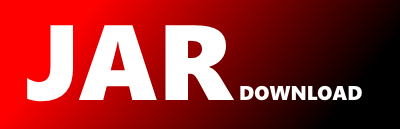
com.google.api.services.backupdr.v1.model.AttachedDisk Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* An instance-attached disk resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AttachedDisk extends com.google.api.client.json.GenericJson {
/**
* Optional. Specifies whether the disk will be auto-deleted when the instance is deleted (but not
* when the disk is detached from the instance).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean autoDelete;
/**
* Optional. Indicates that this is a boot disk. The virtual machine will use the first partition
* of the disk for its root filesystem.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean boot;
/**
* Optional. This is used as an identifier for the disks. This is the unique name has to provided
* to modify disk parameters like disk_name and replica_zones (in case of RePDs)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String deviceName;
/**
* Optional. Encrypts or decrypts a disk using a customer-supplied encryption key.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CustomerEncryptionKey diskEncryptionKey;
/**
* Optional. Specifies the disk interface to use for attaching this disk.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String diskInterface;
/**
* Optional. The size of the disk in GB.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long diskSizeGb;
/**
* Optional. Output only. The URI of the disk type resource. For example:
* projects/project/zones/zone/diskTypes/pd-standard or pd-ssd
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String diskType;
/**
* Specifies the type of the disk.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String diskTypeDeprecated;
/**
* Optional. A list of features to enable on the guest operating system. Applicable only for
* bootable images.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List guestOsFeature;
/**
* Optional. A zero-based index to this disk, where 0 is reserved for the boot disk.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long index;
/**
* Optional. Specifies the parameters to initialize this disk.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private InitializeParams initializeParams;
/**
* Optional. Type of the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Optional. Any valid publicly visible licenses.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List license;
/**
* Optional. The mode in which to attach this disk.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mode;
/**
* Optional. Output only. The state of the disk.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String savedState;
/**
* Optional. Specifies a valid partial or full URL to an existing Persistent Disk resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String source;
/**
* Optional. Specifies the type of the disk.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* Optional. Specifies whether the disk will be auto-deleted when the instance is deleted (but not
* when the disk is detached from the instance).
* @return value or {@code null} for none
*/
public java.lang.Boolean getAutoDelete() {
return autoDelete;
}
/**
* Optional. Specifies whether the disk will be auto-deleted when the instance is deleted (but not
* when the disk is detached from the instance).
* @param autoDelete autoDelete or {@code null} for none
*/
public AttachedDisk setAutoDelete(java.lang.Boolean autoDelete) {
this.autoDelete = autoDelete;
return this;
}
/**
* Optional. Indicates that this is a boot disk. The virtual machine will use the first partition
* of the disk for its root filesystem.
* @return value or {@code null} for none
*/
public java.lang.Boolean getBoot() {
return boot;
}
/**
* Optional. Indicates that this is a boot disk. The virtual machine will use the first partition
* of the disk for its root filesystem.
* @param boot boot or {@code null} for none
*/
public AttachedDisk setBoot(java.lang.Boolean boot) {
this.boot = boot;
return this;
}
/**
* Optional. This is used as an identifier for the disks. This is the unique name has to provided
* to modify disk parameters like disk_name and replica_zones (in case of RePDs)
* @return value or {@code null} for none
*/
public java.lang.String getDeviceName() {
return deviceName;
}
/**
* Optional. This is used as an identifier for the disks. This is the unique name has to provided
* to modify disk parameters like disk_name and replica_zones (in case of RePDs)
* @param deviceName deviceName or {@code null} for none
*/
public AttachedDisk setDeviceName(java.lang.String deviceName) {
this.deviceName = deviceName;
return this;
}
/**
* Optional. Encrypts or decrypts a disk using a customer-supplied encryption key.
* @return value or {@code null} for none
*/
public CustomerEncryptionKey getDiskEncryptionKey() {
return diskEncryptionKey;
}
/**
* Optional. Encrypts or decrypts a disk using a customer-supplied encryption key.
* @param diskEncryptionKey diskEncryptionKey or {@code null} for none
*/
public AttachedDisk setDiskEncryptionKey(CustomerEncryptionKey diskEncryptionKey) {
this.diskEncryptionKey = diskEncryptionKey;
return this;
}
/**
* Optional. Specifies the disk interface to use for attaching this disk.
* @return value or {@code null} for none
*/
public java.lang.String getDiskInterface() {
return diskInterface;
}
/**
* Optional. Specifies the disk interface to use for attaching this disk.
* @param diskInterface diskInterface or {@code null} for none
*/
public AttachedDisk setDiskInterface(java.lang.String diskInterface) {
this.diskInterface = diskInterface;
return this;
}
/**
* Optional. The size of the disk in GB.
* @return value or {@code null} for none
*/
public java.lang.Long getDiskSizeGb() {
return diskSizeGb;
}
/**
* Optional. The size of the disk in GB.
* @param diskSizeGb diskSizeGb or {@code null} for none
*/
public AttachedDisk setDiskSizeGb(java.lang.Long diskSizeGb) {
this.diskSizeGb = diskSizeGb;
return this;
}
/**
* Optional. Output only. The URI of the disk type resource. For example:
* projects/project/zones/zone/diskTypes/pd-standard or pd-ssd
* @return value or {@code null} for none
*/
public java.lang.String getDiskType() {
return diskType;
}
/**
* Optional. Output only. The URI of the disk type resource. For example:
* projects/project/zones/zone/diskTypes/pd-standard or pd-ssd
* @param diskType diskType or {@code null} for none
*/
public AttachedDisk setDiskType(java.lang.String diskType) {
this.diskType = diskType;
return this;
}
/**
* Specifies the type of the disk.
* @return value or {@code null} for none
*/
public java.lang.String getDiskTypeDeprecated() {
return diskTypeDeprecated;
}
/**
* Specifies the type of the disk.
* @param diskTypeDeprecated diskTypeDeprecated or {@code null} for none
*/
public AttachedDisk setDiskTypeDeprecated(java.lang.String diskTypeDeprecated) {
this.diskTypeDeprecated = diskTypeDeprecated;
return this;
}
/**
* Optional. A list of features to enable on the guest operating system. Applicable only for
* bootable images.
* @return value or {@code null} for none
*/
public java.util.List getGuestOsFeature() {
return guestOsFeature;
}
/**
* Optional. A list of features to enable on the guest operating system. Applicable only for
* bootable images.
* @param guestOsFeature guestOsFeature or {@code null} for none
*/
public AttachedDisk setGuestOsFeature(java.util.List guestOsFeature) {
this.guestOsFeature = guestOsFeature;
return this;
}
/**
* Optional. A zero-based index to this disk, where 0 is reserved for the boot disk.
* @return value or {@code null} for none
*/
public java.lang.Long getIndex() {
return index;
}
/**
* Optional. A zero-based index to this disk, where 0 is reserved for the boot disk.
* @param index index or {@code null} for none
*/
public AttachedDisk setIndex(java.lang.Long index) {
this.index = index;
return this;
}
/**
* Optional. Specifies the parameters to initialize this disk.
* @return value or {@code null} for none
*/
public InitializeParams getInitializeParams() {
return initializeParams;
}
/**
* Optional. Specifies the parameters to initialize this disk.
* @param initializeParams initializeParams or {@code null} for none
*/
public AttachedDisk setInitializeParams(InitializeParams initializeParams) {
this.initializeParams = initializeParams;
return this;
}
/**
* Optional. Type of the resource.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Optional. Type of the resource.
* @param kind kind or {@code null} for none
*/
public AttachedDisk setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Optional. Any valid publicly visible licenses.
* @return value or {@code null} for none
*/
public java.util.List getLicense() {
return license;
}
/**
* Optional. Any valid publicly visible licenses.
* @param license license or {@code null} for none
*/
public AttachedDisk setLicense(java.util.List license) {
this.license = license;
return this;
}
/**
* Optional. The mode in which to attach this disk.
* @return value or {@code null} for none
*/
public java.lang.String getMode() {
return mode;
}
/**
* Optional. The mode in which to attach this disk.
* @param mode mode or {@code null} for none
*/
public AttachedDisk setMode(java.lang.String mode) {
this.mode = mode;
return this;
}
/**
* Optional. Output only. The state of the disk.
* @return value or {@code null} for none
*/
public java.lang.String getSavedState() {
return savedState;
}
/**
* Optional. Output only. The state of the disk.
* @param savedState savedState or {@code null} for none
*/
public AttachedDisk setSavedState(java.lang.String savedState) {
this.savedState = savedState;
return this;
}
/**
* Optional. Specifies a valid partial or full URL to an existing Persistent Disk resource.
* @return value or {@code null} for none
*/
public java.lang.String getSource() {
return source;
}
/**
* Optional. Specifies a valid partial or full URL to an existing Persistent Disk resource.
* @param source source or {@code null} for none
*/
public AttachedDisk setSource(java.lang.String source) {
this.source = source;
return this;
}
/**
* Optional. Specifies the type of the disk.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Optional. Specifies the type of the disk.
* @param type type or {@code null} for none
*/
public AttachedDisk setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public AttachedDisk set(String fieldName, Object value) {
return (AttachedDisk) super.set(fieldName, value);
}
@Override
public AttachedDisk clone() {
return (AttachedDisk) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy