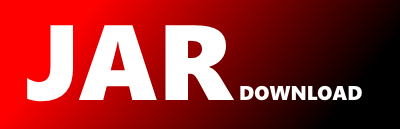
com.google.api.services.backupdr.v1.model.BackupVault Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* Message describing a BackupVault object.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class BackupVault extends com.google.api.client.json.GenericJson {
/**
* Optional. User annotations. See https://google.aip.dev/128#annotations Stores small amounts of
* arbitrary data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map annotations;
/**
* Output only. The number of backups in this backup vault.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long backupCount;
/**
* Required. The default and minimum enforced retention for each backup within the backup vault.
* The enforced retention for each backup can be extended.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String backupMinimumEnforcedRetentionDuration;
/**
* Output only. The time when the instance was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* Output only. Set to true when there are no backups nested under this resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean deletable;
/**
* Optional. The description of the BackupVault instance (2048 characters or less).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Optional. Time after which the BackupVault resource is locked.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String effectiveTime;
/**
* Required. The default retention period for each backup in the backup vault (Deprecated).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String enforcedRetentionDuration;
/**
* Optional. Server specified ETag for the backup vault resource to prevent simultaneous updates
* from overwiting each other.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* Optional. Resource labels to represent user provided metadata. No labels currently defined:
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Output only. Identifier. The resource name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. Service account used by the BackupVault Service for this BackupVault. The user
* should grant this account permissions in their workload project to enable the service to run
* backups and restores there.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceAccount;
/**
* Output only. The BackupVault resource instance state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* Output only. Total size of the storage used by all backup resources.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalStoredBytes;
/**
* Output only. Output only Immutable after resource creation until resource deletion.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uid;
/**
* Output only. The time when the instance was updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Optional. User annotations. See https://google.aip.dev/128#annotations Stores small amounts of
* arbitrary data.
* @return value or {@code null} for none
*/
public java.util.Map getAnnotations() {
return annotations;
}
/**
* Optional. User annotations. See https://google.aip.dev/128#annotations Stores small amounts of
* arbitrary data.
* @param annotations annotations or {@code null} for none
*/
public BackupVault setAnnotations(java.util.Map annotations) {
this.annotations = annotations;
return this;
}
/**
* Output only. The number of backups in this backup vault.
* @return value or {@code null} for none
*/
public java.lang.Long getBackupCount() {
return backupCount;
}
/**
* Output only. The number of backups in this backup vault.
* @param backupCount backupCount or {@code null} for none
*/
public BackupVault setBackupCount(java.lang.Long backupCount) {
this.backupCount = backupCount;
return this;
}
/**
* Required. The default and minimum enforced retention for each backup within the backup vault.
* The enforced retention for each backup can be extended.
* @return value or {@code null} for none
*/
public String getBackupMinimumEnforcedRetentionDuration() {
return backupMinimumEnforcedRetentionDuration;
}
/**
* Required. The default and minimum enforced retention for each backup within the backup vault.
* The enforced retention for each backup can be extended.
* @param backupMinimumEnforcedRetentionDuration backupMinimumEnforcedRetentionDuration or {@code null} for none
*/
public BackupVault setBackupMinimumEnforcedRetentionDuration(String backupMinimumEnforcedRetentionDuration) {
this.backupMinimumEnforcedRetentionDuration = backupMinimumEnforcedRetentionDuration;
return this;
}
/**
* Output only. The time when the instance was created.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The time when the instance was created.
* @param createTime createTime or {@code null} for none
*/
public BackupVault setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* Output only. Set to true when there are no backups nested under this resource.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDeletable() {
return deletable;
}
/**
* Output only. Set to true when there are no backups nested under this resource.
* @param deletable deletable or {@code null} for none
*/
public BackupVault setDeletable(java.lang.Boolean deletable) {
this.deletable = deletable;
return this;
}
/**
* Optional. The description of the BackupVault instance (2048 characters or less).
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Optional. The description of the BackupVault instance (2048 characters or less).
* @param description description or {@code null} for none
*/
public BackupVault setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Optional. Time after which the BackupVault resource is locked.
* @return value or {@code null} for none
*/
public String getEffectiveTime() {
return effectiveTime;
}
/**
* Optional. Time after which the BackupVault resource is locked.
* @param effectiveTime effectiveTime or {@code null} for none
*/
public BackupVault setEffectiveTime(String effectiveTime) {
this.effectiveTime = effectiveTime;
return this;
}
/**
* Required. The default retention period for each backup in the backup vault (Deprecated).
* @return value or {@code null} for none
*/
public String getEnforcedRetentionDuration() {
return enforcedRetentionDuration;
}
/**
* Required. The default retention period for each backup in the backup vault (Deprecated).
* @param enforcedRetentionDuration enforcedRetentionDuration or {@code null} for none
*/
public BackupVault setEnforcedRetentionDuration(String enforcedRetentionDuration) {
this.enforcedRetentionDuration = enforcedRetentionDuration;
return this;
}
/**
* Optional. Server specified ETag for the backup vault resource to prevent simultaneous updates
* from overwiting each other.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Optional. Server specified ETag for the backup vault resource to prevent simultaneous updates
* from overwiting each other.
* @param etag etag or {@code null} for none
*/
public BackupVault setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Optional. Resource labels to represent user provided metadata. No labels currently defined:
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Optional. Resource labels to represent user provided metadata. No labels currently defined:
* @param labels labels or {@code null} for none
*/
public BackupVault setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Output only. Identifier. The resource name.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Identifier. The resource name.
* @param name name or {@code null} for none
*/
public BackupVault setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. Service account used by the BackupVault Service for this BackupVault. The user
* should grant this account permissions in their workload project to enable the service to run
* backups and restores there.
* @return value or {@code null} for none
*/
public java.lang.String getServiceAccount() {
return serviceAccount;
}
/**
* Output only. Service account used by the BackupVault Service for this BackupVault. The user
* should grant this account permissions in their workload project to enable the service to run
* backups and restores there.
* @param serviceAccount serviceAccount or {@code null} for none
*/
public BackupVault setServiceAccount(java.lang.String serviceAccount) {
this.serviceAccount = serviceAccount;
return this;
}
/**
* Output only. The BackupVault resource instance state.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. The BackupVault resource instance state.
* @param state state or {@code null} for none
*/
public BackupVault setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* Output only. Total size of the storage used by all backup resources.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalStoredBytes() {
return totalStoredBytes;
}
/**
* Output only. Total size of the storage used by all backup resources.
* @param totalStoredBytes totalStoredBytes or {@code null} for none
*/
public BackupVault setTotalStoredBytes(java.lang.Long totalStoredBytes) {
this.totalStoredBytes = totalStoredBytes;
return this;
}
/**
* Output only. Output only Immutable after resource creation until resource deletion.
* @return value or {@code null} for none
*/
public java.lang.String getUid() {
return uid;
}
/**
* Output only. Output only Immutable after resource creation until resource deletion.
* @param uid uid or {@code null} for none
*/
public BackupVault setUid(java.lang.String uid) {
this.uid = uid;
return this;
}
/**
* Output only. The time when the instance was updated.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The time when the instance was updated.
* @param updateTime updateTime or {@code null} for none
*/
public BackupVault setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public BackupVault set(String fieldName, Object value) {
return (BackupVault) super.set(fieldName, value);
}
@Override
public BackupVault clone() {
return (BackupVault) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy