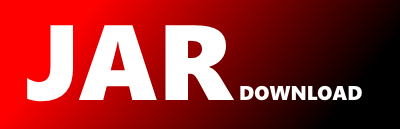
com.google.api.services.bigquery.model.QueryRequest Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2014-04-15 19:10:39 UTC)
* on 2014-04-29 at 15:08:55 UTC
* Modify at your own risk.
*/
package com.google.api.services.bigquery.model;
/**
* Model definition for QueryRequest.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class QueryRequest extends com.google.api.client.json.GenericJson {
/**
* [Optional] Specifies the default datasetId and projectId to assume for any unqualified table
* names in the query. If not set, all table names in the query string must be qualified in the
* format 'datasetId.tableId'.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DatasetReference defaultDataset;
/**
* [Optional] If set, don't actually run the query. A valid query will return an empty response,
* while an invalid query will return the same error it would if it wasn't a dry run. The default
* value is false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean dryRun;
/**
* The resource type of the request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* [Optional] The maximum number of rows of data to return per page of results. Setting this flag
* to a small value such as 1000 and then paging through results might improve reliability when
* the query result set is large. In addition to this limit, responses are also limited to 10 MB.
* By default, there is no maximum row count, and only the byte limit applies.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/**
* [Deprecated] If set to false, maps null values in the query response to the column's default
* value. Only specify if you have older code that can not handle null values in the query
* response. The default value is true. This flag is deprecated and will be ignored in a future
* version of BigQuery.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean preserveNulls;
/**
* [Required] A query string, following the BigQuery query syntax, of the query to execute.
* Example: "SELECT count(f1) FROM [myProjectId:myDatasetId.myTableId]".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String query;
/**
* [Optional] How long to wait for the query to complete, in milliseconds, before the request
* times out and returns. Note that this is only a timeout for the request, not the query. If the
* query takes longer to run than the timeout value, the call returns without any results and with
* the 'jobComplete' flag set to false. You can call GetQueryResults() to wait for the query to
* complete and read the results. The default value is 10000 milliseconds (10 seconds).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Long timeoutMs;
/**
* [Optional] Whether to look for the result in the query cache. The query cache is a best-effort
* cache that will be flushed whenever tables in the query are modified. The default value is
* true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useQueryCache;
/**
* [Optional] Specifies the default datasetId and projectId to assume for any unqualified table
* names in the query. If not set, all table names in the query string must be qualified in the
* format 'datasetId.tableId'.
* @return value or {@code null} for none
*/
public DatasetReference getDefaultDataset() {
return defaultDataset;
}
/**
* [Optional] Specifies the default datasetId and projectId to assume for any unqualified table
* names in the query. If not set, all table names in the query string must be qualified in the
* format 'datasetId.tableId'.
* @param defaultDataset defaultDataset or {@code null} for none
*/
public QueryRequest setDefaultDataset(DatasetReference defaultDataset) {
this.defaultDataset = defaultDataset;
return this;
}
/**
* [Optional] If set, don't actually run the query. A valid query will return an empty response,
* while an invalid query will return the same error it would if it wasn't a dry run. The default
* value is false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDryRun() {
return dryRun;
}
/**
* [Optional] If set, don't actually run the query. A valid query will return an empty response,
* while an invalid query will return the same error it would if it wasn't a dry run. The default
* value is false.
* @param dryRun dryRun or {@code null} for none
*/
public QueryRequest setDryRun(java.lang.Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
/**
* The resource type of the request.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* The resource type of the request.
* @param kind kind or {@code null} for none
*/
public QueryRequest setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* [Optional] The maximum number of rows of data to return per page of results. Setting this flag
* to a small value such as 1000 and then paging through results might improve reliability when
* the query result set is large. In addition to this limit, responses are also limited to 10 MB.
* By default, there is no maximum row count, and only the byte limit applies.
* @return value or {@code null} for none
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/**
* [Optional] The maximum number of rows of data to return per page of results. Setting this flag
* to a small value such as 1000 and then paging through results might improve reliability when
* the query result set is large. In addition to this limit, responses are also limited to 10 MB.
* By default, there is no maximum row count, and only the byte limit applies.
* @param maxResults maxResults or {@code null} for none
*/
public QueryRequest setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/**
* [Deprecated] If set to false, maps null values in the query response to the column's default
* value. Only specify if you have older code that can not handle null values in the query
* response. The default value is true. This flag is deprecated and will be ignored in a future
* version of BigQuery.
* @return value or {@code null} for none
*/
public java.lang.Boolean getPreserveNulls() {
return preserveNulls;
}
/**
* [Deprecated] If set to false, maps null values in the query response to the column's default
* value. Only specify if you have older code that can not handle null values in the query
* response. The default value is true. This flag is deprecated and will be ignored in a future
* version of BigQuery.
* @param preserveNulls preserveNulls or {@code null} for none
*/
public QueryRequest setPreserveNulls(java.lang.Boolean preserveNulls) {
this.preserveNulls = preserveNulls;
return this;
}
/**
* [Required] A query string, following the BigQuery query syntax, of the query to execute.
* Example: "SELECT count(f1) FROM [myProjectId:myDatasetId.myTableId]".
* @return value or {@code null} for none
*/
public java.lang.String getQuery() {
return query;
}
/**
* [Required] A query string, following the BigQuery query syntax, of the query to execute.
* Example: "SELECT count(f1) FROM [myProjectId:myDatasetId.myTableId]".
* @param query query or {@code null} for none
*/
public QueryRequest setQuery(java.lang.String query) {
this.query = query;
return this;
}
/**
* [Optional] How long to wait for the query to complete, in milliseconds, before the request
* times out and returns. Note that this is only a timeout for the request, not the query. If the
* query takes longer to run than the timeout value, the call returns without any results and with
* the 'jobComplete' flag set to false. You can call GetQueryResults() to wait for the query to
* complete and read the results. The default value is 10000 milliseconds (10 seconds).
* @return value or {@code null} for none
*/
public java.lang.Long getTimeoutMs() {
return timeoutMs;
}
/**
* [Optional] How long to wait for the query to complete, in milliseconds, before the request
* times out and returns. Note that this is only a timeout for the request, not the query. If the
* query takes longer to run than the timeout value, the call returns without any results and with
* the 'jobComplete' flag set to false. You can call GetQueryResults() to wait for the query to
* complete and read the results. The default value is 10000 milliseconds (10 seconds).
* @param timeoutMs timeoutMs or {@code null} for none
*/
public QueryRequest setTimeoutMs(java.lang.Long timeoutMs) {
this.timeoutMs = timeoutMs;
return this;
}
/**
* [Optional] Whether to look for the result in the query cache. The query cache is a best-effort
* cache that will be flushed whenever tables in the query are modified. The default value is
* true.
* @return value or {@code null} for none
*/
public java.lang.Boolean getUseQueryCache() {
return useQueryCache;
}
/**
* [Optional] Whether to look for the result in the query cache. The query cache is a best-effort
* cache that will be flushed whenever tables in the query are modified. The default value is
* true.
* @param useQueryCache useQueryCache or {@code null} for none
*/
public QueryRequest setUseQueryCache(java.lang.Boolean useQueryCache) {
this.useQueryCache = useQueryCache;
return this;
}
@Override
public QueryRequest set(String fieldName, Object value) {
return (QueryRequest) super.set(fieldName, value);
}
@Override
public QueryRequest clone() {
return (QueryRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy