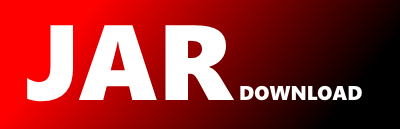
com.google.api.services.bigquery.model.Dataset Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2014-06-09 16:41:44 UTC)
* on 2014-07-02 at 14:03:09 UTC
* Modify at your own risk.
*/
package com.google.api.services.bigquery.model;
/**
* Model definition for Dataset.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
* http://code.google.com/p/google-api-java-client/wiki/Json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Dataset extends com.google.api.client.json.GenericJson {
/**
* [Optional] An array of objects that define dataset access for one or more entities. You can set
* this property when inserting or updating a dataset in order to control who is allowed to access
* the data. If unspecified at dataset creation time, BigQuery adds default dataset access for the
* following entities: access.specialGroup: projectReaders; access.role: READER;
* access.specialGroup: projectWriters; access.role: WRITER; access.specialGroup: projectOwners;
* access.role: OWNER; access.userByEmail: [dataset creator email]; access.role: OWNER;
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List access;
static {
// hack to force ProGuard to consider Access used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(Access.class);
}
/**
* [Output-only] The time when this dataset was created, in milliseconds since the epoch.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long creationTime;
/**
* [Required] A reference that identifies the dataset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DatasetReference datasetReference;
/**
* [Optional] A user-friendly description of the dataset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* [Output-only] A hash of the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/**
* [Optional] A descriptive name for the dataset.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String friendlyName;
/**
* [Output-only] The fully-qualified unique name of the dataset in the format projectId:datasetId.
* The dataset name without the project name is given in the datasetId field. When creating a new
* dataset, leave this field blank, and instead specify the datasetId field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* [Output-only] The resource type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* [Output-only] The date when this dataset or any of its tables was last modified, in
* milliseconds since the epoch.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long lastModifiedTime;
/**
* [Output-only] A URL that can be used to access the resource again. You can use this URL in Get
* or Update requests to the resource.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String selfLink;
/**
* [Optional] An array of objects that define dataset access for one or more entities. You can set
* this property when inserting or updating a dataset in order to control who is allowed to access
* the data. If unspecified at dataset creation time, BigQuery adds default dataset access for the
* following entities: access.specialGroup: projectReaders; access.role: READER;
* access.specialGroup: projectWriters; access.role: WRITER; access.specialGroup: projectOwners;
* access.role: OWNER; access.userByEmail: [dataset creator email]; access.role: OWNER;
* @return value or {@code null} for none
*/
public java.util.List getAccess() {
return access;
}
/**
* [Optional] An array of objects that define dataset access for one or more entities. You can set
* this property when inserting or updating a dataset in order to control who is allowed to access
* the data. If unspecified at dataset creation time, BigQuery adds default dataset access for the
* following entities: access.specialGroup: projectReaders; access.role: READER;
* access.specialGroup: projectWriters; access.role: WRITER; access.specialGroup: projectOwners;
* access.role: OWNER; access.userByEmail: [dataset creator email]; access.role: OWNER;
* @param access access or {@code null} for none
*/
public Dataset setAccess(java.util.List access) {
this.access = access;
return this;
}
/**
* [Output-only] The time when this dataset was created, in milliseconds since the epoch.
* @return value or {@code null} for none
*/
public java.lang.Long getCreationTime() {
return creationTime;
}
/**
* [Output-only] The time when this dataset was created, in milliseconds since the epoch.
* @param creationTime creationTime or {@code null} for none
*/
public Dataset setCreationTime(java.lang.Long creationTime) {
this.creationTime = creationTime;
return this;
}
/**
* [Required] A reference that identifies the dataset.
* @return value or {@code null} for none
*/
public DatasetReference getDatasetReference() {
return datasetReference;
}
/**
* [Required] A reference that identifies the dataset.
* @param datasetReference datasetReference or {@code null} for none
*/
public Dataset setDatasetReference(DatasetReference datasetReference) {
this.datasetReference = datasetReference;
return this;
}
/**
* [Optional] A user-friendly description of the dataset.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* [Optional] A user-friendly description of the dataset.
* @param description description or {@code null} for none
*/
public Dataset setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* [Output-only] A hash of the resource.
* @return value or {@code null} for none
*/
public java.lang.String getEtag() {
return etag;
}
/**
* [Output-only] A hash of the resource.
* @param etag etag or {@code null} for none
*/
public Dataset setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
/**
* [Optional] A descriptive name for the dataset.
* @return value or {@code null} for none
*/
public java.lang.String getFriendlyName() {
return friendlyName;
}
/**
* [Optional] A descriptive name for the dataset.
* @param friendlyName friendlyName or {@code null} for none
*/
public Dataset setFriendlyName(java.lang.String friendlyName) {
this.friendlyName = friendlyName;
return this;
}
/**
* [Output-only] The fully-qualified unique name of the dataset in the format projectId:datasetId.
* The dataset name without the project name is given in the datasetId field. When creating a new
* dataset, leave this field blank, and instead specify the datasetId field.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* [Output-only] The fully-qualified unique name of the dataset in the format projectId:datasetId.
* The dataset name without the project name is given in the datasetId field. When creating a new
* dataset, leave this field blank, and instead specify the datasetId field.
* @param id id or {@code null} for none
*/
public Dataset setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* [Output-only] The resource type.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* [Output-only] The resource type.
* @param kind kind or {@code null} for none
*/
public Dataset setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* [Output-only] The date when this dataset or any of its tables was last modified, in
* milliseconds since the epoch.
* @return value or {@code null} for none
*/
public java.lang.Long getLastModifiedTime() {
return lastModifiedTime;
}
/**
* [Output-only] The date when this dataset or any of its tables was last modified, in
* milliseconds since the epoch.
* @param lastModifiedTime lastModifiedTime or {@code null} for none
*/
public Dataset setLastModifiedTime(java.lang.Long lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
return this;
}
/**
* [Output-only] A URL that can be used to access the resource again. You can use this URL in Get
* or Update requests to the resource.
* @return value or {@code null} for none
*/
public java.lang.String getSelfLink() {
return selfLink;
}
/**
* [Output-only] A URL that can be used to access the resource again. You can use this URL in Get
* or Update requests to the resource.
* @param selfLink selfLink or {@code null} for none
*/
public Dataset setSelfLink(java.lang.String selfLink) {
this.selfLink = selfLink;
return this;
}
@Override
public Dataset set(String fieldName, Object value) {
return (Dataset) super.set(fieldName, value);
}
@Override
public Dataset clone() {
return (Dataset) super.clone();
}
/**
* Model definition for DatasetAccess.
*/
public static final class Access extends com.google.api.client.json.GenericJson {
/**
* [Pick one] A domain to grant access to. Any users signed in with the domain specified will be
* granted the specified access. Example: "example.com".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String domain;
/**
* [Pick one] An email address of a Google Group to grant access to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String groupByEmail;
/**
* [Required] Describes the rights granted to the user specified by the other member of the access
* object. The following string values are supported: READER, WRITER, OWNER.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String role;
/**
* [Pick one] A special group to grant access to. Possible values include: projectOwners: Owners
* of the enclosing project. projectReaders: Readers of the enclosing project. projectWriters:
* Writers of the enclosing project. allAuthenticatedUsers: All authenticated BigQuery users.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String specialGroup;
/**
* [Pick one] An email address of a user to grant access to. For example: [email protected].
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String userByEmail;
/**
* [Pick one] A domain to grant access to. Any users signed in with the domain specified will be
* granted the specified access. Example: "example.com".
* @return value or {@code null} for none
*/
public java.lang.String getDomain() {
return domain;
}
/**
* [Pick one] A domain to grant access to. Any users signed in with the domain specified will be
* granted the specified access. Example: "example.com".
* @param domain domain or {@code null} for none
*/
public Access setDomain(java.lang.String domain) {
this.domain = domain;
return this;
}
/**
* [Pick one] An email address of a Google Group to grant access to.
* @return value or {@code null} for none
*/
public java.lang.String getGroupByEmail() {
return groupByEmail;
}
/**
* [Pick one] An email address of a Google Group to grant access to.
* @param groupByEmail groupByEmail or {@code null} for none
*/
public Access setGroupByEmail(java.lang.String groupByEmail) {
this.groupByEmail = groupByEmail;
return this;
}
/**
* [Required] Describes the rights granted to the user specified by the other member of the access
* object. The following string values are supported: READER, WRITER, OWNER.
* @return value or {@code null} for none
*/
public java.lang.String getRole() {
return role;
}
/**
* [Required] Describes the rights granted to the user specified by the other member of the access
* object. The following string values are supported: READER, WRITER, OWNER.
* @param role role or {@code null} for none
*/
public Access setRole(java.lang.String role) {
this.role = role;
return this;
}
/**
* [Pick one] A special group to grant access to. Possible values include: projectOwners: Owners
* of the enclosing project. projectReaders: Readers of the enclosing project. projectWriters:
* Writers of the enclosing project. allAuthenticatedUsers: All authenticated BigQuery users.
* @return value or {@code null} for none
*/
public java.lang.String getSpecialGroup() {
return specialGroup;
}
/**
* [Pick one] A special group to grant access to. Possible values include: projectOwners: Owners
* of the enclosing project. projectReaders: Readers of the enclosing project. projectWriters:
* Writers of the enclosing project. allAuthenticatedUsers: All authenticated BigQuery users.
* @param specialGroup specialGroup or {@code null} for none
*/
public Access setSpecialGroup(java.lang.String specialGroup) {
this.specialGroup = specialGroup;
return this;
}
/**
* [Pick one] An email address of a user to grant access to. For example: [email protected].
* @return value or {@code null} for none
*/
public java.lang.String getUserByEmail() {
return userByEmail;
}
/**
* [Pick one] An email address of a user to grant access to. For example: [email protected].
* @param userByEmail userByEmail or {@code null} for none
*/
public Access setUserByEmail(java.lang.String userByEmail) {
this.userByEmail = userByEmail;
return this;
}
@Override
public Access set(String fieldName, Object value) {
return (Access) super.set(fieldName, value);
}
@Override
public Access clone() {
return (Access) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy