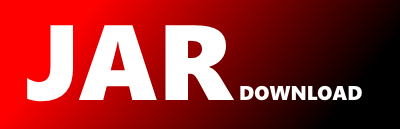
target.apidocs.com.google.api.services.bigquery.model.Dataset.html Maven / Gradle / Ivy
Dataset (BigQuery API v2-rev20190423-1.28.0)
com.google.api.services.bigquery.model
Class Dataset
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.bigquery.model.Dataset
-
public final class Dataset
extends com.google.api.client.json.GenericJson
Model definition for Dataset.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
Dataset.Access
Model definition for DatasetAccess.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Dataset()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Dataset
clone()
List<Dataset.Access>
getAccess()
[Optional] An array of objects that define dataset access for one or more entities.
Long
getCreationTime()
[Output-only] The time when this dataset was created, in milliseconds since the epoch.
DatasetReference
getDatasetReference()
[Required] A reference that identifies the dataset.
Long
getDefaultPartitionExpirationMs()
[Optional] The default partition expiration for all partitioned tables in the dataset, in
milliseconds.
Long
getDefaultTableExpirationMs()
[Optional] The default lifetime of all tables in the dataset, in milliseconds.
String
getDescription()
[Optional] A user-friendly description of the dataset.
String
getEtag()
[Output-only] A hash of the resource.
String
getFriendlyName()
[Optional] A descriptive name for the dataset.
String
getId()
[Output-only] The fully-qualified unique name of the dataset in the format projectId:datasetId.
String
getKind()
[Output-only] The resource type.
Map<String,String>
getLabels()
The labels associated with this dataset.
Long
getLastModifiedTime()
[Output-only] The date when this dataset or any of its tables was last modified, in
milliseconds since the epoch.
String
getLocation()
The geographic location where the dataset should reside.
String
getSelfLink()
[Output-only] A URL that can be used to access the resource again.
Dataset
set(String fieldName,
Object value)
Dataset
setAccess(List<Dataset.Access> access)
[Optional] An array of objects that define dataset access for one or more entities.
Dataset
setCreationTime(Long creationTime)
[Output-only] The time when this dataset was created, in milliseconds since the epoch.
Dataset
setDatasetReference(DatasetReference datasetReference)
[Required] A reference that identifies the dataset.
Dataset
setDefaultPartitionExpirationMs(Long defaultPartitionExpirationMs)
[Optional] The default partition expiration for all partitioned tables in the dataset, in
milliseconds.
Dataset
setDefaultTableExpirationMs(Long defaultTableExpirationMs)
[Optional] The default lifetime of all tables in the dataset, in milliseconds.
Dataset
setDescription(String description)
[Optional] A user-friendly description of the dataset.
Dataset
setEtag(String etag)
[Output-only] A hash of the resource.
Dataset
setFriendlyName(String friendlyName)
[Optional] A descriptive name for the dataset.
Dataset
setId(String id)
[Output-only] The fully-qualified unique name of the dataset in the format projectId:datasetId.
Dataset
setKind(String kind)
[Output-only] The resource type.
Dataset
setLabels(Map<String,String> labels)
The labels associated with this dataset.
Dataset
setLastModifiedTime(Long lastModifiedTime)
[Output-only] The date when this dataset or any of its tables was last modified, in
milliseconds since the epoch.
Dataset
setLocation(String location)
The geographic location where the dataset should reside.
Dataset
setSelfLink(String selfLink)
[Output-only] A URL that can be used to access the resource again.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, get, getClassInfo, getUnknownKeys, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, equals, hashCode, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAccess
public List<Dataset.Access> getAccess()
[Optional] An array of objects that define dataset access for one or more entities. You can set
this property when inserting or updating a dataset in order to control who is allowed to access
the data. If unspecified at dataset creation time, BigQuery adds default dataset access for the
following entities: access.specialGroup: projectReaders; access.role: READER;
access.specialGroup: projectWriters; access.role: WRITER; access.specialGroup: projectOwners;
access.role: OWNER; access.userByEmail: [dataset creator email]; access.role: OWNER;
- Returns:
- value or
null
for none
-
setAccess
public Dataset setAccess(List<Dataset.Access> access)
[Optional] An array of objects that define dataset access for one or more entities. You can set
this property when inserting or updating a dataset in order to control who is allowed to access
the data. If unspecified at dataset creation time, BigQuery adds default dataset access for the
following entities: access.specialGroup: projectReaders; access.role: READER;
access.specialGroup: projectWriters; access.role: WRITER; access.specialGroup: projectOwners;
access.role: OWNER; access.userByEmail: [dataset creator email]; access.role: OWNER;
- Parameters:
access
- access or null
for none
-
getCreationTime
public Long getCreationTime()
[Output-only] The time when this dataset was created, in milliseconds since the epoch.
- Returns:
- value or
null
for none
-
setCreationTime
public Dataset setCreationTime(Long creationTime)
[Output-only] The time when this dataset was created, in milliseconds since the epoch.
- Parameters:
creationTime
- creationTime or null
for none
-
getDatasetReference
public DatasetReference getDatasetReference()
[Required] A reference that identifies the dataset.
- Returns:
- value or
null
for none
-
setDatasetReference
public Dataset setDatasetReference(DatasetReference datasetReference)
[Required] A reference that identifies the dataset.
- Parameters:
datasetReference
- datasetReference or null
for none
-
getDefaultPartitionExpirationMs
public Long getDefaultPartitionExpirationMs()
[Optional] The default partition expiration for all partitioned tables in the dataset, in
milliseconds. Once this property is set, all newly-created partitioned tables in the dataset
will have an expirationMs property in the timePartitioning settings set to this value, and
changing the value will only affect new tables, not existing ones. The storage in a partition
will have an expiration time of its partition time plus this value. Setting this property
overrides the use of defaultTableExpirationMs for partitioned tables: only one of
defaultTableExpirationMs and defaultPartitionExpirationMs will be used for any new partitioned
table. If you provide an explicit timePartitioning.expirationMs when creating or updating a
partitioned table, that value takes precedence over the default partition expiration time
indicated by this property.
- Returns:
- value or
null
for none
-
setDefaultPartitionExpirationMs
public Dataset setDefaultPartitionExpirationMs(Long defaultPartitionExpirationMs)
[Optional] The default partition expiration for all partitioned tables in the dataset, in
milliseconds. Once this property is set, all newly-created partitioned tables in the dataset
will have an expirationMs property in the timePartitioning settings set to this value, and
changing the value will only affect new tables, not existing ones. The storage in a partition
will have an expiration time of its partition time plus this value. Setting this property
overrides the use of defaultTableExpirationMs for partitioned tables: only one of
defaultTableExpirationMs and defaultPartitionExpirationMs will be used for any new partitioned
table. If you provide an explicit timePartitioning.expirationMs when creating or updating a
partitioned table, that value takes precedence over the default partition expiration time
indicated by this property.
- Parameters:
defaultPartitionExpirationMs
- defaultPartitionExpirationMs or null
for none
-
getDefaultTableExpirationMs
public Long getDefaultTableExpirationMs()
[Optional] The default lifetime of all tables in the dataset, in milliseconds. The minimum
value is 3600000 milliseconds (one hour). Once this property is set, all newly-created tables
in the dataset will have an expirationTime property set to the creation time plus the value in
this property, and changing the value will only affect new tables, not existing ones. When the
expirationTime for a given table is reached, that table will be deleted automatically. If a
table's expirationTime is modified or removed before the table expires, or if you provide an
explicit expirationTime when creating a table, that value takes precedence over the default
expiration time indicated by this property.
- Returns:
- value or
null
for none
-
setDefaultTableExpirationMs
public Dataset setDefaultTableExpirationMs(Long defaultTableExpirationMs)
[Optional] The default lifetime of all tables in the dataset, in milliseconds. The minimum
value is 3600000 milliseconds (one hour). Once this property is set, all newly-created tables
in the dataset will have an expirationTime property set to the creation time plus the value in
this property, and changing the value will only affect new tables, not existing ones. When the
expirationTime for a given table is reached, that table will be deleted automatically. If a
table's expirationTime is modified or removed before the table expires, or if you provide an
explicit expirationTime when creating a table, that value takes precedence over the default
expiration time indicated by this property.
- Parameters:
defaultTableExpirationMs
- defaultTableExpirationMs or null
for none
-
getDescription
public String getDescription()
[Optional] A user-friendly description of the dataset.
- Returns:
- value or
null
for none
-
setDescription
public Dataset setDescription(String description)
[Optional] A user-friendly description of the dataset.
- Parameters:
description
- description or null
for none
-
getEtag
public String getEtag()
[Output-only] A hash of the resource.
- Returns:
- value or
null
for none
-
setEtag
public Dataset setEtag(String etag)
[Output-only] A hash of the resource.
- Parameters:
etag
- etag or null
for none
-
getFriendlyName
public String getFriendlyName()
[Optional] A descriptive name for the dataset.
- Returns:
- value or
null
for none
-
setFriendlyName
public Dataset setFriendlyName(String friendlyName)
[Optional] A descriptive name for the dataset.
- Parameters:
friendlyName
- friendlyName or null
for none
-
getId
public String getId()
[Output-only] The fully-qualified unique name of the dataset in the format projectId:datasetId.
The dataset name without the project name is given in the datasetId field. When creating a new
dataset, leave this field blank, and instead specify the datasetId field.
- Returns:
- value or
null
for none
-
setId
public Dataset setId(String id)
[Output-only] The fully-qualified unique name of the dataset in the format projectId:datasetId.
The dataset name without the project name is given in the datasetId field. When creating a new
dataset, leave this field blank, and instead specify the datasetId field.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output-only] The resource type.
- Returns:
- value or
null
for none
-
setKind
public Dataset setKind(String kind)
[Output-only] The resource type.
- Parameters:
kind
- kind or null
for none
-
getLabels
public Map<String,String> getLabels()
The labels associated with this dataset. You can use these to organize and group your datasets.
You can set this property when inserting or updating a dataset. See Creating and Updating
Dataset Labels for more information.
- Returns:
- value or
null
for none
-
setLabels
public Dataset setLabels(Map<String,String> labels)
The labels associated with this dataset. You can use these to organize and group your datasets.
You can set this property when inserting or updating a dataset. See Creating and Updating
Dataset Labels for more information.
- Parameters:
labels
- labels or null
for none
-
getLastModifiedTime
public Long getLastModifiedTime()
[Output-only] The date when this dataset or any of its tables was last modified, in
milliseconds since the epoch.
- Returns:
- value or
null
for none
-
setLastModifiedTime
public Dataset setLastModifiedTime(Long lastModifiedTime)
[Output-only] The date when this dataset or any of its tables was last modified, in
milliseconds since the epoch.
- Parameters:
lastModifiedTime
- lastModifiedTime or null
for none
-
getLocation
public String getLocation()
The geographic location where the dataset should reside. The default value is US. See details
at https://cloud.google.com/bigquery/docs/locations.
- Returns:
- value or
null
for none
-
setLocation
public Dataset setLocation(String location)
The geographic location where the dataset should reside. The default value is US. See details
at https://cloud.google.com/bigquery/docs/locations.
- Parameters:
location
- location or null
for none
-
getSelfLink
public String getSelfLink()
[Output-only] A URL that can be used to access the resource again. You can use this URL in Get
or Update requests to the resource.
- Returns:
- value or
null
for none
-
setSelfLink
public Dataset setSelfLink(String selfLink)
[Output-only] A URL that can be used to access the resource again. You can use this URL in Get
or Update requests to the resource.
- Parameters:
selfLink
- selfLink or null
for none
-
set
public Dataset set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Dataset clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2019 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy