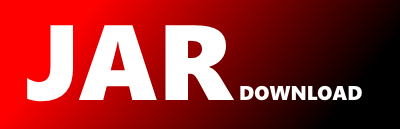
com.google.api.services.backupdr.v1.model.BackupConfigInfo Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* BackupConfigInfo has information about how the resource is configured for Backup and about the
* most recent backup to this vault.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class BackupConfigInfo extends com.google.api.client.json.GenericJson {
/**
* Configuration for an application backed up by a Backup Appliance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BackupApplianceBackupConfig backupApplianceBackupConfig;
/**
* Configuration for a Google Cloud resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GcpBackupConfig gcpBackupConfig;
/**
* Output only. If the last backup failed, this field has the error message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Status lastBackupError;
/**
* Output only. The status of the last backup to this BackupVault
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String lastBackupState;
/**
* Output only. If the last backup were successful, this field has the consistency date.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String lastSuccessfulBackupConsistencyTime;
/**
* Configuration for an application backed up by a Backup Appliance.
* @return value or {@code null} for none
*/
public BackupApplianceBackupConfig getBackupApplianceBackupConfig() {
return backupApplianceBackupConfig;
}
/**
* Configuration for an application backed up by a Backup Appliance.
* @param backupApplianceBackupConfig backupApplianceBackupConfig or {@code null} for none
*/
public BackupConfigInfo setBackupApplianceBackupConfig(BackupApplianceBackupConfig backupApplianceBackupConfig) {
this.backupApplianceBackupConfig = backupApplianceBackupConfig;
return this;
}
/**
* Configuration for a Google Cloud resource.
* @return value or {@code null} for none
*/
public GcpBackupConfig getGcpBackupConfig() {
return gcpBackupConfig;
}
/**
* Configuration for a Google Cloud resource.
* @param gcpBackupConfig gcpBackupConfig or {@code null} for none
*/
public BackupConfigInfo setGcpBackupConfig(GcpBackupConfig gcpBackupConfig) {
this.gcpBackupConfig = gcpBackupConfig;
return this;
}
/**
* Output only. If the last backup failed, this field has the error message.
* @return value or {@code null} for none
*/
public Status getLastBackupError() {
return lastBackupError;
}
/**
* Output only. If the last backup failed, this field has the error message.
* @param lastBackupError lastBackupError or {@code null} for none
*/
public BackupConfigInfo setLastBackupError(Status lastBackupError) {
this.lastBackupError = lastBackupError;
return this;
}
/**
* Output only. The status of the last backup to this BackupVault
* @return value or {@code null} for none
*/
public java.lang.String getLastBackupState() {
return lastBackupState;
}
/**
* Output only. The status of the last backup to this BackupVault
* @param lastBackupState lastBackupState or {@code null} for none
*/
public BackupConfigInfo setLastBackupState(java.lang.String lastBackupState) {
this.lastBackupState = lastBackupState;
return this;
}
/**
* Output only. If the last backup were successful, this field has the consistency date.
* @return value or {@code null} for none
*/
public String getLastSuccessfulBackupConsistencyTime() {
return lastSuccessfulBackupConsistencyTime;
}
/**
* Output only. If the last backup were successful, this field has the consistency date.
* @param lastSuccessfulBackupConsistencyTime lastSuccessfulBackupConsistencyTime or {@code null} for none
*/
public BackupConfigInfo setLastSuccessfulBackupConsistencyTime(String lastSuccessfulBackupConsistencyTime) {
this.lastSuccessfulBackupConsistencyTime = lastSuccessfulBackupConsistencyTime;
return this;
}
@Override
public BackupConfigInfo set(String fieldName, Object value) {
return (BackupConfigInfo) super.set(fieldName, value);
}
@Override
public BackupConfigInfo clone() {
return (BackupConfigInfo) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy