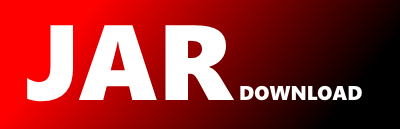
target.apidocs.com.google.api.services.bigquery.model.QueryRequest.html Maven / Gradle / Ivy
QueryRequest (BigQuery API v2-rev20240727-2.0.0)
com.google.api.services.bigquery.model
Class QueryRequest
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.bigquery.model.QueryRequest
-
public final class QueryRequest
extends com.google.api.client.json.GenericJson
Describes the format of the jobs.query request.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
QueryRequest()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
QueryRequest
clone()
List<ConnectionProperty>
getConnectionProperties()
Optional.
Boolean
getContinuous()
[Optional] Specifies whether the query should be executed as a continuous query.
Boolean
getCreateSession()
Optional.
DatasetReference
getDefaultDataset()
Optional.
Boolean
getDryRun()
Optional.
DataFormatOptions
getFormatOptions()
Optional.
String
getJobCreationMode()
Optional.
String
getKind()
The resource type of the request.
Map<String,String>
getLabels()
Optional.
String
getLocation()
The geographic location where the job should run.
Long
getMaximumBytesBilled()
Optional.
Long
getMaxResults()
Optional.
String
getParameterMode()
GoogleSQL only.
Boolean
getPreserveNulls()
This property is deprecated.
String
getQuery()
Required.
List<QueryParameter>
getQueryParameters()
Query parameters for GoogleSQL queries.
String
getRequestId()
Optional.
Long
getTimeoutMs()
Optional.
Boolean
getUseLegacySql()
Specifies whether to use BigQuery's legacy SQL dialect for this query.
Boolean
getUseQueryCache()
Optional.
boolean
isUseLegacySql()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
boolean
isUseQueryCache()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
QueryRequest
set(String fieldName,
Object value)
QueryRequest
setConnectionProperties(List<ConnectionProperty> connectionProperties)
Optional.
QueryRequest
setContinuous(Boolean continuous)
[Optional] Specifies whether the query should be executed as a continuous query.
QueryRequest
setCreateSession(Boolean createSession)
Optional.
QueryRequest
setDefaultDataset(DatasetReference defaultDataset)
Optional.
QueryRequest
setDryRun(Boolean dryRun)
Optional.
QueryRequest
setFormatOptions(DataFormatOptions formatOptions)
Optional.
QueryRequest
setJobCreationMode(String jobCreationMode)
Optional.
QueryRequest
setKind(String kind)
The resource type of the request.
QueryRequest
setLabels(Map<String,String> labels)
Optional.
QueryRequest
setLocation(String location)
The geographic location where the job should run.
QueryRequest
setMaximumBytesBilled(Long maximumBytesBilled)
Optional.
QueryRequest
setMaxResults(Long maxResults)
Optional.
QueryRequest
setParameterMode(String parameterMode)
GoogleSQL only.
QueryRequest
setPreserveNulls(Boolean preserveNulls)
This property is deprecated.
QueryRequest
setQuery(String query)
Required.
QueryRequest
setQueryParameters(List<QueryParameter> queryParameters)
Query parameters for GoogleSQL queries.
QueryRequest
setRequestId(String requestId)
Optional.
QueryRequest
setTimeoutMs(Long timeoutMs)
Optional.
QueryRequest
setUseLegacySql(Boolean useLegacySql)
Specifies whether to use BigQuery's legacy SQL dialect for this query.
QueryRequest
setUseQueryCache(Boolean useQueryCache)
Optional.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getConnectionProperties
public List<ConnectionProperty> getConnectionProperties()
Optional. Connection properties which can modify the query behavior.
- Returns:
- value or
null
for none
-
setConnectionProperties
public QueryRequest setConnectionProperties(List<ConnectionProperty> connectionProperties)
Optional. Connection properties which can modify the query behavior.
- Parameters:
connectionProperties
- connectionProperties or null
for none
-
getContinuous
public Boolean getContinuous()
[Optional] Specifies whether the query should be executed as a continuous query. The default
value is false.
- Returns:
- value or
null
for none
-
setContinuous
public QueryRequest setContinuous(Boolean continuous)
[Optional] Specifies whether the query should be executed as a continuous query. The default
value is false.
- Parameters:
continuous
- continuous or null
for none
-
getCreateSession
public Boolean getCreateSession()
Optional. If true, creates a new session using a randomly generated session_id. If false, runs
query with an existing session_id passed in ConnectionProperty, otherwise runs query in non-
session mode. The session location will be set to QueryRequest.location if it is present,
otherwise it's set to the default location based on existing routing logic.
- Returns:
- value or
null
for none
-
setCreateSession
public QueryRequest setCreateSession(Boolean createSession)
Optional. If true, creates a new session using a randomly generated session_id. If false, runs
query with an existing session_id passed in ConnectionProperty, otherwise runs query in non-
session mode. The session location will be set to QueryRequest.location if it is present,
otherwise it's set to the default location based on existing routing logic.
- Parameters:
createSession
- createSession or null
for none
-
getDefaultDataset
public DatasetReference getDefaultDataset()
Optional. Specifies the default datasetId and projectId to assume for any unqualified table
names in the query. If not set, all table names in the query string must be qualified in the
format 'datasetId.tableId'.
- Returns:
- value or
null
for none
-
setDefaultDataset
public QueryRequest setDefaultDataset(DatasetReference defaultDataset)
Optional. Specifies the default datasetId and projectId to assume for any unqualified table
names in the query. If not set, all table names in the query string must be qualified in the
format 'datasetId.tableId'.
- Parameters:
defaultDataset
- defaultDataset or null
for none
-
getDryRun
public Boolean getDryRun()
Optional. If set to true, BigQuery doesn't run the job. Instead, if the query is valid,
BigQuery returns statistics about the job such as how many bytes would be processed. If the
query is invalid, an error returns. The default value is false.
- Returns:
- value or
null
for none
-
setDryRun
public QueryRequest setDryRun(Boolean dryRun)
Optional. If set to true, BigQuery doesn't run the job. Instead, if the query is valid,
BigQuery returns statistics about the job such as how many bytes would be processed. If the
query is invalid, an error returns. The default value is false.
- Parameters:
dryRun
- dryRun or null
for none
-
getFormatOptions
public DataFormatOptions getFormatOptions()
Optional. Output format adjustments.
- Returns:
- value or
null
for none
-
setFormatOptions
public QueryRequest setFormatOptions(DataFormatOptions formatOptions)
Optional. Output format adjustments.
- Parameters:
formatOptions
- formatOptions or null
for none
-
getJobCreationMode
public String getJobCreationMode()
Optional. If not set, jobs are always required. If set, the query request will follow the
behavior described JobCreationMode. This feature is not yet available. Jobs will always be
created.
- Returns:
- value or
null
for none
-
setJobCreationMode
public QueryRequest setJobCreationMode(String jobCreationMode)
Optional. If not set, jobs are always required. If set, the query request will follow the
behavior described JobCreationMode. This feature is not yet available. Jobs will always be
created.
- Parameters:
jobCreationMode
- jobCreationMode or null
for none
-
getKind
public String getKind()
The resource type of the request.
- Returns:
- value or
null
for none
-
setKind
public QueryRequest setKind(String kind)
The resource type of the request.
- Parameters:
kind
- kind or null
for none
-
getLabels
public Map<String,String> getLabels()
Optional. The labels associated with this query. Labels can be used to organize and group query
jobs. Label keys and values can be no longer than 63 characters, can only contain lowercase
letters, numeric characters, underscores and dashes. International characters are allowed.
Label keys must start with a letter and each label in the list must have a different key.
- Returns:
- value or
null
for none
-
setLabels
public QueryRequest setLabels(Map<String,String> labels)
Optional. The labels associated with this query. Labels can be used to organize and group query
jobs. Label keys and values can be no longer than 63 characters, can only contain lowercase
letters, numeric characters, underscores and dashes. International characters are allowed.
Label keys must start with a letter and each label in the list must have a different key.
- Parameters:
labels
- labels or null
for none
-
getLocation
public String getLocation()
The geographic location where the job should run. See details at
https://cloud.google.com/bigquery/docs/locations#specifying_your_location.
- Returns:
- value or
null
for none
-
setLocation
public QueryRequest setLocation(String location)
The geographic location where the job should run. See details at
https://cloud.google.com/bigquery/docs/locations#specifying_your_location.
- Parameters:
location
- location or null
for none
-
getMaxResults
public Long getMaxResults()
Optional. The maximum number of rows of data to return per page of results. Setting this flag
to a small value such as 1000 and then paging through results might improve reliability when
the query result set is large. In addition to this limit, responses are also limited to 10 MB.
By default, there is no maximum row count, and only the byte limit applies.
- Returns:
- value or
null
for none
-
setMaxResults
public QueryRequest setMaxResults(Long maxResults)
Optional. The maximum number of rows of data to return per page of results. Setting this flag
to a small value such as 1000 and then paging through results might improve reliability when
the query result set is large. In addition to this limit, responses are also limited to 10 MB.
By default, there is no maximum row count, and only the byte limit applies.
- Parameters:
maxResults
- maxResults or null
for none
-
getMaximumBytesBilled
public Long getMaximumBytesBilled()
Optional. Limits the bytes billed for this query. Queries with bytes billed above this limit
will fail (without incurring a charge). If unspecified, the project default is used.
- Returns:
- value or
null
for none
-
setMaximumBytesBilled
public QueryRequest setMaximumBytesBilled(Long maximumBytesBilled)
Optional. Limits the bytes billed for this query. Queries with bytes billed above this limit
will fail (without incurring a charge). If unspecified, the project default is used.
- Parameters:
maximumBytesBilled
- maximumBytesBilled or null
for none
-
getParameterMode
public String getParameterMode()
GoogleSQL only. Set to POSITIONAL to use positional (?) query parameters or to NAMED to use
named (@myparam) query parameters in this query.
- Returns:
- value or
null
for none
-
setParameterMode
public QueryRequest setParameterMode(String parameterMode)
GoogleSQL only. Set to POSITIONAL to use positional (?) query parameters or to NAMED to use
named (@myparam) query parameters in this query.
- Parameters:
parameterMode
- parameterMode or null
for none
-
getPreserveNulls
public Boolean getPreserveNulls()
This property is deprecated.
- Returns:
- value or
null
for none
-
setPreserveNulls
public QueryRequest setPreserveNulls(Boolean preserveNulls)
This property is deprecated.
- Parameters:
preserveNulls
- preserveNulls or null
for none
-
getQuery
public String getQuery()
Required. A query string to execute, using Google Standard SQL or legacy SQL syntax. Example:
"SELECT COUNT(f1) FROM myProjectId.myDatasetId.myTableId".
- Returns:
- value or
null
for none
-
setQuery
public QueryRequest setQuery(String query)
Required. A query string to execute, using Google Standard SQL or legacy SQL syntax. Example:
"SELECT COUNT(f1) FROM myProjectId.myDatasetId.myTableId".
- Parameters:
query
- query or null
for none
-
getQueryParameters
public List<QueryParameter> getQueryParameters()
Query parameters for GoogleSQL queries.
- Returns:
- value or
null
for none
-
setQueryParameters
public QueryRequest setQueryParameters(List<QueryParameter> queryParameters)
Query parameters for GoogleSQL queries.
- Parameters:
queryParameters
- queryParameters or null
for none
-
getRequestId
public String getRequestId()
Optional. A unique user provided identifier to ensure idempotent behavior for queries. Note
that this is different from the job_id. It has the following properties: 1. It is case-
sensitive, limited to up to 36 ASCII characters. A UUID is recommended. 2. Read only queries
can ignore this token since they are nullipotent by definition. 3. For the purposes of
idempotency ensured by the request_id, a request is considered duplicate of another only if
they have the same request_id and are actually duplicates. When determining whether a request
is a duplicate of another request, all parameters in the request that may affect the result are
considered. For example, query, connection_properties, query_parameters, use_legacy_sql are
parameters that affect the result and are considered when determining whether a request is a
duplicate, but properties like timeout_ms don't affect the result and are thus not considered.
Dry run query requests are never considered duplicate of another request. 4. When a duplicate
mutating query request is detected, it returns: a. the results of the mutation if it completes
successfully within the timeout. b. the running operation if it is still in progress at the end
of the timeout. 5. Its lifetime is limited to 15 minutes. In other words, if two requests are
sent with the same request_id, but more than 15 minutes apart, idempotency is not guaranteed.
- Returns:
- value or
null
for none
-
setRequestId
public QueryRequest setRequestId(String requestId)
Optional. A unique user provided identifier to ensure idempotent behavior for queries. Note
that this is different from the job_id. It has the following properties: 1. It is case-
sensitive, limited to up to 36 ASCII characters. A UUID is recommended. 2. Read only queries
can ignore this token since they are nullipotent by definition. 3. For the purposes of
idempotency ensured by the request_id, a request is considered duplicate of another only if
they have the same request_id and are actually duplicates. When determining whether a request
is a duplicate of another request, all parameters in the request that may affect the result are
considered. For example, query, connection_properties, query_parameters, use_legacy_sql are
parameters that affect the result and are considered when determining whether a request is a
duplicate, but properties like timeout_ms don't affect the result and are thus not considered.
Dry run query requests are never considered duplicate of another request. 4. When a duplicate
mutating query request is detected, it returns: a. the results of the mutation if it completes
successfully within the timeout. b. the running operation if it is still in progress at the end
of the timeout. 5. Its lifetime is limited to 15 minutes. In other words, if two requests are
sent with the same request_id, but more than 15 minutes apart, idempotency is not guaranteed.
- Parameters:
requestId
- requestId or null
for none
-
getTimeoutMs
public Long getTimeoutMs()
Optional. Optional: Specifies the maximum amount of time, in milliseconds, that the client is
willing to wait for the query to complete. By default, this limit is 10 seconds (10,000
milliseconds). If the query is complete, the jobComplete field in the response is true. If the
query has not yet completed, jobComplete is false. You can request a longer timeout period in
the timeoutMs field. However, the call is not guaranteed to wait for the specified timeout; it
typically returns after around 200 seconds (200,000 milliseconds), even if the query is not
complete. If jobComplete is false, you can continue to wait for the query to complete by
calling the getQueryResults method until the jobComplete field in the getQueryResults response
is true.
- Returns:
- value or
null
for none
-
setTimeoutMs
public QueryRequest setTimeoutMs(Long timeoutMs)
Optional. Optional: Specifies the maximum amount of time, in milliseconds, that the client is
willing to wait for the query to complete. By default, this limit is 10 seconds (10,000
milliseconds). If the query is complete, the jobComplete field in the response is true. If the
query has not yet completed, jobComplete is false. You can request a longer timeout period in
the timeoutMs field. However, the call is not guaranteed to wait for the specified timeout; it
typically returns after around 200 seconds (200,000 milliseconds), even if the query is not
complete. If jobComplete is false, you can continue to wait for the query to complete by
calling the getQueryResults method until the jobComplete field in the getQueryResults response
is true.
- Parameters:
timeoutMs
- timeoutMs or null
for none
-
getUseLegacySql
public Boolean getUseLegacySql()
Specifies whether to use BigQuery's legacy SQL dialect for this query. The default value is
true. If set to false, the query will use BigQuery's GoogleSQL:
https://cloud.google.com/bigquery/sql-reference/ When useLegacySql is set to false, the value
of flattenResults is ignored; query will be run as if flattenResults is false.
- Returns:
- value or
null
for none
-
setUseLegacySql
public QueryRequest setUseLegacySql(Boolean useLegacySql)
Specifies whether to use BigQuery's legacy SQL dialect for this query. The default value is
true. If set to false, the query will use BigQuery's GoogleSQL:
https://cloud.google.com/bigquery/sql-reference/ When useLegacySql is set to false, the value
of flattenResults is ignored; query will be run as if flattenResults is false.
- Parameters:
useLegacySql
- useLegacySql or null
for none
-
isUseLegacySql
public boolean isUseLegacySql()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Specifies whether to use BigQuery's legacy SQL dialect for this query. The default value is true.
If set to false, the query will use BigQuery's GoogleSQL: https://cloud.google.com/bigquery/sql-
reference/ When useLegacySql is set to false, the value of flattenResults is ignored; query will be
run as if flattenResults is false.
-
getUseQueryCache
public Boolean getUseQueryCache()
Optional. Whether to look for the result in the query cache. The query cache is a best-effort
cache that will be flushed whenever tables in the query are modified. The default value is
true.
- Returns:
- value or
null
for none
-
setUseQueryCache
public QueryRequest setUseQueryCache(Boolean useQueryCache)
Optional. Whether to look for the result in the query cache. The query cache is a best-effort
cache that will be flushed whenever tables in the query are modified. The default value is
true.
- Parameters:
useQueryCache
- useQueryCache or null
for none
-
isUseQueryCache
public boolean isUseQueryCache()
Convenience method that returns only Boolean.TRUE
or Boolean.FALSE
.
Boolean properties can have four possible values:
null
, Data.NULL_BOOLEAN
, Boolean.TRUE
or Boolean.FALSE
.
This method returns Boolean.TRUE
if the default of the property is Boolean.TRUE
and it is null
or Data.NULL_BOOLEAN
.
Boolean.FALSE
is returned if the default of the property is Boolean.FALSE
and
it is null
or Data.NULL_BOOLEAN
.
Optional. Whether to look for the result in the query cache. The query cache is a best-effort cache
that will be flushed whenever tables in the query are modified. The default value is true.
-
set
public QueryRequest set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public QueryRequest clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.