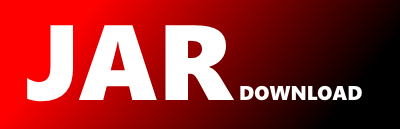
target.apidocs.com.google.api.services.bigquery.model.JobConfiguration.html Maven / Gradle / Ivy
JobConfiguration (BigQuery API v2-rev20240905-2.0.0)
com.google.api.services.bigquery.model
Class JobConfiguration
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.bigquery.model.JobConfiguration
-
public final class JobConfiguration
extends com.google.api.client.json.GenericJson
Model definition for JobConfiguration.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the BigQuery API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
JobConfiguration()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
JobConfiguration
clone()
JobConfigurationTableCopy
getCopy()
[Pick one] Copies a table.
Boolean
getDryRun()
Optional.
JobConfigurationExtract
getExtract()
[Pick one] Configures an extract job.
Long
getJobTimeoutMs()
Optional.
String
getJobType()
Output only.
Map<String,String>
getLabels()
The labels associated with this job.
JobConfigurationLoad
getLoad()
[Pick one] Configures a load job.
JobConfigurationQuery
getQuery()
[Pick one] Configures a query job.
JobConfiguration
set(String fieldName,
Object value)
JobConfiguration
setCopy(JobConfigurationTableCopy copy)
[Pick one] Copies a table.
JobConfiguration
setDryRun(Boolean dryRun)
Optional.
JobConfiguration
setExtract(JobConfigurationExtract extract)
[Pick one] Configures an extract job.
JobConfiguration
setJobTimeoutMs(Long jobTimeoutMs)
Optional.
JobConfiguration
setJobType(String jobType)
Output only.
JobConfiguration
setLabels(Map<String,String> labels)
The labels associated with this job.
JobConfiguration
setLoad(JobConfigurationLoad load)
[Pick one] Configures a load job.
JobConfiguration
setQuery(JobConfigurationQuery query)
[Pick one] Configures a query job.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getCopy
public JobConfigurationTableCopy getCopy()
[Pick one] Copies a table.
- Returns:
- value or
null
for none
-
setCopy
public JobConfiguration setCopy(JobConfigurationTableCopy copy)
[Pick one] Copies a table.
- Parameters:
copy
- copy or null
for none
-
getDryRun
public Boolean getDryRun()
Optional. If set, don't actually run this job. A valid query will return a mostly empty
response with some processing statistics, while an invalid query will return the same error it
would if it wasn't a dry run. Behavior of non-query jobs is undefined.
- Returns:
- value or
null
for none
-
setDryRun
public JobConfiguration setDryRun(Boolean dryRun)
Optional. If set, don't actually run this job. A valid query will return a mostly empty
response with some processing statistics, while an invalid query will return the same error it
would if it wasn't a dry run. Behavior of non-query jobs is undefined.
- Parameters:
dryRun
- dryRun or null
for none
-
getExtract
public JobConfigurationExtract getExtract()
[Pick one] Configures an extract job.
- Returns:
- value or
null
for none
-
setExtract
public JobConfiguration setExtract(JobConfigurationExtract extract)
[Pick one] Configures an extract job.
- Parameters:
extract
- extract or null
for none
-
getJobTimeoutMs
public Long getJobTimeoutMs()
Optional. Job timeout in milliseconds. If this time limit is exceeded, BigQuery will attempt to
stop a longer job, but may not always succeed in canceling it before the job completes. For
example, a job that takes more than 60 seconds to complete has a better chance of being stopped
than a job that takes 10 seconds to complete.
- Returns:
- value or
null
for none
-
setJobTimeoutMs
public JobConfiguration setJobTimeoutMs(Long jobTimeoutMs)
Optional. Job timeout in milliseconds. If this time limit is exceeded, BigQuery will attempt to
stop a longer job, but may not always succeed in canceling it before the job completes. For
example, a job that takes more than 60 seconds to complete has a better chance of being stopped
than a job that takes 10 seconds to complete.
- Parameters:
jobTimeoutMs
- jobTimeoutMs or null
for none
-
getJobType
public String getJobType()
Output only. The type of the job. Can be QUERY, LOAD, EXTRACT, COPY or UNKNOWN.
- Returns:
- value or
null
for none
-
setJobType
public JobConfiguration setJobType(String jobType)
Output only. The type of the job. Can be QUERY, LOAD, EXTRACT, COPY or UNKNOWN.
- Parameters:
jobType
- jobType or null
for none
-
getLabels
public Map<String,String> getLabels()
The labels associated with this job. You can use these to organize and group your jobs. Label
keys and values can be no longer than 63 characters, can only contain lowercase letters,
numeric characters, underscores and dashes. International characters are allowed. Label values
are optional. Label keys must start with a letter and each label in the list must have a
different key.
- Returns:
- value or
null
for none
-
setLabels
public JobConfiguration setLabels(Map<String,String> labels)
The labels associated with this job. You can use these to organize and group your jobs. Label
keys and values can be no longer than 63 characters, can only contain lowercase letters,
numeric characters, underscores and dashes. International characters are allowed. Label values
are optional. Label keys must start with a letter and each label in the list must have a
different key.
- Parameters:
labels
- labels or null
for none
-
getLoad
public JobConfigurationLoad getLoad()
[Pick one] Configures a load job.
- Returns:
- value or
null
for none
-
setLoad
public JobConfiguration setLoad(JobConfigurationLoad load)
[Pick one] Configures a load job.
- Parameters:
load
- load or null
for none
-
getQuery
public JobConfigurationQuery getQuery()
[Pick one] Configures a query job.
- Returns:
- value or
null
for none
-
setQuery
public JobConfiguration setQuery(JobConfigurationQuery query)
[Pick one] Configures a query job.
- Parameters:
query
- query or null
for none
-
set
public JobConfiguration set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public JobConfiguration clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy