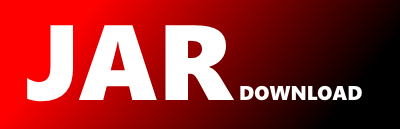
com.google.api.services.backupdr.v1.model.IsolationExpectations Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.backupdr.v1.model;
/**
* Model definition for IsolationExpectations.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup and DR Service API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class IsolationExpectations extends com.google.api.client.json.GenericJson {
/**
* Explicit overrides for ZI and ZS requirements to be used for resources that should be excluded
* from ZI/ZS verification logic.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RequirementOverride requirementOverride;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ziOrgPolicy;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ziRegionPolicy;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ziRegionState;
/**
* Deprecated: use zi_org_policy, zi_region_policy and zi_region_state instead for setting ZI
* expectations as per go/zicy-publish-physical-location.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String zoneIsolation;
/**
* Deprecated: use zs_org_policy, and zs_region_stateinstead for setting Zs expectations as per
* go/zicy-publish-physical-location.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String zoneSeparation;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String zsOrgPolicy;
/**
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String zsRegionState;
/**
* Explicit overrides for ZI and ZS requirements to be used for resources that should be excluded
* from ZI/ZS verification logic.
* @return value or {@code null} for none
*/
public RequirementOverride getRequirementOverride() {
return requirementOverride;
}
/**
* Explicit overrides for ZI and ZS requirements to be used for resources that should be excluded
* from ZI/ZS verification logic.
* @param requirementOverride requirementOverride or {@code null} for none
*/
public IsolationExpectations setRequirementOverride(RequirementOverride requirementOverride) {
this.requirementOverride = requirementOverride;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getZiOrgPolicy() {
return ziOrgPolicy;
}
/**
* @param ziOrgPolicy ziOrgPolicy or {@code null} for none
*/
public IsolationExpectations setZiOrgPolicy(java.lang.String ziOrgPolicy) {
this.ziOrgPolicy = ziOrgPolicy;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getZiRegionPolicy() {
return ziRegionPolicy;
}
/**
* @param ziRegionPolicy ziRegionPolicy or {@code null} for none
*/
public IsolationExpectations setZiRegionPolicy(java.lang.String ziRegionPolicy) {
this.ziRegionPolicy = ziRegionPolicy;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getZiRegionState() {
return ziRegionState;
}
/**
* @param ziRegionState ziRegionState or {@code null} for none
*/
public IsolationExpectations setZiRegionState(java.lang.String ziRegionState) {
this.ziRegionState = ziRegionState;
return this;
}
/**
* Deprecated: use zi_org_policy, zi_region_policy and zi_region_state instead for setting ZI
* expectations as per go/zicy-publish-physical-location.
* @return value or {@code null} for none
*/
public java.lang.String getZoneIsolation() {
return zoneIsolation;
}
/**
* Deprecated: use zi_org_policy, zi_region_policy and zi_region_state instead for setting ZI
* expectations as per go/zicy-publish-physical-location.
* @param zoneIsolation zoneIsolation or {@code null} for none
*/
public IsolationExpectations setZoneIsolation(java.lang.String zoneIsolation) {
this.zoneIsolation = zoneIsolation;
return this;
}
/**
* Deprecated: use zs_org_policy, and zs_region_stateinstead for setting Zs expectations as per
* go/zicy-publish-physical-location.
* @return value or {@code null} for none
*/
public java.lang.String getZoneSeparation() {
return zoneSeparation;
}
/**
* Deprecated: use zs_org_policy, and zs_region_stateinstead for setting Zs expectations as per
* go/zicy-publish-physical-location.
* @param zoneSeparation zoneSeparation or {@code null} for none
*/
public IsolationExpectations setZoneSeparation(java.lang.String zoneSeparation) {
this.zoneSeparation = zoneSeparation;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getZsOrgPolicy() {
return zsOrgPolicy;
}
/**
* @param zsOrgPolicy zsOrgPolicy or {@code null} for none
*/
public IsolationExpectations setZsOrgPolicy(java.lang.String zsOrgPolicy) {
this.zsOrgPolicy = zsOrgPolicy;
return this;
}
/**
* @return value or {@code null} for none
*/
public java.lang.String getZsRegionState() {
return zsRegionState;
}
/**
* @param zsRegionState zsRegionState or {@code null} for none
*/
public IsolationExpectations setZsRegionState(java.lang.String zsRegionState) {
this.zsRegionState = zsRegionState;
return this;
}
@Override
public IsolationExpectations set(String fieldName, Object value) {
return (IsolationExpectations) super.set(fieldName, value);
}
@Override
public IsolationExpectations clone() {
return (IsolationExpectations) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy