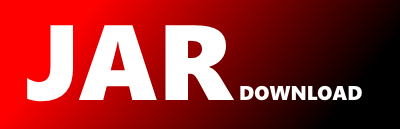
com.google.api.services.backupdr.v1.model.BackupRule Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* `BackupRule` binds the backup schedule to a retention policy.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class BackupRule extends com.google.api.client.json.GenericJson {
/**
* Required. Configures the duration for which backup data will be kept. It is defined in “days”.
* The value should be greater than or equal to minimum enforced retention of the backup vault.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer backupRetentionDays;
/**
* Optional. TODO b/341576760: Remove deprecated BV and Datasource field form BP and BPA once UI
* removed all dependencies on them Required. Resource name of backup vault which will be used as
* storage location for backups. Format:
* projects/{project}/locations/{location}/backupVaults/{backupvault}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String backupVault;
/**
* Output only. TODO b/341576760: Remove deprecated BV and Datasource field form BP and BPA once
* UI removed all dependencies on them Output only. The Google Cloud Platform Service Account to
* be used by the BackupVault for taking backups. Specify the email address of the Backup Vault
* Service Account.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String backupVaultServiceAccount;
/**
* Required. Immutable. The unique id of this `BackupRule`. The `rule_id` is unique per
* `BackupPlan`.The `rule_id` must start with a lowercase letter followed by up to 62 lowercase
* letters, numbers, or hyphens. Pattern, /a-z{,62}/.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ruleId;
/**
* Required. Defines a schedule that runs within the confines of a defined window of time.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private StandardSchedule standardSchedule;
/**
* Required. Configures the duration for which backup data will be kept. It is defined in “days”.
* The value should be greater than or equal to minimum enforced retention of the backup vault.
* @return value or {@code null} for none
*/
public java.lang.Integer getBackupRetentionDays() {
return backupRetentionDays;
}
/**
* Required. Configures the duration for which backup data will be kept. It is defined in “days”.
* The value should be greater than or equal to minimum enforced retention of the backup vault.
* @param backupRetentionDays backupRetentionDays or {@code null} for none
*/
public BackupRule setBackupRetentionDays(java.lang.Integer backupRetentionDays) {
this.backupRetentionDays = backupRetentionDays;
return this;
}
/**
* Optional. TODO b/341576760: Remove deprecated BV and Datasource field form BP and BPA once UI
* removed all dependencies on them Required. Resource name of backup vault which will be used as
* storage location for backups. Format:
* projects/{project}/locations/{location}/backupVaults/{backupvault}
* @return value or {@code null} for none
*/
public java.lang.String getBackupVault() {
return backupVault;
}
/**
* Optional. TODO b/341576760: Remove deprecated BV and Datasource field form BP and BPA once UI
* removed all dependencies on them Required. Resource name of backup vault which will be used as
* storage location for backups. Format:
* projects/{project}/locations/{location}/backupVaults/{backupvault}
* @param backupVault backupVault or {@code null} for none
*/
public BackupRule setBackupVault(java.lang.String backupVault) {
this.backupVault = backupVault;
return this;
}
/**
* Output only. TODO b/341576760: Remove deprecated BV and Datasource field form BP and BPA once
* UI removed all dependencies on them Output only. The Google Cloud Platform Service Account to
* be used by the BackupVault for taking backups. Specify the email address of the Backup Vault
* Service Account.
* @return value or {@code null} for none
*/
public java.lang.String getBackupVaultServiceAccount() {
return backupVaultServiceAccount;
}
/**
* Output only. TODO b/341576760: Remove deprecated BV and Datasource field form BP and BPA once
* UI removed all dependencies on them Output only. The Google Cloud Platform Service Account to
* be used by the BackupVault for taking backups. Specify the email address of the Backup Vault
* Service Account.
* @param backupVaultServiceAccount backupVaultServiceAccount or {@code null} for none
*/
public BackupRule setBackupVaultServiceAccount(java.lang.String backupVaultServiceAccount) {
this.backupVaultServiceAccount = backupVaultServiceAccount;
return this;
}
/**
* Required. Immutable. The unique id of this `BackupRule`. The `rule_id` is unique per
* `BackupPlan`.The `rule_id` must start with a lowercase letter followed by up to 62 lowercase
* letters, numbers, or hyphens. Pattern, /a-z{,62}/.
* @return value or {@code null} for none
*/
public java.lang.String getRuleId() {
return ruleId;
}
/**
* Required. Immutable. The unique id of this `BackupRule`. The `rule_id` is unique per
* `BackupPlan`.The `rule_id` must start with a lowercase letter followed by up to 62 lowercase
* letters, numbers, or hyphens. Pattern, /a-z{,62}/.
* @param ruleId ruleId or {@code null} for none
*/
public BackupRule setRuleId(java.lang.String ruleId) {
this.ruleId = ruleId;
return this;
}
/**
* Required. Defines a schedule that runs within the confines of a defined window of time.
* @return value or {@code null} for none
*/
public StandardSchedule getStandardSchedule() {
return standardSchedule;
}
/**
* Required. Defines a schedule that runs within the confines of a defined window of time.
* @param standardSchedule standardSchedule or {@code null} for none
*/
public BackupRule setStandardSchedule(StandardSchedule standardSchedule) {
this.standardSchedule = standardSchedule;
return this;
}
@Override
public BackupRule set(String fieldName, Object value) {
return (BackupRule) super.set(fieldName, value);
}
@Override
public BackupRule clone() {
return (BackupRule) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy