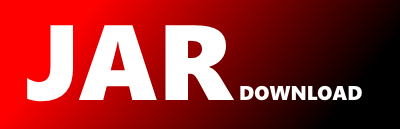
com.google.api.services.backupdr.v1.model.Scheduling Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* Sets the scheduling options for an Instance.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Scheduling extends com.google.api.client.json.GenericJson {
/**
* Optional. Specifies whether the instance should be automatically restarted if it is terminated
* by Compute Engine (not terminated by a user).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean automaticRestart;
/**
* Optional. Specifies the termination action for the instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String instanceTerminationAction;
/**
* Optional. Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the
* Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour
* granularity and the default value being 1 hour.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SchedulingDuration localSsdRecoveryTimeout;
/**
* Optional. The minimum number of virtual CPUs this instance will consume when running on a sole-
* tenant node.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer minNodeCpus;
/**
* Optional. A set of node affinity and anti-affinity configurations. Overrides
* reservationAffinity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List nodeAffinities;
static {
// hack to force ProGuard to consider NodeAffinity used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(NodeAffinity.class);
}
/**
* Optional. Defines the maintenance behavior for this instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String onHostMaintenance;
/**
* Optional. Defines whether the instance is preemptible.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean preemptible;
/**
* Optional. Specifies the provisioning model of the instance.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String provisioningModel;
/**
* Optional. Specifies whether the instance should be automatically restarted if it is terminated
* by Compute Engine (not terminated by a user).
* @return value or {@code null} for none
*/
public java.lang.Boolean getAutomaticRestart() {
return automaticRestart;
}
/**
* Optional. Specifies whether the instance should be automatically restarted if it is terminated
* by Compute Engine (not terminated by a user).
* @param automaticRestart automaticRestart or {@code null} for none
*/
public Scheduling setAutomaticRestart(java.lang.Boolean automaticRestart) {
this.automaticRestart = automaticRestart;
return this;
}
/**
* Optional. Specifies the termination action for the instance.
* @return value or {@code null} for none
*/
public java.lang.String getInstanceTerminationAction() {
return instanceTerminationAction;
}
/**
* Optional. Specifies the termination action for the instance.
* @param instanceTerminationAction instanceTerminationAction or {@code null} for none
*/
public Scheduling setInstanceTerminationAction(java.lang.String instanceTerminationAction) {
this.instanceTerminationAction = instanceTerminationAction;
return this;
}
/**
* Optional. Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the
* Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour
* granularity and the default value being 1 hour.
* @return value or {@code null} for none
*/
public SchedulingDuration getLocalSsdRecoveryTimeout() {
return localSsdRecoveryTimeout;
}
/**
* Optional. Specifies the maximum amount of time a Local Ssd Vm should wait while recovery of the
* Local Ssd state is attempted. Its value should be in between 0 and 168 hours with hour
* granularity and the default value being 1 hour.
* @param localSsdRecoveryTimeout localSsdRecoveryTimeout or {@code null} for none
*/
public Scheduling setLocalSsdRecoveryTimeout(SchedulingDuration localSsdRecoveryTimeout) {
this.localSsdRecoveryTimeout = localSsdRecoveryTimeout;
return this;
}
/**
* Optional. The minimum number of virtual CPUs this instance will consume when running on a sole-
* tenant node.
* @return value or {@code null} for none
*/
public java.lang.Integer getMinNodeCpus() {
return minNodeCpus;
}
/**
* Optional. The minimum number of virtual CPUs this instance will consume when running on a sole-
* tenant node.
* @param minNodeCpus minNodeCpus or {@code null} for none
*/
public Scheduling setMinNodeCpus(java.lang.Integer minNodeCpus) {
this.minNodeCpus = minNodeCpus;
return this;
}
/**
* Optional. A set of node affinity and anti-affinity configurations. Overrides
* reservationAffinity.
* @return value or {@code null} for none
*/
public java.util.List getNodeAffinities() {
return nodeAffinities;
}
/**
* Optional. A set of node affinity and anti-affinity configurations. Overrides
* reservationAffinity.
* @param nodeAffinities nodeAffinities or {@code null} for none
*/
public Scheduling setNodeAffinities(java.util.List nodeAffinities) {
this.nodeAffinities = nodeAffinities;
return this;
}
/**
* Optional. Defines the maintenance behavior for this instance.
* @return value or {@code null} for none
*/
public java.lang.String getOnHostMaintenance() {
return onHostMaintenance;
}
/**
* Optional. Defines the maintenance behavior for this instance.
* @param onHostMaintenance onHostMaintenance or {@code null} for none
*/
public Scheduling setOnHostMaintenance(java.lang.String onHostMaintenance) {
this.onHostMaintenance = onHostMaintenance;
return this;
}
/**
* Optional. Defines whether the instance is preemptible.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPreemptible() {
return preemptible;
}
/**
* Optional. Defines whether the instance is preemptible.
* @param preemptible preemptible or {@code null} for none
*/
public Scheduling setPreemptible(java.lang.Boolean preemptible) {
this.preemptible = preemptible;
return this;
}
/**
* Optional. Specifies the provisioning model of the instance.
* @return value or {@code null} for none
*/
public java.lang.String getProvisioningModel() {
return provisioningModel;
}
/**
* Optional. Specifies the provisioning model of the instance.
* @param provisioningModel provisioningModel or {@code null} for none
*/
public Scheduling setProvisioningModel(java.lang.String provisioningModel) {
this.provisioningModel = provisioningModel;
return this;
}
@Override
public Scheduling set(String fieldName, Object value) {
return (Scheduling) super.set(fieldName, value);
}
@Override
public Scheduling clone() {
return (Scheduling) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy