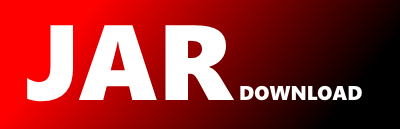
com.google.api.services.backupdr.v1.model.DataSource Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* Message describing a DataSource object. Datasource object used to represent Datasource details
* for both admin and basic view.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DataSource extends com.google.api.client.json.GenericJson {
/**
* Output only. Details of how the resource is configured for backup.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BackupConfigInfo backupConfigInfo;
/**
* Number of backups in the data source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long backupCount;
/**
* Output only. The backup configuration state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String configState;
/**
* Output only. The time when the instance was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* The backed up resource is a backup appliance application.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DataSourceBackupApplianceApplication dataSourceBackupApplianceApplication;
/**
* The backed up resource is a Google Cloud resource. The word 'DataSource' was included in the
* names to indicate that this is the representation of the Google Cloud resource used within the
* DataSource object.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DataSourceGcpResource dataSourceGcpResource;
/**
* Server specified ETag for the ManagementServer resource to prevent simultaneous updates from
* overwiting each other.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* Optional. Resource labels to represent user provided metadata. No labels currently defined:
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* Output only. Identifier. The resource name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Output only. The DataSource resource instance state.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* The number of bytes (metadata and data) stored in this datasource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalStoredBytes;
/**
* Output only. The time when the instance was updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String updateTime;
/**
* Output only. Details of how the resource is configured for backup.
* @return value or {@code null} for none
*/
public BackupConfigInfo getBackupConfigInfo() {
return backupConfigInfo;
}
/**
* Output only. Details of how the resource is configured for backup.
* @param backupConfigInfo backupConfigInfo or {@code null} for none
*/
public DataSource setBackupConfigInfo(BackupConfigInfo backupConfigInfo) {
this.backupConfigInfo = backupConfigInfo;
return this;
}
/**
* Number of backups in the data source.
* @return value or {@code null} for none
*/
public java.lang.Long getBackupCount() {
return backupCount;
}
/**
* Number of backups in the data source.
* @param backupCount backupCount or {@code null} for none
*/
public DataSource setBackupCount(java.lang.Long backupCount) {
this.backupCount = backupCount;
return this;
}
/**
* Output only. The backup configuration state.
* @return value or {@code null} for none
*/
public java.lang.String getConfigState() {
return configState;
}
/**
* Output only. The backup configuration state.
* @param configState configState or {@code null} for none
*/
public DataSource setConfigState(java.lang.String configState) {
this.configState = configState;
return this;
}
/**
* Output only. The time when the instance was created.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Output only. The time when the instance was created.
* @param createTime createTime or {@code null} for none
*/
public DataSource setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* The backed up resource is a backup appliance application.
* @return value or {@code null} for none
*/
public DataSourceBackupApplianceApplication getDataSourceBackupApplianceApplication() {
return dataSourceBackupApplianceApplication;
}
/**
* The backed up resource is a backup appliance application.
* @param dataSourceBackupApplianceApplication dataSourceBackupApplianceApplication or {@code null} for none
*/
public DataSource setDataSourceBackupApplianceApplication(DataSourceBackupApplianceApplication dataSourceBackupApplianceApplication) {
this.dataSourceBackupApplianceApplication = dataSourceBackupApplianceApplication;
return this;
}
/**
* The backed up resource is a Google Cloud resource. The word 'DataSource' was included in the
* names to indicate that this is the representation of the Google Cloud resource used within the
* DataSource object.
* @return value or {@code null} for none
*/
public DataSourceGcpResource getDataSourceGcpResource() {
return dataSourceGcpResource;
}
/**
* The backed up resource is a Google Cloud resource. The word 'DataSource' was included in the
* names to indicate that this is the representation of the Google Cloud resource used within the
* DataSource object.
* @param dataSourceGcpResource dataSourceGcpResource or {@code null} for none
*/
public DataSource setDataSourceGcpResource(DataSourceGcpResource dataSourceGcpResource) {
this.dataSourceGcpResource = dataSourceGcpResource;
return this;
}
/**
* Server specified ETag for the ManagementServer resource to prevent simultaneous updates from
* overwiting each other.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Server specified ETag for the ManagementServer resource to prevent simultaneous updates from
* overwiting each other.
* @param etag etag or {@code null} for none
*/
public DataSource setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* Optional. Resource labels to represent user provided metadata. No labels currently defined:
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* Optional. Resource labels to represent user provided metadata. No labels currently defined:
* @param labels labels or {@code null} for none
*/
public DataSource setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* Output only. Identifier. The resource name.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. Identifier. The resource name.
* @param name name or {@code null} for none
*/
public DataSource setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Output only. The DataSource resource instance state.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* Output only. The DataSource resource instance state.
* @param state state or {@code null} for none
*/
public DataSource setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* The number of bytes (metadata and data) stored in this datasource.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalStoredBytes() {
return totalStoredBytes;
}
/**
* The number of bytes (metadata and data) stored in this datasource.
* @param totalStoredBytes totalStoredBytes or {@code null} for none
*/
public DataSource setTotalStoredBytes(java.lang.Long totalStoredBytes) {
this.totalStoredBytes = totalStoredBytes;
return this;
}
/**
* Output only. The time when the instance was updated.
* @return value or {@code null} for none
*/
public String getUpdateTime() {
return updateTime;
}
/**
* Output only. The time when the instance was updated.
* @param updateTime updateTime or {@code null} for none
*/
public DataSource setUpdateTime(String updateTime) {
this.updateTime = updateTime;
return this;
}
@Override
public DataSource set(String fieldName, Object value) {
return (DataSource) super.set(fieldName, value);
}
@Override
public DataSource clone() {
return (DataSource) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy