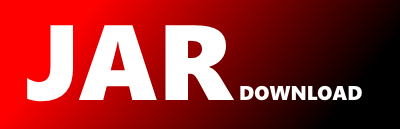
com.google.api.services.backupdr.v1.model.NetworkInterface Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* A network interface resource attached to an instance. s
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class NetworkInterface extends com.google.api.client.json.GenericJson {
/**
* Optional. An array of configurations for this interface. Currently, only one access
* config,ONE_TO_ONE_NAT is supported. If there are no accessConfigs specified, then this instance
* will have no external internet access.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List accessConfigs;
static {
// hack to force ProGuard to consider AccessConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AccessConfig.class);
}
/**
* Optional. An array of alias IP ranges for this network interface. You can only specify this
* field for network interfaces in VPC networks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List aliasIpRanges;
static {
// hack to force ProGuard to consider AliasIpRange used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AliasIpRange.class);
}
/**
* Optional. The prefix length of the primary internal IPv6 range.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer internalIpv6PrefixLength;
/**
* Optional. An array of IPv6 access configurations for this interface. Currently, only one IPv6
* access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this
* instance will have no external IPv6 Internet access.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List ipv6AccessConfigs;
static {
// hack to force ProGuard to consider AccessConfig used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AccessConfig.class);
}
/**
* Optional. [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed
* from the Internet. This field is always inherited from its subnetwork.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ipv6AccessType;
/**
* Optional. An IPv6 internal network address for this network interface. To use a static internal
* IP address, it must be unused and in the same region as the instance's zone. If not specified,
* Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ipv6Address;
/**
* Output only. [Output Only] The name of the network interface, which is generated by the server.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Optional. URL of the VPC network resource for this instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String network;
/**
* Optional. The URL of the network attachment that this interface should connect to in the
* following format:
* projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String networkAttachment;
/**
* Optional. An IPv4 internal IP address to assign to the instance for this network interface. If
* not specified by the user, an unused internal IP is assigned by the system.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String networkIP;
/**
* Optional. The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String nicType;
/**
* Optional. The networking queue count that's specified by users for the network interface. Both
* Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer queueCount;
/**
* The stack type for this network interface.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String stackType;
/**
* Optional. The URL of the Subnetwork resource for this instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String subnetwork;
/**
* Optional. An array of configurations for this interface. Currently, only one access
* config,ONE_TO_ONE_NAT is supported. If there are no accessConfigs specified, then this instance
* will have no external internet access.
* @return value or {@code null} for none
*/
public java.util.List getAccessConfigs() {
return accessConfigs;
}
/**
* Optional. An array of configurations for this interface. Currently, only one access
* config,ONE_TO_ONE_NAT is supported. If there are no accessConfigs specified, then this instance
* will have no external internet access.
* @param accessConfigs accessConfigs or {@code null} for none
*/
public NetworkInterface setAccessConfigs(java.util.List accessConfigs) {
this.accessConfigs = accessConfigs;
return this;
}
/**
* Optional. An array of alias IP ranges for this network interface. You can only specify this
* field for network interfaces in VPC networks.
* @return value or {@code null} for none
*/
public java.util.List getAliasIpRanges() {
return aliasIpRanges;
}
/**
* Optional. An array of alias IP ranges for this network interface. You can only specify this
* field for network interfaces in VPC networks.
* @param aliasIpRanges aliasIpRanges or {@code null} for none
*/
public NetworkInterface setAliasIpRanges(java.util.List aliasIpRanges) {
this.aliasIpRanges = aliasIpRanges;
return this;
}
/**
* Optional. The prefix length of the primary internal IPv6 range.
* @return value or {@code null} for none
*/
public java.lang.Integer getInternalIpv6PrefixLength() {
return internalIpv6PrefixLength;
}
/**
* Optional. The prefix length of the primary internal IPv6 range.
* @param internalIpv6PrefixLength internalIpv6PrefixLength or {@code null} for none
*/
public NetworkInterface setInternalIpv6PrefixLength(java.lang.Integer internalIpv6PrefixLength) {
this.internalIpv6PrefixLength = internalIpv6PrefixLength;
return this;
}
/**
* Optional. An array of IPv6 access configurations for this interface. Currently, only one IPv6
* access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this
* instance will have no external IPv6 Internet access.
* @return value or {@code null} for none
*/
public java.util.List getIpv6AccessConfigs() {
return ipv6AccessConfigs;
}
/**
* Optional. An array of IPv6 access configurations for this interface. Currently, only one IPv6
* access config, DIRECT_IPV6, is supported. If there is no ipv6AccessConfig specified, then this
* instance will have no external IPv6 Internet access.
* @param ipv6AccessConfigs ipv6AccessConfigs or {@code null} for none
*/
public NetworkInterface setIpv6AccessConfigs(java.util.List ipv6AccessConfigs) {
this.ipv6AccessConfigs = ipv6AccessConfigs;
return this;
}
/**
* Optional. [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed
* from the Internet. This field is always inherited from its subnetwork.
* @return value or {@code null} for none
*/
public java.lang.String getIpv6AccessType() {
return ipv6AccessType;
}
/**
* Optional. [Output Only] One of EXTERNAL, INTERNAL to indicate whether the IP can be accessed
* from the Internet. This field is always inherited from its subnetwork.
* @param ipv6AccessType ipv6AccessType or {@code null} for none
*/
public NetworkInterface setIpv6AccessType(java.lang.String ipv6AccessType) {
this.ipv6AccessType = ipv6AccessType;
return this;
}
/**
* Optional. An IPv6 internal network address for this network interface. To use a static internal
* IP address, it must be unused and in the same region as the instance's zone. If not specified,
* Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
* @return value or {@code null} for none
*/
public java.lang.String getIpv6Address() {
return ipv6Address;
}
/**
* Optional. An IPv6 internal network address for this network interface. To use a static internal
* IP address, it must be unused and in the same region as the instance's zone. If not specified,
* Google Cloud will automatically assign an internal IPv6 address from the instance's subnetwork.
* @param ipv6Address ipv6Address or {@code null} for none
*/
public NetworkInterface setIpv6Address(java.lang.String ipv6Address) {
this.ipv6Address = ipv6Address;
return this;
}
/**
* Output only. [Output Only] The name of the network interface, which is generated by the server.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Output only. [Output Only] The name of the network interface, which is generated by the server.
* @param name name or {@code null} for none
*/
public NetworkInterface setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Optional. URL of the VPC network resource for this instance.
* @return value or {@code null} for none
*/
public java.lang.String getNetwork() {
return network;
}
/**
* Optional. URL of the VPC network resource for this instance.
* @param network network or {@code null} for none
*/
public NetworkInterface setNetwork(java.lang.String network) {
this.network = network;
return this;
}
/**
* Optional. The URL of the network attachment that this interface should connect to in the
* following format:
* projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
* @return value or {@code null} for none
*/
public java.lang.String getNetworkAttachment() {
return networkAttachment;
}
/**
* Optional. The URL of the network attachment that this interface should connect to in the
* following format:
* projects/{project_number}/regions/{region_name}/networkAttachments/{network_attachment_name}.
* @param networkAttachment networkAttachment or {@code null} for none
*/
public NetworkInterface setNetworkAttachment(java.lang.String networkAttachment) {
this.networkAttachment = networkAttachment;
return this;
}
/**
* Optional. An IPv4 internal IP address to assign to the instance for this network interface. If
* not specified by the user, an unused internal IP is assigned by the system.
* @return value or {@code null} for none
*/
public java.lang.String getNetworkIP() {
return networkIP;
}
/**
* Optional. An IPv4 internal IP address to assign to the instance for this network interface. If
* not specified by the user, an unused internal IP is assigned by the system.
* @param networkIP networkIP or {@code null} for none
*/
public NetworkInterface setNetworkIP(java.lang.String networkIP) {
this.networkIP = networkIP;
return this;
}
/**
* Optional. The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* @return value or {@code null} for none
*/
public java.lang.String getNicType() {
return nicType;
}
/**
* Optional. The type of vNIC to be used on this interface. This may be gVNIC or VirtioNet.
* @param nicType nicType or {@code null} for none
*/
public NetworkInterface setNicType(java.lang.String nicType) {
this.nicType = nicType;
return this;
}
/**
* Optional. The networking queue count that's specified by users for the network interface. Both
* Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
* @return value or {@code null} for none
*/
public java.lang.Integer getQueueCount() {
return queueCount;
}
/**
* Optional. The networking queue count that's specified by users for the network interface. Both
* Rx and Tx queues will be set to this number. It'll be empty if not specified by the users.
* @param queueCount queueCount or {@code null} for none
*/
public NetworkInterface setQueueCount(java.lang.Integer queueCount) {
this.queueCount = queueCount;
return this;
}
/**
* The stack type for this network interface.
* @return value or {@code null} for none
*/
public java.lang.String getStackType() {
return stackType;
}
/**
* The stack type for this network interface.
* @param stackType stackType or {@code null} for none
*/
public NetworkInterface setStackType(java.lang.String stackType) {
this.stackType = stackType;
return this;
}
/**
* Optional. The URL of the Subnetwork resource for this instance.
* @return value or {@code null} for none
*/
public java.lang.String getSubnetwork() {
return subnetwork;
}
/**
* Optional. The URL of the Subnetwork resource for this instance.
* @param subnetwork subnetwork or {@code null} for none
*/
public NetworkInterface setSubnetwork(java.lang.String subnetwork) {
this.subnetwork = subnetwork;
return this;
}
@Override
public NetworkInterface set(String fieldName, Object value) {
return (NetworkInterface) super.set(fieldName, value);
}
@Override
public NetworkInterface clone() {
return (NetworkInterface) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy