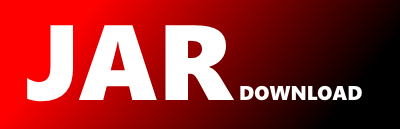
com.google.api.services.bigquery.model.JobConfigurationLoad Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2014-10-28 17:08:27 UTC)
* on 2014-11-14 at 19:51:40 UTC
* Modify at your own risk.
*/
package com.google.api.services.bigquery.model;
/**
* Model definition for JobConfigurationLoad.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class JobConfigurationLoad extends com.google.api.client.json.GenericJson {
/**
* [Optional] Accept rows that are missing trailing optional columns. The missing values are
* treated as nulls. Default is false which treats short rows as errors. Only applicable to CSV,
* ignored for other formats.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowJaggedRows;
/**
* Indicates if BigQuery should allow quoted data sections that contain newline characters in a
* CSV file. The default value is false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowQuotedNewlines;
/**
* [Optional] Specifies whether the job is allowed to create new tables. The following values are
* supported: CREATE_IF_NEEDED: If the table does not exist, BigQuery creates the table.
* CREATE_NEVER: The table must already exist. If it does not, a 'notFound' error is returned in
* the job result. The default value is CREATE_IF_NEEDED. Creation, truncation and append actions
* occur as one atomic update upon job completion.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String createDisposition;
/**
* [Required] The destination table to load the data into.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TableReference destinationTable;
/**
* [Optional] The character encoding of the data. The supported values are UTF-8 or ISO-8859-1.
* The default value is UTF-8. BigQuery decodes the data after the raw, binary data has been split
* using the values of the quote and fieldDelimiter properties.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String encoding;
/**
* [Optional] The separator for fields in a CSV file. BigQuery converts the string to ISO-8859-1
* encoding, and then uses the first byte of the encoded string to split the data in its raw,
* binary state. BigQuery also supports the escape sequence "\t" to specify a tab separator. The
* default value is a comma (',').
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fieldDelimiter;
/**
* [Optional] Accept rows that contain values that do not match the schema. The unknown values are
* ignored. Default is false which treats unknown values as errors. For CSV this ignores extra
* values at the end of a line. For JSON this ignores named values that do not match any column
* name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean ignoreUnknownValues;
/**
* [Optional] The maximum number of bad records that BigQuery can ignore when running the job. If
* the number of bad records exceeds this value, an 'invalid' error is returned in the job result
* and the job fails. The default value is 0, which requires that all records are valid.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxBadRecords;
/**
* [Optional] The value that is used to quote data sections in a CSV file. BigQuery converts the
* string to ISO-8859-1 encoding, and then uses the first byte of the encoded string to split the
* data in its raw, binary state. The default value is a double-quote ('"'). If your data does not
* contain quoted sections, set the property value to an empty string. If your data contains
* quoted newline characters, you must also set the allowQuotedNewlines property to true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String quote;
/**
* [Optional] The schema for the destination table. The schema can be omitted if the destination
* table already exists or if the schema can be inferred from the loaded data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TableSchema schema;
/**
* [Deprecated] The inline schema. For CSV schemas, specify as "Field1:Type1[,Field2:Type2]*". For
* example, "foo:STRING, bar:INTEGER, baz:FLOAT".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String schemaInline;
/**
* [Deprecated] The format of the schemaInline property.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String schemaInlineFormat;
/**
* [Optional] The number of rows at the top of a CSV file that BigQuery will skip when loading the
* data. The default value is 0. This property is useful if you have header rows in the file that
* should be skipped.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer skipLeadingRows;
/**
* [Optional] The format of the data files. For CSV files, specify "CSV". For datastore backups,
* specify "DATASTORE_BACKUP". For newline-delimited JSON, specify "NEWLINE_DELIMITED_JSON". The
* default value is CSV.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sourceFormat;
/**
* [Required] The fully-qualified URIs that point to your data in Google Cloud Storage. Wildcard
* names are only supported when they appear at the end of the URI.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List sourceUris;
/**
* [Optional] Specifies the action that occurs if the destination table already exists. The
* following values are supported: WRITE_TRUNCATE: If the table already exists, BigQuery
* overwrites the table data. WRITE_APPEND: If the table already exists, BigQuery appends the data
* to the table. WRITE_EMPTY: If the table already exists and contains data, a 'duplicate' error
* is returned in the job result. The default value is WRITE_EMPTY. Each action is atomic and only
* occurs if BigQuery is able to complete the job successfully. Creation, truncation and append
* actions occur as one atomic update upon job completion.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String writeDisposition;
/**
* [Optional] Accept rows that are missing trailing optional columns. The missing values are
* treated as nulls. Default is false which treats short rows as errors. Only applicable to CSV,
* ignored for other formats.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllowJaggedRows() {
return allowJaggedRows;
}
/**
* [Optional] Accept rows that are missing trailing optional columns. The missing values are
* treated as nulls. Default is false which treats short rows as errors. Only applicable to CSV,
* ignored for other formats.
* @param allowJaggedRows allowJaggedRows or {@code null} for none
*/
public JobConfigurationLoad setAllowJaggedRows(java.lang.Boolean allowJaggedRows) {
this.allowJaggedRows = allowJaggedRows;
return this;
}
/**
* Indicates if BigQuery should allow quoted data sections that contain newline characters in a
* CSV file. The default value is false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllowQuotedNewlines() {
return allowQuotedNewlines;
}
/**
* Indicates if BigQuery should allow quoted data sections that contain newline characters in a
* CSV file. The default value is false.
* @param allowQuotedNewlines allowQuotedNewlines or {@code null} for none
*/
public JobConfigurationLoad setAllowQuotedNewlines(java.lang.Boolean allowQuotedNewlines) {
this.allowQuotedNewlines = allowQuotedNewlines;
return this;
}
/**
* [Optional] Specifies whether the job is allowed to create new tables. The following values are
* supported: CREATE_IF_NEEDED: If the table does not exist, BigQuery creates the table.
* CREATE_NEVER: The table must already exist. If it does not, a 'notFound' error is returned in
* the job result. The default value is CREATE_IF_NEEDED. Creation, truncation and append actions
* occur as one atomic update upon job completion.
* @return value or {@code null} for none
*/
public java.lang.String getCreateDisposition() {
return createDisposition;
}
/**
* [Optional] Specifies whether the job is allowed to create new tables. The following values are
* supported: CREATE_IF_NEEDED: If the table does not exist, BigQuery creates the table.
* CREATE_NEVER: The table must already exist. If it does not, a 'notFound' error is returned in
* the job result. The default value is CREATE_IF_NEEDED. Creation, truncation and append actions
* occur as one atomic update upon job completion.
* @param createDisposition createDisposition or {@code null} for none
*/
public JobConfigurationLoad setCreateDisposition(java.lang.String createDisposition) {
this.createDisposition = createDisposition;
return this;
}
/**
* [Required] The destination table to load the data into.
* @return value or {@code null} for none
*/
public TableReference getDestinationTable() {
return destinationTable;
}
/**
* [Required] The destination table to load the data into.
* @param destinationTable destinationTable or {@code null} for none
*/
public JobConfigurationLoad setDestinationTable(TableReference destinationTable) {
this.destinationTable = destinationTable;
return this;
}
/**
* [Optional] The character encoding of the data. The supported values are UTF-8 or ISO-8859-1.
* The default value is UTF-8. BigQuery decodes the data after the raw, binary data has been split
* using the values of the quote and fieldDelimiter properties.
* @return value or {@code null} for none
*/
public java.lang.String getEncoding() {
return encoding;
}
/**
* [Optional] The character encoding of the data. The supported values are UTF-8 or ISO-8859-1.
* The default value is UTF-8. BigQuery decodes the data after the raw, binary data has been split
* using the values of the quote and fieldDelimiter properties.
* @param encoding encoding or {@code null} for none
*/
public JobConfigurationLoad setEncoding(java.lang.String encoding) {
this.encoding = encoding;
return this;
}
/**
* [Optional] The separator for fields in a CSV file. BigQuery converts the string to ISO-8859-1
* encoding, and then uses the first byte of the encoded string to split the data in its raw,
* binary state. BigQuery also supports the escape sequence "\t" to specify a tab separator. The
* default value is a comma (',').
* @return value or {@code null} for none
*/
public java.lang.String getFieldDelimiter() {
return fieldDelimiter;
}
/**
* [Optional] The separator for fields in a CSV file. BigQuery converts the string to ISO-8859-1
* encoding, and then uses the first byte of the encoded string to split the data in its raw,
* binary state. BigQuery also supports the escape sequence "\t" to specify a tab separator. The
* default value is a comma (',').
* @param fieldDelimiter fieldDelimiter or {@code null} for none
*/
public JobConfigurationLoad setFieldDelimiter(java.lang.String fieldDelimiter) {
this.fieldDelimiter = fieldDelimiter;
return this;
}
/**
* [Optional] Accept rows that contain values that do not match the schema. The unknown values are
* ignored. Default is false which treats unknown values as errors. For CSV this ignores extra
* values at the end of a line. For JSON this ignores named values that do not match any column
* name.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIgnoreUnknownValues() {
return ignoreUnknownValues;
}
/**
* [Optional] Accept rows that contain values that do not match the schema. The unknown values are
* ignored. Default is false which treats unknown values as errors. For CSV this ignores extra
* values at the end of a line. For JSON this ignores named values that do not match any column
* name.
* @param ignoreUnknownValues ignoreUnknownValues or {@code null} for none
*/
public JobConfigurationLoad setIgnoreUnknownValues(java.lang.Boolean ignoreUnknownValues) {
this.ignoreUnknownValues = ignoreUnknownValues;
return this;
}
/**
* [Optional] The maximum number of bad records that BigQuery can ignore when running the job. If
* the number of bad records exceeds this value, an 'invalid' error is returned in the job result
* and the job fails. The default value is 0, which requires that all records are valid.
* @return value or {@code null} for none
*/
public java.lang.Integer getMaxBadRecords() {
return maxBadRecords;
}
/**
* [Optional] The maximum number of bad records that BigQuery can ignore when running the job. If
* the number of bad records exceeds this value, an 'invalid' error is returned in the job result
* and the job fails. The default value is 0, which requires that all records are valid.
* @param maxBadRecords maxBadRecords or {@code null} for none
*/
public JobConfigurationLoad setMaxBadRecords(java.lang.Integer maxBadRecords) {
this.maxBadRecords = maxBadRecords;
return this;
}
/**
* [Optional] The value that is used to quote data sections in a CSV file. BigQuery converts the
* string to ISO-8859-1 encoding, and then uses the first byte of the encoded string to split the
* data in its raw, binary state. The default value is a double-quote ('"'). If your data does not
* contain quoted sections, set the property value to an empty string. If your data contains
* quoted newline characters, you must also set the allowQuotedNewlines property to true.
* @return value or {@code null} for none
*/
public java.lang.String getQuote() {
return quote;
}
/**
* [Optional] The value that is used to quote data sections in a CSV file. BigQuery converts the
* string to ISO-8859-1 encoding, and then uses the first byte of the encoded string to split the
* data in its raw, binary state. The default value is a double-quote ('"'). If your data does not
* contain quoted sections, set the property value to an empty string. If your data contains
* quoted newline characters, you must also set the allowQuotedNewlines property to true.
* @param quote quote or {@code null} for none
*/
public JobConfigurationLoad setQuote(java.lang.String quote) {
this.quote = quote;
return this;
}
/**
* [Optional] The schema for the destination table. The schema can be omitted if the destination
* table already exists or if the schema can be inferred from the loaded data.
* @return value or {@code null} for none
*/
public TableSchema getSchema() {
return schema;
}
/**
* [Optional] The schema for the destination table. The schema can be omitted if the destination
* table already exists or if the schema can be inferred from the loaded data.
* @param schema schema or {@code null} for none
*/
public JobConfigurationLoad setSchema(TableSchema schema) {
this.schema = schema;
return this;
}
/**
* [Deprecated] The inline schema. For CSV schemas, specify as "Field1:Type1[,Field2:Type2]*". For
* example, "foo:STRING, bar:INTEGER, baz:FLOAT".
* @return value or {@code null} for none
*/
public java.lang.String getSchemaInline() {
return schemaInline;
}
/**
* [Deprecated] The inline schema. For CSV schemas, specify as "Field1:Type1[,Field2:Type2]*". For
* example, "foo:STRING, bar:INTEGER, baz:FLOAT".
* @param schemaInline schemaInline or {@code null} for none
*/
public JobConfigurationLoad setSchemaInline(java.lang.String schemaInline) {
this.schemaInline = schemaInline;
return this;
}
/**
* [Deprecated] The format of the schemaInline property.
* @return value or {@code null} for none
*/
public java.lang.String getSchemaInlineFormat() {
return schemaInlineFormat;
}
/**
* [Deprecated] The format of the schemaInline property.
* @param schemaInlineFormat schemaInlineFormat or {@code null} for none
*/
public JobConfigurationLoad setSchemaInlineFormat(java.lang.String schemaInlineFormat) {
this.schemaInlineFormat = schemaInlineFormat;
return this;
}
/**
* [Optional] The number of rows at the top of a CSV file that BigQuery will skip when loading the
* data. The default value is 0. This property is useful if you have header rows in the file that
* should be skipped.
* @return value or {@code null} for none
*/
public java.lang.Integer getSkipLeadingRows() {
return skipLeadingRows;
}
/**
* [Optional] The number of rows at the top of a CSV file that BigQuery will skip when loading the
* data. The default value is 0. This property is useful if you have header rows in the file that
* should be skipped.
* @param skipLeadingRows skipLeadingRows or {@code null} for none
*/
public JobConfigurationLoad setSkipLeadingRows(java.lang.Integer skipLeadingRows) {
this.skipLeadingRows = skipLeadingRows;
return this;
}
/**
* [Optional] The format of the data files. For CSV files, specify "CSV". For datastore backups,
* specify "DATASTORE_BACKUP". For newline-delimited JSON, specify "NEWLINE_DELIMITED_JSON". The
* default value is CSV.
* @return value or {@code null} for none
*/
public java.lang.String getSourceFormat() {
return sourceFormat;
}
/**
* [Optional] The format of the data files. For CSV files, specify "CSV". For datastore backups,
* specify "DATASTORE_BACKUP". For newline-delimited JSON, specify "NEWLINE_DELIMITED_JSON". The
* default value is CSV.
* @param sourceFormat sourceFormat or {@code null} for none
*/
public JobConfigurationLoad setSourceFormat(java.lang.String sourceFormat) {
this.sourceFormat = sourceFormat;
return this;
}
/**
* [Required] The fully-qualified URIs that point to your data in Google Cloud Storage. Wildcard
* names are only supported when they appear at the end of the URI.
* @return value or {@code null} for none
*/
public java.util.List getSourceUris() {
return sourceUris;
}
/**
* [Required] The fully-qualified URIs that point to your data in Google Cloud Storage. Wildcard
* names are only supported when they appear at the end of the URI.
* @param sourceUris sourceUris or {@code null} for none
*/
public JobConfigurationLoad setSourceUris(java.util.List sourceUris) {
this.sourceUris = sourceUris;
return this;
}
/**
* [Optional] Specifies the action that occurs if the destination table already exists. The
* following values are supported: WRITE_TRUNCATE: If the table already exists, BigQuery
* overwrites the table data. WRITE_APPEND: If the table already exists, BigQuery appends the data
* to the table. WRITE_EMPTY: If the table already exists and contains data, a 'duplicate' error
* is returned in the job result. The default value is WRITE_EMPTY. Each action is atomic and only
* occurs if BigQuery is able to complete the job successfully. Creation, truncation and append
* actions occur as one atomic update upon job completion.
* @return value or {@code null} for none
*/
public java.lang.String getWriteDisposition() {
return writeDisposition;
}
/**
* [Optional] Specifies the action that occurs if the destination table already exists. The
* following values are supported: WRITE_TRUNCATE: If the table already exists, BigQuery
* overwrites the table data. WRITE_APPEND: If the table already exists, BigQuery appends the data
* to the table. WRITE_EMPTY: If the table already exists and contains data, a 'duplicate' error
* is returned in the job result. The default value is WRITE_EMPTY. Each action is atomic and only
* occurs if BigQuery is able to complete the job successfully. Creation, truncation and append
* actions occur as one atomic update upon job completion.
* @param writeDisposition writeDisposition or {@code null} for none
*/
public JobConfigurationLoad setWriteDisposition(java.lang.String writeDisposition) {
this.writeDisposition = writeDisposition;
return this;
}
@Override
public JobConfigurationLoad set(String fieldName, Object value) {
return (JobConfigurationLoad) super.set(fieldName, value);
}
@Override
public JobConfigurationLoad clone() {
return (JobConfigurationLoad) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy