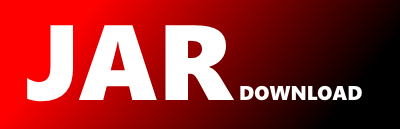
com.google.api.services.backupdr.v1.model.StandardSchedule Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* `StandardSchedule` defines a schedule that run within the confines of a defined window of days.
* We can define recurrence type for schedule as HOURLY, DAILY, WEEKLY, MONTHLY or YEARLY.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class StandardSchedule extends com.google.api.client.json.GenericJson {
/**
* Required. A BackupWindow defines the window of day during which backup jobs will run. Jobs are
* queued at the beginning of the window and will be marked as `NOT_RUN` if they do not start by
* the end of the window. Note: running jobs will not be cancelled at the end of the window.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private BackupWindow backupWindow;
/**
* Optional. Specifies days of months like 1, 5, or 14 on which jobs will run. Values for
* `days_of_month` are only applicable for `recurrence_type`, `MONTHLY` and `YEARLY`. A validation
* error will occur if other values are supplied.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List daysOfMonth;
/**
* Optional. Specifies days of week like, MONDAY or TUESDAY, on which jobs will run. This is
* required for `recurrence_type`, `WEEKLY` and is not applicable otherwise. A validation error
* will occur if a value is supplied and `recurrence_type` is not `WEEKLY`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List daysOfWeek;
/**
* Optional. Specifies frequency for hourly backups. A hourly frequency of 2 means jobs will run
* every 2 hours from start time till end time defined. This is required for `recurrence_type`,
* `HOURLY` and is not applicable otherwise. A validation error will occur if a value is supplied
* and `recurrence_type` is not `HOURLY`. Value of hourly frequency should be between 6 and 23.
* Reason for limit : We found that there is bandwidth limitation of 3GB/S for GMI while taking a
* backup and 5GB/S while doing a restore. Given the amount of parallel backups and restore we are
* targeting, this will potentially take the backup time to mins and hours (in worst case
* scenario).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer hourlyFrequency;
/**
* Optional. Specifies the months of year, like `FEBRUARY` and/or `MAY`, on which jobs will run.
* This field is only applicable when `recurrence_type` is `YEARLY`. A validation error will occur
* if other values are supplied.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List months;
/**
* Required. Specifies the `RecurrenceType` for the schedule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String recurrenceType;
/**
* Required. The time zone to be used when interpreting the schedule. The value of this field must
* be a time zone name from the IANA tz database. See
* https://en.wikipedia.org/wiki/List_of_tz_database_time_zones for the list of valid timezone
* names. For e.g., Europe/Paris.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String timeZone;
/**
* Optional. Specifies a week day of the month like, FIRST SUNDAY or LAST MONDAY, on which jobs
* will run. This will be specified by two fields in `WeekDayOfMonth`, one for the day, e.g.
* `MONDAY`, and one for the week, e.g. `LAST`. This field is only applicable for
* `recurrence_type`, `MONTHLY` and `YEARLY`. A validation error will occur if other values are
* supplied.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private WeekDayOfMonth weekDayOfMonth;
/**
* Required. A BackupWindow defines the window of day during which backup jobs will run. Jobs are
* queued at the beginning of the window and will be marked as `NOT_RUN` if they do not start by
* the end of the window. Note: running jobs will not be cancelled at the end of the window.
* @return value or {@code null} for none
*/
public BackupWindow getBackupWindow() {
return backupWindow;
}
/**
* Required. A BackupWindow defines the window of day during which backup jobs will run. Jobs are
* queued at the beginning of the window and will be marked as `NOT_RUN` if they do not start by
* the end of the window. Note: running jobs will not be cancelled at the end of the window.
* @param backupWindow backupWindow or {@code null} for none
*/
public StandardSchedule setBackupWindow(BackupWindow backupWindow) {
this.backupWindow = backupWindow;
return this;
}
/**
* Optional. Specifies days of months like 1, 5, or 14 on which jobs will run. Values for
* `days_of_month` are only applicable for `recurrence_type`, `MONTHLY` and `YEARLY`. A validation
* error will occur if other values are supplied.
* @return value or {@code null} for none
*/
public java.util.List getDaysOfMonth() {
return daysOfMonth;
}
/**
* Optional. Specifies days of months like 1, 5, or 14 on which jobs will run. Values for
* `days_of_month` are only applicable for `recurrence_type`, `MONTHLY` and `YEARLY`. A validation
* error will occur if other values are supplied.
* @param daysOfMonth daysOfMonth or {@code null} for none
*/
public StandardSchedule setDaysOfMonth(java.util.List daysOfMonth) {
this.daysOfMonth = daysOfMonth;
return this;
}
/**
* Optional. Specifies days of week like, MONDAY or TUESDAY, on which jobs will run. This is
* required for `recurrence_type`, `WEEKLY` and is not applicable otherwise. A validation error
* will occur if a value is supplied and `recurrence_type` is not `WEEKLY`.
* @return value or {@code null} for none
*/
public java.util.List getDaysOfWeek() {
return daysOfWeek;
}
/**
* Optional. Specifies days of week like, MONDAY or TUESDAY, on which jobs will run. This is
* required for `recurrence_type`, `WEEKLY` and is not applicable otherwise. A validation error
* will occur if a value is supplied and `recurrence_type` is not `WEEKLY`.
* @param daysOfWeek daysOfWeek or {@code null} for none
*/
public StandardSchedule setDaysOfWeek(java.util.List daysOfWeek) {
this.daysOfWeek = daysOfWeek;
return this;
}
/**
* Optional. Specifies frequency for hourly backups. A hourly frequency of 2 means jobs will run
* every 2 hours from start time till end time defined. This is required for `recurrence_type`,
* `HOURLY` and is not applicable otherwise. A validation error will occur if a value is supplied
* and `recurrence_type` is not `HOURLY`. Value of hourly frequency should be between 6 and 23.
* Reason for limit : We found that there is bandwidth limitation of 3GB/S for GMI while taking a
* backup and 5GB/S while doing a restore. Given the amount of parallel backups and restore we are
* targeting, this will potentially take the backup time to mins and hours (in worst case
* scenario).
* @return value or {@code null} for none
*/
public java.lang.Integer getHourlyFrequency() {
return hourlyFrequency;
}
/**
* Optional. Specifies frequency for hourly backups. A hourly frequency of 2 means jobs will run
* every 2 hours from start time till end time defined. This is required for `recurrence_type`,
* `HOURLY` and is not applicable otherwise. A validation error will occur if a value is supplied
* and `recurrence_type` is not `HOURLY`. Value of hourly frequency should be between 6 and 23.
* Reason for limit : We found that there is bandwidth limitation of 3GB/S for GMI while taking a
* backup and 5GB/S while doing a restore. Given the amount of parallel backups and restore we are
* targeting, this will potentially take the backup time to mins and hours (in worst case
* scenario).
* @param hourlyFrequency hourlyFrequency or {@code null} for none
*/
public StandardSchedule setHourlyFrequency(java.lang.Integer hourlyFrequency) {
this.hourlyFrequency = hourlyFrequency;
return this;
}
/**
* Optional. Specifies the months of year, like `FEBRUARY` and/or `MAY`, on which jobs will run.
* This field is only applicable when `recurrence_type` is `YEARLY`. A validation error will occur
* if other values are supplied.
* @return value or {@code null} for none
*/
public java.util.List getMonths() {
return months;
}
/**
* Optional. Specifies the months of year, like `FEBRUARY` and/or `MAY`, on which jobs will run.
* This field is only applicable when `recurrence_type` is `YEARLY`. A validation error will occur
* if other values are supplied.
* @param months months or {@code null} for none
*/
public StandardSchedule setMonths(java.util.List months) {
this.months = months;
return this;
}
/**
* Required. Specifies the `RecurrenceType` for the schedule.
* @return value or {@code null} for none
*/
public java.lang.String getRecurrenceType() {
return recurrenceType;
}
/**
* Required. Specifies the `RecurrenceType` for the schedule.
* @param recurrenceType recurrenceType or {@code null} for none
*/
public StandardSchedule setRecurrenceType(java.lang.String recurrenceType) {
this.recurrenceType = recurrenceType;
return this;
}
/**
* Required. The time zone to be used when interpreting the schedule. The value of this field must
* be a time zone name from the IANA tz database. See
* https://en.wikipedia.org/wiki/List_of_tz_database_time_zones for the list of valid timezone
* names. For e.g., Europe/Paris.
* @return value or {@code null} for none
*/
public java.lang.String getTimeZone() {
return timeZone;
}
/**
* Required. The time zone to be used when interpreting the schedule. The value of this field must
* be a time zone name from the IANA tz database. See
* https://en.wikipedia.org/wiki/List_of_tz_database_time_zones for the list of valid timezone
* names. For e.g., Europe/Paris.
* @param timeZone timeZone or {@code null} for none
*/
public StandardSchedule setTimeZone(java.lang.String timeZone) {
this.timeZone = timeZone;
return this;
}
/**
* Optional. Specifies a week day of the month like, FIRST SUNDAY or LAST MONDAY, on which jobs
* will run. This will be specified by two fields in `WeekDayOfMonth`, one for the day, e.g.
* `MONDAY`, and one for the week, e.g. `LAST`. This field is only applicable for
* `recurrence_type`, `MONTHLY` and `YEARLY`. A validation error will occur if other values are
* supplied.
* @return value or {@code null} for none
*/
public WeekDayOfMonth getWeekDayOfMonth() {
return weekDayOfMonth;
}
/**
* Optional. Specifies a week day of the month like, FIRST SUNDAY or LAST MONDAY, on which jobs
* will run. This will be specified by two fields in `WeekDayOfMonth`, one for the day, e.g.
* `MONDAY`, and one for the week, e.g. `LAST`. This field is only applicable for
* `recurrence_type`, `MONTHLY` and `YEARLY`. A validation error will occur if other values are
* supplied.
* @param weekDayOfMonth weekDayOfMonth or {@code null} for none
*/
public StandardSchedule setWeekDayOfMonth(WeekDayOfMonth weekDayOfMonth) {
this.weekDayOfMonth = weekDayOfMonth;
return this;
}
@Override
public StandardSchedule set(String fieldName, Object value) {
return (StandardSchedule) super.set(fieldName, value);
}
@Override
public StandardSchedule clone() {
return (StandardSchedule) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy