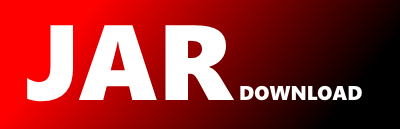
com.google.api.services.batch.v1.model.AgentTaskSpec Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.batch.v1.model;
/**
* AgentTaskSpec is the user's TaskSpec representation between Agent and CLH communication.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Batch API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class AgentTaskSpec extends com.google.api.client.json.GenericJson {
/**
* Environment variables to set before running the Task.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AgentEnvironment environment;
/**
* Logging option for the task.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AgentTaskLoggingOption loggingOption;
/**
* Maximum duration the task should run before being automatically retried (if enabled) or
* automatically failed. Format the value of this field as a time limit in seconds followed by
* `s`—for example, `3600s` for 1 hour. The field accepts any value between 0 and the maximum
* listed for the `Duration` field type at
* https://protobuf.dev/reference/protobuf/google.protobuf/#duration; however, the actual maximum
* run time for a job will be limited to the maximum run time for a job listed at
* https://cloud.google.com/batch/quotas#max-job-duration.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String maxRunDuration;
/**
* AgentTaskRunnable is runanbles that will be executed on the agent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List runnables;
static {
// hack to force ProGuard to consider AgentTaskRunnable used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(AgentTaskRunnable.class);
}
/**
* User account on the VM to run the runnables in the agentTaskSpec. If not set, the runnable will
* be run under root user.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AgentTaskUserAccount userAccount;
/**
* Environment variables to set before running the Task.
* @return value or {@code null} for none
*/
public AgentEnvironment getEnvironment() {
return environment;
}
/**
* Environment variables to set before running the Task.
* @param environment environment or {@code null} for none
*/
public AgentTaskSpec setEnvironment(AgentEnvironment environment) {
this.environment = environment;
return this;
}
/**
* Logging option for the task.
* @return value or {@code null} for none
*/
public AgentTaskLoggingOption getLoggingOption() {
return loggingOption;
}
/**
* Logging option for the task.
* @param loggingOption loggingOption or {@code null} for none
*/
public AgentTaskSpec setLoggingOption(AgentTaskLoggingOption loggingOption) {
this.loggingOption = loggingOption;
return this;
}
/**
* Maximum duration the task should run before being automatically retried (if enabled) or
* automatically failed. Format the value of this field as a time limit in seconds followed by
* `s`—for example, `3600s` for 1 hour. The field accepts any value between 0 and the maximum
* listed for the `Duration` field type at
* https://protobuf.dev/reference/protobuf/google.protobuf/#duration; however, the actual maximum
* run time for a job will be limited to the maximum run time for a job listed at
* https://cloud.google.com/batch/quotas#max-job-duration.
* @return value or {@code null} for none
*/
public String getMaxRunDuration() {
return maxRunDuration;
}
/**
* Maximum duration the task should run before being automatically retried (if enabled) or
* automatically failed. Format the value of this field as a time limit in seconds followed by
* `s`—for example, `3600s` for 1 hour. The field accepts any value between 0 and the maximum
* listed for the `Duration` field type at
* https://protobuf.dev/reference/protobuf/google.protobuf/#duration; however, the actual maximum
* run time for a job will be limited to the maximum run time for a job listed at
* https://cloud.google.com/batch/quotas#max-job-duration.
* @param maxRunDuration maxRunDuration or {@code null} for none
*/
public AgentTaskSpec setMaxRunDuration(String maxRunDuration) {
this.maxRunDuration = maxRunDuration;
return this;
}
/**
* AgentTaskRunnable is runanbles that will be executed on the agent.
* @return value or {@code null} for none
*/
public java.util.List getRunnables() {
return runnables;
}
/**
* AgentTaskRunnable is runanbles that will be executed on the agent.
* @param runnables runnables or {@code null} for none
*/
public AgentTaskSpec setRunnables(java.util.List runnables) {
this.runnables = runnables;
return this;
}
/**
* User account on the VM to run the runnables in the agentTaskSpec. If not set, the runnable will
* be run under root user.
* @return value or {@code null} for none
*/
public AgentTaskUserAccount getUserAccount() {
return userAccount;
}
/**
* User account on the VM to run the runnables in the agentTaskSpec. If not set, the runnable will
* be run under root user.
* @param userAccount userAccount or {@code null} for none
*/
public AgentTaskSpec setUserAccount(AgentTaskUserAccount userAccount) {
this.userAccount = userAccount;
return this;
}
@Override
public AgentTaskSpec set(String fieldName, Object value) {
return (AgentTaskSpec) super.set(fieldName, value);
}
@Override
public AgentTaskSpec clone() {
return (AgentTaskSpec) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy