'false'.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Optional. Only validate the request, but do not perform mutations. The default is
* 'false'.
*/
public Delete setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* FetchUsableBackupVaults lists usable BackupVaults in a given project and location. Usable
* BackupVault are the ones that user has backupdr.backupVaults.get permission.
*
* Create a request for the method "backupVaults.fetchUsable".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link FetchUsable#execute()} method to invoke the remote operation.
*
* @param parent Required. The project and location for which to retrieve backupvault stores information, in the
* format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map
* to Google Cloud regions, for example **us-central1**. To retrieve backupvault stores for
* all locations, use "-" for the '{location}' value.
* @return the request
*/
public FetchUsable fetchUsable(java.lang.String parent) throws java.io.IOException {
FetchUsable result = new FetchUsable(parent);
initialize(result);
return result;
}
public class FetchUsable extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/backupVaults:fetchUsable";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* FetchUsableBackupVaults lists usable BackupVaults in a given project and location. Usable
* BackupVault are the ones that user has backupdr.backupVaults.get permission.
*
* Create a request for the method "backupVaults.fetchUsable".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link FetchUsable#execute()} method to invoke the remote
* operation. {@link
* FetchUsable#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project and location for which to retrieve backupvault stores information, in the
* format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map
* to Google Cloud regions, for example **us-central1**. To retrieve backupvault stores for
* all locations, use "-" for the '{location}' value.
* @since 1.13
*/
protected FetchUsable(java.lang.String parent) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.FetchUsableBackupVaultsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public FetchUsable set$Xgafv(java.lang.String $Xgafv) {
return (FetchUsable) super.set$Xgafv($Xgafv);
}
@Override
public FetchUsable setAccessToken(java.lang.String accessToken) {
return (FetchUsable) super.setAccessToken(accessToken);
}
@Override
public FetchUsable setAlt(java.lang.String alt) {
return (FetchUsable) super.setAlt(alt);
}
@Override
public FetchUsable setCallback(java.lang.String callback) {
return (FetchUsable) super.setCallback(callback);
}
@Override
public FetchUsable setFields(java.lang.String fields) {
return (FetchUsable) super.setFields(fields);
}
@Override
public FetchUsable setKey(java.lang.String key) {
return (FetchUsable) super.setKey(key);
}
@Override
public FetchUsable setOauthToken(java.lang.String oauthToken) {
return (FetchUsable) super.setOauthToken(oauthToken);
}
@Override
public FetchUsable setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FetchUsable) super.setPrettyPrint(prettyPrint);
}
@Override
public FetchUsable setQuotaUser(java.lang.String quotaUser) {
return (FetchUsable) super.setQuotaUser(quotaUser);
}
@Override
public FetchUsable setUploadType(java.lang.String uploadType) {
return (FetchUsable) super.setUploadType(uploadType);
}
@Override
public FetchUsable setUploadProtocol(java.lang.String uploadProtocol) {
return (FetchUsable) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project and location for which to retrieve backupvault stores
* information, in the format 'projects/{project_id}/locations/{location}'. In Cloud
* Backup and DR, locations map to Google Cloud regions, for example **us-central1**. To
* retrieve backupvault stores for all locations, use "-" for the '{location}' value.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project and location for which to retrieve backupvault stores information, in the
format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map to
Google Cloud regions, for example **us-central1**. To retrieve backupvault stores for all
locations, use "-" for the '{location}' value.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project and location for which to retrieve backupvault stores
* information, in the format 'projects/{project_id}/locations/{location}'. In Cloud
* Backup and DR, locations map to Google Cloud regions, for example **us-central1**. To
* retrieve backupvault stores for all locations, use "-" for the '{location}' value.
*/
public FetchUsable setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Filtering results. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filtering results.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filtering results. */
public FetchUsable setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Optional. Hint for how to order the results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Hint for how to order the results.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Optional. Hint for how to order the results. */
public FetchUsable setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server may return fewer items than requested. If unspecified, server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
public FetchUsable setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token identifying a page of results the server should return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results the server should return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token identifying a page of results the server should return. */
public FetchUsable setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public FetchUsable set(String parameterName, Object value) {
return (FetchUsable) super.set(parameterName, value);
}
}
/**
* Gets details of a BackupVault.
*
* Create a request for the method "backupVaults.get".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the backupvault store resource name, in the format
* 'projects/{project_id}/locations/{location}/backupVaults/{resource_name}'
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
/**
* Gets details of a BackupVault.
*
* Create a request for the method "backupVaults.get".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the backupvault store resource name, in the format
* 'projects/{project_id}/locations/{location}/backupVaults/{resource_name}'
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.BackupVault.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the backupvault store resource name, in the format
* 'projects/{project_id}/locations/{location}/backupVaults/{resource_name}'
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the backupvault store resource name, in the format
'projects/{project_id}/locations/{location}/backupVaults/{resource_name}'
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the backupvault store resource name, in the format
* 'projects/{project_id}/locations/{location}/backupVaults/{resource_name}'
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists BackupVaults in a given project and location.
*
* Create a request for the method "backupVaults.list".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The project and location for which to retrieve backupvault stores information, in the
* format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map
* to Google Cloud regions, for example **us-central1**. To retrieve backupvault stores for
* all locations, use "-" for the '{location}' value.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/backupVaults";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists BackupVaults in a given project and location.
*
* Create a request for the method "backupVaults.list".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project and location for which to retrieve backupvault stores information, in the
* format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map
* to Google Cloud regions, for example **us-central1**. To retrieve backupvault stores for
* all locations, use "-" for the '{location}' value.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ListBackupVaultsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project and location for which to retrieve backupvault stores
* information, in the format 'projects/{project_id}/locations/{location}'. In Cloud
* Backup and DR, locations map to Google Cloud regions, for example **us-central1**. To
* retrieve backupvault stores for all locations, use "-" for the '{location}' value.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project and location for which to retrieve backupvault stores information, in the
format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map to
Google Cloud regions, for example **us-central1**. To retrieve backupvault stores for all
locations, use "-" for the '{location}' value.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project and location for which to retrieve backupvault stores
* information, in the format 'projects/{project_id}/locations/{location}'. In Cloud
* Backup and DR, locations map to Google Cloud regions, for example **us-central1**. To
* retrieve backupvault stores for all locations, use "-" for the '{location}' value.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Filtering results. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filtering results.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filtering results. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Optional. Hint for how to order the results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Hint for how to order the results.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Optional. Hint for how to order the results. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server may return fewer items than requested. If unspecified, server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token identifying a page of results the server should return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results the server should return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token identifying a page of results the server should return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the settings of a BackupVault.
*
* Create a request for the method "backupVaults.patch".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Identifier. The resource name.
* @param content the {@link com.google.api.services.backupdr.v1.model.BackupVault}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.backupdr.v1.model.BackupVault content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
/**
* Updates the settings of a BackupVault.
*
* Create a request for the method "backupVaults.patch".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Identifier. The resource name.
* @param content the {@link com.google.api.services.backupdr.v1.model.BackupVault}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.backupdr.v1.model.BackupVault content) {
super(Backupdr.this, "PATCH", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Output only. Identifier. The resource name. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Identifier. The resource name.
*/
public java.lang.String getName() {
return name;
}
/** Output only. Identifier. The resource name. */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. If set to true, will not check plan duration against backup vault enforcement
* duration.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** Optional. If set to true, will not check plan duration against backup vault enforcement duration.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* Optional. If set to true, will not check plan duration against backup vault enforcement
* duration.
*/
public Patch setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes since the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the BackupVault
* resource by the update. The fields specified in the update_mask are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask. If
* the user does not provide a mask then the request will fail.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the BackupVault resource by
the update. The fields specified in the update_mask are relative to the resource, not the full
request. A field will be overwritten if it is in the mask. If the user does not provide a mask then
the request will fail.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the BackupVault
* resource by the update. The fields specified in the update_mask are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask. If
* the user does not provide a mask then the request will fail.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
/**
* Optional. Only validate the request, but do not perform mutations. The default is
* 'false'.
*/
@com.google.api.client.util.Key
private java.lang.Boolean validateOnly;
/** Optional. Only validate the request, but do not perform mutations. The default is 'false'.
*/
public java.lang.Boolean getValidateOnly() {
return validateOnly;
}
/**
* Optional. Only validate the request, but do not perform mutations. The default is
* 'false'.
*/
public Patch setValidateOnly(java.lang.Boolean validateOnly) {
this.validateOnly = validateOnly;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Returns the caller's permissions on a BackupVault resource. A caller is not required to have
* Google IAM permission to make this request.
*
* Create a request for the method "backupVaults.testIamPermissions".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.backupdr.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.backupdr.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends BackupdrRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
/**
* Returns the caller's permissions on a BackupVault resource. A caller is not required to have
* Google IAM permission to make this request.
*
* Create a request for the method "backupVaults.testIamPermissions".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.backupdr.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.backupdr.v1.model.TestIamPermissionsRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the DataSources collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.DataSources.List request = backupdr.dataSources().list(parameters ...)}
*
*
* @return the resource collection
*/
public DataSources dataSources() {
return new DataSources();
}
/**
* The "dataSources" collection of methods.
*/
public class DataSources {
/**
* Internal only. Abandons a backup.
*
* Create a request for the method "dataSources.abandonBackup".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link AbandonBackup#execute()} method to invoke the remote operation.
*
* @param dataSource Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.AbandonBackupRequest}
* @return the request
*/
public AbandonBackup abandonBackup(java.lang.String dataSource, com.google.api.services.backupdr.v1.model.AbandonBackupRequest content) throws java.io.IOException {
AbandonBackup result = new AbandonBackup(dataSource, content);
initialize(result);
return result;
}
public class AbandonBackup extends BackupdrRequest {
private static final String REST_PATH = "v1/{+dataSource}:abandonBackup";
private final java.util.regex.Pattern DATA_SOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Internal only. Abandons a backup.
*
* Create a request for the method "dataSources.abandonBackup".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link AbandonBackup#execute()} method to invoke the remote
* operation. {@link AbandonBackup#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param dataSource Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.AbandonBackupRequest}
* @since 1.13
*/
protected AbandonBackup(java.lang.String dataSource, com.google.api.services.backupdr.v1.model.AbandonBackupRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.dataSource = com.google.api.client.util.Preconditions.checkNotNull(dataSource, "Required parameter dataSource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATA_SOURCE_PATTERN.matcher(dataSource).matches(),
"Parameter dataSource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public AbandonBackup set$Xgafv(java.lang.String $Xgafv) {
return (AbandonBackup) super.set$Xgafv($Xgafv);
}
@Override
public AbandonBackup setAccessToken(java.lang.String accessToken) {
return (AbandonBackup) super.setAccessToken(accessToken);
}
@Override
public AbandonBackup setAlt(java.lang.String alt) {
return (AbandonBackup) super.setAlt(alt);
}
@Override
public AbandonBackup setCallback(java.lang.String callback) {
return (AbandonBackup) super.setCallback(callback);
}
@Override
public AbandonBackup setFields(java.lang.String fields) {
return (AbandonBackup) super.setFields(fields);
}
@Override
public AbandonBackup setKey(java.lang.String key) {
return (AbandonBackup) super.setKey(key);
}
@Override
public AbandonBackup setOauthToken(java.lang.String oauthToken) {
return (AbandonBackup) super.setOauthToken(oauthToken);
}
@Override
public AbandonBackup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (AbandonBackup) super.setPrettyPrint(prettyPrint);
}
@Override
public AbandonBackup setQuotaUser(java.lang.String quotaUser) {
return (AbandonBackup) super.setQuotaUser(quotaUser);
}
@Override
public AbandonBackup setUploadType(java.lang.String uploadType) {
return (AbandonBackup) super.setUploadType(uploadType);
}
@Override
public AbandonBackup setUploadProtocol(java.lang.String uploadProtocol) {
return (AbandonBackup) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
*/
@com.google.api.client.util.Key
private java.lang.String dataSource;
/** Required. The resource name of the instance, in the format
'projects/locations/backupVaults/dataSources/'.
*/
public java.lang.String getDataSource() {
return dataSource;
}
/**
* Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
*/
public AbandonBackup setDataSource(java.lang.String dataSource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATA_SOURCE_PATTERN.matcher(dataSource).matches(),
"Parameter dataSource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.dataSource = dataSource;
return this;
}
@Override
public AbandonBackup set(String parameterName, Object value) {
return (AbandonBackup) super.set(parameterName, value);
}
}
/**
* Internal only. Fetch access token for a given data source.
*
* Create a request for the method "dataSources.fetchAccessToken".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link FetchAccessToken#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name for the location for which static IPs should be returned. Must be in the
* format 'projects/locations/backupVaults/dataSources'.
* @param content the {@link com.google.api.services.backupdr.v1.model.FetchAccessTokenRequest}
* @return the request
*/
public FetchAccessToken fetchAccessToken(java.lang.String name, com.google.api.services.backupdr.v1.model.FetchAccessTokenRequest content) throws java.io.IOException {
FetchAccessToken result = new FetchAccessToken(name, content);
initialize(result);
return result;
}
public class FetchAccessToken extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}:fetchAccessToken";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Internal only. Fetch access token for a given data source.
*
* Create a request for the method "dataSources.fetchAccessToken".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link FetchAccessToken#execute()} method to invoke the remote
* operation. {@link FetchAccessToken#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The resource name for the location for which static IPs should be returned. Must be in the
* format 'projects/locations/backupVaults/dataSources'.
* @param content the {@link com.google.api.services.backupdr.v1.model.FetchAccessTokenRequest}
* @since 1.13
*/
protected FetchAccessToken(java.lang.String name, com.google.api.services.backupdr.v1.model.FetchAccessTokenRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.FetchAccessTokenResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public FetchAccessToken set$Xgafv(java.lang.String $Xgafv) {
return (FetchAccessToken) super.set$Xgafv($Xgafv);
}
@Override
public FetchAccessToken setAccessToken(java.lang.String accessToken) {
return (FetchAccessToken) super.setAccessToken(accessToken);
}
@Override
public FetchAccessToken setAlt(java.lang.String alt) {
return (FetchAccessToken) super.setAlt(alt);
}
@Override
public FetchAccessToken setCallback(java.lang.String callback) {
return (FetchAccessToken) super.setCallback(callback);
}
@Override
public FetchAccessToken setFields(java.lang.String fields) {
return (FetchAccessToken) super.setFields(fields);
}
@Override
public FetchAccessToken setKey(java.lang.String key) {
return (FetchAccessToken) super.setKey(key);
}
@Override
public FetchAccessToken setOauthToken(java.lang.String oauthToken) {
return (FetchAccessToken) super.setOauthToken(oauthToken);
}
@Override
public FetchAccessToken setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FetchAccessToken) super.setPrettyPrint(prettyPrint);
}
@Override
public FetchAccessToken setQuotaUser(java.lang.String quotaUser) {
return (FetchAccessToken) super.setQuotaUser(quotaUser);
}
@Override
public FetchAccessToken setUploadType(java.lang.String uploadType) {
return (FetchAccessToken) super.setUploadType(uploadType);
}
@Override
public FetchAccessToken setUploadProtocol(java.lang.String uploadProtocol) {
return (FetchAccessToken) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name for the location for which static IPs should be returned.
* Must be in the format 'projects/locations/backupVaults/dataSources'.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name for the location for which static IPs should be returned. Must be in
the format 'projects/locations/backupVaults/dataSources'.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name for the location for which static IPs should be returned.
* Must be in the format 'projects/locations/backupVaults/dataSources'.
*/
public FetchAccessToken setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.name = name;
return this;
}
@Override
public FetchAccessToken set(String parameterName, Object value) {
return (FetchAccessToken) super.set(parameterName, value);
}
}
/**
* Internal only. Finalize a backup that was started by a call to InitiateBackup.
*
* Create a request for the method "dataSources.finalizeBackup".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link FinalizeBackup#execute()} method to invoke the remote operation.
*
* @param dataSource Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.FinalizeBackupRequest}
* @return the request
*/
public FinalizeBackup finalizeBackup(java.lang.String dataSource, com.google.api.services.backupdr.v1.model.FinalizeBackupRequest content) throws java.io.IOException {
FinalizeBackup result = new FinalizeBackup(dataSource, content);
initialize(result);
return result;
}
public class FinalizeBackup extends BackupdrRequest {
private static final String REST_PATH = "v1/{+dataSource}:finalizeBackup";
private final java.util.regex.Pattern DATA_SOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Internal only. Finalize a backup that was started by a call to InitiateBackup.
*
* Create a request for the method "dataSources.finalizeBackup".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link FinalizeBackup#execute()} method to invoke the remote
* operation. {@link FinalizeBackup#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param dataSource Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.FinalizeBackupRequest}
* @since 1.13
*/
protected FinalizeBackup(java.lang.String dataSource, com.google.api.services.backupdr.v1.model.FinalizeBackupRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.dataSource = com.google.api.client.util.Preconditions.checkNotNull(dataSource, "Required parameter dataSource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATA_SOURCE_PATTERN.matcher(dataSource).matches(),
"Parameter dataSource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public FinalizeBackup set$Xgafv(java.lang.String $Xgafv) {
return (FinalizeBackup) super.set$Xgafv($Xgafv);
}
@Override
public FinalizeBackup setAccessToken(java.lang.String accessToken) {
return (FinalizeBackup) super.setAccessToken(accessToken);
}
@Override
public FinalizeBackup setAlt(java.lang.String alt) {
return (FinalizeBackup) super.setAlt(alt);
}
@Override
public FinalizeBackup setCallback(java.lang.String callback) {
return (FinalizeBackup) super.setCallback(callback);
}
@Override
public FinalizeBackup setFields(java.lang.String fields) {
return (FinalizeBackup) super.setFields(fields);
}
@Override
public FinalizeBackup setKey(java.lang.String key) {
return (FinalizeBackup) super.setKey(key);
}
@Override
public FinalizeBackup setOauthToken(java.lang.String oauthToken) {
return (FinalizeBackup) super.setOauthToken(oauthToken);
}
@Override
public FinalizeBackup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FinalizeBackup) super.setPrettyPrint(prettyPrint);
}
@Override
public FinalizeBackup setQuotaUser(java.lang.String quotaUser) {
return (FinalizeBackup) super.setQuotaUser(quotaUser);
}
@Override
public FinalizeBackup setUploadType(java.lang.String uploadType) {
return (FinalizeBackup) super.setUploadType(uploadType);
}
@Override
public FinalizeBackup setUploadProtocol(java.lang.String uploadProtocol) {
return (FinalizeBackup) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
*/
@com.google.api.client.util.Key
private java.lang.String dataSource;
/** Required. The resource name of the instance, in the format
'projects/locations/backupVaults/dataSources/'.
*/
public java.lang.String getDataSource() {
return dataSource;
}
/**
* Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
*/
public FinalizeBackup setDataSource(java.lang.String dataSource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATA_SOURCE_PATTERN.matcher(dataSource).matches(),
"Parameter dataSource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.dataSource = dataSource;
return this;
}
@Override
public FinalizeBackup set(String parameterName, Object value) {
return (FinalizeBackup) super.set(parameterName, value);
}
}
/**
* Gets details of a DataSource.
*
* Create a request for the method "dataSources.get".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the data source resource name, in the format
* 'projects/{project_id}/locations/{location}/backupVaults/{resource_name}/dataSource/{resou
* rce_name}'
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Gets details of a DataSource.
*
* Create a request for the method "dataSources.get".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the data source resource name, in the format
* 'projects/{project_id}/locations/{location}/backupVaults/{resource_name}/dataSource/{resou
* rce_name}'
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.DataSource.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the data source resource name, in the format 'projects/{project_id}
* /locations/{location}/backupVaults/{resource_name}/dataSource/{resource_name}'
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the data source resource name, in the format 'projects/{project_id}/locations/{lo
cation}/backupVaults/{resource_name}/dataSource/{resource_name}'
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the data source resource name, in the format 'projects/{project_id}
* /locations/{location}/backupVaults/{resource_name}/dataSource/{resource_name}'
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Internal only. Initiates a backup.
*
* Create a request for the method "dataSources.initiateBackup".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link InitiateBackup#execute()} method to invoke the remote operation.
*
* @param dataSource Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.InitiateBackupRequest}
* @return the request
*/
public InitiateBackup initiateBackup(java.lang.String dataSource, com.google.api.services.backupdr.v1.model.InitiateBackupRequest content) throws java.io.IOException {
InitiateBackup result = new InitiateBackup(dataSource, content);
initialize(result);
return result;
}
public class InitiateBackup extends BackupdrRequest {
private static final String REST_PATH = "v1/{+dataSource}:initiateBackup";
private final java.util.regex.Pattern DATA_SOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Internal only. Initiates a backup.
*
* Create a request for the method "dataSources.initiateBackup".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link InitiateBackup#execute()} method to invoke the remote
* operation. {@link InitiateBackup#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param dataSource Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.InitiateBackupRequest}
* @since 1.13
*/
protected InitiateBackup(java.lang.String dataSource, com.google.api.services.backupdr.v1.model.InitiateBackupRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.InitiateBackupResponse.class);
this.dataSource = com.google.api.client.util.Preconditions.checkNotNull(dataSource, "Required parameter dataSource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATA_SOURCE_PATTERN.matcher(dataSource).matches(),
"Parameter dataSource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public InitiateBackup set$Xgafv(java.lang.String $Xgafv) {
return (InitiateBackup) super.set$Xgafv($Xgafv);
}
@Override
public InitiateBackup setAccessToken(java.lang.String accessToken) {
return (InitiateBackup) super.setAccessToken(accessToken);
}
@Override
public InitiateBackup setAlt(java.lang.String alt) {
return (InitiateBackup) super.setAlt(alt);
}
@Override
public InitiateBackup setCallback(java.lang.String callback) {
return (InitiateBackup) super.setCallback(callback);
}
@Override
public InitiateBackup setFields(java.lang.String fields) {
return (InitiateBackup) super.setFields(fields);
}
@Override
public InitiateBackup setKey(java.lang.String key) {
return (InitiateBackup) super.setKey(key);
}
@Override
public InitiateBackup setOauthToken(java.lang.String oauthToken) {
return (InitiateBackup) super.setOauthToken(oauthToken);
}
@Override
public InitiateBackup setPrettyPrint(java.lang.Boolean prettyPrint) {
return (InitiateBackup) super.setPrettyPrint(prettyPrint);
}
@Override
public InitiateBackup setQuotaUser(java.lang.String quotaUser) {
return (InitiateBackup) super.setQuotaUser(quotaUser);
}
@Override
public InitiateBackup setUploadType(java.lang.String uploadType) {
return (InitiateBackup) super.setUploadType(uploadType);
}
@Override
public InitiateBackup setUploadProtocol(java.lang.String uploadProtocol) {
return (InitiateBackup) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
*/
@com.google.api.client.util.Key
private java.lang.String dataSource;
/** Required. The resource name of the instance, in the format
'projects/locations/backupVaults/dataSources/'.
*/
public java.lang.String getDataSource() {
return dataSource;
}
/**
* Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
*/
public InitiateBackup setDataSource(java.lang.String dataSource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATA_SOURCE_PATTERN.matcher(dataSource).matches(),
"Parameter dataSource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.dataSource = dataSource;
return this;
}
@Override
public InitiateBackup set(String parameterName, Object value) {
return (InitiateBackup) super.set(parameterName, value);
}
}
/**
* Lists DataSources in a given project and location.
*
* Create a request for the method "dataSources.list".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The project and location for which to retrieve data sources information, in the format
* 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map to
* Google Cloud regions, for example **us-central1**. To retrieve data sources for all
* locations, use "-" for the '{location}' value.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/dataSources";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
/**
* Lists DataSources in a given project and location.
*
* Create a request for the method "dataSources.list".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project and location for which to retrieve data sources information, in the format
* 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map to
* Google Cloud regions, for example **us-central1**. To retrieve data sources for all
* locations, use "-" for the '{location}' value.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ListDataSourcesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project and location for which to retrieve data sources information, in
* the format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR,
* locations map to Google Cloud regions, for example **us-central1**. To retrieve data
* sources for all locations, use "-" for the '{location}' value.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project and location for which to retrieve data sources information, in the format
'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map to Google Cloud
regions, for example **us-central1**. To retrieve data sources for all locations, use "-" for the
'{location}' value.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project and location for which to retrieve data sources information, in
* the format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR,
* locations map to Google Cloud regions, for example **us-central1**. To retrieve data
* sources for all locations, use "-" for the '{location}' value.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Filtering results. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filtering results.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filtering results. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Optional. Hint for how to order the results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Hint for how to order the results.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Optional. Hint for how to order the results. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server may return fewer items than requested. If unspecified, server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token identifying a page of results the server should return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results the server should return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token identifying a page of results the server should return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the settings of a DataSource.
*
* Create a request for the method "dataSources.patch".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Identifier. The resource name.
* @param content the {@link com.google.api.services.backupdr.v1.model.DataSource}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.backupdr.v1.model.DataSource content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Updates the settings of a DataSource.
*
* Create a request for the method "dataSources.patch".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Identifier. The resource name.
* @param content the {@link com.google.api.services.backupdr.v1.model.DataSource}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.backupdr.v1.model.DataSource content) {
super(Backupdr.this, "PATCH", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Output only. Identifier. The resource name. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Identifier. The resource name.
*/
public java.lang.String getName() {
return name;
}
/** Output only. Identifier. The resource name. */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.name = name;
return this;
}
/** Optional. Enable upsert. */
@com.google.api.client.util.Key
private java.lang.Boolean allowMissing;
/** Optional. Enable upsert.
*/
public java.lang.Boolean getAllowMissing() {
return allowMissing;
}
/** Optional. Enable upsert. */
public Patch setAllowMissing(java.lang.Boolean allowMissing) {
this.allowMissing = allowMissing;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same
* request ID, the server can check if original operation with the same request ID was
* received, and if so, will ignore the second request. This prevents clients from
* accidentally creating duplicate commitments. The request ID must be a valid UUID with
* the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes since the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same
* request ID, the server can check if original operation with the same request ID was
* received, and if so, will ignore the second request. This prevents clients from
* accidentally creating duplicate commitments. The request ID must be a valid UUID with
* the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* DataSource resource by the update. The fields specified in the update_mask are
* relative to the resource, not the full request. A field will be overwritten if it is
* in the mask. If the user does not provide a mask then the request will fail.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the DataSource resource by
the update. The fields specified in the update_mask are relative to the resource, not the full
request. A field will be overwritten if it is in the mask. If the user does not provide a mask then
the request will fail.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the
* DataSource resource by the update. The fields specified in the update_mask are
* relative to the resource, not the full request. A field will be overwritten if it is
* in the mask. If the user does not provide a mask then the request will fail.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Deletes a DataSource. This is a custom method instead of a standard delete method because
* external clients will not delete DataSources except for BackupDR backup appliances.
*
* Create a request for the method "dataSources.remove".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Remove#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the resource.
* @param content the {@link com.google.api.services.backupdr.v1.model.RemoveDataSourceRequest}
* @return the request
*/
public Remove remove(java.lang.String name, com.google.api.services.backupdr.v1.model.RemoveDataSourceRequest content) throws java.io.IOException {
Remove result = new Remove(name, content);
initialize(result);
return result;
}
public class Remove extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}:remove";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Deletes a DataSource. This is a custom method instead of a standard delete method because
* external clients will not delete DataSources except for BackupDR backup appliances.
*
* Create a request for the method "dataSources.remove".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Remove#execute()} method to invoke the remote operation.
* {@link
* Remove#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the resource.
* @param content the {@link com.google.api.services.backupdr.v1.model.RemoveDataSourceRequest}
* @since 1.13
*/
protected Remove(java.lang.String name, com.google.api.services.backupdr.v1.model.RemoveDataSourceRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public Remove set$Xgafv(java.lang.String $Xgafv) {
return (Remove) super.set$Xgafv($Xgafv);
}
@Override
public Remove setAccessToken(java.lang.String accessToken) {
return (Remove) super.setAccessToken(accessToken);
}
@Override
public Remove setAlt(java.lang.String alt) {
return (Remove) super.setAlt(alt);
}
@Override
public Remove setCallback(java.lang.String callback) {
return (Remove) super.setCallback(callback);
}
@Override
public Remove setFields(java.lang.String fields) {
return (Remove) super.setFields(fields);
}
@Override
public Remove setKey(java.lang.String key) {
return (Remove) super.setKey(key);
}
@Override
public Remove setOauthToken(java.lang.String oauthToken) {
return (Remove) super.setOauthToken(oauthToken);
}
@Override
public Remove setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Remove) super.setPrettyPrint(prettyPrint);
}
@Override
public Remove setQuotaUser(java.lang.String quotaUser) {
return (Remove) super.setQuotaUser(quotaUser);
}
@Override
public Remove setUploadType(java.lang.String uploadType) {
return (Remove) super.setUploadType(uploadType);
}
@Override
public Remove setUploadProtocol(java.lang.String uploadProtocol) {
return (Remove) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the resource.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the resource. */
public Remove setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Remove set(String parameterName, Object value) {
return (Remove) super.set(parameterName, value);
}
}
/**
* Sets the internal status of a DataSource.
*
* Create a request for the method "dataSources.setInternalStatus".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link SetInternalStatus#execute()} method to invoke the remote operation.
*
* @param dataSource Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.SetInternalStatusRequest}
* @return the request
*/
public SetInternalStatus setInternalStatus(java.lang.String dataSource, com.google.api.services.backupdr.v1.model.SetInternalStatusRequest content) throws java.io.IOException {
SetInternalStatus result = new SetInternalStatus(dataSource, content);
initialize(result);
return result;
}
public class SetInternalStatus extends BackupdrRequest {
private static final String REST_PATH = "v1/{+dataSource}:setInternalStatus";
private final java.util.regex.Pattern DATA_SOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Sets the internal status of a DataSource.
*
* Create a request for the method "dataSources.setInternalStatus".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link SetInternalStatus#execute()} method to invoke the remote
* operation. {@link SetInternalStatus#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param dataSource Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.SetInternalStatusRequest}
* @since 1.13
*/
protected SetInternalStatus(java.lang.String dataSource, com.google.api.services.backupdr.v1.model.SetInternalStatusRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.dataSource = com.google.api.client.util.Preconditions.checkNotNull(dataSource, "Required parameter dataSource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATA_SOURCE_PATTERN.matcher(dataSource).matches(),
"Parameter dataSource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public SetInternalStatus set$Xgafv(java.lang.String $Xgafv) {
return (SetInternalStatus) super.set$Xgafv($Xgafv);
}
@Override
public SetInternalStatus setAccessToken(java.lang.String accessToken) {
return (SetInternalStatus) super.setAccessToken(accessToken);
}
@Override
public SetInternalStatus setAlt(java.lang.String alt) {
return (SetInternalStatus) super.setAlt(alt);
}
@Override
public SetInternalStatus setCallback(java.lang.String callback) {
return (SetInternalStatus) super.setCallback(callback);
}
@Override
public SetInternalStatus setFields(java.lang.String fields) {
return (SetInternalStatus) super.setFields(fields);
}
@Override
public SetInternalStatus setKey(java.lang.String key) {
return (SetInternalStatus) super.setKey(key);
}
@Override
public SetInternalStatus setOauthToken(java.lang.String oauthToken) {
return (SetInternalStatus) super.setOauthToken(oauthToken);
}
@Override
public SetInternalStatus setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetInternalStatus) super.setPrettyPrint(prettyPrint);
}
@Override
public SetInternalStatus setQuotaUser(java.lang.String quotaUser) {
return (SetInternalStatus) super.setQuotaUser(quotaUser);
}
@Override
public SetInternalStatus setUploadType(java.lang.String uploadType) {
return (SetInternalStatus) super.setUploadType(uploadType);
}
@Override
public SetInternalStatus setUploadProtocol(java.lang.String uploadProtocol) {
return (SetInternalStatus) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
*/
@com.google.api.client.util.Key
private java.lang.String dataSource;
/** Required. The resource name of the instance, in the format
'projects/locations/backupVaults/dataSources/'.
*/
public java.lang.String getDataSource() {
return dataSource;
}
/**
* Required. The resource name of the instance, in the format
* 'projects/locations/backupVaults/dataSources/'.
*/
public SetInternalStatus setDataSource(java.lang.String dataSource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(DATA_SOURCE_PATTERN.matcher(dataSource).matches(),
"Parameter dataSource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.dataSource = dataSource;
return this;
}
@Override
public SetInternalStatus set(String parameterName, Object value) {
return (SetInternalStatus) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Backups collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.Backups.List request = backupdr.backups().list(parameters ...)}
*
*
* @return the resource collection
*/
public Backups backups() {
return new Backups();
}
/**
* The "backups" collection of methods.
*/
public class Backups {
/**
* Deletes a Backup.
*
* Create a request for the method "backups.delete".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the resource.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
/**
* Deletes a Backup.
*
* Create a request for the method "backups.delete".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the resource.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Backupdr.this, "DELETE", REST_PATH, null, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the resource.
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the resource. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID
* so that if you must retry your request, the server will know to ignore the request
* if it has already been completed. The server will guarantee that for at least 60
* minutes after the first request. For example, consider a situation where you make
* an initial request and the request times out. If you make the request again with
* the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes after the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID
* so that if you must retry your request, the server will know to ignore the request
* if it has already been completed. The server will guarantee that for at least 60
* minutes after the first request. For example, consider a situation where you make
* an initial request and the request times out. If you make the request again with
* the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a Backup.
*
* Create a request for the method "backups.get".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the data source resource name, in the format 'projects/{project_id}/locations/{loc
* ation}/backupVaults/{backupVault}/dataSources/{datasource}/backups/{backup}'
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
/**
* Gets details of a Backup.
*
* Create a request for the method "backups.get".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the data source resource name, in the format 'projects/{project_id}/locations/{loc
* ation}/backupVaults/{backupVault}/dataSources/{datasource}/backups/{backup}'
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.Backup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the data source resource name, in the format 'projects/{project_i
* d}/locations/{location}/backupVaults/{backupVault}/dataSources/{datasource}/backups
* /{backup}'
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the data source resource name, in the format 'projects/{project_id}/locations/{lo
cation}/backupVaults/{backupVault}/dataSources/{datasource}/backups/{backup}'
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the data source resource name, in the format 'projects/{project_i
* d}/locations/{location}/backupVaults/{backupVault}/dataSources/{datasource}/backups
* /{backup}'
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists Backups in a given project and location.
*
* Create a request for the method "backups.list".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The project and location for which to retrieve backup information, in the format
* 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map to
* Google Cloud regions, for example **us-central1**. To retrieve data sources for all
* locations, use "-" for the '{location}' value.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/backups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
/**
* Lists Backups in a given project and location.
*
* Create a request for the method "backups.list".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project and location for which to retrieve backup information, in the format
* 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map to
* Google Cloud regions, for example **us-central1**. To retrieve data sources for all
* locations, use "-" for the '{location}' value.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ListBackupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project and location for which to retrieve backup information, in the
* format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR,
* locations map to Google Cloud regions, for example **us-central1**. To retrieve
* data sources for all locations, use "-" for the '{location}' value.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project and location for which to retrieve backup information, in the format
'projects/{project_id}/locations/{location}'. In Cloud Backup and DR, locations map to Google Cloud
regions, for example **us-central1**. To retrieve data sources for all locations, use "-" for the
'{location}' value.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project and location for which to retrieve backup information, in the
* format 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR,
* locations map to Google Cloud regions, for example **us-central1**. To retrieve
* data sources for all locations, use "-" for the '{location}' value.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Filtering results. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filtering results.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filtering results. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Optional. Hint for how to order the results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Hint for how to order the results.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Optional. Hint for how to order the results. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server may return fewer items than requested. If unspecified, server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token identifying a page of results the server should return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results the server should return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token identifying a page of results the server should return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the settings of a Backup.
*
* Create a request for the method "backups.patch".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Output only. Identifier. Name of the resource.
* @param content the {@link com.google.api.services.backupdr.v1.model.Backup}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.backupdr.v1.model.Backup content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
/**
* Updates the settings of a Backup.
*
* Create a request for the method "backups.patch".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Output only. Identifier. Name of the resource.
* @param content the {@link com.google.api.services.backupdr.v1.model.Backup}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.backupdr.v1.model.Backup content) {
super(Backupdr.this, "PATCH", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** Output only. Identifier. Name of the resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Output only. Identifier. Name of the resource.
*/
public java.lang.String getName() {
return name;
}
/** Output only. Identifier. Name of the resource. */
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID
* so that if you must retry your request, the server will know to ignore the request
* if it has already been completed. The server will guarantee that for at least 60
* minutes since the first request. For example, consider a situation where you make
* an initial request and the request times out. If you make the request again with
* the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes since the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID
* so that if you must retry your request, the server will know to ignore the request
* if it has already been completed. The server will guarantee that for at least 60
* minutes since the first request. For example, consider a situation where you make
* an initial request and the request times out. If you make the request again with
* the same request ID, the server can check if original operation with the same
* request ID was received, and if so, will ignore the second request. This prevents
* clients from accidentally creating duplicate commitments. The request ID must be a
* valid UUID with the exception that zero UUID is not supported
* (00000000-0000-0000-0000-000000000000).
*/
public Patch setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the Backup
* resource by the update. The fields specified in the update_mask are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask.
* If the user does not provide a mask then the request will fail.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. Field mask is used to specify the fields to be overwritten in the Backup resource by the
update. The fields specified in the update_mask are relative to the resource, not the full request.
A field will be overwritten if it is in the mask. If the user does not provide a mask then the
request will fail.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. Field mask is used to specify the fields to be overwritten in the Backup
* resource by the update. The fields specified in the update_mask are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask.
* If the user does not provide a mask then the request will fail.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Restore from a Backup
*
* Create a request for the method "backups.restore".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Restore#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the Backup instance, in the format
* 'projects/locations/backupVaults/dataSources/backups/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.RestoreBackupRequest}
* @return the request
*/
public Restore restore(java.lang.String name, com.google.api.services.backupdr.v1.model.RestoreBackupRequest content) throws java.io.IOException {
Restore result = new Restore(name, content);
initialize(result);
return result;
}
public class Restore extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}:restore";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
/**
* Restore from a Backup
*
* Create a request for the method "backups.restore".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Restore#execute()} method to invoke the remote operation.
* {@link
* Restore#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the Backup instance, in the format
* 'projects/locations/backupVaults/dataSources/backups/'.
* @param content the {@link com.google.api.services.backupdr.v1.model.RestoreBackupRequest}
* @since 1.13
*/
protected Restore(java.lang.String name, com.google.api.services.backupdr.v1.model.RestoreBackupRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
}
}
@Override
public Restore set$Xgafv(java.lang.String $Xgafv) {
return (Restore) super.set$Xgafv($Xgafv);
}
@Override
public Restore setAccessToken(java.lang.String accessToken) {
return (Restore) super.setAccessToken(accessToken);
}
@Override
public Restore setAlt(java.lang.String alt) {
return (Restore) super.setAlt(alt);
}
@Override
public Restore setCallback(java.lang.String callback) {
return (Restore) super.setCallback(callback);
}
@Override
public Restore setFields(java.lang.String fields) {
return (Restore) super.setFields(fields);
}
@Override
public Restore setKey(java.lang.String key) {
return (Restore) super.setKey(key);
}
@Override
public Restore setOauthToken(java.lang.String oauthToken) {
return (Restore) super.setOauthToken(oauthToken);
}
@Override
public Restore setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Restore) super.setPrettyPrint(prettyPrint);
}
@Override
public Restore setQuotaUser(java.lang.String quotaUser) {
return (Restore) super.setQuotaUser(quotaUser);
}
@Override
public Restore setUploadType(java.lang.String uploadType) {
return (Restore) super.setUploadType(uploadType);
}
@Override
public Restore setUploadProtocol(java.lang.String uploadProtocol) {
return (Restore) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the Backup instance, in the format
* 'projects/locations/backupVaults/dataSources/backups/'.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the Backup instance, in the format
'projects/locations/backupVaults/dataSources/backups/'.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the Backup instance, in the format
* 'projects/locations/backupVaults/dataSources/backups/'.
*/
public Restore setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/backupVaults/[^/]+/dataSources/[^/]+/backups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Restore set(String parameterName, Object value) {
return (Restore) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the ManagementServers collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.ManagementServers.List request = backupdr.managementServers().list(parameters ...)}
*
*
* @return the resource collection
*/
public ManagementServers managementServers() {
return new ManagementServers();
}
/**
* The "managementServers" collection of methods.
*/
public class ManagementServers {
/**
* Creates a new ManagementServer in a given project and location.
*
* Create a request for the method "managementServers.create".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The management server project and location in the format
* 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR locations map to
* Google Cloud regions, for example **us-central1**.
* @param content the {@link com.google.api.services.backupdr.v1.model.ManagementServer}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.backupdr.v1.model.ManagementServer content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/managementServers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates a new ManagementServer in a given project and location.
*
* Create a request for the method "managementServers.create".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The management server project and location in the format
* 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR locations map to
* Google Cloud regions, for example **us-central1**.
* @param content the {@link com.google.api.services.backupdr.v1.model.ManagementServer}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.backupdr.v1.model.ManagementServer content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Operation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The management server project and location in the format
* 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR locations map to
* Google Cloud regions, for example **us-central1**.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The management server project and location in the format
'projects/{project_id}/locations/{location}'. In Cloud Backup and DR locations map to Google Cloud
regions, for example **us-central1**.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The management server project and location in the format
* 'projects/{project_id}/locations/{location}'. In Cloud Backup and DR locations map to
* Google Cloud regions, for example **us-central1**.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The name of the management server to create. The name must be unique for the
* specified project and location.
*/
@com.google.api.client.util.Key
private java.lang.String managementServerId;
/** Required. The name of the management server to create. The name must be unique for the specified
project and location.
*/
public java.lang.String getManagementServerId() {
return managementServerId;
}
/**
* Required. The name of the management server to create. The name must be unique for the
* specified project and location.
*/
public Create setManagementServerId(java.lang.String managementServerId) {
this.managementServerId = managementServerId;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes since the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* since the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Create setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a single ManagementServer.
*
* Create a request for the method "managementServers.delete".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the resource
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
/**
* Deletes a single ManagementServer.
*
* Create a request for the method "managementServers.delete".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the resource
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Backupdr.this, "DELETE", REST_PATH, null, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. Name of the resource */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the resource
*/
public java.lang.String getName() {
return name;
}
/** Required. Name of the resource */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* after the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
@com.google.api.client.util.Key
private java.lang.String requestId;
/** Optional. An optional request ID to identify requests. Specify a unique request ID so that if you
must retry your request, the server will know to ignore the request if it has already been
completed. The server will guarantee that for at least 60 minutes after the first request. For
example, consider a situation where you make an initial request and the request times out. If you
make the request again with the same request ID, the server can check if original operation with
the same request ID was received, and if so, will ignore the second request. This prevents clients
from accidentally creating duplicate commitments. The request ID must be a valid UUID with the
exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public java.lang.String getRequestId() {
return requestId;
}
/**
* Optional. An optional request ID to identify requests. Specify a unique request ID so
* that if you must retry your request, the server will know to ignore the request if it
* has already been completed. The server will guarantee that for at least 60 minutes
* after the first request. For example, consider a situation where you make an initial
* request and the request times out. If you make the request again with the same request
* ID, the server can check if original operation with the same request ID was received,
* and if so, will ignore the second request. This prevents clients from accidentally
* creating duplicate commitments. The request ID must be a valid UUID with the exception
* that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
*/
public Delete setRequestId(java.lang.String requestId) {
this.requestId = requestId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets details of a single ManagementServer.
*
* Create a request for the method "managementServers.get".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the management server resource name, in the format
* 'projects/{project_id}/locations/{location}/managementServers/{resource_name}'
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
/**
* Gets details of a single ManagementServer.
*
* Create a request for the method "managementServers.get".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the management server resource name, in the format
* 'projects/{project_id}/locations/{location}/managementServers/{resource_name}'
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ManagementServer.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the management server resource name, in the format
* 'projects/{project_id}/locations/{location}/managementServers/{resource_name}'
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the management server resource name, in the format
'projects/{project_id}/locations/{location}/managementServers/{resource_name}'
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the management server resource name, in the format
* 'projects/{project_id}/locations/{location}/managementServers/{resource_name}'
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists and
* does not have a policy set.
*
* Create a request for the method "managementServers.getIamPolicy".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource);
initialize(result);
return result;
}
public class GetIamPolicy extends BackupdrRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
/**
* Gets the access control policy for a resource. Returns an empty policy if the resource exists
* and does not have a policy set.
*
* Create a request for the method "managementServers.getIamPolicy".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
this.resource = resource;
return this;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
@com.google.api.client.util.Key("options.requestedPolicyVersion")
private java.lang.Integer optionsRequestedPolicyVersion;
/** Optional. The maximum policy version that will be used to format the policy. Valid values are 0, 1,
and 3. Requests specifying an invalid value will be rejected. Requests for policies with any
conditional role bindings must specify version 3. Policies with no conditional role bindings may
specify any valid value or leave the field unset. The policy in the response might use the policy
version that you specified, or it might use a lower policy version. For example, if you specify
version 3, but the policy has no conditional role bindings, the response uses version 1. To learn
which resources support conditions in their IAM policies, see the [IAM
documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public java.lang.Integer getOptionsRequestedPolicyVersion() {
return optionsRequestedPolicyVersion;
}
/**
* Optional. The maximum policy version that will be used to format the policy. Valid
* values are 0, 1, and 3. Requests specifying an invalid value will be rejected. Requests
* for policies with any conditional role bindings must specify version 3. Policies with
* no conditional role bindings may specify any valid value or leave the field unset. The
* policy in the response might use the policy version that you specified, or it might use
* a lower policy version. For example, if you specify version 3, but the policy has no
* conditional role bindings, the response uses version 1. To learn which resources
* support conditions in their IAM policies, see the [IAM
* documentation](https://cloud.google.com/iam/help/conditions/resource-policies).
*/
public GetIamPolicy setOptionsRequestedPolicyVersion(java.lang.Integer optionsRequestedPolicyVersion) {
this.optionsRequestedPolicyVersion = optionsRequestedPolicyVersion;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists ManagementServers in a given project and location.
*
* Create a request for the method "managementServers.list".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The project and location for which to retrieve management servers information, in the
* format 'projects/{project_id}/locations/{location}'. In Cloud BackupDR, locations map to
* Google Cloud regions, for example **us-central1**. To retrieve management servers for all
* locations, use "-" for the '{location}' value.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends BackupdrRequest {
private static final String REST_PATH = "v1/{+parent}/managementServers";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists ManagementServers in a given project and location.
*
* Create a request for the method "managementServers.list".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The project and location for which to retrieve management servers information, in the
* format 'projects/{project_id}/locations/{location}'. In Cloud BackupDR, locations map to
* Google Cloud regions, for example **us-central1**. To retrieve management servers for all
* locations, use "-" for the '{location}' value.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ListManagementServersResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The project and location for which to retrieve management servers
* information, in the format 'projects/{project_id}/locations/{location}'. In Cloud
* BackupDR, locations map to Google Cloud regions, for example **us-central1**. To
* retrieve management servers for all locations, use "-" for the '{location}' value.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The project and location for which to retrieve management servers information, in the
format 'projects/{project_id}/locations/{location}'. In Cloud BackupDR, locations map to Google
Cloud regions, for example **us-central1**. To retrieve management servers for all locations, use
"-" for the '{location}' value.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The project and location for which to retrieve management servers
* information, in the format 'projects/{project_id}/locations/{location}'. In Cloud
* BackupDR, locations map to Google Cloud regions, for example **us-central1**. To
* retrieve management servers for all locations, use "-" for the '{location}' value.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/** Optional. Filtering results. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** Optional. Filtering results.
*/
public java.lang.String getFilter() {
return filter;
}
/** Optional. Filtering results. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Optional. Hint for how to order the results. */
@com.google.api.client.util.Key
private java.lang.String orderBy;
/** Optional. Hint for how to order the results.
*/
public java.lang.String getOrderBy() {
return orderBy;
}
/** Optional. Hint for how to order the results. */
public List setOrderBy(java.lang.String orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. Requested page size. Server may return fewer items than requested. If unspecified, server
will pick an appropriate default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. Requested page size. Server may return fewer items than requested. If
* unspecified, server will pick an appropriate default.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Optional. A token identifying a page of results the server should return. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A token identifying a page of results the server should return.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Optional. A token identifying a page of results the server should return. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "managementServers.setIamPolicy".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.backupdr.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.backupdr.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends BackupdrRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
/**
* Sets the access control policy on the specified resource. Replaces any existing policy. Can
* return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
*
* Create a request for the method "managementServers.setIamPolicy".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.backupdr.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.backupdr.v1.model.SetIamPolicyRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. If the resource does not exist,
* this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
* designed to be used for building permission-aware UIs and command-line tools, not for
* authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "managementServers.testIamPermissions".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.backupdr.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.backupdr.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends BackupdrRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
/**
* Returns permissions that a caller has on the specified resource. If the resource does not
* exist, this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This
* operation is designed to be used for building permission-aware UIs and command-line tools, not
* for authorization checking. This operation may "fail open" without warning.
*
* Create a request for the method "managementServers.testIamPermissions".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.backupdr.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.backupdr.v1.model.TestIamPermissionsRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value
* for this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/managementServers/[^/]+$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Backupdr backupdr = new Backupdr(...);}
* {@code Backupdr.Operations.List request = backupdr.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.backupdr.v1.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.backupdr.v1.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.backupdr.v1.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.backupdr.v1.model.CancelOperationRequest content) {
super(Backupdr.this, "POST", REST_PATH, content, com.google.api.services.backupdr.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be deleted.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Deletes a long-running operation. This method indicates that the client is no longer interested
* in the operation result. It does not cancel the operation. If the server doesn't support this
* method, it returns `google.rpc.Code.UNIMPLEMENTED`.
*
* Create a request for the method "operations.delete".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Backupdr.this, "DELETE", REST_PATH, null, com.google.api.services.backupdr.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be deleted. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be deleted.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be deleted. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the backupdr server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends BackupdrRequest {
private static final String REST_PATH = "v1/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the backupdr server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Backupdr.this, "GET", REST_PATH, null, com.google.api.services.backupdr.v1.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* Builder for {@link Backupdr}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Backupdr}. */
@Override
public Backupdr build() {
return new Backupdr(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link BackupdrRequestInitializer}.
*
* @since 1.12
*/
public Builder setBackupdrRequestInitializer(
BackupdrRequestInitializer backupdrRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(backupdrRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}