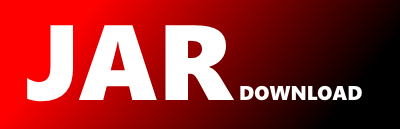
com.google.api.services.batch.v1.model.InstancePolicyOrTemplate Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.batch.v1.model;
/**
* InstancePolicyOrTemplate lets you define the type of resources to use for this job either with an
* InstancePolicy or an instance template. If undefined, Batch picks the type of VM to use and
* doesn't include optional VM resources such as GPUs and extra disks.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Batch API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class InstancePolicyOrTemplate extends com.google.api.client.json.GenericJson {
/**
* Optional. Set this field to `true` if you want Batch to block project-level SSH keys from
* accessing this job's VMs. Alternatively, you can configure the job to specify a VM instance
* template that blocks project-level SSH keys. In either case, Batch blocks project-level SSH
* keys while creating the VMs for this job. Batch allows project-level SSH keys for a job's VMs
* only if all the following are true: + This field is undefined or set to `false`. + The job's VM
* instance template (if any) doesn't block project-level SSH keys. Notably, you can override this
* behavior by manually updating a VM to block or allow project-level SSH keys. For more
* information about blocking project-level SSH keys, see the Compute Engine documentation:
* https://cloud.google.com/compute/docs/connect/restrict-ssh-keys#block-keys
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean blockProjectSshKeys;
/**
* Set this field true if you want Batch to help fetch drivers from a third party location and
* install them for GPUs specified in `policy.accelerators` or `instance_template` on your behalf.
* Default is false. For Container-Optimized Image cases, Batch will install the accelerator
* driver following milestones of https://cloud.google.com/container-optimized-os/docs/release-
* notes. For non Container-Optimized Image cases, following
* https://github.com/GoogleCloudPlatform/compute-gpu-
* installation/blob/main/linux/install_gpu_driver.py.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean installGpuDrivers;
/**
* Optional. Set this field true if you want Batch to install Ops Agent on your behalf. Default is
* false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean installOpsAgent;
/**
* Name of an instance template used to create VMs. Named the field as 'instance_template' instead
* of 'template' to avoid C++ keyword conflict. Batch only supports global instance templates from
* the same project as the job. You can specify the global instance template as a full or partial
* URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String instanceTemplate;
/**
* InstancePolicy.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private InstancePolicy policy;
/**
* Optional. Set this field to `true` if you want Batch to block project-level SSH keys from
* accessing this job's VMs. Alternatively, you can configure the job to specify a VM instance
* template that blocks project-level SSH keys. In either case, Batch blocks project-level SSH
* keys while creating the VMs for this job. Batch allows project-level SSH keys for a job's VMs
* only if all the following are true: + This field is undefined or set to `false`. + The job's VM
* instance template (if any) doesn't block project-level SSH keys. Notably, you can override this
* behavior by manually updating a VM to block or allow project-level SSH keys. For more
* information about blocking project-level SSH keys, see the Compute Engine documentation:
* https://cloud.google.com/compute/docs/connect/restrict-ssh-keys#block-keys
* @return value or {@code null} for none
*/
public java.lang.Boolean getBlockProjectSshKeys() {
return blockProjectSshKeys;
}
/**
* Optional. Set this field to `true` if you want Batch to block project-level SSH keys from
* accessing this job's VMs. Alternatively, you can configure the job to specify a VM instance
* template that blocks project-level SSH keys. In either case, Batch blocks project-level SSH
* keys while creating the VMs for this job. Batch allows project-level SSH keys for a job's VMs
* only if all the following are true: + This field is undefined or set to `false`. + The job's VM
* instance template (if any) doesn't block project-level SSH keys. Notably, you can override this
* behavior by manually updating a VM to block or allow project-level SSH keys. For more
* information about blocking project-level SSH keys, see the Compute Engine documentation:
* https://cloud.google.com/compute/docs/connect/restrict-ssh-keys#block-keys
* @param blockProjectSshKeys blockProjectSshKeys or {@code null} for none
*/
public InstancePolicyOrTemplate setBlockProjectSshKeys(java.lang.Boolean blockProjectSshKeys) {
this.blockProjectSshKeys = blockProjectSshKeys;
return this;
}
/**
* Set this field true if you want Batch to help fetch drivers from a third party location and
* install them for GPUs specified in `policy.accelerators` or `instance_template` on your behalf.
* Default is false. For Container-Optimized Image cases, Batch will install the accelerator
* driver following milestones of https://cloud.google.com/container-optimized-os/docs/release-
* notes. For non Container-Optimized Image cases, following
* https://github.com/GoogleCloudPlatform/compute-gpu-
* installation/blob/main/linux/install_gpu_driver.py.
* @return value or {@code null} for none
*/
public java.lang.Boolean getInstallGpuDrivers() {
return installGpuDrivers;
}
/**
* Set this field true if you want Batch to help fetch drivers from a third party location and
* install them for GPUs specified in `policy.accelerators` or `instance_template` on your behalf.
* Default is false. For Container-Optimized Image cases, Batch will install the accelerator
* driver following milestones of https://cloud.google.com/container-optimized-os/docs/release-
* notes. For non Container-Optimized Image cases, following
* https://github.com/GoogleCloudPlatform/compute-gpu-
* installation/blob/main/linux/install_gpu_driver.py.
* @param installGpuDrivers installGpuDrivers or {@code null} for none
*/
public InstancePolicyOrTemplate setInstallGpuDrivers(java.lang.Boolean installGpuDrivers) {
this.installGpuDrivers = installGpuDrivers;
return this;
}
/**
* Optional. Set this field true if you want Batch to install Ops Agent on your behalf. Default is
* false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getInstallOpsAgent() {
return installOpsAgent;
}
/**
* Optional. Set this field true if you want Batch to install Ops Agent on your behalf. Default is
* false.
* @param installOpsAgent installOpsAgent or {@code null} for none
*/
public InstancePolicyOrTemplate setInstallOpsAgent(java.lang.Boolean installOpsAgent) {
this.installOpsAgent = installOpsAgent;
return this;
}
/**
* Name of an instance template used to create VMs. Named the field as 'instance_template' instead
* of 'template' to avoid C++ keyword conflict. Batch only supports global instance templates from
* the same project as the job. You can specify the global instance template as a full or partial
* URL.
* @return value or {@code null} for none
*/
public java.lang.String getInstanceTemplate() {
return instanceTemplate;
}
/**
* Name of an instance template used to create VMs. Named the field as 'instance_template' instead
* of 'template' to avoid C++ keyword conflict. Batch only supports global instance templates from
* the same project as the job. You can specify the global instance template as a full or partial
* URL.
* @param instanceTemplate instanceTemplate or {@code null} for none
*/
public InstancePolicyOrTemplate setInstanceTemplate(java.lang.String instanceTemplate) {
this.instanceTemplate = instanceTemplate;
return this;
}
/**
* InstancePolicy.
* @return value or {@code null} for none
*/
public InstancePolicy getPolicy() {
return policy;
}
/**
* InstancePolicy.
* @param policy policy or {@code null} for none
*/
public InstancePolicyOrTemplate setPolicy(InstancePolicy policy) {
this.policy = policy;
return this;
}
@Override
public InstancePolicyOrTemplate set(String fieldName, Object value) {
return (InstancePolicyOrTemplate) super.set(fieldName, value);
}
@Override
public InstancePolicyOrTemplate clone() {
return (InstancePolicyOrTemplate) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy