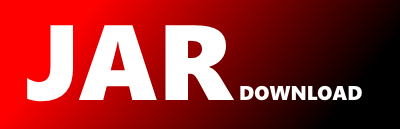
target.apidocs.com.google.api.services.bigquery.model.Table.html Maven / Gradle / Ivy
Table (BigQuery API v2-rev20190423-1.28.0)
com.google.api.services.bigquery.model
Class Table
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.bigquery.model.Table
-
public final class Table
extends com.google.api.client.json.GenericJson
Model definition for Table.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Table()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Table
clone()
Clustering
getClustering()
[Beta] Clustering specification for the table.
Long
getCreationTime()
[Output-only] The time when this table was created, in milliseconds since the epoch.
String
getDescription()
[Optional] A user-friendly description of this table.
EncryptionConfiguration
getEncryptionConfiguration()
Custom encryption configuration (e.g., Cloud KMS keys).
String
getEtag()
[Output-only] A hash of the table metadata.
Long
getExpirationTime()
[Optional] The time when this table expires, in milliseconds since the epoch.
ExternalDataConfiguration
getExternalDataConfiguration()
[Optional] Describes the data format, location, and other properties of a table stored outside
of BigQuery.
String
getFriendlyName()
[Optional] A descriptive name for this table.
String
getId()
[Output-only] An opaque ID uniquely identifying the table.
String
getKind()
[Output-only] The type of the resource.
Map<String,String>
getLabels()
The labels associated with this table.
BigInteger
getLastModifiedTime()
[Output-only] The time when this table was last modified, in milliseconds since the epoch.
String
getLocation()
[Output-only] The geographic location where the table resides.
MaterializedViewDefinition
getMaterializedView()
[Optional] Materialized view definition.
ModelDefinition
getModel()
[Output-only, Beta] Present iff this table represents a ML model.
Long
getNumBytes()
[Output-only] The size of this table in bytes, excluding any data in the streaming buffer.
Long
getNumLongTermBytes()
[Output-only] The number of bytes in the table that are considered "long-term storage".
Long
getNumPhysicalBytes()
[Output-only] [TrustedTester] The physical size of this table in bytes, excluding any data in
the streaming buffer.
BigInteger
getNumRows()
[Output-only] The number of rows of data in this table, excluding any data in the streaming
buffer.
RangePartitioning
getRangePartitioning()
[TrustedTester] Range partitioning specification for this table.
Boolean
getRequirePartitionFilter()
[Beta] [Optional] If set to true, queries over this table require a partition filter that can
be used for partition elimination to be specified.
TableSchema
getSchema()
[Optional] Describes the schema of this table.
String
getSelfLink()
[Output-only] A URL that can be used to access this resource again.
Streamingbuffer
getStreamingBuffer()
[Output-only] Contains information regarding this table's streaming buffer, if one is present.
TableReference
getTableReference()
[Required] Reference describing the ID of this table.
TimePartitioning
getTimePartitioning()
Time-based partitioning specification for this table.
String
getType()
[Output-only] Describes the table type.
ViewDefinition
getView()
[Optional] The view definition.
boolean
isRequirePartitionFilter()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Table
set(String fieldName,
Object value)
Table
setClustering(Clustering clustering)
[Beta] Clustering specification for the table.
Table
setCreationTime(Long creationTime)
[Output-only] The time when this table was created, in milliseconds since the epoch.
Table
setDescription(String description)
[Optional] A user-friendly description of this table.
Table
setEncryptionConfiguration(EncryptionConfiguration encryptionConfiguration)
Custom encryption configuration (e.g., Cloud KMS keys).
Table
setEtag(String etag)
[Output-only] A hash of the table metadata.
Table
setExpirationTime(Long expirationTime)
[Optional] The time when this table expires, in milliseconds since the epoch.
Table
setExternalDataConfiguration(ExternalDataConfiguration externalDataConfiguration)
[Optional] Describes the data format, location, and other properties of a table stored outside
of BigQuery.
Table
setFriendlyName(String friendlyName)
[Optional] A descriptive name for this table.
Table
setId(String id)
[Output-only] An opaque ID uniquely identifying the table.
Table
setKind(String kind)
[Output-only] The type of the resource.
Table
setLabels(Map<String,String> labels)
The labels associated with this table.
Table
setLastModifiedTime(BigInteger lastModifiedTime)
[Output-only] The time when this table was last modified, in milliseconds since the epoch.
Table
setLocation(String location)
[Output-only] The geographic location where the table resides.
Table
setMaterializedView(MaterializedViewDefinition materializedView)
[Optional] Materialized view definition.
Table
setModel(ModelDefinition model)
[Output-only, Beta] Present iff this table represents a ML model.
Table
setNumBytes(Long numBytes)
[Output-only] The size of this table in bytes, excluding any data in the streaming buffer.
Table
setNumLongTermBytes(Long numLongTermBytes)
[Output-only] The number of bytes in the table that are considered "long-term storage".
Table
setNumPhysicalBytes(Long numPhysicalBytes)
[Output-only] [TrustedTester] The physical size of this table in bytes, excluding any data in
the streaming buffer.
Table
setNumRows(BigInteger numRows)
[Output-only] The number of rows of data in this table, excluding any data in the streaming
buffer.
Table
setRangePartitioning(RangePartitioning rangePartitioning)
[TrustedTester] Range partitioning specification for this table.
Table
setRequirePartitionFilter(Boolean requirePartitionFilter)
[Beta] [Optional] If set to true, queries over this table require a partition filter that can
be used for partition elimination to be specified.
Table
setSchema(TableSchema schema)
[Optional] Describes the schema of this table.
Table
setSelfLink(String selfLink)
[Output-only] A URL that can be used to access this resource again.
Table
setStreamingBuffer(Streamingbuffer streamingBuffer)
[Output-only] Contains information regarding this table's streaming buffer, if one is present.
Table
setTableReference(TableReference tableReference)
[Required] Reference describing the ID of this table.
Table
setTimePartitioning(TimePartitioning timePartitioning)
Time-based partitioning specification for this table.
Table
setType(String type)
[Output-only] Describes the table type.
Table
setView(ViewDefinition view)
[Optional] The view definition.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, get, getClassInfo, getUnknownKeys, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, equals, hashCode, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getClustering
public Clustering getClustering()
[Beta] Clustering specification for the table. Must be specified with partitioning, data in the
table will be first partitioned and subsequently clustered.
- Returns:
- value or
null
for none
-
setClustering
public Table setClustering(Clustering clustering)
[Beta] Clustering specification for the table. Must be specified with partitioning, data in the
table will be first partitioned and subsequently clustered.
- Parameters:
clustering
- clustering or null
for none
-
getCreationTime
public Long getCreationTime()
[Output-only] The time when this table was created, in milliseconds since the epoch.
- Returns:
- value or
null
for none
-
setCreationTime
public Table setCreationTime(Long creationTime)
[Output-only] The time when this table was created, in milliseconds since the epoch.
- Parameters:
creationTime
- creationTime or null
for none
-
getDescription
public String getDescription()
[Optional] A user-friendly description of this table.
- Returns:
- value or
null
for none
-
setDescription
public Table setDescription(String description)
[Optional] A user-friendly description of this table.
- Parameters:
description
- description or null
for none
-
getEncryptionConfiguration
public EncryptionConfiguration getEncryptionConfiguration()
Custom encryption configuration (e.g., Cloud KMS keys).
- Returns:
- value or
null
for none
-
setEncryptionConfiguration
public Table setEncryptionConfiguration(EncryptionConfiguration encryptionConfiguration)
Custom encryption configuration (e.g., Cloud KMS keys).
- Parameters:
encryptionConfiguration
- encryptionConfiguration or null
for none
-
getEtag
public String getEtag()
[Output-only] A hash of the table metadata. Used to ensure there were no concurrent
modifications to the resource when attempting an update. Not guaranteed to change when the
table contents or the fields numRows, numBytes, numLongTermBytes or lastModifiedTime change.
- Returns:
- value or
null
for none
-
setEtag
public Table setEtag(String etag)
[Output-only] A hash of the table metadata. Used to ensure there were no concurrent
modifications to the resource when attempting an update. Not guaranteed to change when the
table contents or the fields numRows, numBytes, numLongTermBytes or lastModifiedTime change.
- Parameters:
etag
- etag or null
for none
-
getExpirationTime
public Long getExpirationTime()
[Optional] The time when this table expires, in milliseconds since the epoch. If not present,
the table will persist indefinitely. Expired tables will be deleted and their storage
reclaimed. The defaultTableExpirationMs property of the encapsulating dataset can be used to
set a default expirationTime on newly created tables.
- Returns:
- value or
null
for none
-
setExpirationTime
public Table setExpirationTime(Long expirationTime)
[Optional] The time when this table expires, in milliseconds since the epoch. If not present,
the table will persist indefinitely. Expired tables will be deleted and their storage
reclaimed. The defaultTableExpirationMs property of the encapsulating dataset can be used to
set a default expirationTime on newly created tables.
- Parameters:
expirationTime
- expirationTime or null
for none
-
getExternalDataConfiguration
public ExternalDataConfiguration getExternalDataConfiguration()
[Optional] Describes the data format, location, and other properties of a table stored outside
of BigQuery. By defining these properties, the data source can then be queried as if it were a
standard BigQuery table.
- Returns:
- value or
null
for none
-
setExternalDataConfiguration
public Table setExternalDataConfiguration(ExternalDataConfiguration externalDataConfiguration)
[Optional] Describes the data format, location, and other properties of a table stored outside
of BigQuery. By defining these properties, the data source can then be queried as if it were a
standard BigQuery table.
- Parameters:
externalDataConfiguration
- externalDataConfiguration or null
for none
-
getFriendlyName
public String getFriendlyName()
[Optional] A descriptive name for this table.
- Returns:
- value or
null
for none
-
setFriendlyName
public Table setFriendlyName(String friendlyName)
[Optional] A descriptive name for this table.
- Parameters:
friendlyName
- friendlyName or null
for none
-
getId
public String getId()
[Output-only] An opaque ID uniquely identifying the table.
- Returns:
- value or
null
for none
-
setId
public Table setId(String id)
[Output-only] An opaque ID uniquely identifying the table.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
[Output-only] The type of the resource.
- Returns:
- value or
null
for none
-
setKind
public Table setKind(String kind)
[Output-only] The type of the resource.
- Parameters:
kind
- kind or null
for none
-
getLabels
public Map<String,String> getLabels()
The labels associated with this table. You can use these to organize and group your tables.
Label keys and values can be no longer than 63 characters, can only contain lowercase letters,
numeric characters, underscores and dashes. International characters are allowed. Label values
are optional. Label keys must start with a letter and each label in the list must have a
different key.
- Returns:
- value or
null
for none
-
setLabels
public Table setLabels(Map<String,String> labels)
The labels associated with this table. You can use these to organize and group your tables.
Label keys and values can be no longer than 63 characters, can only contain lowercase letters,
numeric characters, underscores and dashes. International characters are allowed. Label values
are optional. Label keys must start with a letter and each label in the list must have a
different key.
- Parameters:
labels
- labels or null
for none
-
getLastModifiedTime
public BigInteger getLastModifiedTime()
[Output-only] The time when this table was last modified, in milliseconds since the epoch.
- Returns:
- value or
null
for none
-
setLastModifiedTime
public Table setLastModifiedTime(BigInteger lastModifiedTime)
[Output-only] The time when this table was last modified, in milliseconds since the epoch.
- Parameters:
lastModifiedTime
- lastModifiedTime or null
for none
-
getLocation
public String getLocation()
[Output-only] The geographic location where the table resides. This value is inherited from the
dataset.
- Returns:
- value or
null
for none
-
setLocation
public Table setLocation(String location)
[Output-only] The geographic location where the table resides. This value is inherited from the
dataset.
- Parameters:
location
- location or null
for none
-
getMaterializedView
public MaterializedViewDefinition getMaterializedView()
[Optional] Materialized view definition.
- Returns:
- value or
null
for none
-
setMaterializedView
public Table setMaterializedView(MaterializedViewDefinition materializedView)
[Optional] Materialized view definition.
- Parameters:
materializedView
- materializedView or null
for none
-
getModel
public ModelDefinition getModel()
[Output-only, Beta] Present iff this table represents a ML model. Describes the training
information for the model, and it is required to run 'PREDICT' queries.
- Returns:
- value or
null
for none
-
setModel
public Table setModel(ModelDefinition model)
[Output-only, Beta] Present iff this table represents a ML model. Describes the training
information for the model, and it is required to run 'PREDICT' queries.
- Parameters:
model
- model or null
for none
-
getNumBytes
public Long getNumBytes()
[Output-only] The size of this table in bytes, excluding any data in the streaming buffer.
- Returns:
- value or
null
for none
-
setNumBytes
public Table setNumBytes(Long numBytes)
[Output-only] The size of this table in bytes, excluding any data in the streaming buffer.
- Parameters:
numBytes
- numBytes or null
for none
-
getNumLongTermBytes
public Long getNumLongTermBytes()
[Output-only] The number of bytes in the table that are considered "long-term storage".
- Returns:
- value or
null
for none
-
setNumLongTermBytes
public Table setNumLongTermBytes(Long numLongTermBytes)
[Output-only] The number of bytes in the table that are considered "long-term storage".
- Parameters:
numLongTermBytes
- numLongTermBytes or null
for none
-
getNumPhysicalBytes
public Long getNumPhysicalBytes()
[Output-only] [TrustedTester] The physical size of this table in bytes, excluding any data in
the streaming buffer. This includes compression and storage used for time travel.
- Returns:
- value or
null
for none
-
setNumPhysicalBytes
public Table setNumPhysicalBytes(Long numPhysicalBytes)
[Output-only] [TrustedTester] The physical size of this table in bytes, excluding any data in
the streaming buffer. This includes compression and storage used for time travel.
- Parameters:
numPhysicalBytes
- numPhysicalBytes or null
for none
-
getNumRows
public BigInteger getNumRows()
[Output-only] The number of rows of data in this table, excluding any data in the streaming
buffer.
- Returns:
- value or
null
for none
-
setNumRows
public Table setNumRows(BigInteger numRows)
[Output-only] The number of rows of data in this table, excluding any data in the streaming
buffer.
- Parameters:
numRows
- numRows or null
for none
-
getRangePartitioning
public RangePartitioning getRangePartitioning()
[TrustedTester] Range partitioning specification for this table. Only one of timePartitioning
and rangePartitioning should be specified.
- Returns:
- value or
null
for none
-
setRangePartitioning
public Table setRangePartitioning(RangePartitioning rangePartitioning)
[TrustedTester] Range partitioning specification for this table. Only one of timePartitioning
and rangePartitioning should be specified.
- Parameters:
rangePartitioning
- rangePartitioning or null
for none
-
getRequirePartitionFilter
public Boolean getRequirePartitionFilter()
[Beta] [Optional] If set to true, queries over this table require a partition filter that can
be used for partition elimination to be specified.
- Returns:
- value or
null
for none
-
setRequirePartitionFilter
public Table setRequirePartitionFilter(Boolean requirePartitionFilter)
[Beta] [Optional] If set to true, queries over this table require a partition filter that can
be used for partition elimination to be specified.
- Parameters:
requirePartitionFilter
- requirePartitionFilter or null
for none
-
isRequirePartitionFilter
public boolean isRequirePartitionFilter()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
[ Beta] [Optional] If set to true, queries over this table require a partition filter that can be
[ used for partition elimination to be specified.
-
getSchema
public TableSchema getSchema()
[Optional] Describes the schema of this table.
- Returns:
- value or
null
for none
-
setSchema
public Table setSchema(TableSchema schema)
[Optional] Describes the schema of this table.
- Parameters:
schema
- schema or null
for none
-
getSelfLink
public String getSelfLink()
[Output-only] A URL that can be used to access this resource again.
- Returns:
- value or
null
for none
-
setSelfLink
public Table setSelfLink(String selfLink)
[Output-only] A URL that can be used to access this resource again.
- Parameters:
selfLink
- selfLink or null
for none
-
getStreamingBuffer
public Streamingbuffer getStreamingBuffer()
[Output-only] Contains information regarding this table's streaming buffer, if one is present.
This field will be absent if the table is not being streamed to or if there is no data in the
streaming buffer.
- Returns:
- value or
null
for none
-
setStreamingBuffer
public Table setStreamingBuffer(Streamingbuffer streamingBuffer)
[Output-only] Contains information regarding this table's streaming buffer, if one is present.
This field will be absent if the table is not being streamed to or if there is no data in the
streaming buffer.
- Parameters:
streamingBuffer
- streamingBuffer or null
for none
-
getTableReference
public TableReference getTableReference()
[Required] Reference describing the ID of this table.
- Returns:
- value or
null
for none
-
setTableReference
public Table setTableReference(TableReference tableReference)
[Required] Reference describing the ID of this table.
- Parameters:
tableReference
- tableReference or null
for none
-
getTimePartitioning
public TimePartitioning getTimePartitioning()
Time-based partitioning specification for this table. Only one of timePartitioning and
rangePartitioning should be specified.
- Returns:
- value or
null
for none
-
setTimePartitioning
public Table setTimePartitioning(TimePartitioning timePartitioning)
Time-based partitioning specification for this table. Only one of timePartitioning and
rangePartitioning should be specified.
- Parameters:
timePartitioning
- timePartitioning or null
for none
-
getType
public String getType()
[Output-only] Describes the table type. The following values are supported: TABLE: A normal
BigQuery table. VIEW: A virtual table defined by a SQL query. [TrustedTester]
MATERIALIZED_VIEW: SQL query whose result is persisted. EXTERNAL: A table that references data
stored in an external storage system, such as Google Cloud Storage. The default value is TABLE.
- Returns:
- value or
null
for none
-
setType
public Table setType(String type)
[Output-only] Describes the table type. The following values are supported: TABLE: A normal
BigQuery table. VIEW: A virtual table defined by a SQL query. [TrustedTester]
MATERIALIZED_VIEW: SQL query whose result is persisted. EXTERNAL: A table that references data
stored in an external storage system, such as Google Cloud Storage. The default value is TABLE.
- Parameters:
type
- type or null
for none
-
getView
public ViewDefinition getView()
[Optional] The view definition.
- Returns:
- value or
null
for none
-
setView
public Table setView(ViewDefinition view)
[Optional] The view definition.
- Parameters:
view
- view or null
for none
-
set
public Table set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Table clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2019 Google. All rights reserved.